key
stringclasses 5
values | id
stringlengths 13
150
| text
stringlengths 68
54.2k
| filetype
stringclasses 3
values |
---|---|---|---|
llms.txt | Docs: FastHTML quick start | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<docs><doc title="FastHTML quick start" desc="A brief overview of many FastHTML features"># Web Devs Quickstart
## Installation
``` bash
pip install python-fasthtml
```
## A Minimal Application
A minimal FastHTML application looks something like this:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/")
def get():
return Titled("FastHTML", P("Let's do this!"))
serve()
```
</div>
Line 1
We import what we need for rapid development! A carefully-curated set of
FastHTML functions and other Python objects is brought into our global
namespace for convenience.
Line 3
We instantiate a FastHTML app with the `fast_app()` utility function.
This provides a number of really useful defaults that we’ll take
advantage of later in the tutorial.
Line 5
We use the `rt()` decorator to tell FastHTML what to return when a user
visits `/` in their browser.
Line 6
We connect this route to HTTP GET requests by defining a view function
called `get()`.
Line 7
A tree of Python function calls that return all the HTML required to
write a properly formed web page. You’ll soon see the power of this
approach.
Line 9
The `serve()` utility configures and runs FastHTML using a library
called `uvicorn`.
Run the code:
``` bash
python main.py
```
The terminal will look like this:
``` bash
INFO: Uvicorn running on http://0.0.0.0:5001 (Press CTRL+C to quit)
INFO: Started reloader process [58058] using WatchFiles
INFO: Started server process [58060]
INFO: Waiting for application startup.
INFO: Application startup complete.
```
Confirm FastHTML is running by opening your web browser to
[127.0.0.1:5001](http://127.0.0.1:5001). You should see something like
the image below:

<div>
> **Note**
>
> While some linters and developers will complain about the wildcard
> import, it is by design here and perfectly safe. FastHTML is very
> deliberate about the objects it exports in `fasthtml.common`. If it
> bothers you, you can import the objects you need individually, though
> it will make the code more verbose and less readable.
>
> If you want to learn more about how FastHTML handles imports, we cover
> that [here](https://docs.fastht.ml/explains/faq.html#why-use-import).
</div>
## A Minimal Charting Application
The
[`Script`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#script)
function allows you to include JavaScript. You can use Python to
generate parts of your JS or JSON like this:
``` python
import json
from fasthtml.common import *
app, rt = fast_app(hdrs=(Script(src="https://cdn.plot.ly/plotly-2.32.0.min.js"),))
data = json.dumps({
"data": [{"x": [1, 2, 3, 4],"type": "scatter"},
{"x": [1, 2, 3, 4],"y": [16, 5, 11, 9],"type": "scatter"}],
"title": "Plotly chart in FastHTML ",
"description": "This is a demo dashboard",
"type": "scatter"
})
@rt("/")
def get():
return Titled("Chart Demo", Div(id="myDiv"),
Script(f"var data = {data}; Plotly.newPlot('myDiv', data);"))
serve()
```
## Debug Mode
When we can’t figure out a bug in FastHTML, we can run it in `DEBUG`
mode. When an error is thrown, the error screen is displayed in the
browser. This error setting should never be used in a deployed app.
``` python
from fasthtml.common import *
app, rt = fast_app(debug=True)
@rt("/")
def get():
1/0
return Titled("FastHTML Error!", P("Let's error!"))
serve()
```
Line 3
`debug=True` sets debug mode on.
Line 7
Python throws an error when it tries to divide an integer by zero.
## Routing
FastHTML builds upon FastAPI’s friendly decorator pattern for specifying
URLs, with extra features:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/")
def get():
return Titled("FastHTML", P("Let's do this!"))
@rt("/hello")
def get():
return Titled("Hello, world!")
serve()
```
</div>
Line 5
The “/” URL on line 5 is the home of a project. This would be accessed
at [127.0.0.1:5001](http://127.0.0.1:5001).
Line 9
“/hello” URL on line 9 will be found by the project if the user visits
[127.0.0.1:5001/hello](http://127.0.0.1:5001/hello).
<div>
> **Tip**
>
> It looks like `get()` is being defined twice, but that’s not the case.
> Each function decorated with `rt` is totally separate, and is injected
> into the router. We’re not calling them in the module’s namespace
> (`locals()`). Rather, we’re loading them into the routing mechanism
> using the `rt` decorator.
</div>
You can do more! Read on to learn what we can do to make parts of the
URL dynamic.
## Variables in URLs
You can add variable sections to a URL by marking them with
`{variable_name}`. Your function then receives the `{variable_name}` as
a keyword argument, but only if it is the correct type. Here’s an
example:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/{name}/{age}")
def get(name: str, age: int):
return Titled(f"Hello {name.title()}, age {age}")
serve()
```
</div>
Line 5
We specify two variable names, `name` and `age`.
Line 6
We define two function arguments named identically to the variables. You
will note that we specify the Python types to be passed.
Line 7
We use these functions in our project.
Try it out by going to this address:
[127.0.0.1:5001/uma/5](http://127.0.0.1:5001/uma/5). You should get a
page that says,
> “Hello Uma, age 5”.
### What happens if we enter incorrect data?
The [127.0.0.1:5001/uma/5](http://127.0.0.1:5001/uma/5) URL works
because `5` is an integer. If we enter something that is not, such as
[127.0.0.1:5001/uma/five](http://127.0.0.1:5001/uma/five), then FastHTML
will return an error instead of a web page.
<div>
> **FastHTML URL routing supports more complex types**
>
> The two examples we provide here use Python’s built-in `str` and `int`
> types, but you can use your own types, including more complex ones
> such as those defined by libraries like
> [attrs](https://pypi.org/project/attrs/),
> [pydantic](https://pypi.org/project/pydantic/), and even
> [sqlmodel](https://pypi.org/project/sqlmodel/).
</div>
## HTTP Methods
FastHTML matches function names to HTTP methods. So far the URL routes
we’ve defined have been for HTTP GET methods, the most common method for
web pages.
Form submissions often are sent as HTTP POST. When dealing with more
dynamic web page designs, also known as Single Page Apps (SPA for
short), the need can arise for other methods such as HTTP PUT and HTTP
DELETE. The way FastHTML handles this is by changing the function name.
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/")
def get():
return Titled("HTTP GET", P("Handle GET"))
@rt("/")
def post():
return Titled("HTTP POST", P("Handle POST"))
serve()
```
</div>
Line 6
On line 6 because the `get()` function name is used, this will handle
HTTP GETs going to the `/` URI.
Line 10
On line 10 because the `post()` function name is used, this will handle
HTTP POSTs going to the `/` URI.
## CSS Files and Inline Styles
Here we modify default headers to demonstrate how to use the [Sakura CSS
microframework](https://github.com/oxalorg/sakura) instead of FastHTML’s
default of Pico CSS.
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app(
pico=False,
hdrs=(
Link(rel='stylesheet', href='assets/normalize.min.css', type='text/css'),
Link(rel='stylesheet', href='assets/sakura.css', type='text/css'),
Style("p {color: red;}")
))
@app.get("/")
def home():
return Titled("FastHTML",
P("Let's do this!"),
)
serve()
```
</div>
Line 4
By setting `pico` to `False`, FastHTML will not include `pico.min.css`.
Line 7
This will generate an HTML `<link>` tag for sourcing the css for Sakura.
Line 8
If you want an inline styles, the `Style()` function will put the result
into the HTML.
## Other Static Media File Locations
As you saw,
[`Script`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#script)
and `Link` are specific to the most common static media use cases in web
apps: including JavaScript, CSS, and images. But it also works with
videos and other static media files. The default behavior is to look for
these files in the root directory - typically we don’t do anything
special to include them. We can change the default directory that is
looked in for files by adding the `static_path` parameter to the
[`fast_app`](https://AnswerDotAI.github.io/fasthtml/api/fastapp.html#fast_app)
function.
``` python
app, rt = fast_app(static_path='public')
```
FastHTML also allows us to define a route that uses `FileResponse` to
serve the file at a specified path. This is useful for serving images,
videos, and other media files from a different directory without having
to change the paths of many files. So if we move the directory
containing the media files, we only need to change the path in one
place. In the example below, we call images from a directory called
`public`.
``` python
@rt("/{fname:path}.{ext:static}")
async def get(fname:str, ext:str):
return FileResponse(f'public/{fname}.{ext}')
```
## Rendering Markdown
``` python
from fasthtml.common import *
hdrs = (MarkdownJS(), HighlightJS(langs=['python', 'javascript', 'html', 'css']), )
app, rt = fast_app(hdrs=hdrs)
content = """
Here are some _markdown_ elements.
- This is a list item
- This is another list item
- And this is a third list item
**Fenced code blocks work here.**
"""
@rt('/')
def get(req):
return Titled("Markdown rendering example", Div(content,cls="marked"))
serve()
```
## Code highlighting
Here’s how to highlight code without any markdown configuration.
``` python
from fasthtml.common import *
# Add the HighlightJS built-in header
hdrs = (HighlightJS(langs=['python', 'javascript', 'html', 'css']),)
app, rt = fast_app(hdrs=hdrs)
code_example = """
import datetime
import time
for i in range(10):
print(f"{datetime.datetime.now()}")
time.sleep(1)
"""
@rt('/')
def get(req):
return Titled("Markdown rendering example",
Div(
# The code example needs to be surrounded by
# Pre & Code elements
Pre(Code(code_example))
))
serve()
```
## Defining new `ft` components
We can build our own `ft` components and combine them with other
components. The simplest method is defining them as a function.
``` python
from fasthtml.common import *
```
``` python
def hero(title, statement):
return Div(H1(title),P(statement), cls="hero")
# usage example
Main(
hero("Hello World", "This is a hero statement")
)
```
``` html
<main>
<div class="hero">
<h1>Hello World</h1>
<p>This is a hero statement</p>
</div>
</main>
```
### Pass through components
For when we need to define a new component that allows zero-to-many
components to be nested within them, we lean on Python’s `*args` and
`**kwargs` mechanism. Useful for creating page layout controls.
``` python
def layout(*args, **kwargs):
"""Dashboard layout for all our dashboard views"""
return Main(
H1("Dashboard"),
Div(*args, **kwargs),
cls="dashboard",
)
# usage example
layout(
Ul(*[Li(o) for o in range(3)]),
P("Some content", cls="description"),
)
```
``` html
<main class="dashboard">
<h1>Dashboard</h1>
<div>
<ul>
<li>0</li>
<li>1</li>
<li>2</li>
</ul>
<p class="description">Some content</p>
</div>
</main>
```
### Dataclasses as ft components
While functions are easy to read, for more complex components some might
find it easier to use a dataclass.
``` python
from dataclasses import dataclass
@dataclass
class Hero:
title: str
statement: str
def __ft__(self):
""" The __ft__ method renders the dataclass at runtime."""
return Div(H1(self.title),P(self.statement), cls="hero")
# usage example
Main(
Hero("Hello World", "This is a hero statement")
)
```
``` html
<main>
<div class="hero">
<h1>Hello World</h1>
<p>This is a hero statement</p>
</div>
</main>
```
## Testing views in notebooks
Because of the ASGI event loop it is currently impossible to run
FastHTML inside a notebook. However, we can still test the output of our
views. To do this, we leverage Starlette, an ASGI toolkit that FastHTML
uses.
``` python
# First we instantiate our app, in this case we remove the
# default headers to reduce the size of the output.
app, rt = fast_app(default_hdrs=False)
# Setting up the Starlette test client
from starlette.testclient import TestClient
client = TestClient(app)
# Usage example
@rt("/")
def get():
return Titled("FastHTML is awesome",
P("The fastest way to create web apps in Python"))
print(client.get("/").text)
```
<!doctype html>
<html>
<head>
<title>FastHTML is awesome</title>
</head>
<body>
<main class="container">
<h1>FastHTML is awesome</h1>
<p>The fastest way to create web apps in Python</p>
</main>
</body>
</html>
## Forms
To validate data coming from users, first define a dataclass
representing the data you want to check. Here’s an example representing
a signup form.
``` python
from dataclasses import dataclass
@dataclass
class Profile: email:str; phone:str; age:int
```
Create an FT component representing an empty version of that form. Don’t
pass in any value to fill the form, that gets handled later.
``` python
profile_form = Form(method="post", action="/profile")(
Fieldset(
Label('Email', Input(name="email")),
Label("Phone", Input(name="phone")),
Label("Age", Input(name="age")),
),
Button("Save", type="submit"),
)
profile_form
```
``` html
<form enctype="multipart/form-data" method="post" action="/profile">
<fieldset>
<label>
Email
<input name="email">
</label>
<label>
Phone
<input name="phone">
</label>
<label>
Age
<input name="age">
</label>
</fieldset>
<button type="submit">Save</button>
</form>
```
Once the dataclass and form function are completed, we can add data to
the form. To do that, instantiate the profile dataclass:
``` python
profile = Profile(email='[email protected]', phone='123456789', age=5)
profile
```
Profile(email='[email protected]', phone='123456789', age=5)
Then add that data to the `profile_form` using FastHTML’s
[`fill_form`](https://AnswerDotAI.github.io/fasthtml/api/components.html#fill_form)
class:
``` python
fill_form(profile_form, profile)
```
``` html
<form enctype="multipart/form-data" method="post" action="/profile">
<fieldset>
<label>
Email
<input name="email" value="[email protected]">
</label>
<label>
Phone
<input name="phone" value="123456789">
</label>
<label>
Age
<input name="age" value="5">
</label>
</fieldset>
<button type="submit">Save</button>
</form>
```
### Forms with views
The usefulness of FastHTML forms becomes more apparent when they are
combined with FastHTML views. We’ll show how this works by using the
test client from above. First, let’s create a SQlite database:
``` python
db = Database("profiles.db")
profiles = db.create(Profile, pk="email")
```
Now we insert a record into the database:
``` python
profiles.insert(profile)
```
Profile(email='[email protected]', phone='123456789', age=5)
And we can then demonstrate in the code that form is filled and
displayed to the user.
``` python
@rt("/profile/{email}")
def profile(email:str):
profile = profiles[email]
filled_profile_form = fill_form(profile_form, profile)
return Titled(f'Profile for {profile.email}', filled_profile_form)
print(client.get(f"/profile/[email protected]").text)
```
Line 3
Fetch the profile using the profile table’s `email` primary key
Line 4
Fill the form for display.
<!doctype html>
<html>
<head>
<title>Profile for [email protected]</title>
</head>
<body>
<main class="container">
<h1>Profile for [email protected]</h1>
<form enctype="multipart/form-data" method="post" action="/profile">
<fieldset>
<label>
Email
<input name="email" value="[email protected]">
</label>
<label>
Phone
<input name="phone" value="123456789">
</label>
<label>
Age
<input name="age" value="5">
</label>
</fieldset>
<button type="submit">Save</button>
</form>
</main>
</body>
</html>
And now let’s demonstrate making a change to the data.
``` python
@rt("/profile")
def post(profile: Profile):
profiles.update(profile)
return RedirectResponse(url=f"/profile/{profile.email}")
new_data = dict(email='[email protected]', phone='7654321', age=25)
print(client.post("/profile", data=new_data).text)
```
Line 2
We use the `Profile` dataclass definition to set the type for the
incoming `profile` content. This validates the field types for the
incoming data
Line 3
Taking our validated data, we updated the profiles table
Line 4
We redirect the user back to their profile view
Line 7
The display is of the profile form view showing the changes in data.
<!doctype html>
<html>
<head>
<title>Profile for [email protected]</title>
</head>
<body>
<main class="container">
<h1>Profile for [email protected]</h1>
<form enctype="multipart/form-data" method="post" action="/profile">
<fieldset>
<label>
Email
<input name="email" value="[email protected]">
</label>
<label>
Phone
<input name="phone" value="7654321">
</label>
<label>
Age
<input name="age" value="25">
</label>
</fieldset>
<button type="submit">Save</button>
</form>
</main>
</body>
</html>
## Strings and conversion order
The general rules for rendering are: - `__ft__` method will be called
(for default components like `P`, `H2`, etc. or if you define your own
components) - If you pass a string, it will be escaped - On other python
objects, `str()` will be called
As a consequence, if you want to include plain HTML tags directly into
e.g. a `Div()` they will get escaped by default (as a security measure
to avoid code injections). This can be avoided by using `NotStr()`, a
convenient way to reuse python code that returns already HTML. If you
use pandas, you can use `pandas.DataFrame.to_html()` to get a nice
table. To include the output a FastHTML, wrap it in `NotStr()`, like
`Div(NotStr(df.to_html()))`.
Above we saw how a dataclass behaves with the `__ft__` method defined.
On a plain dataclass, `str()` will be called (but not escaped).
``` python
from dataclasses import dataclass
@dataclass
class Hero:
title: str
statement: str
# rendering the dataclass with the default method
Main(
Hero("<h1>Hello World</h1>", "This is a hero statement")
)
```
``` html
<main>Hero(title='<h1>Hello World</h1>', statement='This is a hero statement')</main>
```
``` python
# This will display the HTML as text on your page
Div("Let's include some HTML here: <div>Some HTML</div>")
```
``` html
<div>Let's include some HTML here: <div>Some HTML</div></div>
```
``` python
# Keep the string untouched, will be rendered on the page
Div(NotStr("<div><h1>Some HTML</h1></div>"))
```
``` html
<div><div><h1>Some HTML</h1></div></div>
```
## Custom exception handlers
FastHTML allows customization of exception handlers, but does so
gracefully. What this means is by default it includes all the `<html>`
tags needed to display attractive content. Try it out!
``` python
from fasthtml.common import *
def not_found(req, exc): return Titled("404: I don't exist!")
exception_handlers = {404: not_found}
app, rt = fast_app(exception_handlers=exception_handlers)
@rt('/')
def get():
return (Titled("Home page", P(A(href="/oops")("Click to generate 404 error"))))
serve()
```
We can also use lambda to make things more terse:
``` python
from fasthtml.common import *
exception_handlers={
404: lambda req, exc: Titled("404: I don't exist!"),
418: lambda req, exc: Titled("418: I'm a teapot!")
}
app, rt = fast_app(exception_handlers=exception_handlers)
@rt('/')
def get():
return (Titled("Home page", P(A(href="/oops")("Click to generate 404 error"))))
serve()
```
## Cookies
We can set cookies using the `cookie()` function. In our example, we’ll
create a `timestamp` cookie.
``` python
from datetime import datetime
from IPython.display import HTML
```
``` python
@rt("/settimestamp")
def get(req):
now = datetime.now()
return P(f'Set to {now}'), cookie('now', datetime.now())
HTML(client.get('/settimestamp').text)
```
<!doctype html>
<html>
<head>
<title>FastHTML page</title>
</head>
<body>
<p>Set to 2024-09-04 18:30:34.896373</p>
</body>
</html>
Now let’s get it back using the same name for our parameter as the
cookie name.
``` python
@rt('/gettimestamp')
def get(now:parsed_date): return f'Cookie was set at time {now.time()}'
client.get('/gettimestamp').text
```
'Cookie was set at time 18:30:34.896405'
## Sessions
For convenience and security, FastHTML has a mechanism for storing small
amounts of data in the user’s browser. We can do this by adding a
`session` argument to routes. FastHTML sessions are Python dictionaries,
and we can leverage to our benefit. The example below shows how to
concisely set and get sessions.
``` python
@rt('/adder/{num}')
def get(session, num: int):
session.setdefault('sum', 0)
session['sum'] = session.get('sum') + num
return Response(f'The sum is {session["sum"]}.')
```
## Toasts (also known as Messages)
Toasts, sometimes called “Messages” are small notifications usually in
colored boxes used to notify users that something has happened. Toasts
can be of four types:
- info
- success
- warning
- error
Examples toasts might include:
- “Payment accepted”
- “Data submitted”
- “Request approved”
Toasts require the use of the `setup_toasts()` function plus every view
needs these two features:
- The session argument
- Must return FT components
``` python
setup_toasts(app)
@rt('/toasting')
def get(session):
# Normally one toast is enough, this allows us to see
# different toast types in action.
add_toast(session, f"Toast is being cooked", "info")
add_toast(session, f"Toast is ready", "success")
add_toast(session, f"Toast is getting a bit crispy", "warning")
add_toast(session, f"Toast is burning!", "error")
return Titled("I like toast")
```
Line 1
`setup_toasts` is a helper function that adds toast dependencies.
Usually this would be declared right after `fast_app()`
Line 4
Toasts require sessions
Line 11
Views with Toasts must return FT components.
## Authentication and authorization
In FastHTML the tasks of authentication and authorization are handled
with Beforeware. Beforeware are functions that run before the route
handler is called. They are useful for global tasks like ensuring users
are authenticated or have permissions to access a view.
First, we write a function that accepts a request and session arguments:
``` python
# Status code 303 is a redirect that can change POST to GET,
# so it's appropriate for a login page.
login_redir = RedirectResponse('/login', status_code=303)
def user_auth_before(req, sess):
# The `auth` key in the request scope is automatically provided
# to any handler which requests it, and can not be injected
# by the user using query params, cookies, etc, so it should
# be secure to use.
auth = req.scope['auth'] = sess.get('auth', None)
# If the session key is not there, it redirects to the login page.
if not auth: return login_redir
```
Now we pass our `user_auth_before` function as the first argument into a
[`Beforeware`](https://AnswerDotAI.github.io/fasthtml/api/core.html#beforeware)
class. We also pass a list of regular expressions to the `skip`
argument, designed to allow users to still get to the home and login
pages.
``` python
beforeware = Beforeware(
user_auth_before,
skip=[r'/favicon\.ico', r'/static/.*', r'.*\.css', r'.*\.js', '/login', '/']
)
app, rt = fast_app(before=beforeware)
```
## Server-sent events (SSE)
With [server-sent
events](https://developer.mozilla.org/en-US/docs/Web/API/Server-sent_events),
it’s possible for a server to send new data to a web page at any time,
by pushing messages to the web page. Unlike WebSockets, SSE can only go
in one direction: server to client. SSE is also part of the HTTP
specification unlike WebSockets which uses its own specification.
FastHTML introduces several tools for working with SSE which are covered
in the example below. While concise, there’s a lot going on in this
function so we’ve annotated it quite a bit.
``` python
import random
from asyncio import sleep
from fasthtml.common import *
hdrs=(Script(src="https://unpkg.com/[email protected]/sse.js"),)
app,rt = fast_app(hdrs=hdrs)
@rt
def index():
return Titled("SSE Random Number Generator",
P("Generate pairs of random numbers, as the list grows scroll downwards."),
Div(hx_ext="sse",
sse_connect="/number-stream",
hx_swap="beforeend show:bottom",
sse_swap="message"))
shutdown_event = signal_shutdown()
async def number_generator():
while not shutdown_event.is_set():
data = Article(random.randint(1, 100))
yield sse_message(data)
await sleep(1)
@rt("/number-stream")
async def get(): return EventStream(number_generator())
```
Line 5
Import the HTMX SSE extension
Line 12
Tell HTMX to load the SSE extension
Line 13
Look at the `/number-stream` endpoint for SSE content
Line 14
When new items come in from the SSE endpoint, add them at the end of the
current content within the div. If they go beyond the screen, scroll
downwards
Line 15
Specify the name of the event. FastHTML’s default event name is
“message”. Only change if you have more than one call to SSE endpoints
within a view
Line 17
Set up the asyncio event loop
Line 19
Don’t forget to make this an `async` function!
Line 20
Iterate through the asyncio event loop
Line 22
We yield the data. Data ideally should be comprised of FT components as
that plugs nicely into HTMX in the browser
Line 26
The endpoint view needs to be an async function that returns a
[`EventStream`](https://AnswerDotAI.github.io/fasthtml/api/core.html#eventstream)
<div>
> **New content as of September 1, 2024**
>
> - [Forms](../tutorials/quickstart_for_web_devs.html#forms)
> - [Server-side Events
> (SSE)](../tutorials/quickstart_for_web_devs.html#server-sent-events-sse)
>
> We’re going to be adding more to this document, so check back
> frequently for updates.
</div>
## Unwritten quickstart sections
- Websockets
- Tables</doc></docs></project> | xml |
llms.txt | Docs: HTMX reference | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<docs><doc title="HTMX reference" desc="Brief description of all HTMX attributes, CSS classes, headers, events, extensions, js lib methods, and config options">+++
title = "Reference"
+++
## Contents
* [htmx Core Attributes](#attributes)
* [htmx Additional Attributes](#attributes-additional)
* [htmx CSS Classes](#classes)
* [htmx Request Headers](#request_headers)
* [htmx Response Headers](#response_headers)
* [htmx Events](#events)
* [htmx Extensions](https://extensions.htmx.org)
* [JavaScript API](#api)
* [Configuration Options](#config)
## Core Attribute Reference {#attributes}
The most common attributes when using htmx.
<div class="info-table">
| Attribute | Description |
|--------------------------------------------------|--------------------------------------------------------------------------------------------------------------------|
| [`hx-get`](@/attributes/hx-get.md) | issues a `GET` to the specified URL |
| [`hx-post`](@/attributes/hx-post.md) | issues a `POST` to the specified URL |
| [`hx-on*`](@/attributes/hx-on.md) | handle events with inline scripts on elements |
| [`hx-push-url`](@/attributes/hx-push-url.md) | push a URL into the browser location bar to create history |
| [`hx-select`](@/attributes/hx-select.md) | select content to swap in from a response |
| [`hx-select-oob`](@/attributes/hx-select-oob.md) | select content to swap in from a response, somewhere other than the target (out of band) |
| [`hx-swap`](@/attributes/hx-swap.md) | controls how content will swap in (`outerHTML`, `beforeend`, `afterend`, ...) |
| [`hx-swap-oob`](@/attributes/hx-swap-oob.md) | mark element to swap in from a response (out of band) |
| [`hx-target`](@/attributes/hx-target.md) | specifies the target element to be swapped |
| [`hx-trigger`](@/attributes/hx-trigger.md) | specifies the event that triggers the request |
| [`hx-vals`](@/attributes/hx-vals.md) | add values to submit with the request (JSON format) |
</div>
## Additional Attribute Reference {#attributes-additional}
All other attributes available in htmx.
<div class="info-table">
| Attribute | Description |
|------------------------------------------------------|------------------------------------------------------------------------------------------------------------------------------------|
| [`hx-boost`](@/attributes/hx-boost.md) | add [progressive enhancement](https://en.wikipedia.org/wiki/Progressive_enhancement) for links and forms |
| [`hx-confirm`](@/attributes/hx-confirm.md) | shows a `confirm()` dialog before issuing a request |
| [`hx-delete`](@/attributes/hx-delete.md) | issues a `DELETE` to the specified URL |
| [`hx-disable`](@/attributes/hx-disable.md) | disables htmx processing for the given node and any children nodes |
| [`hx-disabled-elt`](@/attributes/hx-disabled-elt.md) | adds the `disabled` attribute to the specified elements while a request is in flight |
| [`hx-disinherit`](@/attributes/hx-disinherit.md) | control and disable automatic attribute inheritance for child nodes |
| [`hx-encoding`](@/attributes/hx-encoding.md) | changes the request encoding type |
| [`hx-ext`](@/attributes/hx-ext.md) | extensions to use for this element |
| [`hx-headers`](@/attributes/hx-headers.md) | adds to the headers that will be submitted with the request |
| [`hx-history`](@/attributes/hx-history.md) | prevent sensitive data being saved to the history cache |
| [`hx-history-elt`](@/attributes/hx-history-elt.md) | the element to snapshot and restore during history navigation |
| [`hx-include`](@/attributes/hx-include.md) | include additional data in requests |
| [`hx-indicator`](@/attributes/hx-indicator.md) | the element to put the `htmx-request` class on during the request |
| [`hx-inherit`](@/attributes/hx-inherit.md) | control and enable automatic attribute inheritance for child nodes if it has been disabled by default |
| [`hx-params`](@/attributes/hx-params.md) | filters the parameters that will be submitted with a request |
| [`hx-patch`](@/attributes/hx-patch.md) | issues a `PATCH` to the specified URL |
| [`hx-preserve`](@/attributes/hx-preserve.md) | specifies elements to keep unchanged between requests |
| [`hx-prompt`](@/attributes/hx-prompt.md) | shows a `prompt()` before submitting a request |
| [`hx-put`](@/attributes/hx-put.md) | issues a `PUT` to the specified URL |
| [`hx-replace-url`](@/attributes/hx-replace-url.md) | replace the URL in the browser location bar |
| [`hx-request`](@/attributes/hx-request.md) | configures various aspects of the request |
| [`hx-sync`](@/attributes/hx-sync.md) | control how requests made by different elements are synchronized |
| [`hx-validate`](@/attributes/hx-validate.md) | force elements to validate themselves before a request |
| [`hx-vars`](@/attributes/hx-vars.md) | adds values dynamically to the parameters to submit with the request (deprecated, please use [`hx-vals`](@/attributes/hx-vals.md)) |
</div>
## CSS Class Reference {#classes}
<div class="info-table">
| Class | Description |
|-----------|-------------|
| `htmx-added` | Applied to a new piece of content before it is swapped, removed after it is settled.
| `htmx-indicator` | A dynamically generated class that will toggle visible (opacity:1) when a `htmx-request` class is present
| `htmx-request` | Applied to either the element or the element specified with [`hx-indicator`](@/attributes/hx-indicator.md) while a request is ongoing
| `htmx-settling` | Applied to a target after content is swapped, removed after it is settled. The duration can be modified via [`hx-swap`](@/attributes/hx-swap.md).
| `htmx-swapping` | Applied to a target before any content is swapped, removed after it is swapped. The duration can be modified via [`hx-swap`](@/attributes/hx-swap.md).
</div>
## HTTP Header Reference {#headers}
### Request Headers Reference {#request_headers}
<div class="info-table">
| Header | Description |
|--------|-------------|
| `HX-Boosted` | indicates that the request is via an element using [hx-boost](@/attributes/hx-boost.md)
| `HX-Current-URL` | the current URL of the browser
| `HX-History-Restore-Request` | "true" if the request is for history restoration after a miss in the local history cache
| `HX-Prompt` | the user response to an [hx-prompt](@/attributes/hx-prompt.md)
| `HX-Request` | always "true"
| `HX-Target` | the `id` of the target element if it exists
| `HX-Trigger-Name` | the `name` of the triggered element if it exists
| `HX-Trigger` | the `id` of the triggered element if it exists
</div>
### Response Headers Reference {#response_headers}
<div class="info-table">
| Header | Description |
|------------------------------------------------------|-------------|
| [`HX-Location`](@/headers/hx-location.md) | allows you to do a client-side redirect that does not do a full page reload
| [`HX-Push-Url`](@/headers/hx-push-url.md) | pushes a new url into the history stack
| [`HX-Redirect`](@/headers/hx-redirect.md) | can be used to do a client-side redirect to a new location
| `HX-Refresh` | if set to "true" the client-side will do a full refresh of the page
| [`HX-Replace-Url`](@/headers/hx-replace-url.md) | replaces the current URL in the location bar
| `HX-Reswap` | allows you to specify how the response will be swapped. See [hx-swap](@/attributes/hx-swap.md) for possible values
| `HX-Retarget` | a CSS selector that updates the target of the content update to a different element on the page
| `HX-Reselect` | a CSS selector that allows you to choose which part of the response is used to be swapped in. Overrides an existing [`hx-select`](@/attributes/hx-select.md) on the triggering element
| [`HX-Trigger`](@/headers/hx-trigger.md) | allows you to trigger client-side events
| [`HX-Trigger-After-Settle`](@/headers/hx-trigger.md) | allows you to trigger client-side events after the settle step
| [`HX-Trigger-After-Swap`](@/headers/hx-trigger.md) | allows you to trigger client-side events after the swap step
</div>
## Event Reference {#events}
<div class="info-table">
| Event | Description |
|-------|-------------|
| [`htmx:abort`](@/events.md#htmx:abort) | send this event to an element to abort a request
| [`htmx:afterOnLoad`](@/events.md#htmx:afterOnLoad) | triggered after an AJAX request has completed processing a successful response
| [`htmx:afterProcessNode`](@/events.md#htmx:afterProcessNode) | triggered after htmx has initialized a node
| [`htmx:afterRequest`](@/events.md#htmx:afterRequest) | triggered after an AJAX request has completed
| [`htmx:afterSettle`](@/events.md#htmx:afterSettle) | triggered after the DOM has settled
| [`htmx:afterSwap`](@/events.md#htmx:afterSwap) | triggered after new content has been swapped in
| [`htmx:beforeCleanupElement`](@/events.md#htmx:beforeCleanupElement) | triggered before htmx [disables](@/attributes/hx-disable.md) an element or removes it from the DOM
| [`htmx:beforeOnLoad`](@/events.md#htmx:beforeOnLoad) | triggered before any response processing occurs
| [`htmx:beforeProcessNode`](@/events.md#htmx:beforeProcessNode) | triggered before htmx initializes a node
| [`htmx:beforeRequest`](@/events.md#htmx:beforeRequest) | triggered before an AJAX request is made
| [`htmx:beforeSwap`](@/events.md#htmx:beforeSwap) | triggered before a swap is done, allows you to configure the swap
| [`htmx:beforeSend`](@/events.md#htmx:beforeSend) | triggered just before an ajax request is sent
| [`htmx:configRequest`](@/events.md#htmx:configRequest) | triggered before the request, allows you to customize parameters, headers
| [`htmx:confirm`](@/events.md#htmx:confirm) | triggered after a trigger occurs on an element, allows you to cancel (or delay) issuing the AJAX request
| [`htmx:historyCacheError`](@/events.md#htmx:historyCacheError) | triggered on an error during cache writing
| [`htmx:historyCacheMiss`](@/events.md#htmx:historyCacheMiss) | triggered on a cache miss in the history subsystem
| [`htmx:historyCacheMissError`](@/events.md#htmx:historyCacheMissError) | triggered on a unsuccessful remote retrieval
| [`htmx:historyCacheMissLoad`](@/events.md#htmx:historyCacheMissLoad) | triggered on a successful remote retrieval
| [`htmx:historyRestore`](@/events.md#htmx:historyRestore) | triggered when htmx handles a history restoration action
| [`htmx:beforeHistorySave`](@/events.md#htmx:beforeHistorySave) | triggered before content is saved to the history cache
| [`htmx:load`](@/events.md#htmx:load) | triggered when new content is added to the DOM
| [`htmx:noSSESourceError`](@/events.md#htmx:noSSESourceError) | triggered when an element refers to a SSE event in its trigger, but no parent SSE source has been defined
| [`htmx:onLoadError`](@/events.md#htmx:onLoadError) | triggered when an exception occurs during the onLoad handling in htmx
| [`htmx:oobAfterSwap`](@/events.md#htmx:oobAfterSwap) | triggered after an out of band element as been swapped in
| [`htmx:oobBeforeSwap`](@/events.md#htmx:oobBeforeSwap) | triggered before an out of band element swap is done, allows you to configure the swap
| [`htmx:oobErrorNoTarget`](@/events.md#htmx:oobErrorNoTarget) | triggered when an out of band element does not have a matching ID in the current DOM
| [`htmx:prompt`](@/events.md#htmx:prompt) | triggered after a prompt is shown
| [`htmx:pushedIntoHistory`](@/events.md#htmx:pushedIntoHistory) | triggered after an url is pushed into history
| [`htmx:responseError`](@/events.md#htmx:responseError) | triggered when an HTTP response error (non-`200` or `300` response code) occurs
| [`htmx:sendError`](@/events.md#htmx:sendError) | triggered when a network error prevents an HTTP request from happening
| [`htmx:sseError`](@/events.md#htmx:sseError) | triggered when an error occurs with a SSE source
| [`htmx:sseOpen`](/events#htmx:sseOpen) | triggered when a SSE source is opened
| [`htmx:swapError`](@/events.md#htmx:swapError) | triggered when an error occurs during the swap phase
| [`htmx:targetError`](@/events.md#htmx:targetError) | triggered when an invalid target is specified
| [`htmx:timeout`](@/events.md#htmx:timeout) | triggered when a request timeout occurs
| [`htmx:validation:validate`](@/events.md#htmx:validation:validate) | triggered before an element is validated
| [`htmx:validation:failed`](@/events.md#htmx:validation:failed) | triggered when an element fails validation
| [`htmx:validation:halted`](@/events.md#htmx:validation:halted) | triggered when a request is halted due to validation errors
| [`htmx:xhr:abort`](@/events.md#htmx:xhr:abort) | triggered when an ajax request aborts
| [`htmx:xhr:loadend`](@/events.md#htmx:xhr:loadend) | triggered when an ajax request ends
| [`htmx:xhr:loadstart`](@/events.md#htmx:xhr:loadstart) | triggered when an ajax request starts
| [`htmx:xhr:progress`](@/events.md#htmx:xhr:progress) | triggered periodically during an ajax request that supports progress events
</div>
## JavaScript API Reference {#api}
<div class="info-table">
| Method | Description |
|-------|-------------|
| [`htmx.addClass()`](@/api.md#addClass) | Adds a class to the given element
| [`htmx.ajax()`](@/api.md#ajax) | Issues an htmx-style ajax request
| [`htmx.closest()`](@/api.md#closest) | Finds the closest parent to the given element matching the selector
| [`htmx.config`](@/api.md#config) | A property that holds the current htmx config object
| [`htmx.createEventSource`](@/api.md#createEventSource) | A property holding the function to create SSE EventSource objects for htmx
| [`htmx.createWebSocket`](@/api.md#createWebSocket) | A property holding the function to create WebSocket objects for htmx
| [`htmx.defineExtension()`](@/api.md#defineExtension) | Defines an htmx [extension](https://extensions.htmx.org)
| [`htmx.find()`](@/api.md#find) | Finds a single element matching the selector
| [`htmx.findAll()` `htmx.findAll(elt, selector)`](@/api.md#find) | Finds all elements matching a given selector
| [`htmx.logAll()`](@/api.md#logAll) | Installs a logger that will log all htmx events
| [`htmx.logger`](@/api.md#logger) | A property set to the current logger (default is `null`)
| [`htmx.off()`](@/api.md#off) | Removes an event listener from the given element
| [`htmx.on()`](@/api.md#on) | Creates an event listener on the given element, returning it
| [`htmx.onLoad()`](@/api.md#onLoad) | Adds a callback handler for the `htmx:load` event
| [`htmx.parseInterval()`](@/api.md#parseInterval) | Parses an interval declaration into a millisecond value
| [`htmx.process()`](@/api.md#process) | Processes the given element and its children, hooking up any htmx behavior
| [`htmx.remove()`](@/api.md#remove) | Removes the given element
| [`htmx.removeClass()`](@/api.md#removeClass) | Removes a class from the given element
| [`htmx.removeExtension()`](@/api.md#removeExtension) | Removes an htmx [extension](https://extensions.htmx.org)
| [`htmx.swap()`](@/api.md#swap) | Performs swapping (and settling) of HTML content
| [`htmx.takeClass()`](@/api.md#takeClass) | Takes a class from other elements for the given element
| [`htmx.toggleClass()`](@/api.md#toggleClass) | Toggles a class from the given element
| [`htmx.trigger()`](@/api.md#trigger) | Triggers an event on an element
| [`htmx.values()`](@/api.md#values) | Returns the input values associated with the given element
</div>
## Configuration Reference {#config}
Htmx has some configuration options that can be accessed either programmatically or declaratively. They are
listed below:
<div class="info-table">
| Config Variable | Info |
|---------------------------------------|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| `htmx.config.historyEnabled` | defaults to `true`, really only useful for testing |
| `htmx.config.historyCacheSize` | defaults to 10 |
| `htmx.config.refreshOnHistoryMiss` | defaults to `false`, if set to `true` htmx will issue a full page refresh on history misses rather than use an AJAX request |
| `htmx.config.defaultSwapStyle` | defaults to `innerHTML` |
| `htmx.config.defaultSwapDelay` | defaults to 0 |
| `htmx.config.defaultSettleDelay` | defaults to 20 |
| `htmx.config.includeIndicatorStyles` | defaults to `true` (determines if the indicator styles are loaded) |
| `htmx.config.indicatorClass` | defaults to `htmx-indicator` |
| `htmx.config.requestClass` | defaults to `htmx-request` |
| `htmx.config.addedClass` | defaults to `htmx-added` |
| `htmx.config.settlingClass` | defaults to `htmx-settling` |
| `htmx.config.swappingClass` | defaults to `htmx-swapping` |
| `htmx.config.allowEval` | defaults to `true`, can be used to disable htmx's use of eval for certain features (e.g. trigger filters) |
| `htmx.config.allowScriptTags` | defaults to `true`, determines if htmx will process script tags found in new content |
| `htmx.config.inlineScriptNonce` | defaults to `''`, meaning that no nonce will be added to inline scripts |
| `htmx.config.inlineStyleNonce` | defaults to `''`, meaning that no nonce will be added to inline styles |
| `htmx.config.attributesToSettle` | defaults to `["class", "style", "width", "height"]`, the attributes to settle during the settling phase |
| `htmx.config.wsReconnectDelay` | defaults to `full-jitter` |
| `htmx.config.wsBinaryType` | defaults to `blob`, the [the type of binary data](https://developer.mozilla.org/docs/Web/API/WebSocket/binaryType) being received over the WebSocket connection |
| `htmx.config.disableSelector` | defaults to `[hx-disable], [data-hx-disable]`, htmx will not process elements with this attribute on it or a parent |
| `htmx.config.withCredentials` | defaults to `false`, allow cross-site Access-Control requests using credentials such as cookies, authorization headers or TLS client certificates |
| `htmx.config.timeout` | defaults to 0, the number of milliseconds a request can take before automatically being terminated |
| `htmx.config.scrollBehavior` | defaults to 'instant', the behavior for a boosted link on page transitions. The allowed values are `auto`, `instant` and `smooth`. Instant will scroll instantly in a single jump, smooth will scroll smoothly, while auto will behave like a vanilla link. |
| `htmx.config.defaultFocusScroll` | if the focused element should be scrolled into view, defaults to false and can be overridden using the [focus-scroll](@/attributes/hx-swap.md#focus-scroll) swap modifier. |
| `htmx.config.getCacheBusterParam` | defaults to false, if set to true htmx will append the target element to the `GET` request in the format `org.htmx.cache-buster=targetElementId` |
| `htmx.config.globalViewTransitions` | if set to `true`, htmx will use the [View Transition](https://developer.mozilla.org/en-US/docs/Web/API/View_Transitions_API) API when swapping in new content. |
| `htmx.config.methodsThatUseUrlParams` | defaults to `["get", "delete"]`, htmx will format requests with these methods by encoding their parameters in the URL, not the request body |
| `htmx.config.selfRequestsOnly` | defaults to `true`, whether to only allow AJAX requests to the same domain as the current document |
| `htmx.config.ignoreTitle` | defaults to `false`, if set to `true` htmx will not update the title of the document when a `title` tag is found in new content |
| `htmx.config.scrollIntoViewOnBoost` | defaults to `true`, whether or not the target of a boosted element is scrolled into the viewport. If `hx-target` is omitted on a boosted element, the target defaults to `body`, causing the page to scroll to the top. |
| `htmx.config.triggerSpecsCache` | defaults to `null`, the cache to store evaluated trigger specifications into, improving parsing performance at the cost of more memory usage. You may define a simple object to use a never-clearing cache, or implement your own system using a [proxy object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Proxy) |
| `htmx.config.responseHandling` | the default [Response Handling](@/docs.md#response-handling) behavior for response status codes can be configured here to either swap or error |
| `htmx.config.allowNestedOobSwaps` | defaults to `true`, whether to process OOB swaps on elements that are nested within the main response element. See [Nested OOB Swaps](@/attributes/hx-swap-oob.md#nested-oob-swaps). |
</div>
You can set them directly in javascript, or you can use a `meta` tag:
```html
<meta name="htmx-config" content='{"defaultSwapStyle":"outerHTML"}'>
```</doc></docs></project> | xml |
llms.txt | Docs: Surreal | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<docs><doc title="Surreal" desc="Tiny jQuery alternative for plain Javascript with inline Locality of Behavior, providing `me` and `any` functions"># 🗿 Surreal
### Tiny jQuery alternative for plain Javascript with inline [Locality of Behavior](https://htmx.org/essays/locality-of-behaviour/)!
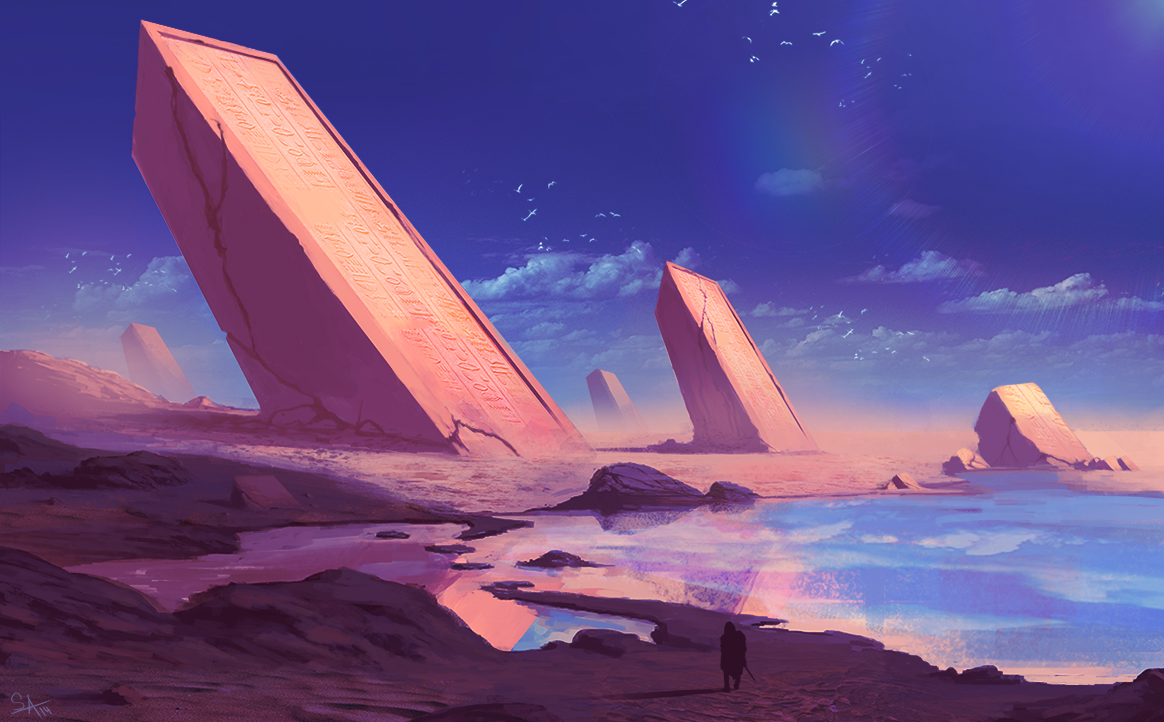
(Art by [shahabalizadeh](https://www.deviantart.com/shahabalizadeh))
<!--
<a href="https://github.com/gnat/surreal/archive/refs/heads/main.zip"><img src="https://img.shields.io/badge/Download%20.zip-ff9800?style=for-the-badge&color=%234400e5" alt="Download badge" /></a>
<a href="https://github.com/gnat/surreal"><img src="https://img.shields.io/github/workflow/status/gnat/surreal/ci?label=ci&style=for-the-badge&color=%237d91ce" alt="CI build badge" /></a>
<a href="https://github.com/gnat/surreal/releases"><img src="https://img.shields.io/github/workflow/status/gnat/surreal/release?label=Mini&style=for-the-badge&color=%237d91ce" alt="Mini build badge" /></a>
<a href="https://github.com/gnat/surreal/blob/main/LICENSE"><img src="https://img.shields.io/github/license/gnat/surreal?style=for-the-badge&color=%234400e5" alt="License badge" /></a>-->
## Why does this exist?
For devs who love ergonomics! You may appreciate Surreal if:
* You want to stay as close as possible to Vanilla JS.
* Hate typing `document.querySelector` over.. and over..
* Hate typing `addEventListener` over.. and over..
* Really wish `document.querySelectorAll` had Array functions..
* Really wish `this` would work in any inline `<script>` tag
* Enjoyed using jQuery selector syntax.
* [Animations, timelines, tweens](#-quick-start) with no extra libraries.
* Only 320 lines. No build step. No dependencies.
* Pairs well with [htmx](https://htmx.org)
* Want fewer layers, less complexity. Are aware of the cargo cult. ✈️
## ✨ What does it add to Javascript?
* ⚡️ [Locality of Behavior (LoB)](https://htmx.org/essays/locality-of-behaviour/) Use `me()` inside `<script>`
* No **.class** or **#id** needed! Get an element without creating a unique name.
* `this` but much more flexible!
* Want `me` in your CSS `<style>` tags, too? See our [companion script](https://github.com/gnat/css-scope-inline)
* 🔗 Call chaining, jQuery style.
* ♻️ Functions work seamlessly on 1 element or arrays of elements!
* All functions can use: `me()`, `any()`, `NodeList`, `HTMLElement` (..or arrays of these!)
* Get 1 element: `me()`
* ..or many elements: `any()`
* `me()` or `any()` can chain with any Surreal function.
* `me()` can be used directly as a single element (like `querySelector()` or `$()`)
* `any()` can use: `for` / `forEach` / `filter` / `map` (like `querySelectorAll()` or `$()`)
* 🌗 No forced style. Use: `classAdd` or `class_add` or `addClass` or `add_class`
* Use `camelCase` (Javascript) or `snake_case` (Python, Rust, PHP, Ruby, SQL, CSS).
### 🤔 Why use `me()` / `any()` instead of `$()`
* 💡 Solves the classic jQuery bloat problem: Am I getting 1 element or an array of elements?
* `me()` is guaranteed to return 1 element (or first found, or null).
* `any()` is guaranteed to return an array (or empty array).
* No more checks = write less code. Bonus: Reads more like self-documenting english.
## 👁️ How does it look?
Do surreal things with [Locality of Behavior](https://htmx.org/essays/locality-of-behaviour/) like:
```html
<label for="file-input" >
<div class="uploader"></div>
<script>
me().on("dragover", ev => { halt(ev); me(ev).classAdd('.hover'); console.log("Files in drop zone.") })
me().on("dragleave", ev => { halt(ev); me(ev).classRemove('.hover'); console.log("Files left drop zone.") })
me().on("drop", ev => { halt(ev); me(ev).classRemove('.hover').classAdd('.loading'); me('#file-input').attribute('files', ev.dataTransfer.files); me('#form').send('change') })
</script>
</label>
```
See the [Live Example](https://gnat.github.io/surreal/example.html)! Then [view source](https://github.com/gnat/surreal/blob/main/example.html).
## 🎁 Install
Surreal is only 320 lines. No build step. No dependencies.
[📥 Download](https://raw.githubusercontent.com/gnat/surreal/main/surreal.js) into your project, and add `<script src="/surreal.js"></script>` in your `<head>`
Or, 🌐 via CDN: `<script src="https://cdnjs.cloudflare.com/ajax/libs/surreal/1.3.2/surreal.js"></script>`
## ⚡ Usage
### <a name="selectors"></a>🔍️ DOM Selection
* Select **one** element: `me(...)`
* Can be any of:
* CSS selector: `".button"`, `"#header"`, `"h1"`, `"body > .block"`
* Variables: `body`, `e`, `some_element`
* Events: `event.currentTarget` will be used.
* Surreal selectors: `me()`,`any()`
* Choose the start location in the DOM with the 2nd arg. (Default: `document`)
* 🔥 `any('button', me('#header')).classAdd('red')`
* Add `.red` to any `<button>` inside of `#header`
* `me()` ⭐ Get parent element of `<script>` without a **.class** or **#id** !
* `me("body")` Gets `<body>`
* `me(".button")` Gets the first `<div class="button">...</div>`. To get all of them use `any()`
* Select **one or more** elements as an array: `any(...)`
* Like `me()` but guaranteed to return an array (or empty array).
* `any(".foo")` ⭐ Get all matching elements.
* Convert between arrays of elements and single elements: `any(me())`, `me(any(".something"))`
### 🔥 DOM Functions
* ♻️ All functions work on single elements or arrays of elements.
* 🔗 Start a chain using `me()` and `any()`
* 🟢 Style A `me().classAdd('red')` ⭐ Chain style. Recommended!
* 🟠 Style B: `classAdd(me(), 'red')`
* 🌐 Global conveniences help you write less code.
* `globalsAdd()` will automatically warn you of any clobbering issues!
* 💀🩸 If you want no conveniences, or are a masochist, delete `globalsAdd()`
* 🟢 `me().classAdd('red')` becomes `surreal.me().classAdd('red')`
* 🟠 `classAdd(me(), 'red')` becomes `surreal.classAdd(surreal.me(), 'red')`
See: [Quick Start](#quick-start) and [Reference](#reference) and [No Surreal Needed](#no-surreal)
## <a name="quick-start"></a>⚡ Quick Start
* Add a class
* `me().classAdd('red')`
* `any("button").classAdd('red')`
* Events
* `me().on("click", ev => me(ev).fadeOut() )`
* `any('button').on('click', ev => { me(ev).styles('color: red') })`
* Run functions over elements.
* `any('button').run(_ => { alert(_) })`
* Styles / CSS
* `me().styles('color: red')`
* `me().styles({ 'color':'red', 'background':'blue' })`
* Attributes
* `me().attribute('active', true)`
<a name="timelines"></a>
#### Timeline animations without any libraries.
```html
<div>I change color every second.
<script>
// On click, animate something new every second.
me().on("click", async ev => {
let el = me(ev) // Save target because async will lose it.
me(el).styles({ "transition": "background 1s" })
await sleep(1000)
me(el).styles({ "background": "red" })
await sleep(1000)
me(el).styles({ "background": "green" })
await sleep(1000)
me(el).styles({ "background": "blue" })
await sleep(1000)
me(el).styles({ "background": "none" })
await sleep(1000)
me(el).remove()
})
</script>
</div>
```
```html
<div>I fade out and remove myself.
<script>me().on("click", ev => { me(ev).fadeOut() })</script>
</div>
```
```html
<div>Change color every second.
<script>
// Run immediately.
(async (e = me()) => {
me(e).styles({ "transition": "background 1s" })
await sleep(1000)
me(e).styles({ "background": "red" })
await sleep(1000)
me(e).styles({ "background": "green" })
await sleep(1000)
me(e).styles({ "background": "blue" })
await sleep(1000)
me(e).styles({ "background": "none" })
await sleep(1000)
me(e).remove()
})()
</script>
</div>
```
```html
<script>
// Run immediately, for every <button> globally!
(async () => {
any("button").fadeOut()
})()
</script>
```
#### Array methods
```js
any('button')?.forEach(...)
any('button')?.map(...)
```
## <a name="reference"></a>👁️ Functions
Looking for [DOM Selectors](#selectors)?
Looking for stuff [we recommend doing in vanilla JS](#no-surreal)?
### 🧭 Legend
* 🔗 Chainable off `me()` and `any()`
* 🌐 Global shortcut.
* 🔥 Runnable example.
* 🔌 Built-in Plugin
### 👁️ At a glance
* 🔗 `run`
* It's `forEach` but less wordy and works on single elements, too!
* 🔥 `me().run(e => { alert(e) })`
* 🔥 `any('button').run(e => { alert(e) })`
* 🔗 `remove`
* 🔥 `me().remove()`
* 🔥 `any('button').remove()`
* 🔗 `classAdd` 🌗 `class_add` 🌗 `addClass` 🌗 `add_class`
* 🔥 `me().classAdd('active')`
* Leading `.` is **optional**
* Same thing: `me().classAdd('active')` 🌗 `me().classAdd('.active')`
* 🔗 `classRemove` 🌗 `class_remove` 🌗 `removeClass` 🌗 `remove_class`
* 🔥 `me().classRemove('active')`
* 🔗 `classToggle` 🌗 `class_toggle` 🌗 `toggleClass` 🌗 `toggle_class`
* 🔥 `me().classToggle('active')`
* 🔗 `styles`
* 🔥 `me().styles('color: red')` Add style.
* 🔥 `me().styles({ 'color':'red', 'background':'blue' })` Add multiple styles.
* 🔥 `me().styles({ 'background':null })` Remove style.
* 🔗 `attribute` 🌗 `attributes` 🌗 `attr`
* Get: 🔥 `me().attribute('data-x')`
* For single elements.
* For many elements, wrap it in: `any(...).run(...)` or `any(...).forEach(...)`
* Set: 🔥`me().attribute('data-x', true)`
* Set multiple: 🔥 `me().attribute({ 'data-x':'yes', 'data-y':'no' })`
* Remove: 🔥 `me().attribute('data-x', null)`
* Remove multiple: 🔥 `me().attribute({ 'data-x': null, 'data-y':null })`
* 🔗 `send` 🌗 `trigger`
* 🔥 `me().send('change')`
* 🔥 `me().send('change', {'data':'thing'})`
* Wraps `dispatchEvent`
* 🔗 `on`
* 🔥 `me().on('click', ev => { me(ev).styles('background', 'red') })`
* Wraps `addEventListener`
* 🔗 `off`
* 🔥 `me().off('click', fn)`
* Wraps `removeEventListener`
* 🔗 `offAll`
* 🔥 `me().offAll()`
* 🔗 `disable`
* 🔥 `me().disable()`
* Easy alternative to `off()`. Disables click, key, submit events.
* 🔗 `enable`
* 🔥 `me().enable()`
* Opposite of `disable()`
* 🌐 `sleep`
* 🔥 `await sleep(1000, ev => { alert(ev) })`
* `async` version of `setTimeout`
* Wonderful for animation timelines.
* 🌐 `tick`
* 🔥 `await tick()`
* `await` version of `rAF` / `requestAnimationFrame`.
* Animation tick. Waits 1 frame.
* Great if you need to wait for events to propagate.
* 🌐 `rAF`
* 🔥 `rAF(e => { return e })`
* Animation tick. Fires when 1 frame has passed. Alias of [requestAnimationFrame](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame)
* Great if you need to wait for events to propagate.
* 🌐 `rIC`
* 🔥 `rIC(e => { return e })`
* Great time to compute. Fires function when JS is idle. Alias of [requestIdleCallback](https://developer.mozilla.org/en-US/docs/Web/API/Window/requestIdleCallback)
* 🌐 `halt`
* 🔥 `halt(event)`
* Prevent default browser behaviors.
* Wrapper for [preventDefault](https://developer.mozilla.org/en-US/docs/Web/API/Event/preventDefault)
* 🌐 `createElement` 🌗 `create_element`
* 🔥 `e_new = createElement("div"); me().prepend(e_new)`
* Alias of vanilla `document.createElement`
* 🌐 `onloadAdd` 🌗 `onload_add` 🌗 `addOnload` 🌗 `add_onload`
* 🔥 `onloadAdd(_ => { alert("loaded!"); })`
* 🔥 `<script>let e = me(); onloadAdd(_ => { me(e).on("click", ev => { alert("clicked") }) })</script>`
* Execute after the DOM is ready. Similar to jquery `ready()`
* Add to `window.onload` while preventing overwrites of `window.onload` and predictable loading!
* Alternatives:
* Skip missing elements using `?.` example: `me("video")?.requestFullscreen()`
* Place `<script>` after the loaded element.
* See `me('-')` / `me('prev')`
* 🔌 `fadeOut`
* See below
* 🔌 `fadeIn`
* See below
### <a name="plugin-included"></a>🔌 Built-in Plugins
### Effects
Build effects with `me().styles({...})` with timelines using [CSS transitioned `await` or callbacks](#timelines).
Common effects included:
* 🔗 `fadeOut` 🌗 `fade_out`
* Fade out and remove element.
* Keep element with `remove=false`.
* 🔥 `me().fadeOut()`
* 🔥 `me().fadeOut(ev => { alert("Faded out!") }, 3000)` Over 3 seconds then call function.
* 🔗 `fadeIn` 🌗 `fade_in`
* Fade in existing element which has `opacity: 0`
* 🔥 `me().fadeIn()`
* 🔥 `me().fadeIn(ev => { alert("Faded in!") }, 3000)` Over 3 seconds then call function.
## <a name="no-surreal"></a>⚪ No Surreal Needed
More often than not, Vanilla JS is the easiest way!
Logging
* 🔥 `console.log()` `console.warn()` `console.error()`
* Event logging: 🔥 `monitorEvents(me())` See: [Chrome Blog](https://developer.chrome.com/blog/quickly-monitor-events-from-the-console-panel-2/)
Benchmarking / Time It!
* 🔥 `console.time('name')`
* 🔥 `console.timeEnd('name')`
Text / HTML Content
* 🔥 `me().textContent = "hello world"`
* XSS Safe! See: [MDN](https://developer.mozilla.org/en-US/docs/Web/API/Node/textContent)
* 🔥 `me().innerHTML = "<p>hello world</p>"`
* 🔥 `me().innerText = "hello world"`
Children
* 🔥 `me().children`
* 🔥 `me().children.hidden = true`
Append / Prepend elements.
* 🔥 `me().prepend(new_element)`
* 🔥 `me().appendChild(new_element)`
* 🔥 `me().insertBefore(element, other_element.firstChild)`
* 🔥 `me().insertAdjacentHTML("beforebegin", new_element)`
AJAX (replace jQuery `ajax()`)
* Use [htmx](https://htmx.org/) or [htmz](https://leanrada.com/htmz/) or [fetch()](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) or [XMLHttpRequest()](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest) directly.
* Using `fetch()`
```js
me().on("click", async event => {
let e = me(event)
// EXAMPLE 1: Hit an endpoint.
if((await fetch("/webhook")).ok) console.log("Did the thing.")
// EXAMPLE 2: Get content and replace me()
try {
let response = await fetch('/endpoint')
if (response.ok) e.innerHTML = await response.text()
else console.warn('fetch(): Bad response')
}
catch (error) { console.warn(`fetch(): ${error}`) }
})
```
* Using `XMLHttpRequest()`
```js
me().on("click", async event => {
let e = me(event)
// EXAMPLE 1: Hit an endpoint.
var xhr = new XMLHttpRequest()
xhr.open("GET", "/webhook")
xhr.send()
// EXAMPLE 2: Get content and replace me()
var xhr = new XMLHttpRequest()
xhr.open("GET", "/endpoint")
xhr.onreadystatechange = () => {
if (xhr.readyState == 4 && xhr.status >= 200 && xhr.status < 300) e.innerHTML = xhr.responseText
}
xhr.send()
})
```
## 💎 Conventions & Tips
* Many ideas can be done in HTML / CSS (ex: dropdowns)
* `_` = for temporary or unused variables. Keep it short and sweet!
* `e`, `el`, `elt` = element
* `e`, `ev`, `evt` = event
* `f`, `fn` = function
#### Scope functions and variables inside `<script>`
* ⭐ Use a block `{ let note = "hi"; function hey(text) { alert(text) }; me().on('click', ev => { hey(note) }) }`
* `let` and `function` is scoped within `{ }`
* ⭐ Use `me()`
* `me().hey = (text) => { alert(text) }`
* `me().on('click', (ev) => { me(ev).hey("hi") })`
* ⭐ Use an event `me().on('click', ev => { /* add and call function here */ })`
* Use an inline module: `<script type="module">`
* Note: `me()` in modules will not see `parentElement`, explicit selectors are required: `me(".mybutton")`
#### Select a void element like `<input type="text" />`
* Use: `me('-')` or `me('prev')` or `me('previous')`
* 🔥 `<input type="text" /> <script>me('-').value = "hello"</script>`
* Inspired by the CSS "next sibling" combinator `+` but in reverse `-`
* Or, use a relative start.
* 🔥 `<form> <input type="text" n1 /> <script>me('[n1]', me()).value = "hello"</script> </form>`
#### Ignore call chain when element is missing.
* 🔥 `me("#i_dont_exist")?.classAdd('active')`
* No warnings: 🔥 `me("#i_dont_exist", document, false)?.classAdd('active')`
## <a name="plugins"></a>🔌 Your own plugin
Feel free to edit Surreal directly- but if you prefer, you can use plugins to effortlessly merge with new versions.
```javascript
function pluginHello(e) {
function hello(e, name="World") {
console.log(`Hello ${name} from ${e}`)
return e // Make chainable.
}
// Add sugar
e.hello = (name) => { return hello(e, name) }
}
surreal.plugins.push(pluginHello)
```
Now use your function like: `me().hello("Internet")`
* See the included `pluginEffects` for a more comprehensive example.
* Your functions are added globally by `globalsAdd()` If you do not want this, add it to the `restricted` list.
* Refer to an existing function to see how to make yours work with 1 or many elements.
Make an [issue](https://github.com/gnat/surreal/issues) or [pull request](https://github.com/gnat/surreal/pulls) if you think people would like to use it! If it's useful enough we'll want it in core.
### ⭐ Awesome Surreal examples, plugins, and resources: [awesome-surreal](https://github.com/gnat/awesome-surreal) !
## 📚️ Inspired by
* [jQuery](https://jquery.com/) for the chainable syntax we all love.
* [BlingBling.js](https://github.com/argyleink/blingblingjs) for modern minimalism.
* [Bliss.js](https://blissfuljs.com/) for a focus on single elements and extensibility.
* [Hyperscript](https://hyperscript.org) for Locality of Behavior and awesome ergonomics.
* Shout out to [Umbrella](https://umbrellajs.com/), [Cash](https://github.com/fabiospampinato/cash), [Zepto](https://zeptojs.com/)- Not quite as ergonomic. Requires build step to extend.
## 🌘 Future
* Always more `example.html` goodies!
* Automated browser testing perhaps with:
* [Fava](https://github.com/fabiospampinato/fava). See: https://github.com/avajs/ava/issues/24#issuecomment-885949036
* [Ava](https://github.com/avajs/ava/blob/main/docs/recipes/browser-testing.md)
* [jsdom](https://github.com/jsdom/jsdom)
* [jsdom notes](https://github.com/jsdom/jsdom#executing-scripts)</doc></docs></project> | xml |
llms.txt | Docs: CSS Scope Inline | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<docs><doc title="CSS Scope Inline" desc="A JS library which allow `me` to be used in CSS selectors, by using a `MutationObserver` to monitor the DOM"># 🌘 CSS Scope Inline
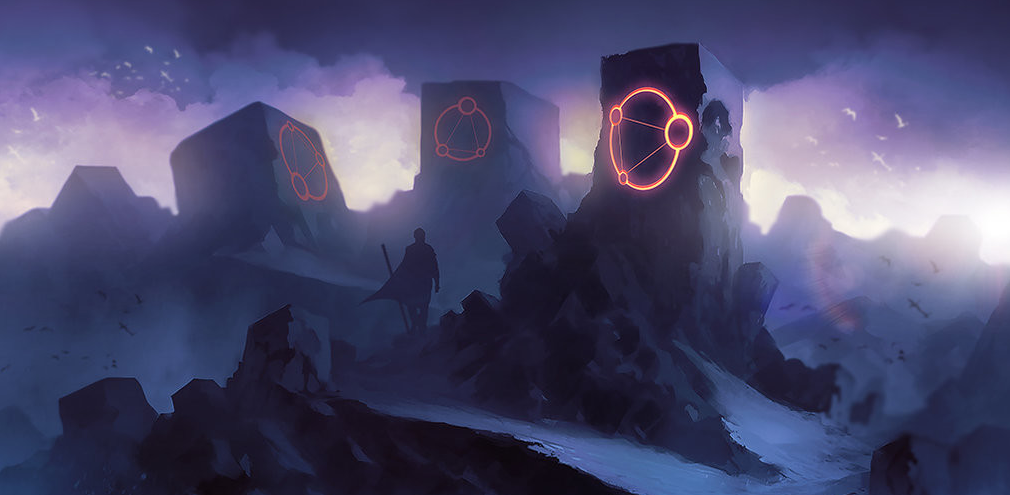
(Art by [shahabalizadeh](https://www.artstation.com/artwork/zDgdd))
## Why does this exist?
* You want an easy inline vanilla CSS experience without Tailwind CSS.
* Hate creating unique class names over.. and over.. to use once.
* You want to co-locate your styles for ⚡️ [Locality of Behavior (LoB)](https://htmx.org/essays/locality-of-behaviour/)
* You wish `this` would work in `<style>` tags.
* Want all CSS features: [Nesting](https://caniuse.com/css-nesting), animations. Get scoped [`@keyframes`](https://github.com/gnat/css-scope-inline/blob/main/example.html#L50)!
* You wish `@media` queries were shorter for [responsive design](https://tailwindcss.com/docs/responsive-design).
* Only 16 lines. No build step. No dependencies.
* Pairs well with [htmx](https://htmx.org) and [Surreal](https://github.com/gnat/surreal)
* Want fewer layers, less complexity. Are aware of the cargo cult. ✈️
✨ Want to also scope your `<script>` tags? See our companion project [Surreal](https://github.com/gnat/surreal)
## 👁️ How does it look?
```html
<div>
<style>
me { background: red; } /* ✨ this & self also work! */
me button { background: blue; } /* style child elements inline! */
</style>
<button>I'm blue</button>
</div>
```
See the [Live Example](https://gnat.github.io/css-scope-inline/example.html)! Then [view source](https://github.com/gnat/css-scope-inline/blob/main/example.html).
## 🌘 How does it work?
This uses `MutationObserver` to monitor the DOM, and the moment a `<style>` tag is seen, it scopes the styles to whatever the parent element is. No flashing or popping.
This method also leaves your existing styles untouched, allowing you to mix and match at your leisure.
## 🎁 Install
✂️ copy + 📋 paste the snippet into `<script>` in your `<head>`
Or, [📥 download](https://raw.githubusercontent.com/gnat/css-scope-inline/main/script.js) into your project, and add `<script src="script.js"></script>` in your `<head>`
Or, 🌐 CDN: `<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>`
## 🤔 Why consider this over Tailwind CSS?
Use whatever you'd like, but there's a few advantages with this approach over Tailwind, Twind, UnoCSS:
* No more [repeating styles](https://tailwindcss.com/docs/reusing-styles) on child elements (..no [@apply](https://tailwindcss.com/docs/reusing-styles#extracting-classes-with-apply), no `[&>thing]` per style). It's just CSS!
* No endless visual noise on every `<div>`. Use a local `<style>` per group.
* No high risk of eventually requiring a build step.
* No chance of [deprecations](https://windicss.org/posts/sunsetting.html). 16 lines is infinitely maintainable.
* Get the ultra-fast "inspect, play with styles, paste" workflow back.
* No suffering from missing syntax highlighting on properties and units.
* No suffering from FOUC (a flash of unstyled content).
* Zero friction movement of styles between inline and `.css` files. Just replace `me`
* No special tooling or plugins to install. Universal vanilla CSS.
## ⚡ Workflow Tips
* Flat, 1 selector per line can be very short like Tailwind. See the examples.
* Use just plain CSS variables in your design system.
* Use the short `@media` queries for responsive design.
* Mobile First (flow: **above** breakpoint): **🟢 None** `sm` `md` `lg` `xl` `xx` 🏁
* Desktop First (flow: **below** breakpoint): 🏁 `xs-` `sm-` `md-` `lg-` `xl-` **🟢 None**
* 🟢 = No breakpoint. Default. See the [Live Example](https://gnat.github.io/css-scope-inline/example.html)!
* Based on [Tailwind](https://tailwindcss.com/docs/responsive-design) breakpoints. We use `xx` not `2xl` to not break CSS highlighters.
* Unlike Tailwind, you can [nest your @media styles](https://developer.chrome.com/articles/css-nesting/#nesting-media)!
* Positional selectors may be easier using `div[n1]` for `<div n1>` instead of `div:nth-child(1)`
* Try tools like- Auto complete styles: [VSCode](https://code.visualstudio.com/) or [Sublime](https://packagecontrol.io/packages/Emmet)
## 👁️ CSS Scope Inline vs Tailwind CSS Showdowns
### Basics
Tailwind verbosity goes up with more child elements.
```html
<div>
<style>
me { background: red; }
me div { background: green; }
me div[n1] { background: yellow; }
me div[n2] { background: blue; }
</style>
red
<div>green</div>
<div>green</div>
<div>green</div>
<div n1>yellow</div>
<div n2>blue</div>
<div>green</div>
<div>green</div>
</div>
<div class="bg-[red]">
red
<div class="bg-[green]">green</div>
<div class="bg-[green]">green</div>
<div class="bg-[green]">green</div>
<div class="bg-[yellow]">yellow</div>
<div class="bg-[blue]">blue</div>
<div class="bg-[green]">green</div>
<div class="bg-[green]">green</div>
</div>
```
### CSS variables and child elements
At first glance, **Tailwind Example 2** looks very promising! Exciting ...but:
* 🔴 **Every child style requires an explicit selector.**
* Tailwinds' shorthand advantages sadly disappear.
* Any more child styles added in Tailwind will become longer than vanilla CSS.
* This limited example is the best case scenario for Tailwind.
* 🔴 Not visible on github: **no highlighting for properties and units** begins to be painful.
```html
<!doctype html>
<html>
<head>
<style>
:root {
--color-1: hsl(0 0% 88%);
--color-1-active: hsl(214 20% 70%);
}
</style>
<script src="https://cdn.tailwindcss.com"></script>
<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>
</head>
<body>
<!-- CSS Scope Inline -->
<div>
<style>
me { margin:8px 6px; }
me div a { display:block; padding:8px 12px; margin:10px 0; background:var(--color-1); border-radius:10px; text-align:center; }
me div a:hover { background:var(--color-1-active); color:white; }
</style>
<div><a href="#">Home</a></div>
<div><a href="#">Team</a></div>
<div><a href="#">Profile</a></div>
<div><a href="#">Settings</a></div>
<div><a href="#">Log Out</a></div>
</div>
<!-- Tailwind Example 1 -->
<div class="mx-2 my-4">
<div><a href="#" class="block py-2 px-3 my-2 bg-[--color-1] rounded-lg text-center hover:bg-[--color-1-active] hover:text-white">Home</a></div>
<div><a href="#" class="block py-2 px-3 my-2 bg-[--color-1] rounded-lg text-center hover:bg-[--color-1-active] hover:text-white">Team</a></div>
<div><a href="#" class="block py-2 px-3 my-2 bg-[--color-1] rounded-lg text-center hover:bg-[--color-1-active] hover:text-white">Profile</a></div>
<div><a href="#" class="block py-2 px-3 my-2 bg-[--color-1] rounded-lg text-center hover:bg-[--color-1-active] hover:text-white">Settings</a></div>
<div><a href="#" class="block py-2 px-3 my-2 bg-[--color-1] rounded-lg text-center hover:bg-[--color-1-active] hover:text-white">Log Out</a></div>
</div>
<!-- Tailwind Example 2 -->
<div class="mx-2 my-4
[&_div_a]:block [&_div_a]:py-2 [&_div_a]:px-3 [&_div_a]:my-2 [&_div_a]:bg-[--color-1] [&_div_a]:rounded-lg [&_div_a]:text-center
[&_div_a:hover]:bg-[--color-1-active] [&_div_a:hover]:text-white">
<div><a href="#">Home</a></div>
<div><a href="#">Team</a></div>
<div><a href="#">Profile</a></div>
<div><a href="#">Settings</a></div>
<div><a href="#">Log Out</a></div>
</div>
</body>
</html>
```
## 🔎 Technical FAQ
* Why do you use `querySelectorAll()` and not just process the `MutationObserver` results directly?
* This was indeed the original design; it will work well up until you begin recieving subtrees (ex: DOM swaps with [htmx](https://htmx.org), ajax, jquery, etc.) which requires walking all subtree elements to ensure we do not miss a `<style>`. This unfortunately involves re-scanning thousands of repeated elements. This is why `querySelectorAll()` ends up the performance (and simplicity) winner.</doc></docs></project> | xml |
llms.txt | Docs: Starlette quick guide | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<docs><doc title="Starlette quick guide" desc="A quick overview of some Starlette features useful to FastHTML devs."># 🌟 Starlette Quick Manual
2020-02-09
Starlette is the ASGI web framework used as the foundation of FastHTML. Listed here are some Starlette features FastHTML developers can use directly, since the `FastHTML` class inherits from the `Starlette` class (but note that FastHTML has its own customised `RouteX` and `RouterX` classes for routing, to handle FT element trees etc).
## Get uploaded file content
```
async def handler(request):
inp = await request.form()
uploaded_file = inp["filename"]
filename = uploaded_file.filename # abc.png
content_type = uploaded.content_type # MIME type, e.g. image/png
content = await uploaded_file.read() # image content
```
## Return a customized response (status code and headers)
```
import json
from starlette.responses import Response
async def handler(request):
data = {
"name": "Bo"
}
return Response(json.dumps(data), media_type="application/json")
```
`Response` takes `status_code`, `headers` and `media_type`, so if we want to change a response's status code, we can do:
```
return Response(content, statu_code=404)
```
And customized headers:
```
headers = {
"x-extra-key": "value"
}
return Response(content, status_code=200, headers=headers)
```
## Redirect
```
from starlette.responses import RedirectResponse
async handler(request):
# Customize status_code:
# 301: permanent redirect
# 302: temporary redirect
# 303: see others
# 307: temporary redirect (default)
return RedirectResponse(url=url, status_code=303)
```
## Request context
### URL Object: `request.url`
* Get request full url: `url = str(request.url)`
* Get scheme: `request.url.scheme` (http, https, ws, wss)
* Get netloc: `request.url.netloc`, e.g.: example.com:8080
* Get path: `request.url.path`, e.g.: /search
* Get query string: `request.url.query`, e.g.: kw=hello
* Get hostname: `request.url.hostname`, e.g.: example.com
* Get port: `request.url.port`, e.g.: 8080
* If using secure scheme: `request.url.is_secure`, True is schme is `https` or `wss`
### Headers: `request.headers`
```
{
'host': 'example.com:8080',
'connection': 'keep-alive',
'cache-control': 'max-age=0',
'sec-ch-ua': 'Google Chrome 80',
'dnt': '1',
'upgrade-insecure-requests': '1',
'user-agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_3) ...',
'sec-fetch-dest': 'document',
'accept': 'text/html,image/apng,*/*;q=0.8;v=b3;q=0.9',
'sec-origin-policy': '0',
'sec-fetch-site': 'none',
'sec-fetch-mode': 'navigate',
'sec-fetch-user': '?1',
'accept-encoding': 'gzip, deflate, br',
'accept-language': 'en-US,en;q=0.9,zh-CN;q=0.8,zh;q=0.7,zh-TW;q=0.6',
'cookie': 'session=eyJhZG1pbl91c2_KiQ...'
}
```
### Client: `request.client`
* `request.client.host`: get client sock IP
* `request.client.port`: get client sock port
### Method: `request.method`
* `request.method`: GET, POST, etc.
### Get Data
* `await request.body()`: get raw data from body
* `await request.json()`: get passed data and parse it as JSON
* `await request.form()`: get posted data and pass it as dictionary
### Scope: `request.scope`
```
{
'type': 'http',
'http_version': '1.1',
'server': ('127.0.0.1', 9092),
'client': ('127.0.0.1', 53102),
'scheme': 'https',
'method': 'GET',
'root_path': '',
'path': '/',
'raw_path': b'/',
'query_string': b'kw=hello',
'headers': [
(b'host', b'example.com:8080'),
(b'connection', b'keep-alive'),
(b'cache-control', b'max-age=0'),
...
],
'app': <starlette.applications.Starlette object at 0x1081bd650>,
'session': {'uid': '57ba03ea7333f72a25f837cf'},
'router': <starlette.routing.Router object at 0x1081bd6d0>,
'endpoint': <class 'app.index.Index'>,
'path_params': {}
}
```
## Put varaible in request & app scope
```
app.state.dbconn = get_db_conn()
request.state.start_time = time.time()
# use app-scope state variable in a request
request.app.state.dbconn
```
## Utility functions
### Use `State` to wrap a dictionary
```
from starlette.datastructures import State
data = {
"name": "Bo"
}
print(data["name"])
# now wrap it with State function
wrapped = State(data)
# You can use the dot syntaxt, but can't use `wrapped["name"]` any more.
print(wrapped.name)
```
### login_required wrapper function
NB: This is easier to do in FastHTML using Beforeware.
```
import functools
from starlette.endpoints import HTTPEndpoint
from starlette.responses import Response
def login_required(login_url="/signin"):
def decorator(handler):
@functools.wraps(handler)
async def new_handler(obj, req, *args, **kwargs):
user = req.session.get("login_user")
if user is None:
return seeother(login_url)
return await handler(obj, req, *args, **kwargs)
return new_handler
return decorator
class MyAccount(HTTPEndpiont):
@login_required()
async def get(self, request):
# some logic here
content = "hello"
return Response(content)
```
## Exceptions
Handle exception and customize 403, 404, 503, 500 page:
```
from starlette.exceptions import HTTPException
async def exc_handle_403(request, exc):
return HTMLResponse("My 403 page", status_code=exc.status_code)
async def exc_handle_404(request, exc):
return HTMLResponse("My 404 page", status_code=exc.status_code)
async def exc_handle_503(request, exc):
return HTMLResponse("Failed, please try it later", status_code=exc.status_code)
# error is not exception, 500 is server side unexpected error, all other status code will be treated as Exception
async def err_handle_500(request, exc):
import traceback
Log.error(traceback.format_exc())
return HTMLResponse("My 500 page", status_code=500)
# To add handler, we can add either status_code or Exception itself as key
exception_handlers = {
403: exc_handle_403,
404: exc_handle_404,
503: exc_handle_503,
500: err_handle_500,
#HTTPException: exc_handle_500,
}
app = Starlette(routes=routes, exception_handlers=exception_handlers)
```
## Background Task
### Put some async task as background task
```
import aiofiles
from starlette.background import BackgroundTask
from starlette.responses import Response
aiofiles_remove = aiofiles.os.wrap(os.remove)
async def del_file(fpath):
await aiofiles_remove(fpath)
async def handler(request):
content = ""
fpath = "/tmp/tmpfile.txt"
task = BackgroundTask(del_file, fpath=fpath)
return Response(content, background=task)
```
### Put multiple tasks as background task
```
from starlette.background import BackgroundTasks
async def task1(name):
pass
async def task2(email):
pass
async def handler(request):
tasks = BackgroundTasks()
tasks.add_task(task1, name="John")
tasks.add_task(task2, email="[email protected]")
content = ""
return Response(content, background=tasks)
```
## Write middleware
There are 2 ways to write middleware:
### Define `__call__` function:
```
class MyMiddleware:
def __init__(self, app):
self.app = app
async def __call__(self, scope, receive, send):
# see above scope dictionary as reference
headers = dict(scope["headers"])
# do something
# pass to next middleware
return await self.app(scope, receive, send)
```
### Use `BaseHTTPMiddleware`
```
from starlette.middleware.base import BaseHTTPMiddleware
class CustomHeaderMiddleware(BaseHTTPMiddleware):
async def dispatch(self, request, call_next):
# do something before pass to next middleware
response = await call_next(request)
# do something after next middleware returned
response.headers['X-Author'] = 'John'
return response
```</doc></docs></project> | xml |
llms.txt | API: API List | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<api><doc title="API List" desc="A succint list of all functions and methods in fasthtml."># fasthtml Module Documentation
## fasthtml.authmw
- `class BasicAuthMiddleware`
- `def __init__(self, app, cb, skip)`
- `def __call__(self, scope, receive, send)`
- `def authenticate(self, conn)`
## fasthtml.cli
- `@call_parse def railway_link()`
Link the current directory to the current project's Railway service
- `@call_parse def railway_deploy(name, mount)`
Deploy a FastHTML app to Railway
## fasthtml.components
> `ft_html` and `ft_hx` functions to add some conveniences to `ft`, along with a full set of basic HTML components, and functions to work with forms and `FT` conversion
- `def show(ft, *rest)`
Renders FT Components into HTML within a Jupyter notebook.
- `def File(fname)`
Use the unescaped text in file `fname` directly
- `def fill_form(form, obj)`
Fills named items in `form` using attributes in `obj`
- `def fill_dataclass(src, dest)`
Modifies dataclass in-place and returns it
- `def find_inputs(e, tags, **kw)`
Recursively find all elements in `e` with `tags` and attrs matching `kw`
- `def html2ft(html, attr1st)`
Convert HTML to an `ft` expression
- `def sse_message(elm, event)`
Convert element `elm` into a format suitable for SSE streaming
## fasthtml.core
> The `FastHTML` subclass of `Starlette`, along with the `RouterX` and `RouteX` classes it automatically uses.
- `def parsed_date(s)`
Convert `s` to a datetime
- `def snake2hyphens(s)`
Convert `s` from snake case to hyphenated and capitalised
- `@dataclass class HtmxHeaders`
- `def __bool__(self)`
- `def __init__(self, boosted, current_url, history_restore_request, prompt, request, target, trigger_name, trigger)`
- `def str2int(s)`
Convert `s` to an `int`
- `def str2date(s)`
`date.fromisoformat` with empty string handling
- `@dataclass class HttpHeader`
- `def __init__(self, k, v)`
- `@use_kwargs_dict(**htmx_resps) def HtmxResponseHeaders(**kwargs)`
HTMX response headers
- `def form2dict(form)`
Convert starlette form data to a dict
- `def parse_form(req)`
Starlette errors on empty multipart forms, so this checks for that situation
- `def flat_xt(lst)`
Flatten lists
- `class Beforeware`
- `def __init__(self, f, skip)`
- `def EventStream(s)`
Create a text/event-stream response from `s`
- `class WS_RouteX`
- `def __init__(self, app, path, recv, conn, disconn)`
- `def flat_tuple(o)`
Flatten lists
- `class Redirect`
Use HTMX or Starlette RedirectResponse as required to redirect to `loc`
- `def __init__(self, loc)`
- `def __response__(self, req)`
- `class RouteX`
- `def __init__(self, app, path, endpoint)`
- `class RouterX`
- `def __init__(self, app, routes, redirect_slashes, default)`
- `def add_route(self, path, endpoint, methods, name, include_in_schema)`
- `def add_ws(self, path, recv, conn, disconn, name)`
- `class FastHTML`
- `def __init__(self, debug, routes, middleware, exception_handlers, on_startup, on_shutdown, lifespan, hdrs, ftrs, before, after, ws_hdr, ct_hdr, surreal, htmx, default_hdrs, sess_cls, secret_key, session_cookie, max_age, sess_path, same_site, sess_https_only, sess_domain, key_fname, htmlkw, **bodykw)`
- `def ws(self, path, conn, disconn, name)`
Add a websocket route at `path`
- `@patch def route(self, path, methods, name, include_in_schema)`
Add a route at `path`
- `def serve(appname, app, host, port, reload, reload_includes, reload_excludes)`
Run the app in an async server, with live reload set as the default.
- `class Client`
A simple httpx ASGI client that doesn't require `async`
- `def __init__(self, app, url)`
- `def cookie(key, value, max_age, expires, path, domain, secure, httponly, samesite)`
Create a 'set-cookie' `HttpHeader`
- `@patch def static_route_exts(self, prefix, static_path, exts)`
Add a static route at URL path `prefix` with files from `static_path` and `exts` defined by `reg_re_param()`
- `@patch def static_route(self, ext, prefix, static_path)`
Add a static route at URL path `prefix` with files from `static_path` and single `ext` (including the '.')
- `class MiddlewareBase`
- `def __call__(self, scope, receive, send)`
- `class FtResponse`
Wrap an FT response with any Starlette `Response`
- `def __init__(self, content, status_code, headers, cls, media_type, background)`
- `def __response__(self, req)`
## fasthtml.fastapp
> The `fast_app` convenience wrapper
- `def fast_app(db_file, render, hdrs, ftrs, tbls, before, middleware, live, debug, routes, exception_handlers, on_startup, on_shutdown, lifespan, default_hdrs, pico, surreal, htmx, ws_hdr, secret_key, key_fname, session_cookie, max_age, sess_path, same_site, sess_https_only, sess_domain, htmlkw, bodykw, reload_attempts, reload_interval, static_path, **kwargs)`
Create a FastHTML or FastHTMLWithLiveReload app.
## fasthtml.js
> Basic external Javascript lib wrappers
- `def light_media(css)`
Render light media for day mode views
- `def dark_media(css)`
Render dark media for nught mode views
- `def MarkdownJS(sel)`
Implements browser-based markdown rendering.
- `def HighlightJS(sel, langs, light, dark)`
Implements browser-based syntax highlighting. Usage example [here](/tutorials/quickstart_for_web_devs.html#code-highlighting).
## fasthtml.live_reload
- `class FastHTMLWithLiveReload`
`FastHTMLWithLiveReload` enables live reloading.
This means that any code changes saved on the server will automatically
trigger a reload of both the server and browser window.
How does it work?
- a websocket is created at `/live-reload`
- a small js snippet `LIVE_RELOAD_SCRIPT` is injected into each webpage
- this snippet connects to the websocket at `/live-reload` and listens for an `onclose` event
- when the `onclose` event is detected the browser is reloaded
Why do we listen for an `onclose` event?
When code changes are saved the server automatically reloads if the --reload flag is set.
The server reload kills the websocket connection. The `onclose` event serves as a proxy
for "developer has saved some changes".
Usage
>>> from fasthtml.common import *
>>> app = FastHTMLWithLiveReload()
Run:
run_uv()
- `def __init__(self, *args, **kwargs)`
## fasthtml.oauth
> Basic scaffolding for handling OAuth
- `class GoogleAppClient`
A `WebApplicationClient` for Google oauth2
- `def __init__(self, client_id, client_secret, code, scope, **kwargs)`
- `@classmethod def from_file(cls, fname, code, scope, **kwargs)`
- `class GitHubAppClient`
A `WebApplicationClient` for GitHub oauth2
- `def __init__(self, client_id, client_secret, code, scope, **kwargs)`
- `class HuggingFaceClient`
A `WebApplicationClient` for HuggingFace oauth2
- `def __init__(self, client_id, client_secret, code, scope, state, **kwargs)`
- `class DiscordAppClient`
A `WebApplicationClient` for Discord oauth2
- `def __init__(self, client_id, client_secret, is_user, perms, scope, **kwargs)`
- `def login_link(self)`
- `def parse_response(self, code)`
- `@patch def login_link(self, redirect_uri, scope, state)`
Get a login link for this client
- `@patch def parse_response(self, code, redirect_uri)`
Get the token from the oauth2 server response
- `@patch def get_info(self, token)`
Get the info for authenticated user
- `@patch def retr_info(self, code, redirect_uri)`
Combines `parse_response` and `get_info`
- `@patch def retr_id(self, code, redirect_uri)`
Call `retr_info` and then return id/subscriber value
- `class OAuth`
- `def __init__(self, app, cli, skip, redir_path, logout_path, login_path)`
- `def redir_url(self, req)`
- `def login_link(self, req, scope, state)`
- `def login(self, info, state)`
- `def logout(self, session)`
- `def chk_auth(self, info, ident, session)`
## fasthtml.pico
> Basic components for generating Pico CSS tags
- `@delegates(ft_hx, keep=True) def Card(*c, **kwargs)`
A PicoCSS Card, implemented as an Article with optional Header and Footer
- `@delegates(ft_hx, keep=True) def Group(*c, **kwargs)`
A PicoCSS Group, implemented as a Fieldset with role 'group'
- `@delegates(ft_hx, keep=True) def Search(*c, **kwargs)`
A PicoCSS Search, implemented as a Form with role 'search'
- `@delegates(ft_hx, keep=True) def Grid(*c, **kwargs)`
A PicoCSS Grid, implemented as child Divs in a Div with class 'grid'
- `@delegates(ft_hx, keep=True) def DialogX(*c, **kwargs)`
A PicoCSS Dialog, with children inside a Card
- `@delegates(ft_hx, keep=True) def Container(*args, **kwargs)`
A PicoCSS Container, implemented as a Main with class 'container'
## fasthtml.svg
> Simple SVG FT elements
- `def Svg(*args, **kwargs)`
An SVG tag; xmlns is added automatically, and viewBox defaults to height and width if not provided
- `@delegates(ft_hx) def ft_svg(tag, *c, **kwargs)`
Create a standard `FT` element with some SVG-specific attrs
- `@delegates(ft_svg) def Rect(width, height, x, y, fill, stroke, stroke_width, rx, ry, **kwargs)`
A standard SVG `rect` element
- `@delegates(ft_svg) def Circle(r, cx, cy, fill, stroke, stroke_width, **kwargs)`
A standard SVG `circle` element
- `@delegates(ft_svg) def Ellipse(rx, ry, cx, cy, fill, stroke, stroke_width, **kwargs)`
A standard SVG `ellipse` element
- `def transformd(translate, scale, rotate, skewX, skewY, matrix)`
Create an SVG `transform` kwarg dict
- `@delegates(ft_svg) def Line(x1, y1, x2, y2, stroke, w, stroke_width, **kwargs)`
A standard SVG `line` element
- `@delegates(ft_svg) def Polyline(*args, **kwargs)`
A standard SVG `polyline` element
- `@delegates(ft_svg) def Polygon(*args, **kwargs)`
A standard SVG `polygon` element
- `@delegates(ft_svg) def Text(*args, **kwargs)`
A standard SVG `text` element
- `class PathFT`
- `def M(self, x, y)`
Move to.
- `def L(self, x, y)`
Line to.
- `def H(self, x)`
Horizontal line to.
- `def V(self, y)`
Vertical line to.
- `def Z(self)`
Close path.
- `def C(self, x1, y1, x2, y2, x, y)`
Cubic Bézier curve.
- `def S(self, x2, y2, x, y)`
Smooth cubic Bézier curve.
- `def Q(self, x1, y1, x, y)`
Quadratic Bézier curve.
- `def T(self, x, y)`
Smooth quadratic Bézier curve.
- `def A(self, rx, ry, x_axis_rotation, large_arc_flag, sweep_flag, x, y)`
Elliptical Arc.
- `def SvgOob(*args, **kwargs)`
Wraps an SVG shape as required for an HTMX OOB swap
- `def SvgInb(*args, **kwargs)`
Wraps an SVG shape as required for an HTMX inband swap
## fasthtml.xtend
> Simple extensions to standard HTML components, such as adding sensible defaults
- `@delegates(ft_hx, keep=True) def A(*c, **kwargs)`
An A tag; `href` defaults to '#' for more concise use with HTMX
- `@delegates(ft_hx, keep=True) def AX(txt, hx_get, target_id, hx_swap, href, **kwargs)`
An A tag with just one text child, allowing hx_get, target_id, and hx_swap to be positional params
- `@delegates(ft_hx, keep=True) def Form(*c, **kwargs)`
A Form tag; identical to plain `ft_hx` version except default `enctype='multipart/form-data'`
- `@delegates(ft_hx, keep=True) def Hidden(value, id, **kwargs)`
An Input of type 'hidden'
- `@delegates(ft_hx, keep=True) def CheckboxX(checked, label, value, id, name, **kwargs)`
A Checkbox optionally inside a Label, preceded by a `Hidden` with matching name
- `@delegates(ft_html, keep=True) def Script(code, **kwargs)`
A Script tag that doesn't escape its code
- `@delegates(ft_html, keep=True) def Style(*c, **kwargs)`
A Style tag that doesn't escape its code
- `def double_braces(s)`
Convert single braces to double braces if next to special chars or newline
- `def undouble_braces(s)`
Convert double braces to single braces if next to special chars or newline
- `def loose_format(s, **kw)`
String format `s` using `kw`, without being strict about braces outside of template params
- `def ScriptX(fname, src, nomodule, type, _async, defer, charset, crossorigin, integrity, **kw)`
A `script` element with contents read from `fname`
- `def replace_css_vars(css, pre, **kwargs)`
Replace `var(--)` CSS variables with `kwargs` if name prefix matches `pre`
- `def StyleX(fname, **kw)`
A `style` element with contents read from `fname` and variables replaced from `kw`
- `def Surreal(code)`
Wrap `code` in `domReadyExecute` and set `m=me()` and `p=me('-')`
- `def On(code, event, sel, me)`
An async surreal.js script block event handler for `event` on selector `sel,p`, making available parent `p`, event `ev`, and target `e`
- `def Prev(code, event)`
An async surreal.js script block event handler for `event` on previous sibling, with same vars as `On`
- `def Now(code, sel)`
An async surreal.js script block on selector `me(sel)`
- `def AnyNow(sel, code)`
An async surreal.js script block on selector `any(sel)`
- `def run_js(js, id, **kw)`
Run `js` script, auto-generating `id` based on name of caller if needed, and js-escaping any `kw` params
- `def jsd(org, repo, root, path, prov, typ, ver, esm, **kwargs)`
jsdelivr `Script` or CSS `Link` tag, or URL
- `@delegates(ft_hx, keep=True) def Titled(title, *args, **kwargs)`
An HTML partial containing a `Title`, and `H1`, and any provided children
- `def Socials(title, site_name, description, image, url, w, h, twitter_site, creator, card)`
OG and Twitter social card headers
- `def Favicon(light_icon, dark_icon)`
Light and dark favicon headers
</doc></api></project> | xml |
llms.txt | Examples: Websockets application | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<examples><doc title="Websockets application" desc="Very brief example of using websockets with HTMX and FastHTML">from asyncio import sleep
from fasthtml.common import *
app = FastHTML(ws_hdr=True)
rt = app.route
def mk_inp(): return Input(id='msg')
nid = 'notifications'
@rt('/')
async def get():
cts = Div(
Div(id=nid),
Form(mk_inp(), id='form', ws_send=True),
hx_ext='ws', ws_connect='/ws')
return Titled('Websocket Test', cts)
async def on_connect(send): await send(Div('Hello, you have connected', id=nid))
async def on_disconnect( ): print('Disconnected!')
@app.ws('/ws', conn=on_connect, disconn=on_disconnect)
async def ws(msg:str, send):
await send(Div('Hello ' + msg, id=nid))
await sleep(2)
return Div('Goodbye ' + msg, id=nid), mk_inp()
serve()
</doc></examples></project> | xml |
llms.txt | Examples: Todo list application | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<examples><doc title="Todo list application" desc="Detailed walk-thru of a complete CRUD app in FastHTML showing idiomatic use of FastHTML and HTMX patterns.">###
# Walkthrough of an idiomatic fasthtml app
###
# This fasthtml app includes functionality from fastcore, starlette, fastlite, and fasthtml itself.
# Run with: `python adv_app.py`
# Importing from `fasthtml.common` brings the key parts of all of these together.
# For simplicity, you can just `from fasthtml.common import *`:
from fasthtml.common import *
# ...or you can import everything into a namespace:
# from fasthtml import common as fh
# ...or you can import each symbol explicitly (which we're commenting out here but including for completeness):
"""
from fasthtml.common import (
# These are the HTML components we use in this app
A, AX, Button, Card, CheckboxX, Container, Div, Form, Grid, Group, H1, H2, Hidden, Input, Li, Main, Script, Style, Textarea, Title, Titled, Ul,
# These are FastHTML symbols we'll use
Beforeware, FastHTML, fast_app, SortableJS, fill_form, picolink, serve,
# These are from Starlette, Fastlite, fastcore, and the Python stdlib
FileResponse, NotFoundError, RedirectResponse, database, patch, dataclass
)
"""
from hmac import compare_digest
# You can use any database you want; it'll be easier if you pick a lib that supports the MiniDataAPI spec.
# Here we are using SQLite, with the FastLite library, which supports the MiniDataAPI spec.
db = database('data/utodos.db')
# The `t` attribute is the table collection. The `todos` and `users` tables are not created if they don't exist.
# Instead, you can use the `create` method to create them if needed.
todos,users = db.t.todos,db.t.users
if todos not in db.t:
# You can pass a dict, or kwargs, to most MiniDataAPI methods.
users.create(dict(name=str, pwd=str), pk='name')
todos.create(id=int, title=str, done=bool, name=str, details=str, priority=int, pk='id')
# Although you can just use dicts, it can be helpful to have types for your DB objects.
# The `dataclass` method creates that type, and stores it in the object, so it will use it for any returned items.
Todo,User = todos.dataclass(),users.dataclass()
# Any Starlette response class can be returned by a FastHTML route handler.
# In that case, FastHTML won't change it at all.
# Status code 303 is a redirect that can change POST to GET, so it's appropriate for a login page.
login_redir = RedirectResponse('/login', status_code=303)
# The `before` function is a *Beforeware* function. These are functions that run before a route handler is called.
def before(req, sess):
# This sets the `auth` attribute in the request scope, and gets it from the session.
# The session is a Starlette session, which is a dict-like object which is cryptographically signed,
# so it can't be tampered with.
# The `auth` key in the scope is automatically provided to any handler which requests it, and can not
# be injected by the user using query params, cookies, etc, so it should be secure to use.
auth = req.scope['auth'] = sess.get('auth', None)
# If the session key is not there, it redirects to the login page.
if not auth: return login_redir
# `xtra` is part of the MiniDataAPI spec. It adds a filter to queries and DDL statements,
# to ensure that the user can only see/edit their own todos.
todos.xtra(name=auth)
markdown_js = """
import { marked } from "https://cdn.jsdelivr.net/npm/marked/lib/marked.esm.js";
import { proc_htmx} from "https://cdn.jsdelivr.net/gh/answerdotai/fasthtml-js/fasthtml.js";
proc_htmx('.markdown', e => e.innerHTML = marked.parse(e.textContent));
"""
# We will use this in our `exception_handlers` dict
def _not_found(req, exc): return Titled('Oh no!', Div('We could not find that page :('))
# To create a Beforeware object, we pass the function itself, and optionally a list of regexes to skip.
bware = Beforeware(before, skip=[r'/favicon\.ico', r'/static/.*', r'.*\.css', '/login'])
# The `FastHTML` class is a subclass of `Starlette`, so you can use any parameters that `Starlette` accepts.
# In addition, you can add your Beforeware here, and any headers you want included in HTML responses.
# FastHTML includes the "HTMX" and "Surreal" libraries in headers, unless you pass `default_hdrs=False`.
app = FastHTML(before=bware,
# These are the same as Starlette exception_handlers, except they also support `FT` results
exception_handlers={404: _not_found},
# PicoCSS is a particularly simple CSS framework, with some basic integration built in to FastHTML.
# `picolink` is pre-defined with the header for the PicoCSS stylesheet.
# You can use any CSS framework you want, or none at all.
hdrs=(picolink,
# `Style` is an `FT` object, which are 3-element lists consisting of:
# (tag_name, children_list, attrs_dict).
# FastHTML composes them from trees and auto-converts them to HTML when needed.
# You can also use plain HTML strings in handlers and headers,
# which will be auto-escaped, unless you use `NotStr(...string...)`.
Style(':root { --pico-font-size: 100%; }'),
# Have a look at fasthtml/js.py to see how these Javascript libraries are added to FastHTML.
# They are only 5-10 lines of code each, and you can add your own too.
SortableJS('.sortable'),
# MarkdownJS is actually provided as part of FastHTML, but we've included the js code here
# so that you can see how it works.
Script(markdown_js, type='module'))
)
# We add `rt` as a shortcut for `app.route`, which is what we'll use to decorate our route handlers.
# When using `app.route` (or this shortcut), the only required argument is the path.
# The name of the decorated function (eg `get`, `post`, etc) is used as the HTTP verb for the handler.
rt = app.route
# For instance, this function handles GET requests to the `/login` path.
@rt("/login")
def get():
# This creates a form with two input fields, and a submit button.
# All of these components are `FT` objects. All HTML tags are provided in this form by FastHTML.
# If you want other custom tags (e.g. `MyTag`), they can be auto-generated by e.g
# `from fasthtml.components import MyTag`.
# Alternatively, manually call e.g `ft(tag_name, *children, **attrs)`.
frm = Form(
# Tags with a `name` attr will have `name` auto-set to the same as `id` if not provided
Input(id='name', placeholder='Name'),
Input(id='pwd', type='password', placeholder='Password'),
Button('login'),
action='/login', method='post')
# If a user visits the URL directly, FastHTML auto-generates a full HTML page.
# However, if the URL is accessed by HTMX, then one HTML partial is created for each element of the tuple.
# To avoid this auto-generation of a full page, return a `HTML` object, or a Starlette `Response`.
# `Titled` returns a tuple of a `Title` with the first arg and a `Container` with the rest.
# See the comments for `Title` later for details.
return Titled("Login", frm)
# Handlers are passed whatever information they "request" in the URL, as keyword arguments.
# Dataclasses, dicts, namedtuples, TypedDicts, and custom classes are automatically instantiated
# from form data.
# In this case, the `Login` class is a dataclass, so the handler will be passed `name` and `pwd`.
@dataclass
class Login: name:str; pwd:str
# This handler is called when a POST request is made to the `/login` path.
# The `login` argument is an instance of the `Login` class, which has been auto-instantiated from the form data.
# There are a number of special parameter names, which will be passed useful information about the request:
# `session`: the Starlette session; `request`: the Starlette request; `auth`: the value of `scope['auth']`,
# `htmx`: the HTMX headers, if any; `app`: the FastHTML app object.
# You can also pass any string prefix of `request` or `session`.
@rt("/login")
def post(login:Login, sess):
if not login.name or not login.pwd: return login_redir
# Indexing into a MiniDataAPI table queries by primary key, which is `name` here.
# It returns a dataclass object, if `dataclass()` has been called at some point, or a dict otherwise.
try: u = users[login.name]
# If the primary key does not exist, the method raises a `NotFoundError`.
# Here we use this to just generate a user -- in practice you'd probably to redirect to a signup page.
except NotFoundError: u = users.insert(login)
# This compares the passwords using a constant time string comparison
# https://sqreen.github.io/DevelopersSecurityBestPractices/timing-attack/python
if not compare_digest(u.pwd.encode("utf-8"), login.pwd.encode("utf-8")): return login_redir
# Because the session is signed, we can securely add information to it. It's stored in the browser cookies.
# If you don't pass a secret signing key to `FastHTML`, it will auto-generate one and store it in a file `./sesskey`.
sess['auth'] = u.name
return RedirectResponse('/', status_code=303)
# Instead of using `app.route` (or the `rt` shortcut), you can also use `app.get`, `app.post`, etc.
# In this case, the function name is not used to determine the HTTP verb.
@app.get("/logout")
def logout(sess):
del sess['auth']
return login_redir
# FastHTML uses Starlette's path syntax, and adds a `static` type which matches standard static file extensions.
# You can define your own regex path specifiers -- for instance this is how `static` is defined in FastHTML
# `reg_re_param("static", "ico|gif|jpg|jpeg|webm|css|js|woff|png|svg|mp4|webp|ttf|otf|eot|woff2|txt|xml|html")`
# In this app, we only actually have one static file, which is `favicon.ico`. But it would also be needed if
# we were referencing images, CSS/JS files, etc.
# Note, this function is unnecessary, as the `fast_app()` call already includes this functionality.
# However, it's included here to show how you can define your own static file handler.
@rt("/{fname:path}.{ext:static}")
def get(fname:str, ext:str): return FileResponse(f'{fname}.{ext}')
# The `patch` decorator, which is defined in `fastcore`, adds a method to an existing class.
# Here we are adding a method to the `Todo` class, which is returned by the `todos` table.
# The `__ft__` method is a special method that FastHTML uses to convert the object into an `FT` object,
# so that it can be composed into an FT tree, and later rendered into HTML.
@patch
def __ft__(self:Todo):
# Some FastHTML tags have an 'X' suffix, which means they're "extended" in some way.
# For instance, here `AX` is an extended `A` tag, which takes 3 positional arguments:
# `(text, hx_get, target_id)`.
# All underscores in FT attrs are replaced with hyphens, so this will create an `hx-get` attr,
# which HTMX uses to trigger a GET request.
# Generally, most of your route handlers in practice (as in this demo app) are likely to be HTMX handlers.
# For instance, for this demo, we only have two full-page handlers: the '/login' and '/' GET handlers.
show = AX(self.title, f'/todos/{self.id}', 'current-todo')
edit = AX('edit', f'/edit/{self.id}' , 'current-todo')
dt = '✅ ' if self.done else ''
# FastHTML provides some shortcuts. For instance, `Hidden` is defined as simply:
# `return Input(type="hidden", value=value, **kwargs)`
cts = (dt, show, ' | ', edit, Hidden(id="id", value=self.id), Hidden(id="priority", value="0"))
# Any FT object can take a list of children as positional args, and a dict of attrs as keyword args.
return Li(*cts, id=f'todo-{self.id}')
# This is the handler for the main todo list application.
# By including the `auth` parameter, it gets passed the current username, for displaying in the title.
@rt("/")
def get(auth):
title = f"{auth}'s Todo list"
top = Grid(H1(title), Div(A('logout', href='/logout'), style='text-align: right'))
# We don't normally need separate "screens" for adding or editing data. Here for instance,
# we're using an `hx-post` to add a new todo, which is added to the start of the list (using 'afterbegin').
new_inp = Input(id="new-title", name="title", placeholder="New Todo")
add = Form(Group(new_inp, Button("Add")),
hx_post="/", target_id='todo-list', hx_swap="afterbegin")
# In the MiniDataAPI spec, treating a table as a callable (i.e with `todos(...)` here) queries the table.
# Because we called `xtra` in our Beforeware, this queries the todos for the current user only.
# We can include the todo objects directly as children of the `Form`, because the `Todo` class has `__ft__` defined.
# This is automatically called by FastHTML to convert the `Todo` objects into `FT` objects when needed.
# The reason we put the todo list inside a form is so that we can use the 'sortable' js library to reorder them.
# That library calls the js `end` event when dragging is complete, so our trigger here causes our `/reorder`
# handler to be called.
frm = Form(*todos(order_by='priority'),
id='todo-list', cls='sortable', hx_post="/reorder", hx_trigger="end")
# We create an empty 'current-todo' Div at the bottom of our page, as a target for the details and editing views.
card = Card(Ul(frm), header=add, footer=Div(id='current-todo'))
# PicoCSS uses `<Main class='container'>` page content; `Container` is a tiny function that generates that.
# A handler can return either a single `FT` object or string, or a tuple of them.
# In the case of a tuple, the stringified objects are concatenated and returned to the browser.
# The `Title` tag has a special purpose: it sets the title of the page.
return Title(title), Container(top, card)
# This is the handler for the reordering of todos.
# It's a POST request, which is used by the 'sortable' js library.
# Because the todo list form created earlier included hidden inputs with the todo IDs,
# they are passed as form data. By using a parameter called (e.g) "id", FastHTML will try to find
# something suitable in the request with this name. In order, it searches as follows:
# path; query; cookies; headers; session keys; form data.
# Although all these are provided in the request as strings, FastHTML will use your parameter's type
# annotation to try to cast the value to the requested type.
# In the case of form data, there can be multiple values with the same key. So in this case,
# the parameter is a list of ints.
@rt("/reorder")
def post(id:list[int]):
for i,id_ in enumerate(id): todos.update({'priority':i}, id_)
# HTMX by default replaces the inner HTML of the calling element, which in this case is the todo list form.
# Therefore, we return the list of todos, now in the correct order, which will be auto-converted to FT for us.
# In this case, it's not strictly necessary, because sortable.js has already reorder the DOM elements.
# However, by returning the updated data, we can be assured that there aren't sync issues between the DOM
# and the server.
return tuple(todos(order_by='priority'))
# Refactoring components in FastHTML is as simple as creating Python functions.
# The `clr_details` function creates a Div with specific HTMX attributes.
# `hx_swap_oob='innerHTML'` tells HTMX to swap the inner HTML of the target element out-of-band,
# meaning it will update this element regardless of where the HTMX request originated from.
def clr_details(): return Div(hx_swap_oob='innerHTML', id='current-todo')
# This route handler uses a path parameter `{id}` which is automatically parsed and passed as an int.
@rt("/todos/{id}")
def delete(id:int):
# The `delete` method is part of the MiniDataAPI spec, removing the item with the given primary key.
todos.delete(id)
# Returning `clr_details()` ensures the details view is cleared after deletion,
# leveraging HTMX's out-of-band swap feature.
# Note that we are not returning *any* FT component that doesn't have an "OOB" swap, so the target element
# inner HTML is simply deleted. That's why the deleted todo is removed from the list.
return clr_details()
@rt("/edit/{id}")
def get(id:int):
# The `hx_put` attribute tells HTMX to send a PUT request when the form is submitted.
# `target_id` specifies which element will be updated with the server's response.
res = Form(Group(Input(id="title"), Button("Save")),
Hidden(id="id"), CheckboxX(id="done", label='Done'),
Textarea(id="details", name="details", rows=10),
hx_put="/", target_id=f'todo-{id}', id="edit")
# `fill_form` populates the form with existing todo data, and returns the result.
# Indexing into a table (`todos`) queries by primary key, which is `id` here. It also includes
# `xtra`, so this will only return the id if it belongs to the current user.
return fill_form(res, todos[id])
@rt("/")
def put(todo: Todo):
# `update` is part of the MiniDataAPI spec.
# Note that the updated todo is returned. By returning the updated todo, we can update the list directly.
# Because we return a tuple with `clr_details()`, the details view is also cleared.
return todos.update(todo), clr_details()
@rt("/")
def post(todo:Todo):
# `hx_swap_oob='true'` tells HTMX to perform an out-of-band swap, updating this element wherever it appears.
# This is used to clear the input field after adding the new todo.
new_inp = Input(id="new-title", name="title", placeholder="New Todo", hx_swap_oob='true')
# `insert` returns the inserted todo, which is appended to the start of the list, because we used
# `hx_swap='afterbegin'` when creating the todo list form.
return todos.insert(todo), new_inp
@rt("/todos/{id}")
def get(id:int):
todo = todos[id]
# `hx_swap` determines how the update should occur. We use "outerHTML" to replace the entire todo `Li` element.
btn = Button('delete', hx_delete=f'/todos/{todo.id}',
target_id=f'todo-{todo.id}', hx_swap="outerHTML")
# The "markdown" class is used here because that's the CSS selector we used in the JS earlier.
# Therefore this will trigger the JS to parse the markdown in the details field.
# Because `class` is a reserved keyword in Python, we use `cls` instead, which FastHTML auto-converts.
return Div(H2(todo.title), Div(todo.details, cls="markdown"), btn)
serve()</doc></examples></project> | xml |
llms.txt | Optional: Starlette full documentation | <project title="FastHTML" summary='FastHTML is a python library which brings together Starlette, Uvicorn, HTMX, and fastcore's `FT` "FastTags" into a library for creating server-rendered hypermedia applications. The `FastHTML` class itself inherits from `Starlette`, and adds decorator-based routing with many additions, Beforeware, automatic `FT` to HTML rendering, and much more.'>Things to remember when writing FastHTML apps:
- Although parts of its API are inspired by FastAPI, it is *not* compatible with FastAPI syntax and is not targeted at creating API services
- FastHTML includes support for Pico CSS and the fastlite sqlite library, although using both are optional; sqlalchemy can be used directly or via the fastsql library, and any CSS framework can be used. Support for the Surreal and css-scope-inline libraries are also included, but both are optional
- FastHTML is compatible with JS-native web components and any vanilla JS library, but not with React, Vue, or Svelte
- Use `serve()` for running uvicorn (`if __name__ == "__main__"` is not needed since it's automatic)
- When a title is needed with a response, use `Titled`; note that that already wraps children in `Container`, and already includes both the meta title as well as the H1 element.<optional><doc title="Starlette full documentation" desc="A subset of the Starlette documentation useful for FastHTML development."># index.md
---
# Starlette Introduction
Starlette is a lightweight [ASGI][asgi] framework/toolkit,
which is ideal for building async web services in Python.
It is production-ready, and gives you the following:
* A lightweight, low-complexity HTTP web framework.
* WebSocket support.
* In-process background tasks.
* Startup and shutdown events.
* Test client built on `httpx`.
* CORS, GZip, Static Files, Streaming responses.
* Session and Cookie support.
* 100% test coverage.
* 100% type annotated codebase.
* Few hard dependencies.
* Compatible with `asyncio` and `trio` backends.
* Great overall performance [against independent benchmarks][techempower].
## Requirements
Python 3.8+
## Installation
```shell
$ pip3 install starlette
```
You'll also want to install an ASGI server, such as [uvicorn](http://www.uvicorn.org/), [daphne](https://github.com/django/daphne/), or [hypercorn](https://pgjones.gitlab.io/hypercorn/).
```shell
$ pip3 install uvicorn
```
## Example
**example.py**:
```python
from starlette.applications import Starlette
from starlette.responses import JSONResponse
from starlette.routing import Route
async def homepage(request):
return JSONResponse({'hello': 'world'})
app = Starlette(debug=True, routes=[
Route('/', homepage),
])
```
Then run the application...
```shell
$ uvicorn example:app
```
For a more complete example, [see here](https://github.com/encode/starlette-example).
## Dependencies
Starlette only requires `anyio`, and the following dependencies are optional:
* [`httpx`][httpx] - Required if you want to use the `TestClient`.
* [`jinja2`][jinja2] - Required if you want to use `Jinja2Templates`.
* [`python-multipart`][python-multipart] - Required if you want to support form parsing, with `request.form()`.
* [`itsdangerous`][itsdangerous] - Required for `SessionMiddleware` support.
* [`pyyaml`][pyyaml] - Required for `SchemaGenerator` support.
You can install all of these with `pip3 install starlette[full]`.
## Framework or Toolkit
Starlette is designed to be used either as a complete framework, or as
an ASGI toolkit. You can use any of its components independently.
```python
from starlette.responses import PlainTextResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = PlainTextResponse('Hello, world!')
await response(scope, receive, send)
```
Run the `app` application in `example.py`:
```shell
$ uvicorn example:app
INFO: Started server process [11509]
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
```
Run uvicorn with `--reload` to enable auto-reloading on code changes.
## Modularity
The modularity that Starlette is designed on promotes building re-usable
components that can be shared between any ASGI framework. This should enable
an ecosystem of shared middleware and mountable applications.
The clean API separation also means it's easier to understand each component
in isolation.
---
# applications.md
Starlette includes an application class `Starlette` that nicely ties together all of
its other functionality.
```python
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.routing import Route, Mount, WebSocketRoute
from starlette.staticfiles import StaticFiles
def homepage(request):
return PlainTextResponse('Hello, world!')
def user_me(request):
username = "John Doe"
return PlainTextResponse('Hello, %s!' % username)
def user(request):
username = request.path_params['username']
return PlainTextResponse('Hello, %s!' % username)
async def websocket_endpoint(websocket):
await websocket.accept()
await websocket.send_text('Hello, websocket!')
await websocket.close()
def startup():
print('Ready to go')
routes = [
Route('/', homepage),
Route('/user/me', user_me),
Route('/user/{username}', user),
WebSocketRoute('/ws', websocket_endpoint),
Mount('/static', StaticFiles(directory="static")),
]
app = Starlette(debug=True, routes=routes, on_startup=[startup])
```
### Instantiating the application
::: starlette.applications.Starlette
:docstring:
### Storing state on the app instance
You can store arbitrary extra state on the application instance, using the
generic `app.state` attribute.
For example:
```python
app.state.ADMIN_EMAIL = '[email protected]'
```
### Accessing the app instance
Where a `request` is available (i.e. endpoints and middleware), the app is available on `request.app`.
# requests.md
Starlette includes a `Request` class that gives you a nicer interface onto
the incoming request, rather than accessing the ASGI scope and receive channel directly.
### Request
Signature: `Request(scope, receive=None)`
```python
from starlette.requests import Request
from starlette.responses import Response
async def app(scope, receive, send):
assert scope['type'] == 'http'
request = Request(scope, receive)
content = '%s %s' % (request.method, request.url.path)
response = Response(content, media_type='text/plain')
await response(scope, receive, send)
```
Requests present a mapping interface, so you can use them in the same
way as a `scope`.
For instance: `request['path']` will return the ASGI path.
If you don't need to access the request body you can instantiate a request
without providing an argument to `receive`.
#### Method
The request method is accessed as `request.method`.
#### URL
The request URL is accessed as `request.url`.
The property is a string-like object that exposes all the
components that can be parsed out of the URL.
For example: `request.url.path`, `request.url.port`, `request.url.scheme`.
#### Headers
Headers are exposed as an immutable, case-insensitive, multi-dict.
For example: `request.headers['content-type']`
#### Query Parameters
Query parameters are exposed as an immutable multi-dict.
For example: `request.query_params['search']`
#### Path Parameters
Router path parameters are exposed as a dictionary interface.
For example: `request.path_params['username']`
#### Client Address
The client's remote address is exposed as a named two-tuple `request.client` (or `None`).
The hostname or IP address: `request.client.host`
The port number from which the client is connecting: `request.client.port`
#### Cookies
Cookies are exposed as a regular dictionary interface.
For example: `request.cookies.get('mycookie')`
Cookies are ignored in case of an invalid cookie. (RFC2109)
#### Body
There are a few different interfaces for returning the body of the request:
The request body as bytes: `await request.body()`
The request body, parsed as form data or multipart: `async with request.form() as form:`
The request body, parsed as JSON: `await request.json()`
You can also access the request body as a stream, using the `async for` syntax:
```python
from starlette.requests import Request
from starlette.responses import Response
async def app(scope, receive, send):
assert scope['type'] == 'http'
request = Request(scope, receive)
body = b''
async for chunk in request.stream():
body += chunk
response = Response(body, media_type='text/plain')
await response(scope, receive, send)
```
If you access `.stream()` then the byte chunks are provided without storing
the entire body to memory. Any subsequent calls to `.body()`, `.form()`, or `.json()`
will raise an error.
In some cases such as long-polling, or streaming responses you might need to
determine if the client has dropped the connection. You can determine this
state with `disconnected = await request.is_disconnected()`.
#### Request Files
Request files are normally sent as multipart form data (`multipart/form-data`).
Signature: `request.form(max_files=1000, max_fields=1000)`
You can configure the number of maximum fields or files with the parameters `max_files` and `max_fields`:
```python
async with request.form(max_files=1000, max_fields=1000):
...
```
!!! info
These limits are for security reasons, allowing an unlimited number of fields or files could lead to a denial of service attack by consuming a lot of CPU and memory parsing too many empty fields.
When you call `async with request.form() as form` you receive a `starlette.datastructures.FormData` which is an immutable
multidict, containing both file uploads and text input. File upload items are represented as instances of `starlette.datastructures.UploadFile`.
`UploadFile` has the following attributes:
* `filename`: An `str` with the original file name that was uploaded or `None` if its not available (e.g. `myimage.jpg`).
* `content_type`: An `str` with the content type (MIME type / media type) or `None` if it's not available (e.g. `image/jpeg`).
* `file`: A <a href="https://docs.python.org/3/library/tempfile.html#tempfile.SpooledTemporaryFile" target="_blank">`SpooledTemporaryFile`</a> (a <a href="https://docs.python.org/3/glossary.html#term-file-like-object" target="_blank">file-like</a> object). This is the actual Python file that you can pass directly to other functions or libraries that expect a "file-like" object.
* `headers`: A `Headers` object. Often this will only be the `Content-Type` header, but if additional headers were included in the multipart field they will be included here. Note that these headers have no relationship with the headers in `Request.headers`.
* `size`: An `int` with uploaded file's size in bytes. This value is calculated from request's contents, making it better choice to find uploaded file's size than `Content-Length` header. `None` if not set.
`UploadFile` has the following `async` methods. They all call the corresponding file methods underneath (using the internal `SpooledTemporaryFile`).
* `async write(data)`: Writes `data` (`bytes`) to the file.
* `async read(size)`: Reads `size` (`int`) bytes of the file.
* `async seek(offset)`: Goes to the byte position `offset` (`int`) in the file.
* E.g., `await myfile.seek(0)` would go to the start of the file.
* `async close()`: Closes the file.
As all these methods are `async` methods, you need to "await" them.
For example, you can get the file name and the contents with:
```python
async with request.form() as form:
filename = form["upload_file"].filename
contents = await form["upload_file"].read()
```
!!! info
As settled in [RFC-7578: 4.2](https://www.ietf.org/rfc/rfc7578.txt), form-data content part that contains file
assumed to have `name` and `filename` fields in `Content-Disposition` header: `Content-Disposition: form-data;
name="user"; filename="somefile"`. Though `filename` field is optional according to RFC-7578, it helps
Starlette to differentiate which data should be treated as file. If `filename` field was supplied, `UploadFile`
object will be created to access underlying file, otherwise form-data part will be parsed and available as a raw
string.
#### Application
The originating Starlette application can be accessed via `request.app`.
#### Other state
If you want to store additional information on the request you can do so
using `request.state`.
For example:
`request.state.time_started = time.time()`
# responses.md
Starlette includes a few response classes that handle sending back the
appropriate ASGI messages on the `send` channel.
### Response
Signature: `Response(content, status_code=200, headers=None, media_type=None)`
* `content` - A string or bytestring.
* `status_code` - An integer HTTP status code.
* `headers` - A dictionary of strings.
* `media_type` - A string giving the media type. eg. "text/html"
Starlette will automatically include a Content-Length header. It will also
include a Content-Type header, based on the media_type and appending a charset
for text types, unless a charset has already been specified in the `media_type`.
Once you've instantiated a response, you can send it by calling it as an
ASGI application instance.
```python
from starlette.responses import Response
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = Response('Hello, world!', media_type='text/plain')
await response(scope, receive, send)
```
#### Set Cookie
Starlette provides a `set_cookie` method to allow you to set cookies on the response object.
Signature: `Response.set_cookie(key, value, max_age=None, expires=None, path="/", domain=None, secure=False, httponly=False, samesite="lax")`
* `key` - A string that will be the cookie's key.
* `value` - A string that will be the cookie's value.
* `max_age` - An integer that defines the lifetime of the cookie in seconds. A negative integer or a value of `0` will discard the cookie immediately. `Optional`
* `expires` - Either an integer that defines the number of seconds until the cookie expires, or a datetime. `Optional`
* `path` - A string that specifies the subset of routes to which the cookie will apply. `Optional`
* `domain` - A string that specifies the domain for which the cookie is valid. `Optional`
* `secure` - A bool indicating that the cookie will only be sent to the server if request is made using SSL and the HTTPS protocol. `Optional`
* `httponly` - A bool indicating that the cookie cannot be accessed via JavaScript through `Document.cookie` property, the `XMLHttpRequest` or `Request` APIs. `Optional`
* `samesite` - A string that specifies the samesite strategy for the cookie. Valid values are `'lax'`, `'strict'` and `'none'`. Defaults to `'lax'`. `Optional`
#### Delete Cookie
Conversely, Starlette also provides a `delete_cookie` method to manually expire a set cookie.
Signature: `Response.delete_cookie(key, path='/', domain=None)`
### HTMLResponse
Takes some text or bytes and returns an HTML response.
```python
from starlette.responses import HTMLResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = HTMLResponse('<html><body><h1>Hello, world!</h1></body></html>')
await response(scope, receive, send)
```
### PlainTextResponse
Takes some text or bytes and returns a plain text response.
```python
from starlette.responses import PlainTextResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = PlainTextResponse('Hello, world!')
await response(scope, receive, send)
```
### JSONResponse
Takes some data and returns an `application/json` encoded response.
```python
from starlette.responses import JSONResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = JSONResponse({'hello': 'world'})
await response(scope, receive, send)
```
#### Custom JSON serialization
If you need fine-grained control over JSON serialization, you can subclass
`JSONResponse` and override the `render` method.
For example, if you wanted to use a third-party JSON library such as
[orjson](https://pypi.org/project/orjson/):
```python
from typing import Any
import orjson
from starlette.responses import JSONResponse
class OrjsonResponse(JSONResponse):
def render(self, content: Any) -> bytes:
return orjson.dumps(content)
```
In general you *probably* want to stick with `JSONResponse` by default unless
you are micro-optimising a particular endpoint or need to serialize non-standard
object types.
### RedirectResponse
Returns an HTTP redirect. Uses a 307 status code by default.
```python
from starlette.responses import PlainTextResponse, RedirectResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
if scope['path'] != '/':
response = RedirectResponse(url='/')
else:
response = PlainTextResponse('Hello, world!')
await response(scope, receive, send)
```
### StreamingResponse
Takes an async generator or a normal generator/iterator and streams the response body.
```python
from starlette.responses import StreamingResponse
import asyncio
async def slow_numbers(minimum, maximum):
yield '<html><body><ul>'
for number in range(minimum, maximum + 1):
yield '<li>%d</li>' % number
await asyncio.sleep(0.5)
yield '</ul></body></html>'
async def app(scope, receive, send):
assert scope['type'] == 'http'
generator = slow_numbers(1, 10)
response = StreamingResponse(generator, media_type='text/html')
await response(scope, receive, send)
```
Have in mind that <a href="https://docs.python.org/3/glossary.html#term-file-like-object" target="_blank">file-like</a> objects (like those created by `open()`) are normal iterators. So, you can return them directly in a `StreamingResponse`.
### FileResponse
Asynchronously streams a file as the response.
Takes a different set of arguments to instantiate than the other response types:
* `path` - The filepath to the file to stream.
* `headers` - Any custom headers to include, as a dictionary.
* `media_type` - A string giving the media type. If unset, the filename or path will be used to infer a media type.
* `filename` - If set, this will be included in the response `Content-Disposition`.
* `content_disposition_type` - will be included in the response `Content-Disposition`. Can be set to "attachment" (default) or "inline".
File responses will include appropriate `Content-Length`, `Last-Modified` and `ETag` headers.
```python
from starlette.responses import FileResponse
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = FileResponse('statics/favicon.ico')
await response(scope, receive, send)
```
## Third party responses
#### [EventSourceResponse](https://github.com/sysid/sse-starlette)
A response class that implements [Server-Sent Events](https://html.spec.whatwg.org/multipage/server-sent-events.html). It enables event streaming from the server to the client without the complexity of websockets.
#### [baize.asgi.FileResponse](https://baize.aber.sh/asgi#fileresponse)
As a smooth replacement for Starlette [`FileResponse`](https://www.starlette.io/responses/#fileresponse), it will automatically handle [Head method](https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/HEAD) and [Range requests](https://developer.mozilla.org/en-US/docs/Web/HTTP/Range_requests).
# websockets.md
Starlette includes a `WebSocket` class that fulfils a similar role
to the HTTP request, but that allows sending and receiving data on a websocket.
### WebSocket
Signature: `WebSocket(scope, receive=None, send=None)`
```python
from starlette.websockets import WebSocket
async def app(scope, receive, send):
websocket = WebSocket(scope=scope, receive=receive, send=send)
await websocket.accept()
await websocket.send_text('Hello, world!')
await websocket.close()
```
WebSockets present a mapping interface, so you can use them in the same
way as a `scope`.
For instance: `websocket['path']` will return the ASGI path.
#### URL
The websocket URL is accessed as `websocket.url`.
The property is actually a subclass of `str`, and also exposes all the
components that can be parsed out of the URL.
For example: `websocket.url.path`, `websocket.url.port`, `websocket.url.scheme`.
#### Headers
Headers are exposed as an immutable, case-insensitive, multi-dict.
For example: `websocket.headers['sec-websocket-version']`
#### Query Parameters
Query parameters are exposed as an immutable multi-dict.
For example: `websocket.query_params['search']`
#### Path Parameters
Router path parameters are exposed as a dictionary interface.
For example: `websocket.path_params['username']`
### Accepting the connection
* `await websocket.accept(subprotocol=None, headers=None)`
### Sending data
* `await websocket.send_text(data)`
* `await websocket.send_bytes(data)`
* `await websocket.send_json(data)`
JSON messages default to being sent over text data frames, from version 0.10.0 onwards.
Use `websocket.send_json(data, mode="binary")` to send JSON over binary data frames.
### Receiving data
* `await websocket.receive_text()`
* `await websocket.receive_bytes()`
* `await websocket.receive_json()`
May raise `starlette.websockets.WebSocketDisconnect()`.
JSON messages default to being received over text data frames, from version 0.10.0 onwards.
Use `websocket.receive_json(data, mode="binary")` to receive JSON over binary data frames.
### Iterating data
* `websocket.iter_text()`
* `websocket.iter_bytes()`
* `websocket.iter_json()`
Similar to `receive_text`, `receive_bytes`, and `receive_json` but returns an
async iterator.
```python hl_lines="7-8"
from starlette.websockets import WebSocket
async def app(scope, receive, send):
websocket = WebSocket(scope=scope, receive=receive, send=send)
await websocket.accept()
async for message in websocket.iter_text():
await websocket.send_text(f"Message text was: {message}")
await websocket.close()
```
When `starlette.websockets.WebSocketDisconnect` is raised, the iterator will exit.
### Closing the connection
* `await websocket.close(code=1000, reason=None)`
### Sending and receiving messages
If you need to send or receive raw ASGI messages then you should use
`websocket.send()` and `websocket.receive()` rather than using the raw `send` and
`receive` callables. This will ensure that the websocket's state is kept
correctly updated.
* `await websocket.send(message)`
* `await websocket.receive()`
### Send Denial Response
If you call `websocket.close()` before calling `websocket.accept()` then
the server will automatically send a HTTP 403 error to the client.
If you want to send a different error response, you can use the
`websocket.send_denial_response()` method. This will send the response
and then close the connection.
* `await websocket.send_denial_response(response)`
This requires the ASGI server to support the WebSocket Denial Response
extension. If it is not supported a `RuntimeError` will be raised.
# routing.md
## HTTP Routing
Starlette has a simple but capable request routing system. A routing table
is defined as a list of routes, and passed when instantiating the application.
```python
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.routing import Route
async def homepage(request):
return PlainTextResponse("Homepage")
async def about(request):
return PlainTextResponse("About")
routes = [
Route("/", endpoint=homepage),
Route("/about", endpoint=about),
]
app = Starlette(routes=routes)
```
The `endpoint` argument can be one of:
* A regular function or async function, which accepts a single `request`
argument and which should return a response.
* A class that implements the ASGI interface, such as Starlette's [HTTPEndpoint](endpoints.md#httpendpoint).
## Path Parameters
Paths can use URI templating style to capture path components.
```python
Route('/users/{username}', user)
```
By default this will capture characters up to the end of the path or the next `/`.
You can use convertors to modify what is captured. The available convertors are:
* `str` returns a string, and is the default.
* `int` returns a Python integer.
* `float` returns a Python float.
* `uuid` return a Python `uuid.UUID` instance.
* `path` returns the rest of the path, including any additional `/` characters.
Convertors are used by prefixing them with a colon, like so:
```python
Route('/users/{user_id:int}', user)
Route('/floating-point/{number:float}', floating_point)
Route('/uploaded/{rest_of_path:path}', uploaded)
```
If you need a different converter that is not defined, you can create your own.
See below an example on how to create a `datetime` convertor, and how to register it:
```python
from datetime import datetime
from starlette.convertors import Convertor, register_url_convertor
class DateTimeConvertor(Convertor):
regex = "[0-9]{4}-[0-9]{2}-[0-9]{2}T[0-9]{2}:[0-9]{2}:[0-9]{2}(.[0-9]+)?"
def convert(self, value: str) -> datetime:
return datetime.strptime(value, "%Y-%m-%dT%H:%M:%S")
def to_string(self, value: datetime) -> str:
return value.strftime("%Y-%m-%dT%H:%M:%S")
register_url_convertor("datetime", DateTimeConvertor())
```
After registering it, you'll be able to use it as:
```python
Route('/history/{date:datetime}', history)
```
Path parameters are made available in the request, as the `request.path_params`
dictionary.
```python
async def user(request):
user_id = request.path_params['user_id']
...
```
## Handling HTTP methods
Routes can also specify which HTTP methods are handled by an endpoint:
```python
Route('/users/{user_id:int}', user, methods=["GET", "POST"])
```
By default function endpoints will only accept `GET` requests, unless specified.
## Submounting routes
In large applications you might find that you want to break out parts of the
routing table, based on a common path prefix.
```python
routes = [
Route('/', homepage),
Mount('/users', routes=[
Route('/', users, methods=['GET', 'POST']),
Route('/{username}', user),
])
]
```
This style allows you to define different subsets of the routing table in
different parts of your project.
```python
from myproject import users, auth
routes = [
Route('/', homepage),
Mount('/users', routes=users.routes),
Mount('/auth', routes=auth.routes),
]
```
You can also use mounting to include sub-applications within your Starlette
application. For example...
```python
# This is a standalone static files server:
app = StaticFiles(directory="static")
# This is a static files server mounted within a Starlette application,
# underneath the "/static" path.
routes = [
...
Mount("/static", app=StaticFiles(directory="static"), name="static")
]
app = Starlette(routes=routes)
```
## Reverse URL lookups
You'll often want to be able to generate the URL for a particular route,
such as in cases where you need to return a redirect response.
* Signature: `url_for(name, **path_params) -> URL`
```python
routes = [
Route("/", homepage, name="homepage")
]
# We can use the following to return a URL...
url = request.url_for("homepage")
```
URL lookups can include path parameters...
```python
routes = [
Route("/users/{username}", user, name="user_detail")
]
# We can use the following to return a URL...
url = request.url_for("user_detail", username=...)
```
If a `Mount` includes a `name`, then submounts should use a `{prefix}:{name}`
style for reverse URL lookups.
```python
routes = [
Mount("/users", name="users", routes=[
Route("/", user, name="user_list"),
Route("/{username}", user, name="user_detail")
])
]
# We can use the following to return URLs...
url = request.url_for("users:user_list")
url = request.url_for("users:user_detail", username=...)
```
Mounted applications may include a `path=...` parameter.
```python
routes = [
...
Mount("/static", app=StaticFiles(directory="static"), name="static")
]
# We can use the following to return URLs...
url = request.url_for("static", path="/css/base.css")
```
For cases where there is no `request` instance, you can make reverse lookups
against the application, although these will only return the URL path.
```python
url = app.url_path_for("user_detail", username=...)
```
## Host-based routing
If you want to use different routes for the same path based on the `Host` header.
Note that port is removed from the `Host` header when matching.
For example, `Host (host='example.org:3600', ...)` will be processed
even if the `Host` header contains or does not contain a port other than `3600`
(`example.org:5600`, `example.org`).
Therefore, you can specify the port if you need it for use in `url_for`.
There are several ways to connect host-based routes to your application
```python
site = Router() # Use eg. `@site.route()` to configure this.
api = Router() # Use eg. `@api.route()` to configure this.
news = Router() # Use eg. `@news.route()` to configure this.
routes = [
Host('api.example.org', api, name="site_api")
]
app = Starlette(routes=routes)
app.host('www.example.org', site, name="main_site")
news_host = Host('news.example.org', news)
app.router.routes.append(news_host)
```
URL lookups can include host parameters just like path parameters
```python
routes = [
Host("{subdomain}.example.org", name="sub", app=Router(routes=[
Mount("/users", name="users", routes=[
Route("/", user, name="user_list"),
Route("/{username}", user, name="user_detail")
])
]))
]
...
url = request.url_for("sub:users:user_detail", username=..., subdomain=...)
url = request.url_for("sub:users:user_list", subdomain=...)
```
## Route priority
Incoming paths are matched against each `Route` in order.
In cases where more that one route could match an incoming path, you should
take care to ensure that more specific routes are listed before general cases.
For example:
```python
# Don't do this: `/users/me` will never match incoming requests.
routes = [
Route('/users/{username}', user),
Route('/users/me', current_user),
]
# Do this: `/users/me` is tested first.
routes = [
Route('/users/me', current_user),
Route('/users/{username}', user),
]
```
## Working with Router instances
If you're working at a low-level you might want to use a plain `Router`
instance, rather that creating a `Starlette` application. This gives you
a lightweight ASGI application that just provides the application routing,
without wrapping it up in any middleware.
```python
app = Router(routes=[
Route('/', homepage),
Mount('/users', routes=[
Route('/', users, methods=['GET', 'POST']),
Route('/{username}', user),
])
])
```
## WebSocket Routing
When working with WebSocket endpoints, you should use `WebSocketRoute`
instead of the usual `Route`.
Path parameters, and reverse URL lookups for `WebSocketRoute` work the the same
as HTTP `Route`, which can be found in the HTTP [Route](#http-routing) section above.
The `endpoint` argument can be one of:
* An async function, which accepts a single `websocket` argument.
* A class that implements the ASGI interface, such as Starlette's [WebSocketEndpoint](endpoints.md#websocketendpoint).
# endpoints.md
Starlette includes the classes `HTTPEndpoint` and `WebSocketEndpoint` that provide a class-based view pattern for
handling HTTP method dispatching and WebSocket sessions.
### HTTPEndpoint
The `HTTPEndpoint` class can be used as an ASGI application:
```python
from starlette.responses import PlainTextResponse
from starlette.endpoints import HTTPEndpoint
class App(HTTPEndpoint):
async def get(self, request):
return PlainTextResponse(f"Hello, world!")
```
If you're using a Starlette application instance to handle routing, you can
dispatch to an `HTTPEndpoint` class. Make sure to dispatch to the class itself,
rather than to an instance of the class:
```python
from starlette.applications import Starlette
from starlette.responses import PlainTextResponse
from starlette.endpoints import HTTPEndpoint
from starlette.routing import Route
class Homepage(HTTPEndpoint):
async def get(self, request):
return PlainTextResponse(f"Hello, world!")
class User(HTTPEndpoint):
async def get(self, request):
username = request.path_params['username']
return PlainTextResponse(f"Hello, {username}")
routes = [
Route("/", Homepage),
Route("/{username}", User)
]
app = Starlette(routes=routes)
```
HTTP endpoint classes will respond with "405 Method not allowed" responses for any
request methods which do not map to a corresponding handler.
### WebSocketEndpoint
The `WebSocketEndpoint` class is an ASGI application that presents a wrapper around
the functionality of a `WebSocket` instance.
The ASGI connection scope is accessible on the endpoint instance via `.scope` and
has an attribute `encoding` which may optionally be set, in order to validate the expected websocket data in the `on_receive` method.
The encoding types are:
* `'json'`
* `'bytes'`
* `'text'`
There are three overridable methods for handling specific ASGI websocket message types:
* `async def on_connect(websocket, **kwargs)`
* `async def on_receive(websocket, data)`
* `async def on_disconnect(websocket, close_code)`
The `WebSocketEndpoint` can also be used with the `Starlette` application class.
# middleware.md
Starlette includes several middleware classes for adding behavior that is applied across
your entire application. These are all implemented as standard ASGI
middleware classes, and can be applied either to Starlette or to any other ASGI application.
## Using middleware
The Starlette application class allows you to include the ASGI middleware
in a way that ensures that it remains wrapped by the exception handler.
```python
from starlette.applications import Starlette
from starlette.middleware import Middleware
from starlette.middleware.httpsredirect import HTTPSRedirectMiddleware
from starlette.middleware.trustedhost import TrustedHostMiddleware
routes = ...
# Ensure that all requests include an 'example.com' or
# '*.example.com' host header, and strictly enforce https-only access.
middleware = [
Middleware(
TrustedHostMiddleware,
allowed_hosts=['example.com', '*.example.com'],
),
Middleware(HTTPSRedirectMiddleware)
]
app = Starlette(routes=routes, middleware=middleware)
```
Every Starlette application automatically includes two pieces of middleware by default:
* `ServerErrorMiddleware` - Ensures that application exceptions may return a custom 500 page, or display an application traceback in DEBUG mode. This is *always* the outermost middleware layer.
* `ExceptionMiddleware` - Adds exception handlers, so that particular types of expected exception cases can be associated with handler functions. For example raising `HTTPException(status_code=404)` within an endpoint will end up rendering a custom 404 page.
Middleware is evaluated from top-to-bottom, so the flow of execution in our example
application would look like this:
* Middleware
* `ServerErrorMiddleware`
* `TrustedHostMiddleware`
* `HTTPSRedirectMiddleware`
* `ExceptionMiddleware`
* Routing
* Endpoint
The following middleware implementations are available in the Starlette package:
- CORSMiddleware
- SessionMiddleware
- HTTPSRedirectMiddleware
- TrustedHostMiddleware
- GZipMiddleware
- BaseHTTPMiddleware
# lifespan.md
Starlette applications can register a lifespan handler for dealing with
code that needs to run before the application starts up, or when the application
is shutting down.
```python
import contextlib
from starlette.applications import Starlette
@contextlib.asynccontextmanager
async def lifespan(app):
async with some_async_resource():
print("Run at startup!")
yield
print("Run on shutdown!")
routes = [
...
]
app = Starlette(routes=routes, lifespan=lifespan)
```
Starlette will not start serving any incoming requests until the lifespan has been run.
The lifespan teardown will run once all connections have been closed, and
any in-process background tasks have completed.
Consider using [`anyio.create_task_group()`](https://anyio.readthedocs.io/en/stable/tasks.html)
for managing asynchronous tasks.
## Lifespan State
The lifespan has the concept of `state`, which is a dictionary that
can be used to share the objects between the lifespan, and the requests.
```python
import contextlib
from typing import AsyncIterator, TypedDict
import httpx
from starlette.applications import Starlette
from starlette.requests import Request
from starlette.responses import PlainTextResponse
from starlette.routing import Route
class State(TypedDict):
http_client: httpx.AsyncClient
@contextlib.asynccontextmanager
async def lifespan(app: Starlette) -> AsyncIterator[State]:
async with httpx.AsyncClient() as client:
yield {"http_client": client}
async def homepage(request: Request) -> PlainTextResponse:
client = request.state.http_client
response = await client.get("https://www.example.com")
return PlainTextResponse(response.text)
app = Starlette(
lifespan=lifespan,
routes=[Route("/", homepage)]
)
```
The `state` received on the requests is a **shallow** copy of the state received on the
lifespan handler.
## Running lifespan in tests
You should use `TestClient` as a context manager, to ensure that the lifespan is called.
```python
from example import app
from starlette.testclient import TestClient
def test_homepage():
with TestClient(app) as client:
# Application's lifespan is called on entering the block.
response = client.get("/")
assert response.status_code == 200
# And the lifespan's teardown is run when exiting the block.
```
# background.md
Starlette includes a `BackgroundTask` class for in-process background tasks.
A background task should be attached to a response, and will run only once
the response has been sent.
### Background Task
Used to add a single background task to a response.
Signature: `BackgroundTask(func, *args, **kwargs)`
```python
from starlette.applications import Starlette
from starlette.responses import JSONResponse
from starlette.routing import Route
from starlette.background import BackgroundTask
...
async def signup(request):
data = await request.json()
username = data['username']
email = data['email']
task = BackgroundTask(send_welcome_email, to_address=email)
message = {'status': 'Signup successful'}
return JSONResponse(message, background=task)
async def send_welcome_email(to_address):
...
routes = [
...
Route('/user/signup', endpoint=signup, methods=['POST'])
]
app = Starlette(routes=routes)
```
### BackgroundTasks
Used to add multiple background tasks to a response.
Signature: `BackgroundTasks(tasks=[])`
!!! important
The tasks are executed in order. In case one of the tasks raises
an exception, the following tasks will not get the opportunity to be executed.
# server-push.md
Starlette includes support for HTTP/2 and HTTP/3 server push, making it
possible to push resources to the client to speed up page load times.
### `Request.send_push_promise`
Used to initiate a server push for a resource. If server push is not available
this method does nothing.
Signature: `send_push_promise(path)`
* `path` - A string denoting the path of the resource.
```python
from starlette.applications import Starlette
from starlette.responses import HTMLResponse
from starlette.routing import Route, Mount
from starlette.staticfiles import StaticFiles
async def homepage(request):
"""
Homepage which uses server push to deliver the stylesheet.
"""
await request.send_push_promise("/static/style.css")
return HTMLResponse(
'<html><head><link rel="stylesheet" href="/static/style.css"/></head></html>'
)
routes = [
Route("/", endpoint=homepage),
Mount("/static", StaticFiles(directory="static"), name="static")
]
app = Starlette(routes=routes)
```
# exceptions.md
Starlette allows you to install custom exception handlers to deal with
how you return responses when errors or handled exceptions occur.
```python
from starlette.applications import Starlette
from starlette.exceptions import HTTPException
from starlette.requests import Request
from starlette.responses import HTMLResponse
HTML_404_PAGE = ...
HTML_500_PAGE = ...
async def not_found(request: Request, exc: HTTPException):
return HTMLResponse(content=HTML_404_PAGE, status_code=exc.status_code)
async def server_error(request: Request, exc: HTTPException):
return HTMLResponse(content=HTML_500_PAGE, status_code=exc.status_code)
exception_handlers = {
404: not_found,
500: server_error
}
app = Starlette(routes=routes, exception_handlers=exception_handlers)
```
If `debug` is enabled and an error occurs, then instead of using the installed
500 handler, Starlette will respond with a traceback response.
```python
app = Starlette(debug=True, routes=routes, exception_handlers=exception_handlers)
```
As well as registering handlers for specific status codes, you can also
register handlers for classes of exceptions.
In particular you might want to override how the built-in `HTTPException` class
is handled. For example, to use JSON style responses:
```python
async def http_exception(request: Request, exc: HTTPException):
return JSONResponse({"detail": exc.detail}, status_code=exc.status_code)
exception_handlers = {
HTTPException: http_exception
}
```
The `HTTPException` is also equipped with the `headers` argument. Which allows the propagation
of the headers to the response class:
```python
async def http_exception(request: Request, exc: HTTPException):
return JSONResponse(
{"detail": exc.detail},
status_code=exc.status_code,
headers=exc.headers
)
```
You might also want to override how `WebSocketException` is handled:
```python
async def websocket_exception(websocket: WebSocket, exc: WebSocketException):
await websocket.close(code=1008)
exception_handlers = {
WebSocketException: websocket_exception
}
```
## Errors and handled exceptions
It is important to differentiate between handled exceptions and errors.
Handled exceptions do not represent error cases. They are coerced into appropriate
HTTP responses, which are then sent through the standard middleware stack. By default
the `HTTPException` class is used to manage any handled exceptions.
Errors are any other exception that occurs within the application. These cases
should bubble through the entire middleware stack as exceptions. Any error
logging middleware should ensure that it re-raises the exception all the
way up to the server.
In practical terms, the error handled used is `exception_handler[500]` or `exception_handler[Exception]`.
Both keys `500` and `Exception` can be used. See below:
```python
async def handle_error(request: Request, exc: HTTPException):
# Perform some logic
return JSONResponse({"detail": exc.detail}, status_code=exc.status_code)
exception_handlers = {
Exception: handle_error # or "500: handle_error"
}
```
It's important to notice that in case a [`BackgroundTask`](https://www.starlette.io/background/) raises an exception,
it will be handled by the `handle_error` function, but at that point, the response was already sent. In other words,
the response created by `handle_error` will be discarded. In case the error happens before the response was sent, then
it will use the response object - in the above example, the returned `JSONResponse`.
In order to deal with this behaviour correctly, the middleware stack of a
`Starlette` application is configured like this:
* `ServerErrorMiddleware` - Returns 500 responses when server errors occur.
* Installed middleware
* `ExceptionMiddleware` - Deals with handled exceptions, and returns responses.
* Router
* Endpoints
## HTTPException
The `HTTPException` class provides a base class that you can use for any
handled exceptions. The `ExceptionMiddleware` implementation defaults to
returning plain-text HTTP responses for any `HTTPException`.
* `HTTPException(status_code, detail=None, headers=None)`
You should only raise `HTTPException` inside routing or endpoints. Middleware
classes should instead just return appropriate responses directly.
## WebSocketException
You can use the `WebSocketException` class to raise errors inside of WebSocket endpoints.
* `WebSocketException(code=1008, reason=None)`
You can set any code valid as defined [in the specification](https://tools.ietf.org/html/rfc6455#section-7.4.1).
# testclient.md
The test client allows you to make requests against your ASGI application,
using the `httpx` library.
```python
from starlette.responses import HTMLResponse
from starlette.testclient import TestClient
async def app(scope, receive, send):
assert scope['type'] == 'http'
response = HTMLResponse('<html><body>Hello, world!</body></html>')
await response(scope, receive, send)
def test_app():
client = TestClient(app)
response = client.get('/')
assert response.status_code == 200
```
The test client exposes the same interface as any other `httpx` session.
In particular, note that the calls to make a request are just standard
function calls, not awaitables.
You can use any of `httpx` standard API, such as authentication, session
cookies handling, or file uploads.
For example, to set headers on the TestClient you can do:
```python
client = TestClient(app)
# Set headers on the client for future requests
client.headers = {"Authorization": "..."}
response = client.get("/")
# Set headers for each request separately
response = client.get("/", headers={"Authorization": "..."})
```
And for example to send files with the TestClient:
```python
client = TestClient(app)
# Send a single file
with open("example.txt", "rb") as f:
response = client.post("/form", files={"file": f})
# Send multiple files
with open("example.txt", "rb") as f1:
with open("example.png", "rb") as f2:
files = {"file1": f1, "file2": ("filename", f2, "image/png")}
response = client.post("/form", files=files)
```
### Testing WebSocket sessions
You can also test websocket sessions with the test client.
The `httpx` library will be used to build the initial handshake, meaning you
can use the same authentication options and other headers between both http and
websocket testing.
```python
from starlette.testclient import TestClient
from starlette.websockets import WebSocket
async def app(scope, receive, send):
assert scope['type'] == 'websocket'
websocket = WebSocket(scope, receive=receive, send=send)
await websocket.accept()
await websocket.send_text('Hello, world!')
await websocket.close()
def test_app():
client = TestClient(app)
with client.websocket_connect('/') as websocket:
data = websocket.receive_text()
assert data == 'Hello, world!'
```
#### Sending data
* `.send_text(data)` - Send the given text to the application.
* `.send_bytes(data)` - Send the given bytes to the application.
* `.send_json(data, mode="text")` - Send the given data to the application. Use `mode="binary"` to send JSON over binary data frames.
#### Receiving data
* `.receive_text()` - Wait for incoming text sent by the application and return it.
* `.receive_bytes()` - Wait for incoming bytestring sent by the application and return it.
* `.receive_json(mode="text")` - Wait for incoming json data sent by the application and return it. Use `mode="binary"` to receive JSON over binary data frames.
May raise `starlette.websockets.WebSocketDisconnect`.
#### Closing the connection
* `.close(code=1000)` - Perform a client-side close of the websocket connection.
### Asynchronous tests
Sometimes you will want to do async things outside of your application.
For example, you might want to check the state of your database after calling your app using your existing async database client / infrastructure.
For these situations, using `TestClient` is difficult because it creates it's own event loop and async resources (like a database connection) often cannot be shared across event loops.
The simplest way to work around this is to just make your entire test async and use an async client, like [httpx.AsyncClient].
Here is an example of such a test:
```python
from httpx import AsyncClient
from starlette.applications import Starlette
from starlette.routing import Route
from starlette.requests import Request
from starlette.responses import PlainTextResponse
def hello(request: Request) -> PlainTextResponse:
return PlainTextResponse("Hello World!")
app = Starlette(routes=[Route("/", hello)])
# if you're using pytest, you'll need to to add an async marker like:
# @pytest.mark.anyio # using https://github.com/agronholm/anyio
# or install and configure pytest-asyncio (https://github.com/pytest-dev/pytest-asyncio)
async def test_app() -> None:
# note: you _must_ set `base_url` for relative urls like "/" to work
async with AsyncClient(app=app, base_url="http://testserver") as client:
r = await client.get("/")
assert r.status_code == 200
assert r.text == "Hello World!"
```</doc></optional></project> | xml |
FastHTML Docs | index.html.md | # FastHTML
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
Welcome to the official FastHTML documentation.
FastHTML is a new next-generation web framework for fast, scalable web
applications with minimal, compact code. It’s designed to be:
- Powerful and expressive enough to build the most advanced, interactive
web apps you can imagine.
- Fast and lightweight, so you can write less code and get more done.
- Easy to learn and use, with a simple, intuitive syntax that makes it
easy to build complex apps quickly.
FastHTML apps are just Python code, so you can use FastHTML with the
full power of the Python language and ecosystem. FastHTML’s
functionality maps 1:1 directly to HTML and HTTP, but allows them to be
encapsulated using good software engineering practices—so you’ll need to
understand these foundations to use this library fully. To understand
how and why this works, please read this first:
[about.fastht.ml](https://about.fastht.ml/).
## Installation
Since `fasthtml` is a Python library, you can install it with:
``` sh
pip install python-fasthtml
```
In the near future, we hope to add component libraries that can likewise
be installed via `pip`.
## Usage
For a minimal app, create a file “main.py” as follows:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app,rt = fast_app()
@rt('/')
def get(): return Div(P('Hello World!'), hx_get="/change")
serve()
```
</div>
Running the app with `python main.py` prints out a link to your running
app: `http://localhost:5001`. Visit that link in your browser and you
should see a page with the text “Hello World!”. Congratulations, you’ve
just created your first FastHTML app!
Adding interactivity is surprisingly easy, thanks to HTMX. Modify the
file to add this function:
<div class="code-with-filename">
**main.py**
``` python
@rt('/change')
def get(): return P('Nice to be here!')
```
</div>
You now have a page with a clickable element that changes the text when
clicked. When clicking on this link, the server will respond with an
“HTML partial”—that is, just a snippet of HTML which will be inserted
into the existing page. In this case, the returned element will replace
the original P element (since that’s the default behavior of HTMX) with
the new version returned by the second route.
This “hypermedia-based” approach to web development is a powerful way to
build web applications.
### Getting help from AI
Because FastHTML is newer than most LLMs, AI systems like Cursor,
ChatGPT, Claude, and Copilot won’t give useful answers about it. To fix
that problem, we’ve provided an LLM-friendly guide that teaches them how
to use FastHTML. To use it, add this link for your AI helper to use:
- [/llms-ctx.txt](https://docs.fastht.ml/llms-ctx.txt)
This example is in a format based on recommendations from Anthropic for
use with [Claude
Projects](https://support.anthropic.com/en/articles/9517075-what-are-projects).
This works so well that we’ve actually found that Claude can provide
even better information than our own documentation! For instance, read
through [this annotated Claude
chat](https://gist.github.com/jph00/9559b0a563f6a370029bec1d1cc97b74)
for some great getting-started information, entirely generated from a
project using the above text file as context.
If you use Cursor, type `@doc` then choose “*Add new doc*”, and use the
/llms-ctx.txt link above. The context file is auto-generated from our
[`llms.txt`](https://llmstxt.org/) (our proposed standard for providing
AI-friendly information)—you can generate alternative versions suitable
for other models as needed.
## Next Steps
Start with the official sources to learn more about FastHTML:
- [About](https://about.fastht.ml): Learn about the core ideas behind
FastHTML
- [Documentation](https://docs.fastht.ml): Learn from examples how to
write FastHTML code
- [Idiomatic
app](https://github.com/AnswerDotAI/fasthtml/blob/main/examples/adv_app.py):
Heavily commented source code walking through a complete application,
including custom authentication, JS library connections, and database
use.
We also have a 1-hour intro video:
<https://www.youtube.com/embed/Auqrm7WFc0I>
The capabilities of FastHTML are vast and growing, and not all the
features and patterns have been documented yet. Be prepared to invest
time into studying and modifying source code, such as the main FastHTML
repo’s notebooks and the official FastHTML examples repo:
- [FastHTML Examples Repo on
GitHub](https://github.com/AnswerDotAI/fasthtml-example)
- [FastHTML Repo on GitHub](https://github.com/AnswerDotAI/fasthtml)
Then explore the small but growing third-party ecosystem of FastHTML
tutorials, notebooks, libraries, and components:
- [FastHTML Gallery](https://gallery.fastht.ml): Learn from minimal
examples of components (ie chat bubbles, click-to-edit, infinite
scroll, etc)
- [Creating Custom FastHTML Tags for Markdown
Rendering](https://isaac-flath.github.io/website/posts/boots/FasthtmlTutorial.html)
by Isaac Flath
- Your tutorial here!
Finally, join the FastHTML community to ask questions, share your work,
and learn from others:
- [Discord](https://discord.gg/qcXvcxMhdP)
| md |
FastHTML Docs | api/cli.html.md | # Command Line Tools
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/cli.py#L15"
target="_blank" style="float:right; font-size:smaller">source</a>
### railway_link
> railway_link ()
*Link the current directory to the current project’s Railway service*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/cli.py#L33"
target="_blank" style="float:right; font-size:smaller">source</a>
### railway_deploy
> railway_deploy (name:str, mount:<function bool_arg>=True)
*Deploy a FastHTML app to Railway*
<table>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>name</td>
<td>str</td>
<td></td>
<td>The project name to deploy</td>
</tr>
<tr class="even">
<td>mount</td>
<td>bool_arg</td>
<td>True</td>
<td>Create a mounted volume at /app/data?</td>
</tr>
</tbody>
</table>
| md |
FastHTML Docs | api/components.html.md | # Components
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
``` python
from lxml import html as lx
from pprint import pprint
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L34"
target="_blank" style="float:right; font-size:smaller">source</a>
### show
> show (ft, *rest)
*Renders FT Components into HTML within a Jupyter notebook.*
``` python
sentence = P(Strong("FastHTML is", I("Fast")))
# When placed within the `show()` function, this will render
# the HTML in Jupyter notebooks.
show(sentence)
```
<p>
<strong>
FastHTML is
<i>Fast</i>
</strong>
</p>
``` python
# Called without the `show()` function, the raw HTML is displayed
sentence
```
``` html
<p>
<strong>
FastHTML is
<i>Fast</i>
</strong>
</p>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L46"
target="_blank" style="float:right; font-size:smaller">source</a>
### attrmap_x
> attrmap_x (o)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L55"
target="_blank" style="float:right; font-size:smaller">source</a>
### ft_html
> ft_html (tag:str, *c, id=None, cls=None, title=None, style=None,
> attrmap=None, valmap=None, ft_cls=<class 'fastcore.xml.FT'>,
> **kwargs)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L67"
target="_blank" style="float:right; font-size:smaller">source</a>
### ft_hx
> ft_hx (tag:str, *c, target_id=None, hx_vals=None, id=None, cls=None,
> title=None, style=None, accesskey=None, contenteditable=None,
> dir=None, draggable=None, enterkeyhint=None, hidden=None,
> inert=None, inputmode=None, lang=None, popover=None,
> spellcheck=None, tabindex=None, translate=None, hx_get=None,
> hx_post=None, hx_put=None, hx_delete=None, hx_patch=None,
> hx_trigger=None, hx_target=None, hx_swap=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None,
> hx_history_elt=None, hx_inherit=None, hx_params=None,
> hx_preserve=None, hx_prompt=None, hx_request=None, hx_sync=None,
> hx_validate=None, **kwargs)
``` python
ft_html('a', _at_click_dot_away=1)
```
``` html
<a @click_dot_away="1"></a>
```
``` python
ft_html('a', **{'@click.away':1})
```
``` html
<a @click.away="1"></a>
```
``` python
ft_html('a', {'@click.away':1})
```
``` html
<a @click.away="1"></a>
```
``` python
ft_hx('a', hx_vals={'a':1})
```
``` html
<a hx-vals='{"a": 1}'></a>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L87"
target="_blank" style="float:right; font-size:smaller">source</a>
### File
> File (fname)
*Use the unescaped text in file `fname` directly*
For tags that have a `name` attribute, it will be set to the value of
`id` if not provided explicitly:
``` python
Form(Button(target_id='foo', id='btn'),
hx_post='/', target_id='tgt', id='frm')
```
``` html
<form hx-post="/" hx-target="#tgt" id="frm" name="frm">
<button hx-target="#foo" id="btn" name="btn"></button>
</form>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L114"
target="_blank" style="float:right; font-size:smaller">source</a>
### fill_form
> fill_form (form:fastcore.xml.FT, obj)
*Fills named items in `form` using attributes in `obj`*
``` python
@dataclass
class TodoItem:
title:str; id:int; done:bool; details:str; opt:str='a'
todo = TodoItem(id=2, title="Profit", done=True, details="Details", opt='b')
check = Label(Input(type="checkbox", cls="checkboxer", name="done", data_foo="bar"), "Done", cls='px-2')
form = Form(Fieldset(Input(cls="char", id="title", value="a"), check, Input(type="hidden", id="id"),
Select(Option(value='a'), Option(value='b'), name='opt'),
Textarea(id='details'), Button("Save"),
name="stuff"))
form = fill_form(form, todo)
assert '<textarea id="details" name="details">Details</textarea>' in to_xml(form)
form
```
``` html
<form>
<fieldset name="stuff">
<input value="Profit" id="title" class="char" name="title">
<label class="px-2">
<input type="checkbox" name="done" data-foo="bar" class="checkboxer" checked="1">
Done
</label>
<input type="hidden" id="id" name="id" value="2">
<select name="opt">
<option value="a"></option>
<option value="b" selected="1"></option>
</select>
<textarea id="details" name="details">Details</textarea>
<button>Save</button>
</fieldset>
</form>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L121"
target="_blank" style="float:right; font-size:smaller">source</a>
### fill_dataclass
> fill_dataclass (src, dest)
*Modifies dataclass in-place and returns it*
``` python
nt = TodoItem('', 0, False, '')
fill_dataclass(todo, nt)
nt
```
TodoItem(title='Profit', id=2, done=True, details='Details', opt='b')
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L127"
target="_blank" style="float:right; font-size:smaller">source</a>
### find_inputs
> find_inputs (e, tags='input', **kw)
*Recursively find all elements in `e` with `tags` and attrs matching
`kw`*
``` python
inps = find_inputs(form, id='title')
test_eq(len(inps), 1)
inps
```
[input((),{'value': 'Profit', 'id': 'title', 'class': 'char', 'name': 'title'})]
You can also use lxml for more sophisticated searching:
``` python
elem = lx.fromstring(to_xml(form))
test_eq(elem.xpath("//input[@id='title']/@value"), ['Profit'])
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L141"
target="_blank" style="float:right; font-size:smaller">source</a>
### **getattr**
> __getattr__ (tag)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L149"
target="_blank" style="float:right; font-size:smaller">source</a>
### html2ft
> html2ft (html, attr1st=False)
*Convert HTML to an `ft` expression*
``` python
h = to_xml(form)
hl_md(html2ft(h), 'python')
```
``` python
Form(
Fieldset(
Input(value='Profit', id='title', name='title', cls='char'),
Label(
Input(type='checkbox', name='done', data_foo='bar', checked='1', cls='checkboxer'),
'Done',
cls='px-2'
),
Input(type='hidden', id='id', name='id', value='2'),
Select(
Option(value='a'),
Option(value='b', selected='1'),
name='opt'
),
Textarea('Details', id='details', name='details'),
Button('Save'),
name='stuff'
)
)
```
``` python
hl_md(html2ft(h, attr1st=True), 'python')
```
``` python
Form(
Fieldset(name='stuff')(
Input(value='Profit', id='title', name='title', cls='char'),
Label(cls='px-2')(
Input(type='checkbox', name='done', data_foo='bar', checked='1', cls='checkboxer'),
'Done'
),
Input(type='hidden', id='id', name='id', value='2'),
Select(name='opt')(
Option(value='a'),
Option(value='b', selected='1')
),
Textarea('Details', id='details', name='details'),
Button('Save')
)
)
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/components.py#L182"
target="_blank" style="float:right; font-size:smaller">source</a>
### sse_message
> sse_message (elm, event='message')
*Convert element `elm` into a format suitable for SSE streaming*
``` python
print(sse_message(Div(P('hi'), P('there'))))
```
event: message
data: <div>
data: <p>hi</p>
data: <p>there</p>
data: </div>
| md |
FastHTML Docs | api/core.html.md | # Core
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
This is the source code to fasthtml. You won’t need to read this unless
you want to understand how things are built behind the scenes, or need
full details of a particular API. The notebook is converted to the
Python module
[fasthtml/core.py](https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py)
using [nbdev](https://nbdev.fast.ai/).
## Imports and utils
``` python
import time
from IPython import display
from enum import Enum
from pprint import pprint
from fastcore.test import *
from starlette.testclient import TestClient
from starlette.requests import Headers
from starlette.datastructures import UploadFile
```
We write source code *first*, and then tests come *after*. The tests
serve as both a means to confirm that the code works and also serves as
working examples. The first declared function, `date`, is an example of
this pattern.
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L42"
target="_blank" style="float:right; font-size:smaller">source</a>
### parsed_date
> parsed_date (s:str)
*Convert `s` to a datetime*
``` python
parsed_date('2pm')
```
datetime.datetime(2024, 9, 17, 14, 0)
``` python
isinstance(date.fromtimestamp(0), date)
```
True
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L47"
target="_blank" style="float:right; font-size:smaller">source</a>
### snake2hyphens
> snake2hyphens (s:str)
*Convert `s` from snake case to hyphenated and capitalised*
``` python
snake2hyphens("snake_case")
```
'Snake-Case'
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L64"
target="_blank" style="float:right; font-size:smaller">source</a>
### HtmxHeaders
> HtmxHeaders (boosted:str|None=None, current_url:str|None=None,
> history_restore_request:str|None=None, prompt:str|None=None,
> request:str|None=None, target:str|None=None,
> trigger_name:str|None=None, trigger:str|None=None)
``` python
def test_request(url: str='/', headers: dict={}, method: str='get') -> Request:
scope = {
'type': 'http',
'method': method,
'path': url,
'headers': Headers(headers).raw,
'query_string': b'',
'scheme': 'http',
'client': ('127.0.0.1', 8000),
'server': ('127.0.0.1', 8000),
}
receive = lambda: {"body": b"", "more_body": False}
return Request(scope, receive)
```
``` python
h = test_request(headers=Headers({'HX-Request':'1'}))
_get_htmx(h.headers)
```
HtmxHeaders(boosted=None, current_url=None, history_restore_request=None, prompt=None, request='1', target=None, trigger_name=None, trigger=None)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L74"
target="_blank" style="float:right; font-size:smaller">source</a>
### str2int
> str2int (s)
*Convert `s` to an `int`*
``` python
str2int('1'),str2int('none')
```
(1, 0)
## Request and response
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L88"
target="_blank" style="float:right; font-size:smaller">source</a>
### str2date
> str2date (s:str)
*`date.fromisoformat` with empty string handling*
``` python
test_eq(_fix_anno(Union[str,None])('a'), 'a')
test_eq(_fix_anno(float)(0.9), 0.9)
test_eq(_fix_anno(int)('1'), 1)
test_eq(_fix_anno(int)(['1','2']), 2)
test_eq(_fix_anno(list[int])(['1','2']), [1,2])
test_eq(_fix_anno(list[int])('1'), [1])
```
``` python
d = dict(k=int, l=List[int])
test_eq(_form_arg('k', "1", d), 1)
test_eq(_form_arg('l', "1", d), [1])
test_eq(_form_arg('l', ["1","2"], d), [1,2])
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L115"
target="_blank" style="float:right; font-size:smaller">source</a>
### HttpHeader
> HttpHeader (k:str, v:str)
``` python
_to_htmx_header('trigger_after_settle')
```
'HX-Trigger-After-Settle'
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L126"
target="_blank" style="float:right; font-size:smaller">source</a>
### HtmxResponseHeaders
> HtmxResponseHeaders (location=None, push_url=None, redirect=None,
> refresh=None, replace_url=None, reswap=None,
> retarget=None, reselect=None, trigger=None,
> trigger_after_settle=None, trigger_after_swap=None)
*HTMX response headers*
``` python
HtmxResponseHeaders(trigger_after_settle='hi')
```
HttpHeader(k='HX-Trigger-After-Settle', v='hi')
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L147"
target="_blank" style="float:right; font-size:smaller">source</a>
### form2dict
> form2dict (form:starlette.datastructures.FormData)
*Convert starlette form data to a dict*
``` python
d = [('a',1),('a',2),('b',0)]
fd = FormData(d)
res = form2dict(fd)
test_eq(res['a'], [1,2])
test_eq(res['b'], 0)
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L152"
target="_blank" style="float:right; font-size:smaller">source</a>
### parse_form
> parse_form (req:starlette.requests.Request)
*Starlette errors on empty multipart forms, so this checks for that
situation*
``` python
async def f(req):
def _f(p:HttpHeader): ...
p = first(_sig(_f).parameters.values())
result = await _from_body(req, p)
return JSONResponse(result.__dict__)
app = Starlette(routes=[Route('/', f, methods=['POST'])])
client = TestClient(app)
d = dict(k='value1',v=['value2','value3'])
response = client.post('/', data=d)
print(response.json())
```
{'k': 'value1', 'v': 'value3'}
``` python
async def f(req): return Response(str(req.query_params.getlist('x')))
app = Starlette(routes=[Route('/', f, methods=['GET'])])
client = TestClient(app)
client.get('/?x=1&x=2').text
```
"['1', '2']"
``` python
def g(req, this:Starlette, a:str, b:HttpHeader): ...
async def f(req):
a = await _wrap_req(req, _sig(g).parameters)
return Response(str(a))
app = Starlette(routes=[Route('/', f, methods=['POST'])])
client = TestClient(app)
response = client.post('/?a=1', data=d)
print(response.text)
```
[<starlette.requests.Request object>, <starlette.applications.Starlette object>, '1', HttpHeader(k='value1', v='value3')]
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L216"
target="_blank" style="float:right; font-size:smaller">source</a>
### flat_xt
> flat_xt (lst)
*Flatten lists*
``` python
x = ft('a',1)
test_eq(flat_xt([x, x, [x,x]]), [x]*4)
test_eq(flat_xt(x), [x])
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L226"
target="_blank" style="float:right; font-size:smaller">source</a>
### Beforeware
> Beforeware (f, skip=None)
*Initialize self. See help(type(self)) for accurate signature.*
## Websockets / SSE
``` python
def on_receive(self, msg:str): return f"Message text was: {msg}"
c = _ws_endp(on_receive)
app = Starlette(routes=[WebSocketRoute('/', _ws_endp(on_receive))])
cli = TestClient(app)
with cli.websocket_connect('/') as ws:
ws.send_text('{"msg":"Hi!"}')
data = ws.receive_text()
assert data == 'Message text was: Hi!'
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L286"
target="_blank" style="float:right; font-size:smaller">source</a>
### EventStream
> EventStream (s)
*Create a text/event-stream response from `s`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L291"
target="_blank" style="float:right; font-size:smaller">source</a>
### signal_shutdown
> signal_shutdown ()
## Routing and application
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L302"
target="_blank" style="float:right; font-size:smaller">source</a>
### WS_RouteX
> WS_RouteX (app, path:str, recv, conn:<built-infunctioncallable>=None,
> disconn:<built-infunctioncallable>=None, name=None,
> middleware=None)
*Initialize self. See help(type(self)) for accurate signature.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L308"
target="_blank" style="float:right; font-size:smaller">source</a>
### uri
> uri (_arg, **kwargs)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L312"
target="_blank" style="float:right; font-size:smaller">source</a>
### decode_uri
> decode_uri (s)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L323"
target="_blank" style="float:right; font-size:smaller">source</a>
### StringConvertor.to_string
> StringConvertor.to_string (value:str)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L331"
target="_blank" style="float:right; font-size:smaller">source</a>
### HTTPConnection.url_path_for
> HTTPConnection.url_path_for (name:str, **path_params)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L366"
target="_blank" style="float:right; font-size:smaller">source</a>
### flat_tuple
> flat_tuple (o)
*Flatten lists*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L414"
target="_blank" style="float:right; font-size:smaller">source</a>
### Redirect
> Redirect (loc)
*Use HTMX or Starlette RedirectResponse as required to redirect to
`loc`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L427"
target="_blank" style="float:right; font-size:smaller">source</a>
### RouteX
> RouteX (app, path:str, endpoint, methods=None, name=None,
> include_in_schema=True, middleware=None)
*Initialize self. See help(type(self)) for accurate signature.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L451"
target="_blank" style="float:right; font-size:smaller">source</a>
### RouterX
> RouterX (app, routes=None, redirect_slashes=True, default=None,
> middleware=None)
*Initialize self. See help(type(self)) for accurate signature.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L476"
target="_blank" style="float:right; font-size:smaller">source</a>
### get_key
> get_key (key=None, fname='.sesskey')
``` python
get_key()
```
'a604e4a2-08e8-462d-aff9-15468891fe09'
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L505"
target="_blank" style="float:right; font-size:smaller">source</a>
### FastHTML
> FastHTML (debug=False, routes=None, middleware=None,
> exception_handlers=None, on_startup=None, on_shutdown=None,
> lifespan=None, hdrs=None, ftrs=None, before=None, after=None,
> ws_hdr=False, ct_hdr=False, surreal=True, htmx=True,
> default_hdrs=True, sess_cls=<class
> 'starlette.middleware.sessions.SessionMiddleware'>,
> secret_key=None, session_cookie='session_', max_age=31536000,
> sess_path='/', same_site='lax', sess_https_only=False,
> sess_domain=None, key_fname='.sesskey', htmlkw=None, **bodykw)
\*Creates an application instance.
**Parameters:**
- **debug** - Boolean indicating if debug tracebacks should be returned
on errors.
- **routes** - A list of routes to serve incoming HTTP and WebSocket
requests.
- **middleware** - A list of middleware to run for every request. A
starlette application will always automatically include two middleware
classes. `ServerErrorMiddleware` is added as the very outermost
middleware, to handle any uncaught errors occurring anywhere in the
entire stack. `ExceptionMiddleware` is added as the very innermost
middleware, to deal with handled exception cases occurring in the
routing or endpoints.
- **exception_handlers** - A mapping of either integer status codes, or
exception class types onto callables which handle the exceptions.
Exception handler callables should be of the form
`handler(request, exc) -> response` and may be either standard
functions, or async functions.
- **on_startup** - A list of callables to run on application startup.
Startup handler callables do not take any arguments, and may be either
standard functions, or async functions.
- **on_shutdown** - A list of callables to run on application shutdown.
Shutdown handler callables do not take any arguments, and may be
either standard functions, or async functions.
- **lifespan** - A lifespan context function, which can be used to
perform startup and shutdown tasks. This is a newer style that
replaces the `on_startup` and `on_shutdown` handlers. Use one or the
other, not both.\*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L549"
target="_blank" style="float:right; font-size:smaller">source</a>
### FastHTML.route
> FastHTML.route (path:str=None, methods=None, name=None,
> include_in_schema=True)
*Add a route at `path`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L569"
target="_blank" style="float:right; font-size:smaller">source</a>
### serve
> serve (appname=None, app='app', host='0.0.0.0', port=None, reload=True,
> reload_includes:list[str]|str|None=None,
> reload_excludes:list[str]|str|None=None)
*Run the app in an async server, with live reload set as the default.*
<table>
<colgroup>
<col style="width: 6%" />
<col style="width: 25%" />
<col style="width: 34%" />
<col style="width: 34%" />
</colgroup>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>appname</td>
<td>NoneType</td>
<td>None</td>
<td>Name of the module</td>
</tr>
<tr class="even">
<td>app</td>
<td>str</td>
<td>app</td>
<td>App instance to be served</td>
</tr>
<tr class="odd">
<td>host</td>
<td>str</td>
<td>0.0.0.0</td>
<td>If host is 0.0.0.0 will convert to localhost</td>
</tr>
<tr class="even">
<td>port</td>
<td>NoneType</td>
<td>None</td>
<td>If port is None it will default to 5001 or the PORT environment
variable</td>
</tr>
<tr class="odd">
<td>reload</td>
<td>bool</td>
<td>True</td>
<td>Default is to reload the app upon code changes</td>
</tr>
<tr class="even">
<td>reload_includes</td>
<td>list[str] | str | None</td>
<td>None</td>
<td>Additional files to watch for changes</td>
</tr>
<tr class="odd">
<td>reload_excludes</td>
<td>list[str] | str | None</td>
<td>None</td>
<td>Files to ignore for changes</td>
</tr>
</tbody>
</table>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L591"
target="_blank" style="float:right; font-size:smaller">source</a>
### Client
> Client (app, url='http://testserver')
*A simple httpx ASGI client that doesn’t require `async`*
``` python
app = FastHTML(routes=[Route('/', lambda _: Response('test'))])
cli = Client(app)
cli.get('/').text
```
'test'
Note that you can also use Starlette’s `TestClient` instead of
FastHTML’s
[`Client`](https://AnswerDotAI.github.io/fasthtml/api/core.html#client).
They should be largely interchangable.
## FastHTML Tests
``` python
def get_cli(app): return app,Client(app),app.route
```
``` python
app,cli,rt = get_cli(FastHTML(secret_key='soopersecret'))
```
``` python
@rt("/hi")
def get(): return 'Hi there'
r = cli.get('/hi')
r.text
```
'Hi there'
``` python
@rt("/hi")
def post(): return 'Postal'
cli.post('/hi').text
```
'Postal'
``` python
@app.get("/hostie")
def show_host(req): return req.headers['host']
cli.get('/hostie').text
```
'testserver'
``` python
@rt
def yoyo(): return 'a yoyo'
cli.post('/yoyo').text
```
'a yoyo'
``` python
@app.get
def autopost(): return Html(Div('Text.', hx_post=yoyo()))
print(cli.get('/autopost').text)
```
<!doctype html>
<html>
<div hx-post="a yoyo">Text.</div>
</html>
``` python
@app.get
def autopost2(): return Html(Body(Div('Text.', cls='px-2', hx_post=show_host.rt(a='b'))))
print(cli.get('/autopost2').text)
```
<!doctype html>
<html>
<body>
<div class="px-2" hx-post="/hostie?a=b">Text.</div>
</body>
</html>
``` python
@app.get
def autoget2(): return Html(Div('Text.', hx_get=show_host))
print(cli.get('/autoget2').text)
```
<!doctype html>
<html>
<div hx-get="/hostie">Text.</div>
</html>
``` python
@rt('/user/{nm}', name='gday')
def get(nm:str=''): return f"Good day to you, {nm}!"
cli.get('/user/Alexis').text
```
'Good day to you, Alexis!'
``` python
@app.get
def autolink(): return Html(Div('Text.', link=uri('gday', nm='Alexis')))
print(cli.get('/autolink').text)
```
<!doctype html>
<html>
<div href="/user/Alexis">Text.</div>
</html>
``` python
@rt('/link')
def get(req): return f"{req.url_for('gday', nm='Alexis')}; {req.url_for('show_host')}"
cli.get('/link').text
```
'http://testserver/user/Alexis; http://testserver/hostie'
``` python
test_eq(app.router.url_path_for('gday', nm='Jeremy'), '/user/Jeremy')
```
``` python
hxhdr = {'headers':{'hx-request':"1"}}
@rt('/ft')
def get(): return Title('Foo'),H1('bar')
txt = cli.get('/ft').text
assert '<title>Foo</title>' in txt and '<h1>bar</h1>' in txt and '<html>' in txt
@rt('/xt2')
def get(): return H1('bar')
txt = cli.get('/xt2').text
assert '<title>FastHTML page</title>' in txt and '<h1>bar</h1>' in txt and '<html>' in txt
assert cli.get('/xt2', **hxhdr).text.strip() == '<h1>bar</h1>'
@rt('/xt3')
def get(): return Html(Head(Title('hi')), Body(P('there')))
txt = cli.get('/xt3').text
assert '<title>FastHTML page</title>' not in txt and '<title>hi</title>' in txt and '<p>there</p>' in txt
```
``` python
@rt('/oops')
def get(nope): return nope
test_warns(lambda: cli.get('/oops?nope=1'))
```
``` python
def test_r(cli, path, exp, meth='get', hx=False, **kwargs):
if hx: kwargs['headers'] = {'hx-request':"1"}
test_eq(getattr(cli, meth)(path, **kwargs).text, exp)
ModelName = str_enum('ModelName', "alexnet", "resnet", "lenet")
fake_db = [{"name": "Foo"}, {"name": "Bar"}]
```
``` python
@rt('/html/{idx}')
async def get(idx:int): return Body(H4(f'Next is {idx+1}.'))
```
``` python
@rt("/models/{nm}")
def get(nm:ModelName): return nm
@rt("/files/{path}")
async def get(path: Path): return path.with_suffix('.txt')
@rt("/items/")
def get(idx:int|None = 0): return fake_db[idx]
@rt("/idxl/")
def get(idx:list[int]): return str(idx)
```
``` python
r = cli.get('/html/1', headers={'hx-request':"1"})
assert '<h4>Next is 2.</h4>' in r.text
test_r(cli, '/models/alexnet', 'alexnet')
test_r(cli, '/files/foo', 'foo.txt')
test_r(cli, '/items/?idx=1', '{"name":"Bar"}')
test_r(cli, '/items/', '{"name":"Foo"}')
assert cli.get('/items/?idx=g').text=='404 Not Found'
assert cli.get('/items/?idx=g').status_code == 404
test_r(cli, '/idxl/?idx=1&idx=2', '[1, 2]')
assert cli.get('/idxl/?idx=1&idx=g').status_code == 404
```
``` python
app = FastHTML()
rt = app.route
cli = TestClient(app)
@app.route(r'/static/{path:path}.jpg')
def index(path:str): return f'got {path}'
cli.get('/static/sub/a.b.jpg').text
```
'got sub/a.b'
``` python
app.chk = 'foo'
```
``` python
@app.get("/booly/")
def _(coming:bool=True): return 'Coming' if coming else 'Not coming'
@app.get("/datie/")
def _(d:parsed_date): return d
@app.get("/ua")
async def _(user_agent:str): return user_agent
@app.get("/hxtest")
def _(htmx): return htmx.request
@app.get("/hxtest2")
def _(foo:HtmxHeaders, req): return foo.request
@app.get("/app")
def _(app): return app.chk
@app.get("/app2")
def _(foo:FastHTML): return foo.chk,HttpHeader("mykey", "myval")
@app.get("/app3")
def _(foo:FastHTML): return HtmxResponseHeaders(location="http://example.org")
@app.get("/app4")
def _(foo:FastHTML): return Redirect("http://example.org")
```
``` python
test_r(cli, '/booly/?coming=true', 'Coming')
test_r(cli, '/booly/?coming=no', 'Not coming')
date_str = "17th of May, 2024, 2p"
test_r(cli, f'/datie/?d={date_str}', '2024-05-17 14:00:00')
test_r(cli, '/ua', 'FastHTML', headers={'User-Agent':'FastHTML'})
test_r(cli, '/hxtest' , '1', headers={'HX-Request':'1'})
test_r(cli, '/hxtest2', '1', headers={'HX-Request':'1'})
test_r(cli, '/app' , 'foo')
```
``` python
r = cli.get('/app2', **hxhdr)
test_eq(r.text, 'foo')
test_eq(r.headers['mykey'], 'myval')
```
``` python
r = cli.get('/app3')
test_eq(r.headers['HX-Location'], 'http://example.org')
```
``` python
r = cli.get('/app4', follow_redirects=False)
test_eq(r.status_code, 303)
```
``` python
r = cli.get('/app4', headers={'HX-Request':'1'})
test_eq(r.headers['HX-Redirect'], 'http://example.org')
```
``` python
@rt
def meta():
return ((Title('hi'),H1('hi')),
(Meta(property='image'), Meta(property='site_name'))
)
t = cli.post('/meta').text
assert re.search(r'<body>\s*<h1>hi</h1>\s*</body>', t)
assert '<meta' in t
```
``` python
@app.post('/profile/me')
def profile_update(username: str): return username
test_r(cli, '/profile/me', 'Alexis', 'post', data={'username' : 'Alexis'})
test_r(cli, '/profile/me', 'Missing required field: username', 'post', data={})
```
``` python
# Example post request with parameter that has a default value
@app.post('/pet/dog')
def pet_dog(dogname: str = None): return dogname
# Working post request with optional parameter
test_r(cli, '/pet/dog', '', 'post', data={})
```
``` python
@dataclass
class Bodie: a:int;b:str
@rt("/bodie/{nm}")
def post(nm:str, data:Bodie):
res = asdict(data)
res['nm'] = nm
return res
@app.post("/bodied/")
def bodied(data:dict): return data
nt = namedtuple('Bodient', ['a','b'])
@app.post("/bodient/")
def bodient(data:nt): return asdict(data)
class BodieTD(TypedDict): a:int;b:str='foo'
@app.post("/bodietd/")
def bodient(data:BodieTD): return data
class Bodie2:
a:int|None; b:str
def __init__(self, a, b='foo'): store_attr()
@app.post("/bodie2/")
def bodie(d:Bodie2): return f"a: {d.a}; b: {d.b}"
```
``` python
from fasthtml.xtend import Titled
```
``` python
# Testing POST with Content-Type: application/json
@app.post("/")
def index(it: Bodie): return Titled("It worked!", P(f"{it.a}, {it.b}"))
s = json.dumps({"b": "Lorem", "a": 15})
response = cli.post('/', headers={"Content-Type": "application/json"}, data=s).text
assert "<title>It worked!</title>" in response and "<p>15, Lorem</p>" in response
```
``` python
# Testing POST with Content-Type: application/json
@app.post("/bodytext")
def index(body): return body
response = cli.post('/bodytext', headers={"Content-Type": "application/json"}, data=s).text
test_eq(response, '{"b": "Lorem", "a": 15}')
```
``` python
d = dict(a=1, b='foo')
test_r(cli, '/bodie/me', '{"a":1,"b":"foo","nm":"me"}', 'post', data=dict(a=1, b='foo', nm='me'))
test_r(cli, '/bodied/', '{"a":"1","b":"foo"}', 'post', data=d)
test_r(cli, '/bodie2/', 'a: 1; b: foo', 'post', data={'a':1})
test_r(cli, '/bodient/', '{"a":"1","b":"foo"}', 'post', data=d)
test_r(cli, '/bodietd/', '{"a":1,"b":"foo"}', 'post', data=d)
```
``` python
@rt("/setsess")
def get(sess, foo:str=''):
now = datetime.now()
sess['auth'] = str(now)
return f'Set to {now}'
@rt("/getsess")
def get(sess): return f'Session time: {sess["auth"]}'
print(cli.get('/setsess').text)
time.sleep(0.01)
cli.get('/getsess').text
```
Set to 2024-09-15 07:09:53.847227
'Session time: 2024-09-15 07:09:53.847227'
``` python
@rt("/sess-first")
def post(sess, name: str):
sess["name"] = name
return str(sess)
cli.post('/sess-first', data={'name': 2})
@rt("/getsess-all")
def get(sess): return sess['name']
test_eq(cli.get('/getsess-all').text, '2')
```
``` python
@rt("/upload")
async def post(uf:UploadFile): return (await uf.read()).decode()
with open('../../CHANGELOG.md', 'rb') as f:
print(cli.post('/upload', files={'uf':f}, data={'msg':'Hello'}).text[:15])
```
# Release notes
``` python
@rt("/form-submit/{list_id}")
def options(list_id: str):
headers = {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'POST',
'Access-Control-Allow-Headers': '*',
}
return Response(status_code=200, headers=headers)
```
``` python
h = cli.options('/form-submit/2').headers
test_eq(h['Access-Control-Allow-Methods'], 'POST')
```
``` python
from fasthtml.authmw import user_pwd_auth
```
``` python
def _not_found(req, exc): return Div('nope')
app,cli,rt = get_cli(FastHTML(exception_handlers={404:_not_found}))
txt = cli.get('/').text
assert '<div>nope</div>' in txt
assert '<!doctype html>' in txt
```
``` python
app,cli,rt = get_cli(FastHTML())
@rt("/{name}/{age}")
def get(name: str, age: int):
return Titled(f"Hello {name.title()}, age {age}")
assert '<title>Hello Uma, age 5</title>' in cli.get('/uma/5').text
assert '404 Not Found' in cli.get('/uma/five').text
```
``` python
auth = user_pwd_auth(testuser='spycraft')
app,cli,rt = get_cli(FastHTML(middleware=[auth]))
@rt("/locked")
def get(auth): return 'Hello, ' + auth
test_eq(cli.get('/locked').text, 'not authenticated')
test_eq(cli.get('/locked', auth=("testuser","spycraft")).text, 'Hello, testuser')
```
``` python
auth = user_pwd_auth(testuser='spycraft')
app,cli,rt = get_cli(FastHTML(middleware=[auth]))
@rt("/locked")
def get(auth): return 'Hello, ' + auth
test_eq(cli.get('/locked').text, 'not authenticated')
test_eq(cli.get('/locked', auth=("testuser","spycraft")).text, 'Hello, testuser')
```
## Extras
``` python
app,cli,rt = get_cli(FastHTML(secret_key='soopersecret'))
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L603"
target="_blank" style="float:right; font-size:smaller">source</a>
### cookie
> cookie (key:str, value='', max_age=None, expires=None, path='/',
> domain=None, secure=False, httponly=False, samesite='lax')
*Create a ‘set-cookie’
[`HttpHeader`](https://AnswerDotAI.github.io/fasthtml/api/core.html#httpheader)*
``` python
@rt("/setcookie")
def get(req): return cookie('now', datetime.now())
@rt("/getcookie")
def get(now:parsed_date): return f'Cookie was set at time {now.time()}'
print(cli.get('/setcookie').text)
time.sleep(0.01)
cli.get('/getcookie').text
```
'Cookie was set at time 07:09:55.075607'
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L621"
target="_blank" style="float:right; font-size:smaller">source</a>
### reg_re_param
> reg_re_param (m, s)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L631"
target="_blank" style="float:right; font-size:smaller">source</a>
### FastHTML.static_route_exts
> FastHTML.static_route_exts (prefix='/', static_path='.', exts='static')
*Add a static route at URL path `prefix` with files from `static_path`
and `exts` defined by `reg_re_param()`*
``` python
reg_re_param("imgext", "ico|gif|jpg|jpeg|webm")
@rt(r'/static/{path:path}{fn}.{ext:imgext}')
def get(fn:str, path:str, ext:str): return f"Getting {fn}.{ext} from /{path}"
test_r(cli, '/static/foo/jph.me.ico', 'Getting jph.me.ico from /foo/')
```
``` python
app.static_route_exts()
assert 'These are the source notebooks for FastHTML' in cli.get('/README.txt').text
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L638"
target="_blank" style="float:right; font-size:smaller">source</a>
### FastHTML.static_route
> FastHTML.static_route (ext='', prefix='/', static_path='.')
*Add a static route at URL path `prefix` with files from `static_path`
and single `ext` (including the ‘.’)*
``` python
app.static_route('.md', static_path='../..')
assert 'THIS FILE WAS AUTOGENERATED' in cli.get('/README.md').text
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L644"
target="_blank" style="float:right; font-size:smaller">source</a>
### MiddlewareBase
> MiddlewareBase ()
*Initialize self. See help(type(self)) for accurate signature.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/core.py#L652"
target="_blank" style="float:right; font-size:smaller">source</a>
### FtResponse
> FtResponse (content, status_code:int=200, headers=None, cls=<class
> 'starlette.responses.HTMLResponse'>,
> media_type:str|None=None, background=None)
*Wrap an FT response with any Starlette `Response`*
``` python
@rt('/ftr')
def get():
cts = Title('Foo'),H1('bar')
return FtResponse(cts, status_code=201, headers={'Location':'/foo/1'})
r = cli.get('/ftr')
test_eq(r.status_code, 201)
test_eq(r.headers['location'], '/foo/1')
txt = r.text
assert '<title>Foo</title>' in txt and '<h1>bar</h1>' in txt and '<html>' in txt
```
| md |
FastHTML Docs | api/fastapp.html.md | # fastapp
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
Usage can be summarized as:
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt('/')
def get(): return Titled("A demo of fast_app()@")
serve()
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/fastapp.py#L38"
target="_blank" style="float:right; font-size:smaller">source</a>
### fast_app
> fast_app (db_file:Optional[str]=None, render:Optional[<built-
> infunctioncallable>]=None, hdrs:Optional[tuple]=None,
> ftrs:Optional[tuple]=None, tbls:Optional[dict]=None,
> before:Union[tuple,NoneType,fasthtml.core.Beforeware]=None,
> middleware:Optional[tuple]=None, live:bool=False,
> debug:bool=False, routes:Optional[tuple]=None,
> exception_handlers:Optional[dict]=None,
> on_startup:Optional[<built-infunctioncallable>]=None,
> on_shutdown:Optional[<built-infunctioncallable>]=None,
> lifespan:Optional[<built-infunctioncallable>]=None,
> default_hdrs=True, pico:Optional[bool]=None,
> surreal:Optional[bool]=True, htmx:Optional[bool]=True,
> ws_hdr:bool=False, secret_key:Optional[str]=None,
> key_fname:str='.sesskey', session_cookie:str='session_',
> max_age:int=31536000, sess_path:str='/', same_site:str='lax',
> sess_https_only:bool=False, sess_domain:Optional[str]=None,
> htmlkw:Optional[dict]=None, bodykw:Optional[dict]=None,
> reload_attempts:Optional[int]=1,
> reload_interval:Optional[int]=1000, static_path:str='.',
> **kwargs)
*Create a FastHTML or FastHTMLWithLiveReload app.*
<table>
<colgroup>
<col style="width: 6%" />
<col style="width: 25%" />
<col style="width: 34%" />
<col style="width: 34%" />
</colgroup>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>db_file</td>
<td>Optional</td>
<td>None</td>
<td>Database file name, if needed</td>
</tr>
<tr class="even">
<td>render</td>
<td>Optional</td>
<td>None</td>
<td>Function used to render default database class</td>
</tr>
<tr class="odd">
<td>hdrs</td>
<td>Optional</td>
<td>None</td>
<td>Additional FT elements to add to </td>
</tr>
<tr class="even">
<td>ftrs</td>
<td>Optional</td>
<td>None</td>
<td>Additional FT elements to add to end of </td>
</tr>
<tr class="odd">
<td>tbls</td>
<td>Optional</td>
<td>None</td>
<td>Experimental mapping from DB table names to dict table
definitions</td>
</tr>
<tr class="even">
<td>before</td>
<td>Union</td>
<td>None</td>
<td>Functions to call prior to calling handler</td>
</tr>
<tr class="odd">
<td>middleware</td>
<td>Optional</td>
<td>None</td>
<td>Standard Starlette middleware</td>
</tr>
<tr class="even">
<td>live</td>
<td>bool</td>
<td>False</td>
<td>Enable live reloading</td>
</tr>
<tr class="odd">
<td>debug</td>
<td>bool</td>
<td>False</td>
<td>Passed to Starlette, indicating if debug tracebacks should be
returned on errors</td>
</tr>
<tr class="even">
<td>routes</td>
<td>Optional</td>
<td>None</td>
<td>Passed to Starlette</td>
</tr>
<tr class="odd">
<td>exception_handlers</td>
<td>Optional</td>
<td>None</td>
<td>Passed to Starlette</td>
</tr>
<tr class="even">
<td>on_startup</td>
<td>Optional</td>
<td>None</td>
<td>Passed to Starlette</td>
</tr>
<tr class="odd">
<td>on_shutdown</td>
<td>Optional</td>
<td>None</td>
<td>Passed to Starlette</td>
</tr>
<tr class="even">
<td>lifespan</td>
<td>Optional</td>
<td>None</td>
<td>Passed to Starlette</td>
</tr>
<tr class="odd">
<td>default_hdrs</td>
<td>bool</td>
<td>True</td>
<td>Include default FastHTML headers such as HTMX script?</td>
</tr>
<tr class="even">
<td>pico</td>
<td>Optional</td>
<td>None</td>
<td>Include PicoCSS header?</td>
</tr>
<tr class="odd">
<td>surreal</td>
<td>Optional</td>
<td>True</td>
<td>Include surreal.js/scope headers?</td>
</tr>
<tr class="even">
<td>htmx</td>
<td>Optional</td>
<td>True</td>
<td>Include HTMX header?</td>
</tr>
<tr class="odd">
<td>ws_hdr</td>
<td>bool</td>
<td>False</td>
<td>Include HTMX websocket extension header?</td>
</tr>
<tr class="even">
<td>secret_key</td>
<td>Optional</td>
<td>None</td>
<td>Signing key for sessions</td>
</tr>
<tr class="odd">
<td>key_fname</td>
<td>str</td>
<td>.sesskey</td>
<td>Session cookie signing key file name</td>
</tr>
<tr class="even">
<td>session_cookie</td>
<td>str</td>
<td>session_</td>
<td>Session cookie name</td>
</tr>
<tr class="odd">
<td>max_age</td>
<td>int</td>
<td>31536000</td>
<td>Session cookie expiry time</td>
</tr>
<tr class="even">
<td>sess_path</td>
<td>str</td>
<td>/</td>
<td>Session cookie path</td>
</tr>
<tr class="odd">
<td>same_site</td>
<td>str</td>
<td>lax</td>
<td>Session cookie same site policy</td>
</tr>
<tr class="even">
<td>sess_https_only</td>
<td>bool</td>
<td>False</td>
<td>Session cookie HTTPS only?</td>
</tr>
<tr class="odd">
<td>sess_domain</td>
<td>Optional</td>
<td>None</td>
<td>Session cookie domain</td>
</tr>
<tr class="even">
<td>htmlkw</td>
<td>Optional</td>
<td>None</td>
<td>Attrs to add to the HTML tag</td>
</tr>
<tr class="odd">
<td>bodykw</td>
<td>Optional</td>
<td>None</td>
<td>Attrs to add to the Body tag</td>
</tr>
<tr class="even">
<td>reload_attempts</td>
<td>Optional</td>
<td>1</td>
<td>Number of reload attempts when live reloading</td>
</tr>
<tr class="odd">
<td>reload_interval</td>
<td>Optional</td>
<td>1000</td>
<td>Time between reload attempts in ms</td>
</tr>
<tr class="even">
<td>static_path</td>
<td>str</td>
<td>.</td>
<td>Where the static file route points to, defaults to root dir</td>
</tr>
<tr class="odd">
<td>kwargs</td>
<td></td>
<td></td>
<td></td>
</tr>
<tr class="even">
<td><strong>Returns</strong></td>
<td><strong>Any</strong></td>
<td></td>
<td></td>
</tr>
</tbody>
</table>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/fastapp.py#L97"
target="_blank" style="float:right; font-size:smaller">source</a>
### PageX
> PageX (title, *con)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/fastapp.py#L96"
target="_blank" style="float:right; font-size:smaller">source</a>
### ContainerX
> ContainerX (*cs, **kwargs)
| md |
FastHTML Docs | api/js.html.md | # Javascript examples
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
To expedite fast development, FastHTML comes with several built-in
Javascript and formatting components. These are largely provided to
demonstrate FastHTML JS patterns. There’s far too many JS libs for
FastHTML to wrap them all, and as shown here the code to add FastHTML
support is very simple anyway.
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L15"
target="_blank" style="float:right; font-size:smaller">source</a>
### light_media
> light_media (css:str)
*Render light media for day mode views*
<table>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>css</td>
<td>str</td>
<td>CSS to be included in the light media query</td>
</tr>
</tbody>
</table>
``` python
light_media('.body {color: green;}')
```
``` html
<style>@media (prefers-color-scheme: light) {.body {color: green;}}</style>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L22"
target="_blank" style="float:right; font-size:smaller">source</a>
### dark_media
> dark_media (css:str)
*Render dark media for nught mode views*
<table>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>css</td>
<td>str</td>
<td>CSS to be included in the dark media query</td>
</tr>
</tbody>
</table>
``` python
dark_media('.body {color: white;}')
```
``` html
<style>@media (prefers-color-scheme: dark) {.body {color: white;}}</style>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L35"
target="_blank" style="float:right; font-size:smaller">source</a>
### MarkdownJS
> MarkdownJS (sel='.marked')
*Implements browser-based markdown rendering.*
<table>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>sel</td>
<td>str</td>
<td>.marked</td>
<td>CSS selector for markdown elements</td>
</tr>
</tbody>
</table>
Usage example
[here](../tutorials/quickstart_for_web_devs.html#rendering-markdown).
``` python
__file__ = '../../fasthtml/katex.js'
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L43"
target="_blank" style="float:right; font-size:smaller">source</a>
### KatexMarkdownJS
> KatexMarkdownJS (sel='.marked', inline_delim='$', display_delim='$$',
> math_envs=None)
<table>
<colgroup>
<col style="width: 6%" />
<col style="width: 25%" />
<col style="width: 34%" />
<col style="width: 34%" />
</colgroup>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>sel</td>
<td>str</td>
<td>.marked</td>
<td>CSS selector for markdown elements</td>
</tr>
<tr class="even">
<td>inline_delim</td>
<td>str</td>
<td>$</td>
<td>Delimiter for inline math</td>
</tr>
<tr class="odd">
<td>display_delim</td>
<td>str</td>
<td>$$</td>
<td>Delimiter for long math</td>
</tr>
<tr class="even">
<td>math_envs</td>
<td>NoneType</td>
<td>None</td>
<td>List of environments to render as display math</td>
</tr>
</tbody>
</table>
KatexMarkdown usage example:
``` python
longexample = r"""
Long example:
$$\begin{array}{c}
\nabla \times \vec{\mathbf{B}} -\, \frac1c\, \frac{\partial\vec{\mathbf{E}}}{\partial t} &
= \frac{4\pi}{c}\vec{\mathbf{j}} \nabla \cdot \vec{\mathbf{E}} & = 4 \pi \rho \\
\nabla \times \vec{\mathbf{E}}\, +\, \frac1c\, \frac{\partial\vec{\mathbf{B}}}{\partial t} & = \vec{\mathbf{0}} \\
\nabla \cdot \vec{\mathbf{B}} & = 0
\end{array}$$
"""
app, rt = fast_app(hdrs=[KatexMarkdownJS()])
@rt('/')
def get():
return Titled("Katex Examples",
# Assigning 'marked' class to components renders content as markdown
P(cls='marked')("Inline example: $\sqrt{3x-1}+(1+x)^2$"),
Div(cls='marked')(longexample)
)
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L58"
target="_blank" style="float:right; font-size:smaller">source</a>
### HighlightJS
> HighlightJS (sel='pre code', langs:str|list|tuple='python', light='atom-
> one-light', dark='atom-one-dark')
*Implements browser-based syntax highlighting. Usage example
[here](../tutorials/quickstart_for_web_devs.html#code-highlighting).*
<table>
<colgroup>
<col style="width: 6%" />
<col style="width: 25%" />
<col style="width: 34%" />
<col style="width: 34%" />
</colgroup>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>sel</td>
<td>str</td>
<td>pre code</td>
<td>CSS selector for code elements. Default is industry standard, be
careful before adjusting it</td>
</tr>
<tr class="even">
<td>langs</td>
<td>str | list | tuple</td>
<td>python</td>
<td>Language(s) to highlight</td>
</tr>
<tr class="odd">
<td>light</td>
<td>str</td>
<td>atom-one-light</td>
<td>Light theme</td>
</tr>
<tr class="even">
<td>dark</td>
<td>str</td>
<td>atom-one-dark</td>
<td>Dark theme</td>
</tr>
</tbody>
</table>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py#L82"
target="_blank" style="float:right; font-size:smaller">source</a>
### SortableJS
> SortableJS (sel='.sortable', ghost_class='blue-background-class')
<table>
<colgroup>
<col style="width: 6%" />
<col style="width: 25%" />
<col style="width: 34%" />
<col style="width: 34%" />
</colgroup>
<thead>
<tr class="header">
<th></th>
<th><strong>Type</strong></th>
<th><strong>Default</strong></th>
<th><strong>Details</strong></th>
</tr>
</thead>
<tbody>
<tr class="odd">
<td>sel</td>
<td>str</td>
<td>.sortable</td>
<td>CSS selector for sortable elements</td>
</tr>
<tr class="even">
<td>ghost_class</td>
<td>str</td>
<td>blue-background-class</td>
<td>When an element is being dragged, this is the class used to
distinguish it from the rest</td>
</tr>
</tbody>
</table>
| md |
FastHTML Docs | api/oauth.html.md | # OAuth
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
This is not yet thoroughly tested. See the [docs
page](https://docs.fastht.ml/explains/oauth.html) for an explanation of
how to use this.
``` python
from IPython.display import Markdown
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L22"
target="_blank" style="float:right; font-size:smaller">source</a>
### GoogleAppClient
> GoogleAppClient (client_id, client_secret, code=None, scope=None,
> **kwargs)
*A `WebApplicationClient` for Google oauth2*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L40"
target="_blank" style="float:right; font-size:smaller">source</a>
### GitHubAppClient
> GitHubAppClient (client_id, client_secret, code=None, scope=None,
> **kwargs)
*A `WebApplicationClient` for GitHub oauth2*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L52"
target="_blank" style="float:right; font-size:smaller">source</a>
### HuggingFaceClient
> HuggingFaceClient (client_id, client_secret, code=None, scope=None,
> state=None, **kwargs)
*A `WebApplicationClient` for HuggingFace oauth2*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L66"
target="_blank" style="float:right; font-size:smaller">source</a>
### DiscordAppClient
> DiscordAppClient (client_id, client_secret, is_user=False, perms=0,
> scope=None, **kwargs)
*A `WebApplicationClient` for Discord oauth2*
``` python
cli = GoogleAppClient.from_file('/Users/jhoward/git/nbs/oauth-test/client_secret.json')
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L93"
target="_blank" style="float:right; font-size:smaller">source</a>
### WebApplicationClient.login_link
> WebApplicationClient.login_link (redirect_uri, scope=None, state=None)
*Get a login link for this client*
Generating a login link that sends the user to the OAuth provider is
done with `client.login_link()`.
It can sometimes be useful to pass state to the OAuth provider, so that
when the user returns you can pick up where they left off. This can be
done by passing the `state` parameter.
``` python
redir='http://localhost:8000/redirect'
cli.login_link(redir)
```
'https://accounts.google.com/o/oauth2/v2/auth?response_type=code&client_id=457681028261-5i71skrhb7ko4l8mlug5i0230q980do7.apps.googleusercontent.com&redirect_uri=http%3A%2F%2Flocalhost%3A8000%2Fredirect&scope=openid+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile'
``` python
def login_md(cli, redirect_uri, scope=None, state=None):
"Display login link in notebook (for testing)"
return Markdown(f'[login]({cli.login_link(redirect_uri, scope, state=state)})')
```
``` python
login_md(cli, redir, state='test_state')
```
[login](https://accounts.google.com/o/oauth2/v2/auth?response_type=code&client_id=457681028261-5i71skrhb7ko4l8mlug5i0230q980do7.apps.googleusercontent.com&redirect_uri=http%3A%2F%2Flocalhost%3A8000%2Fredirect&scope=openid+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile&state=test_state)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L101"
target="_blank" style="float:right; font-size:smaller">source</a>
### \_AppClient.parse_response
> _AppClient.parse_response (code, redirect_uri)
*Get the token from the oauth2 server response*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L110"
target="_blank" style="float:right; font-size:smaller">source</a>
### decode
> decode (code_url)
``` python
code_url = 'http://localhost:8000/redirect?state=test_state&code=4%2F0AQlEd8xCOSfc7yjmmylO6BTVgWtAmji4GkfITsWecq0CXlm-8wBRgwNmkDmXQEdOqw0REQ&scope=email+profile+openid+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.profile+https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fuserinfo.email&authuser=0&hd=answer.ai&prompt=consent'
code,state,redir = decode(code_url)
```
``` python
cli.parse_response(code, redir)
print(state)
```
test_state
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L117"
target="_blank" style="float:right; font-size:smaller">source</a>
### \_AppClient.get_info
> _AppClient.get_info (token=None)
*Get the info for authenticated user*
``` python
info
```
{'sub': '100000802623412015452',
'name': 'Jeremy Howard',
'given_name': 'Jeremy',
'family_name': 'Howard',
'picture': 'https://lh3.googleusercontent.com/a/ACg8ocID3bYiwh1wJNVjvlSUy0dGxvXbNjDt1hdhypQDinDf28DfEA=s96-c',
'email': '[email protected]',
'email_verified': True,
'hd': 'answer.ai'}
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L125"
target="_blank" style="float:right; font-size:smaller">source</a>
### \_AppClient.retr_info
> _AppClient.retr_info (code, redirect_uri)
*Combines `parse_response` and `get_info`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L132"
target="_blank" style="float:right; font-size:smaller">source</a>
### \_AppClient.retr_id
> _AppClient.retr_id (code, redirect_uri)
*Call `retr_info` and then return id/subscriber value*
After logging in via the provider, the user will be redirected back to
the supplied redirect URL. The request to this URL will contain a `code`
parameter, which is used to get an access token and fetch the user’s
profile information. See [the explanation
here](https://docs.fastht.ml/explains/oauth.html) for a worked example.
You can either:
- Use client.retr_info(code) to get all the profile information, or
- Use client.retr_id(code) to get just the user’s ID.
After either of these calls, you can also access the access token (used
to revoke access, for example) with `client.token["access_token"]`.
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/oauth.py#L137"
target="_blank" style="float:right; font-size:smaller">source</a>
### OAuth
> OAuth (app, cli, skip=None, redir_path='/redirect',
> logout_path='/logout', login_path='/login')
*Initialize self. See help(type(self)) for accurate signature.*
| md |
FastHTML Docs | api/pico.html.md | # Pico.css components
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
`picocondlink` is the class-conditional css `link` tag, and `picolink`
is the regular tag.
``` python
show(picocondlink)
```
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@latest/css/pico.conditional.min.css">
<style>:root { --pico-font-size: 100%; }</style>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L30"
target="_blank" style="float:right; font-size:smaller">source</a>
### set_pico_cls
> set_pico_cls ()
Run this to make jupyter outputs styled with pico:
``` python
set_pico_cls()
```
<IPython.core.display.Javascript object>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L49"
target="_blank" style="float:right; font-size:smaller">source</a>
### Card
> Card (*c, header=None, footer=None, target_id=None, hx_vals=None,
> id=None, cls=None, title=None, style=None, accesskey=None,
> contenteditable=None, dir=None, draggable=None, enterkeyhint=None,
> hidden=None, inert=None, inputmode=None, lang=None, popover=None,
> spellcheck=None, tabindex=None, translate=None, hx_get=None,
> hx_post=None, hx_put=None, hx_delete=None, hx_patch=None,
> hx_trigger=None, hx_target=None, hx_swap=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A PicoCSS Card, implemented as an Article with optional Header and
Footer*
``` python
show(Card('body', header=P('head'), footer=P('foot')))
```
<article>
<header><p>head</p>
</header>
body
<footer><p>foot</p>
</footer>
</article>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L57"
target="_blank" style="float:right; font-size:smaller">source</a>
### Group
> Group (*c, target_id=None, hx_vals=None, id=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None, hx_include=None,
> hx_select=None, hx_select_oob=None, hx_indicator=None,
> hx_push_url=None, hx_confirm=None, hx_disable=None,
> hx_replace_url=None, hx_disabled_elt=None, hx_ext=None,
> hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A PicoCSS Group, implemented as a Fieldset with role ‘group’*
``` python
show(Group(Input(), Button("Save")))
```
<fieldset role="group">
<input>
<button>Save</button>
</fieldset>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L63"
target="_blank" style="float:right; font-size:smaller">source</a>
### Search
> Search (*c, target_id=None, hx_vals=None, id=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None, hx_include=None,
> hx_select=None, hx_select_oob=None, hx_indicator=None,
> hx_push_url=None, hx_confirm=None, hx_disable=None,
> hx_replace_url=None, hx_disabled_elt=None, hx_ext=None,
> hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None,
> hx_prompt=None, hx_request=None, hx_sync=None, hx_validate=None,
> **kwargs)
*A PicoCSS Search, implemented as a Form with role ‘search’*
``` python
show(Search(Input(type="search"), Button("Search")))
```
<form enctype="multipart/form-data" role="search">
<input type="search">
<button>Search</button>
</form>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L69"
target="_blank" style="float:right; font-size:smaller">source</a>
### Grid
> Grid (*c, cls='grid', target_id=None, hx_vals=None, id=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None, hx_include=None,
> hx_select=None, hx_select_oob=None, hx_indicator=None,
> hx_push_url=None, hx_confirm=None, hx_disable=None,
> hx_replace_url=None, hx_disabled_elt=None, hx_ext=None,
> hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A PicoCSS Grid, implemented as child Divs in a Div with class ‘grid’*
``` python
colors = [Input(type="color", value=o) for o in ('#e66465', '#53d2c5', '#f6b73c')]
show(Grid(*colors))
```
<div class="grid">
<div><input type="color" value="#e66465">
</div>
<div><input type="color" value="#53d2c5">
</div>
<div><input type="color" value="#f6b73c">
</div>
</div>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L76"
target="_blank" style="float:right; font-size:smaller">source</a>
### DialogX
> DialogX (*c, open=None, header=None, footer=None, id=None,
> target_id=None, hx_vals=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A PicoCSS Dialog, with children inside a Card*
``` python
hdr = Div(Button(aria_label="Close", rel="prev"), P('confirm'))
ftr = Div(Button('Cancel', cls="secondary"), Button('Confirm'))
d = DialogX('thank you!', header=hdr, footer=ftr, open=None, id='dlgtest')
# use js or htmx to display modal
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L83"
target="_blank" style="float:right; font-size:smaller">source</a>
### Container
> Container (*args, target_id=None, hx_vals=None, id=None, cls=None,
> title=None, style=None, accesskey=None, contenteditable=None,
> dir=None, draggable=None, enterkeyhint=None, hidden=None,
> inert=None, inputmode=None, lang=None, popover=None,
> spellcheck=None, tabindex=None, translate=None, hx_get=None,
> hx_post=None, hx_put=None, hx_delete=None, hx_patch=None,
> hx_trigger=None, hx_target=None, hx_swap=None,
> hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A PicoCSS Container, implemented as a Main with class ‘container’*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/pico.py#L88"
target="_blank" style="float:right; font-size:smaller">source</a>
### PicoBusy
> PicoBusy ()
| md |
FastHTML Docs | api/svg.html.md | # SVG
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
``` python
from nbdev.showdoc import show_doc
```
You can create SVGs directly from strings, for instance (as always, use
`NotStr` or `Safe` to tell FastHTML to not escape the text):
``` python
svg = '<svg width="50" height="50"><circle cx="20" cy="20" r="15" fill="red"></circle></svg>'
show(NotStr(svg))
```
<svg width="50" height="50"><circle cx="20" cy="20" r="15" fill="red"></circle></svg>
You can also use libraries such as
[fa6-icons](https://answerdotai.github.io/fa6-icons/).
To create and modify SVGs using a Python API, use the FT elements in
`fasthtml.svg`, discussed below.
**Note**: `fasthtml.common` does NOT automatically export SVG elements.
To get access to them, you need to import `fasthtml.svg` like so
``` python
from fasthtml.svg import *
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L34"
target="_blank" style="float:right; font-size:smaller">source</a>
### Svg
> Svg (*args, viewBox=None, h=None, w=None, height=None, width=None,
> **kwargs)
*An SVG tag; xmlns is added automatically, and viewBox defaults to
height and width if not provided*
To create your own SVGs, use `SVG`. It will automatically set the
`viewBox` from height and width if not provided.
All of our shapes will have some convenient kwargs added by using
[`ft_svg`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#ft_svg):
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L43"
target="_blank" style="float:right; font-size:smaller">source</a>
### ft_svg
> ft_svg (tag:str, *c, transform=None, opacity=None, clip=None, mask=None,
> filter=None, vector_effect=None, pointer_events=None,
> target_id=None, hx_vals=None, id=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None, hx_include=None,
> hx_select=None, hx_select_oob=None, hx_indicator=None,
> hx_push_url=None, hx_confirm=None, hx_disable=None,
> hx_replace_url=None, hx_disabled_elt=None, hx_ext=None,
> hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None,
> hx_prompt=None, hx_request=None, hx_sync=None, hx_validate=None)
*Create a standard `FT` element with some SVG-specific attrs*
## Basic shapes
We’ll define a simple function to display SVG shapes in this notebook:
``` python
def demo(el, h=50, w=50): return show(Svg(h=h,w=w)(el))
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L51"
target="_blank" style="float:right; font-size:smaller">source</a>
### Rect
> Rect (width, height, x=0, y=0, fill=None, stroke=None, stroke_width=None,
> rx=None, ry=None, transform=None, opacity=None, clip=None,
> mask=None, filter=None, vector_effect=None, pointer_events=None,
> target_id=None, hx_vals=None, id=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None, hx_include=None,
> hx_select=None, hx_select_oob=None, hx_indicator=None,
> hx_push_url=None, hx_confirm=None, hx_disable=None,
> hx_replace_url=None, hx_disabled_elt=None, hx_ext=None,
> hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None)
*A standard SVG `rect` element*
All our shapes just create regular `FT` elements. The only extra
functionality provided by most of them is to add additional defined
kwargs to improve auto-complete in IDEs and notebooks, and re-order
parameters so that positional args can also be used to save a bit of
typing, e.g:
``` python
demo(Rect(30, 30, fill='blue', rx=8, ry=8))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<rect width="30" height="30" x="0" y="0" fill="blue" rx="8" ry="8"></rect>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L58"
target="_blank" style="float:right; font-size:smaller">source</a>
### Circle
> Circle (r, cx=0, cy=0, fill=None, stroke=None, stroke_width=None,
> transform=None, opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None)
*A standard SVG `circle` element*
``` python
demo(Circle(20, 25, 25, stroke='red', stroke_width=3))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<circle r="20" cx="25" cy="25" stroke="red" stroke-width="3"></circle>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L64"
target="_blank" style="float:right; font-size:smaller">source</a>
### Ellipse
> Ellipse (rx, ry, cx=0, cy=0, fill=None, stroke=None, stroke_width=None,
> transform=None, opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None)
*A standard SVG `ellipse` element*
``` python
demo(Ellipse(20, 10, 25, 25))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<ellipse rx="20" ry="10" cx="25" cy="25"></ellipse>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L69"
target="_blank" style="float:right; font-size:smaller">source</a>
### transformd
> transformd (translate=None, scale=None, rotate=None, skewX=None,
> skewY=None, matrix=None)
*Create an SVG `transform` kwarg dict*
``` python
rot = transformd(rotate=(45, 25, 25))
rot
```
{'transform': 'rotate(45,25,25)'}
``` python
demo(Ellipse(20, 10, 25, 25, **rot))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<ellipse rx="20" ry="10" cx="25" cy="25" transform="rotate(45,25,25)"></ellipse>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L82"
target="_blank" style="float:right; font-size:smaller">source</a>
### Line
> Line (x1, y1, x2=0, y2=0, stroke='black', w=None, stroke_width=1,
> transform=None, opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None, hx_request=None,
> hx_sync=None, hx_validate=None)
*A standard SVG `line` element*
``` python
demo(Line(20, 30, w=3))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<line x1="20" y1="30" x2="0" y2="0" stroke="black" stroke-width="3"></line>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L89"
target="_blank" style="float:right; font-size:smaller">source</a>
### Polyline
> Polyline (*args, points=None, fill=None, stroke=None, stroke_width=None,
> transform=None, opacity=None, clip=None, mask=None,
> filter=None, vector_effect=None, pointer_events=None,
> target_id=None, hx_vals=None, id=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None,
> hx_history_elt=None, hx_inherit=None, hx_params=None,
> hx_preserve=None, hx_prompt=None, hx_request=None,
> hx_sync=None, hx_validate=None)
*A standard SVG `polyline` element*
``` python
demo(Polyline((0,0), (10,10), (20,0), (30,10), (40,0),
fill='yellow', stroke='blue', stroke_width=2))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<polyline points="0,0 10,10 20,0 30,10 40,0" fill="yellow" stroke="blue" stroke-width="2"></polyline>
</svg>
``` python
demo(Polyline(points='0,0 10,10 20,0 30,10 40,0', fill='purple', stroke_width=2))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<polyline points="0,0 10,10 20,0 30,10 40,0" fill="purple" stroke-width="2"></polyline>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L96"
target="_blank" style="float:right; font-size:smaller">source</a>
### Polygon
> Polygon (*args, points=None, fill=None, stroke=None, stroke_width=None,
> transform=None, opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None)
*A standard SVG `polygon` element*
``` python
demo(Polygon((25,5), (43.3,15), (43.3,35), (25,45), (6.7,35), (6.7,15),
fill='lightblue', stroke='navy', stroke_width=2))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<polygon points="25,5 43.3,15 43.3,35 25,45 6.7,35 6.7,15" fill="lightblue" stroke="navy" stroke-width="2"></polygon>
</svg>
``` python
demo(Polygon(points='25,5 43.3,15 43.3,35 25,45 6.7,35 6.7,15',
fill='lightgreen', stroke='darkgreen', stroke_width=2))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<polygon points="25,5 43.3,15 43.3,35 25,45 6.7,35 6.7,15" fill="lightgreen" stroke="darkgreen" stroke-width="2"></polygon>
</svg>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L103"
target="_blank" style="float:right; font-size:smaller">source</a>
### Text
> Text (*args, x=0, y=0, font_family=None, font_size=None, fill=None,
> text_anchor=None, dominant_baseline=None, font_weight=None,
> font_style=None, text_decoration=None, transform=None,
> opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None, hx_request=None,
> hx_sync=None, hx_validate=None)
*A standard SVG `text` element*
``` python
demo(Text("Hello!", x=10, y=30))
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<text x="10" y="30">Hello!</text>
</svg>
## Paths
Paths in SVGs are more complex, so we add a small (optional) fluent
interface for constructing them:
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L111"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT
> PathFT (tag:str, cs:tuple, attrs:dict=None, void_=False, **kwargs)
*A ‘Fast Tag’ structure, containing `tag`,`children`,and `attrs`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L159"
target="_blank" style="float:right; font-size:smaller">source</a>
### Path
> Path (d='', fill=None, stroke=None, stroke_width=None, transform=None,
> opacity=None, clip=None, mask=None, filter=None,
> vector_effect=None, pointer_events=None, target_id=None,
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None, hx_request=None,
> hx_sync=None, hx_validate=None)
*Create a standard `path` SVG element. This is a special object*
Let’s create a square shape, but using
[`Path`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#path)
instead of
[`Rect`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#rect):
- M(10, 10): Move to starting point (10, 10)
- L(40, 10): Line to (40, 10) - top edge
- L(40, 40): Line to (40, 40) - right edge
- L(10, 40): Line to (10, 40) - bottom edge
- Z(): Close path - connects back to start
M = Move to, L = Line to, Z = Close path
``` python
demo(Path(fill='none', stroke='purple', stroke_width=2
).M(10, 10).L(40, 10).L(40, 40).L(10, 40).Z())
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<path fill="none" stroke="purple" stroke-width="2" d="M10 10 L40 10 L40 40 L10 40 Z"></path>
</svg>
Using curves we can create a spiral:
``` python
p = (Path(fill='none', stroke='purple', stroke_width=2)
.M(25, 25)
.C(25, 25, 20, 20, 30, 20)
.C(40, 20, 40, 30, 30, 30)
.C(20, 30, 20, 15, 35, 15)
.C(50, 15, 50, 35, 25, 35)
.C(0, 35, 0, 10, 40, 10)
.C(80, 10, 80, 40, 25, 40))
demo(p, 50, 100)
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 100 50" height="50" width="100">
<path fill="none" stroke="purple" stroke-width="2" d="M25 25 C25 25 20 20 30 20 C40 20 40 30 30 30 C20 30 20 15 35 15 C50 15 50 35 25 35 C0 35 0 10 40 10 C80 10 80 40 25 40"></path>
</svg>
Using arcs and curves we can create a map marker icon:
``` python
p = (Path(fill='red')
.M(25,45)
.C(25,45,10,35,10,25)
.A(15,15,0,1,1,40,25)
.C(40,35,25,45,25,45)
.Z())
demo(p)
```
<svg xmlns="http://www.w3.org/2000/svg" viewbox="0 0 50 50" height="50" width="50">
<path fill="red" d="M25 45 C25 45 10 35 10 25 A15 15 0 1 1 40 25 C40 35 25 45 25 45 Z"></path>
</svg>
Behind the scenes it’s just creating regular SVG path `d` attr – you can
pass `d` in directly if you prefer.
``` python
print(p.d)
```
M25 45 C25 45 10 35 10 25 A15 15 0 1 1 40 25 C40 35 25 45 25 45 Z
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L117"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.M
> PathFT.M (x, y)
*Move to.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L121"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.L
> PathFT.L (x, y)
*Line to.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L125"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.H
> PathFT.H (x)
*Horizontal line to.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L129"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.V
> PathFT.V (y)
*Vertical line to.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L133"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.Z
> PathFT.Z ()
*Close path.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L137"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.C
> PathFT.C (x1, y1, x2, y2, x, y)
*Cubic Bézier curve.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L141"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.S
> PathFT.S (x2, y2, x, y)
*Smooth cubic Bézier curve.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L145"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.Q
> PathFT.Q (x1, y1, x, y)
*Quadratic Bézier curve.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L149"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.T
> PathFT.T (x, y)
*Smooth quadratic Bézier curve.*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L153"
target="_blank" style="float:right; font-size:smaller">source</a>
### PathFT.A
> PathFT.A (rx, ry, x_axis_rotation, large_arc_flag, sweep_flag, x, y)
*Elliptical Arc.*
## HTMX helpers
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L167"
target="_blank" style="float:right; font-size:smaller">source</a>
### SvgOob
> SvgOob (*args, **kwargs)
*Wraps an SVG shape as required for an HTMX OOB swap*
When returning an SVG shape out-of-band (OOB) in HTMX, you need to wrap
it with
[`SvgOob`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#svgoob)
to have it appear correctly.
([`SvgOob`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#svgoob)
is just a shortcut for `Template(Svg(...))`, which is the trick that
makes SVG OOB swaps work.)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/svg.py#L172"
target="_blank" style="float:right; font-size:smaller">source</a>
### SvgInb
> SvgInb (*args, **kwargs)
*Wraps an SVG shape as required for an HTMX inband swap*
When returning an SVG shape in-band in HTMX, either have the calling
element include `hx_select='svg>*'`, or `**svg_inb` (which are two ways
of saying the same thing), or wrap the response with
[`SvgInb`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#svginb)
to have it appear correctly.
([`SvgInb`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#svginb)
is just a shortcut for the tuple
`(Svg(...), HtmxResponseHeaders(hx_reselect='svg>*'))`, which is the
trick that makes SVG in-band swaps work.)
| md |
FastHTML Docs | api/xtend.html.md | # Component extensions
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
``` python
from pprint import pprint
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L25"
target="_blank" style="float:right; font-size:smaller">source</a>
### A
> A (*c, hx_get=None, target_id=None, hx_swap=None, href='#', hx_vals=None,
> id=None, cls=None, title=None, style=None, accesskey=None,
> contenteditable=None, dir=None, draggable=None, enterkeyhint=None,
> hidden=None, inert=None, inputmode=None, lang=None, popover=None,
> spellcheck=None, tabindex=None, translate=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None, hx_history=None,
> hx_history_elt=None, hx_inherit=None, hx_params=None,
> hx_preserve=None, hx_prompt=None, hx_request=None, hx_sync=None,
> hx_validate=None, **kwargs)
*An A tag; `href` defaults to ‘\#’ for more concise use with HTMX*
``` python
A('text', ht_get='/get', target_id='id')
```
``` html
<a href="#" ht-get="/get" hx-target="#id">text</a>
```
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L31"
target="_blank" style="float:right; font-size:smaller">source</a>
### AX
> AX (txt, hx_get=None, target_id=None, hx_swap=None, href='#',
> hx_vals=None, id=None, cls=None, title=None, style=None,
> accesskey=None, contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_post=None, hx_put=None, hx_delete=None,
> hx_patch=None, hx_trigger=None, hx_target=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*An A tag with just one text child, allowing hx_get, target_id, and
hx_swap to be positional params*
``` python
AX('text', '/get', 'id')
```
``` html
<a href="#" hx-get="/get" hx-target="#id">text</a>
```
## Forms
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L37"
target="_blank" style="float:right; font-size:smaller">source</a>
### Form
> Form (*c, enctype='multipart/form-data', target_id=None, hx_vals=None,
> id=None, cls=None, title=None, style=None, accesskey=None,
> contenteditable=None, dir=None, draggable=None, enterkeyhint=None,
> hidden=None, inert=None, inputmode=None, lang=None, popover=None,
> spellcheck=None, tabindex=None, translate=None, hx_get=None,
> hx_post=None, hx_put=None, hx_delete=None, hx_patch=None,
> hx_trigger=None, hx_target=None, hx_swap=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None, hx_history_elt=None,
> hx_inherit=None, hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*A Form tag; identical to plain
[`ft_hx`](https://AnswerDotAI.github.io/fasthtml/api/components.html#ft_hx)
version except default `enctype='multipart/form-data'`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L43"
target="_blank" style="float:right; font-size:smaller">source</a>
### Hidden
> Hidden (value:Any='', id:Any=None, target_id=None, hx_vals=None,
> cls=None, title=None, style=None, accesskey=None,
> contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*An Input of type ‘hidden’*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L49"
target="_blank" style="float:right; font-size:smaller">source</a>
### CheckboxX
> CheckboxX (checked:bool=False, label=None, value='1', id=None, name=None,
> target_id=None, hx_vals=None, cls=None, title=None,
> style=None, accesskey=None, contenteditable=None, dir=None,
> draggable=None, enterkeyhint=None, hidden=None, inert=None,
> inputmode=None, lang=None, popover=None, spellcheck=None,
> tabindex=None, translate=None, hx_get=None, hx_post=None,
> hx_put=None, hx_delete=None, hx_patch=None, hx_trigger=None,
> hx_target=None, hx_swap=None, hx_swap_oob=None,
> hx_include=None, hx_select=None, hx_select_oob=None,
> hx_indicator=None, hx_push_url=None, hx_confirm=None,
> hx_disable=None, hx_replace_url=None, hx_disabled_elt=None,
> hx_ext=None, hx_headers=None, hx_history=None,
> hx_history_elt=None, hx_inherit=None, hx_params=None,
> hx_preserve=None, hx_prompt=None, hx_request=None,
> hx_sync=None, hx_validate=None, **kwargs)
*A Checkbox optionally inside a Label, preceded by a
[`Hidden`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#hidden)
with matching name*
``` python
show(CheckboxX(True, 'Check me out!'))
```
<input type="hidden" skip>
<label> <input type="checkbox" checked value="1">
Check me out!</label>
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L59"
target="_blank" style="float:right; font-size:smaller">source</a>
### Script
> Script (code:str='', id=None, cls=None, title=None, style=None,
> attrmap=None, valmap=None, ft_cls=<class 'fastcore.xml.FT'>,
> **kwargs)
*A Script tag that doesn’t escape its code*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L65"
target="_blank" style="float:right; font-size:smaller">source</a>
### Style
> Style (*c, id=None, cls=None, title=None, style=None, attrmap=None,
> valmap=None, ft_cls=<class 'fastcore.xml.FT'>, **kwargs)
*A Style tag that doesn’t escape its code*
## Style and script templates
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L70"
target="_blank" style="float:right; font-size:smaller">source</a>
### double_braces
> double_braces (s)
*Convert single braces to double braces if next to special chars or
newline*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L76"
target="_blank" style="float:right; font-size:smaller">source</a>
### undouble_braces
> undouble_braces (s)
*Convert double braces to single braces if next to special chars or
newline*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L82"
target="_blank" style="float:right; font-size:smaller">source</a>
### loose_format
> loose_format (s, **kw)
*String format `s` using `kw`, without being strict about braces outside
of template params*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L88"
target="_blank" style="float:right; font-size:smaller">source</a>
### ScriptX
> ScriptX (fname, src=None, nomodule=None, type=None, _async=None,
> defer=None, charset=None, crossorigin=None, integrity=None,
> **kw)
*A `script` element with contents read from `fname`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L96"
target="_blank" style="float:right; font-size:smaller">source</a>
### replace_css_vars
> replace_css_vars (css, pre='tpl', **kwargs)
*Replace `var(--)` CSS variables with `kwargs` if name prefix matches
`pre`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L105"
target="_blank" style="float:right; font-size:smaller">source</a>
### StyleX
> StyleX (fname, **kw)
*A `style` element with contents read from `fname` and variables
replaced from `kw`*
``` python
def Nbsp():
"A non-breaking space"
return Safe('nbsp;')
```
## Surreal and JS
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L113"
target="_blank" style="float:right; font-size:smaller">source</a>
### Surreal
> Surreal (code:str)
*Wrap `code` in `domReadyExecute` and set `m=me()` and `p=me('-')`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L126"
target="_blank" style="float:right; font-size:smaller">source</a>
### On
> On (code:str, event:str='click', sel:str='', me=True)
*An async surreal.js script block event handler for `event` on selector
`sel,p`, making available parent `p`, event `ev`, and target `e`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L139"
target="_blank" style="float:right; font-size:smaller">source</a>
### Prev
> Prev (code:str, event:str='click')
*An async surreal.js script block event handler for `event` on previous
sibling, with same vars as
[`On`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#on)*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L144"
target="_blank" style="float:right; font-size:smaller">source</a>
### Now
> Now (code:str, sel:str='')
*An async surreal.js script block on selector `me(sel)`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L150"
target="_blank" style="float:right; font-size:smaller">source</a>
### AnyNow
> AnyNow (sel:str, code:str)
*An async surreal.js script block on selector `any(sel)`*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L155"
target="_blank" style="float:right; font-size:smaller">source</a>
### run_js
> run_js (js, id=None, **kw)
*Run `js` script, auto-generating `id` based on name of caller if
needed, and js-escaping any `kw` params*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L162"
target="_blank" style="float:right; font-size:smaller">source</a>
### HtmxOn
> HtmxOn (eventname:str, code:str)
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L168"
target="_blank" style="float:right; font-size:smaller">source</a>
### jsd
> jsd (org, repo, root, path, prov='gh', typ='script', ver=None, esm=False,
> **kwargs)
*jsdelivr
[`Script`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#script)
or CSS `Link` tag, or URL*
## Other helpers
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L177"
target="_blank" style="float:right; font-size:smaller">source</a>
### Titled
> Titled (title:str='FastHTML app', *args, cls='container', target_id=None,
> hx_vals=None, id=None, style=None, accesskey=None,
> contenteditable=None, dir=None, draggable=None,
> enterkeyhint=None, hidden=None, inert=None, inputmode=None,
> lang=None, popover=None, spellcheck=None, tabindex=None,
> translate=None, hx_get=None, hx_post=None, hx_put=None,
> hx_delete=None, hx_patch=None, hx_trigger=None, hx_target=None,
> hx_swap=None, hx_swap_oob=None, hx_include=None, hx_select=None,
> hx_select_oob=None, hx_indicator=None, hx_push_url=None,
> hx_confirm=None, hx_disable=None, hx_replace_url=None,
> hx_disabled_elt=None, hx_ext=None, hx_headers=None,
> hx_history=None, hx_history_elt=None, hx_inherit=None,
> hx_params=None, hx_preserve=None, hx_prompt=None,
> hx_request=None, hx_sync=None, hx_validate=None, **kwargs)
*An HTML partial containing a `Title`, and `H1`, and any provided
children*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L182"
target="_blank" style="float:right; font-size:smaller">source</a>
### Socials
> Socials (title, site_name, description, image, url=None, w=1200, h=630,
> twitter_site=None, creator=None, card='summary')
*OG and Twitter social card headers*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L205"
target="_blank" style="float:right; font-size:smaller">source</a>
### Favicon
> Favicon (light_icon, dark_icon)
*Light and dark favicon headers*
------------------------------------------------------------------------
<a
href="https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/xtend.py#L211"
target="_blank" style="float:right; font-size:smaller">source</a>
### clear
> clear (id)
| md |
FastHTML Docs | explains/explaining_xt_components.html.md | # **FT** Components
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
**FT**, or ‘FastTags’, are the display components of FastHTML. In fact,
the word “components” in the context of FastHTML is often synonymous
with **FT**.
For example, when we look at a FastHTML app, in particular the views, as
well as various functions and other objects, we see something like the
code snippet below. It’s the `return` statement that we want to pay
attention to:
``` python
from fasthtml.common import *
def example():
# The code below is a set of ft components
return Div(
H1("FastHTML APP"),
P("Let's do this"),
cls="go"
)
```
Let’s go ahead and call our function and print the result:
``` python
example()
```
``` xml
<div class="go">
<h1>FastHTML APP</h1>
<p>Let's do this</p>
</div>
```
As you can see, when returned to the user from a Python callable, like a
function, the ft components are transformed into their string
representations of XML or XML-like content such as HTML. More concisely,
*ft turns Python objects into HTML*.
Now that we know what ft components look and behave like we can begin to
understand them. At their most fundamental level, ft components:
1. Are Python callables, specifically functions, classes, methods of
classes, lambda functions, and anything else called with parenthesis
that returns a value.
2. Return a sequence of values which has three elements:
1. The tag to be generated
2. The content of the tag, which is a tuple of strings/tuples. If a
tuple, it is the three-element structure of an ft component
3. A dictionary of XML attributes and their values
3. FastHTML’s default ft components words begin with an uppercase
letter. Examples include `Title()`, `Ul()`, and `Div()` Custom
components have included things like `BlogPost` and `CityMap`.
## How FastHTML names ft components
When it comes to naming ft components, FastHTML appears to break from
PEP8. Specifically, PEP8 specifies that when naming variables, functions
and instantiated classes we use the `snake_case_pattern`. That is to
say, lowercase with words separated by underscores. However, FastHTML
uses `PascalCase` for ft components.
There’s a couple of reasons for this:
1. ft components can be made from any callable type, so adhering to any
one pattern doesn’t make much sense
2. It makes for easier reading of FastHTML code, as anything that is
PascalCase is probably an ft component
## Default **FT** components
FastHTML has over 150 **FT** components designed to accelerate web
development. Most of these mirror HTML tags such as `<div>`, `<p>`,
`<a>`, `<title>`, and more. However, there are some extra tags added,
including:
- [`Titled`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#titled),
a combination of the `Title()` and `H1()` tags
- [`Socials`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#socials),
renders popular social media tags
## The `fasthtml.ft` Namespace
Some people prefer to write code using namespaces while adhering to
PEP8. If that’s a preference, projects can be coded using the
`fasthtml.ft` namespace.
``` python
from fasthtml import ft
ft.Ul(
ft.Li("one"),
ft.Li("two"),
ft.Li("three")
)
```
``` xml
<ul>
<li>one</li>
<li>two</li>
<li>three</li>
</ul>
```
## Attributes
This example demonstrates many important things to know about how ft
components handle attributes.
``` python
#| echo: False
Label(
"Choose an option",
Select(
Option("one", value="1", selected=True),
Option("two", value="2", selected=False),
Option("three", value=3),
cls="selector",
_id="counter",
**{'@click':"alert('Clicked');"},
),
_for="counter",
)
```
Line 2
Line 2 demonstrates that FastHTML appreciates `Label`s surrounding their
fields.
Line 5
On line 5, we can see that attributes set to the `boolean` value of
`True` are rendered with just the name of the attribute.
Line 6
On line 6, we demonstrate that attributes set to the `boolean` value of
`False` do not appear in the rendered output.
Line 7
Line 7 is an example of how integers and other non-string values in the
rendered output are converted to strings.
Line 8
Line 8 is where we set the HTML class using the `cls` argument. We use
`cls` here as `class` is a reserved word in Python. During the rendering
process this will be converted to the word “class”.
Line 9
Line 9 demonstrates that any named argument passed into an ft component
will have the leading underscore stripped away before rendering. Useful
for handling reserved words in Python.
Line 10
On line 10 we have an attribute name that cannot be represented as a
python variable. In cases like these, we can use an unpacked `dict` to
represent these values.
Line 12
The use of `_for` on line 12 is another demonstration of an argument
having the leading underscore stripped during render. We can also use
`fr` as that will be expanded to `for`.
This renders the following HTML snippet:
``` python
Label(
"Choose an option",
Select(
Option("one", value="1", selected=True),
Option("two", value="2", selected=False),
Option("three", value=3), # <4>,
cls="selector",
_id="counter",
**{'@click':"alert('Clicked');"},
),
_for="counter",
)
```
``` xml
<label for="counter">
Choose an option
<select id="counter" @click="alert('Clicked');" class="selector" name="counter">
<option value="1" selected>one</option>
<option value="2" >two</option>
<option value="3">three</option>
</select>
</label>
```
## Defining new ft components
It is possible and sometimes useful to create your own ft components
that generate non-standard tags that are not in the FastHTML library.
FastHTML supports created and defining those new tags flexibly.
For more information, see the [Defining new ft
components](../ref/defining_xt_component) reference page.
## FT components and type hints
If you use type hints, we strongly suggest that FT components be treated
as the `Any` type.
The reason is that FastHTML leverages python’s dynamic features to a
great degree. Especially when it comes to `FT` components, which can
evaluate out to be `FT|str|None|tuple` as well as anything that supports
the `__ft__`, `__html__`, and `__str__` method. That’s enough of the
Python stack that assigning anything but `Any` to be the FT type will
prove an exercise in frustation.
| md |
FastHTML Docs | explains/faq.html.md | # FAQ
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
## Why does my editor say that I have errors in my FastHTML code?
Many editors, including Visual Studio Code, use PyLance to provide error
checking for Python. However, PyLance’s error checking is just a guess –
it can’t actually know whether your code is correct or not. PyLance
particularly struggles with FastHTML’s syntax, which leads to it often
reporting false error messages in FastHTML projects.
To avoid these misleading error messages, it’s best to disable some
PyLance error checking in your FastHTML projects. Here’s how to do it in
Visual Studio Code (the same approach should also work in other editors
based on vscode, such as Cursor and GitHub Codespaces):
1. Open your FastHTML project
2. Press `Ctrl+Shift+P` (or `Cmd+Shift+P` on Mac) to open the Command
Palette
3. Type “Preferences: Open Workspace Settings (JSON)” and select it
4. In the JSON file that opens, add the following lines:
``` json
{
"python.analysis.diagnosticSeverityOverrides": {
"reportGeneralTypeIssues": "none",
"reportOptionalMemberAccess": "none",
"reportWildcardImportFromLibrary": "none",
"reportRedeclaration": "none",
"reportAttributeAccessIssue": "none",
"reportInvalidTypeForm": "none",
"reportAssignmentType": "none",
}
}
```
5. Save the file
Even with PyLance diagnostics turned off, your FastHTML code will still
run correctly. If you’re still seeing some false errors from PyLance,
you can disable it entirely by adding this to your settings:
``` json
{
"python.analysis.ignore": [ "*" ]
}
```
## Why the distinctive coding style?
FastHTML coding style is the [fastai coding
style](https://docs.fast.ai/dev/style.html).
If you are coming from a data science background the **fastai coding
style** may already be your preferred style.
If you are coming from a PEP-8 background where the use of ruff is
encouraged, there is a learning curve. However, once you get used to the
**fastai coding style** you may discover yourself appreciating the
concise nature of this style. It also encourages using more functional
programming tooling, which is both productive and fun. Having said that,
it’s entirely optional!
## Why not JSX?
Many have asked! We think there’s no benefit… Python’s positional and kw
args precisely 1:1 map already to html/xml children and attrs, so
there’s no need for a new syntax.
We wrote some more thoughts on Why Python HTML components over Jinja2,
Mako, or JSX
[here](https://www.answer.ai/posts/2024-08-03-fasthtml.html#why).
## Why use `import *`
First, through the use of the
[`__all__`](https://docs.python.org/3/tutorial/modules.html#importing-from-a-package)
attribute in our Python modules we control what actually gets imported.
So there’s no risk of namespace pollution.
Second, our style lends itself to working in rather compact Jupyter
notebooks and small Python modules. Hence we know about the source code
whose libraries we `import *` from. This terseness means we can develop
faster. We’re a small team, and any edge we can gain is important to us.
Third, for external libraries, be it core Python, SQLAlchemy, or other
things we do tend to use explicit imports. In part to avoid namespace
collisions, and also as reference to know where things are coming from.
We’ll finish by saying a lot of our users employ explicit imports. If
that’s the path you want to take, we encourage the use of
`from fasthtml import common as fh`. The acronym of `fh` makes it easy
to recognize that a symbol is from the FastHTML library.
## Can FastHTML be used for dashboards?
Yes it can. In fact, it excels at building dashboards. In addition to
being great for building static dashboards, because of its
[foundation](https://about.fastht.ml/foundation) in ASGI and [tech
stack](https://about.fastht.ml/tech), FastHTML natively supports
Websockets. That means using FastHTML we can create dashboards that
autoupdate.
## Why is FastHTML developed using notebooks?
Some people are under the impression that writing software in notebooks
is bad.
[Watch this
video](https://www.youtube.com/watch?v=9Q6sLbz37gk&ab_channel=JeremyHoward).
We’ve used Jupyter notebooks exported via `nbdev` to write a wide range
of “very serious” software projects over the last three years. This
includes deep learning libraries, API clients, Python language
extensions, terminal user interfaces, web frameworks, and more!
[nbdev](https://nbdev.fast.ai/) is a Jupyter-powered tool for writing
software. Traditional programming environments throw away the result of
your exploration in REPLs or notebooks. `nbdev` makes exploration an
integral part of your workflow, all while promoting software engineering
best practices.
## Why not pyproject.toml for packaging?
FastHTML uses a `setup.py` module instead of a `pyproject.toml` file to
configure itself for installation. The reason for this is
`pyproject.toml` is not compatible with [nbdev](https://nbdev.fast.ai/),
which is what is used to write and build FastHTML.
The nbdev project spent around a year trying to move to pyproject.toml
but there was insufficient functionality in the toml-based approach to
complete the transition.
| md |
FastHTML Docs | explains/oauth.html.md | # OAuth
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
OAuth is an open standard for ‘access delegation’, commonly used as a
way for Internet users to grant websites or applications access to their
information on other websites but without giving them the passwords. It
is the mechanism that enables “Log in with Google” on many sites, saving
you from having to remember and manage yet another password. Like many
auth-related topics, there’s a lot of depth and complexity to the OAuth
standard, but once you understand the basic usage it can be a very
convenient alternative to managing your own user accounts.
On this page you’ll see how to use OAuth with FastHTML to implement some
common pieces of functionality.
In FastHTML you set up a client like
[`GoogleAppClient`](https://AnswerDotAI.github.io/fasthtml/api/oauth.html#googleappclient).
The client is responsible for storing the client ID and client secret,
and for handling the OAuth flow. Let’s run through three examples,
illustrating some important concepts across three different OAuth
providers.
## A Minimal Login Flow (GitHub)
Let’s begin by building a minimal ‘Sign in with GitHub’ flow. This will
demonstrate the basic steps of OAuth.
OAuth requires a “provider” (in this case, GitHub) to authenticate the
user. So the first step when setting up our app is to register with
GitHub to set things up.
Go to https://github.com/settings/developers and click “New OAuth App”.
Fill in the form with the following values, then click ‘Register
application’.
- Application name: Your app name
- Homepage URL: http://localhost:8000 (or whatever URL you’re using -
you can change this later)
- Authorization callback URL: http://localhost:8000/auth_redirect (you
can modify this later too)
<figure>
<img src="attachment:Screenshot%202024-08-15%20101702.png"
alt="Setting up an OAuth app in GitHub" />
<figcaption aria-hidden="true">Setting up an OAuth app in
GitHub</figcaption>
</figure>
You’ll then see a screen where you can view the client ID and generate a
client secret. Copy these values and store them in a safe place.
These values are used to create a
[`GitHubAppClient`](https://AnswerDotAI.github.io/fasthtml/api/oauth.html#githubappclient)
object in FastHTML. This object is responsible for handling the OAuth
flow. Here’s how you’d set this up:
``` python
client = GitHubAppClient(
client_id="your_client_id",
client_secret="your_client_secret",
redirect_uri="http://localhost:8000/auth_redirect",
)
```
(It is recommended to store the client ID and secret in environment
variables, rather than hardcoding them in your code.)
To start the OAuth flow, you need to redirect the user to the provider’s
authorization URL. This URL is obtained by calling
`client.login_link()`.
Once you send a user to that link, they’ll be asked to grant your app
permission to access their GitHub account. If they agree, GitHub will
redirect them back to your site with a code that you can use to get an
access token. To receive this code, you need to set up a route in
FastHTML that listens for requests to your redirect uri
(`/auth_redirect` in this case). For example:
``` python
@app.get('/auth_redirect')
def auth_redirect(code:str):
return P(f"code: {code}")
```
This code is temporary, and is used to send a request to the provider
from the server (up until now only the client has communicated with the
provider). You can think of the exchange so far as:
- Client to us: “I want to log in”
- Us to client: “Here’s a link to log in”
- Client to provider: “I want to log in via this link”
- Provider to client: “OK, redirecting you to this URL (with a code)”
- Client to us: /auth_redirect?code=… (“Here’s the code you need to get
the token”)
Next we need:
- Us to provider: “A user I told to log in just gave me this code, can I
have a token please?”
- Provider to us: “Here’s the token”
- Us to provider: “Can I have the user’s details please? Here’s the
token”
- Provider to us: “Here’s the user’s details”
To go from code to user details, you can use
`info = client.retr_info(code)`. Or, if all you need is a unique
identifier for the user, you can just use `retr_id` instead:
``` python
@app.get('/auth_redirect')
def auth_redirect(code:str):
user_id = client.retr_id(code)
return P(f"User id: {user_id}")
```
There’s not much use in just printing the user info - going forward we
want to be able to persistently keep track of who this user is. One
conveneint way to do this is to store the user ID in the `session`
object. Since this is cryptographically signed, it’s safe to store
sensitive information here - the user can’t read it, but we can fetch it
back out for any future requests they make. On the server side, you
could also store this information in a database if you need to keep
track of user info.
Here’s a minimal app that puts all these pieces together:
``` python
from fasthtml.common import *
from fasthtml.oauth import GitHubAppClient
db = database('data/counts.db')
counts = db.t.counts
if counts not in db.t: counts.create(dict(name=str, count=int), pk='name')
Count = counts.dataclass()
# Auth client setup for GitHub
client = GitHubAppClient(os.getenv("AUTH_CLIENT_ID"),
os.getenv("AUTH_CLIENT_SECRET"),
redirect_uri="http://localhost:5001/auth_redirect")
login_link = client.login_link()
def before(req, session):
auth = req.scope['auth'] = session.get('user_id', None)
if not auth: return RedirectResponse('/login', status_code=303)
counts.xtra(name=auth)
bware = Beforeware(before, skip=['/login', '/auth_redirect'])
app = FastHTML(before=bware)
@app.get('/')
def home(auth):
return Div(
P("Count demo"),
P(f"Count: ", Span(counts[auth].count, id='count')),
Button('Increment', hx_get='/increment', hx_target='#count'),
P(A('Logout', href='/logout'))
)
@app.get('/increment')
def increment(auth):
c = counts[auth]
c.count += 1
return counts.upsert(c).count
@app.get('/login')
def login(): return P(A('Login with GitHub', href=client.login_link()))
@app.get('/logout')
def logout(session):
session.pop('user_id', None)
return RedirectResponse('/login', status_code=303)
@app.get('/auth_redirect')
def auth_redirect(code:str, session):
if not code: return "No code provided!"
user_id = client.retr_id(code)
session['user_id'] = user_id
if user_id not in counts: counts.insert(name=user_id, count=0)
return RedirectResponse('/', status_code=303)
serve()
```
Some things to note:
- The `before` function is used to check if the user is authenticated.
If not, they are redirected to the login page.
- To log the user out, we remove the user ID from the session.
- Calling `counts.xtra(name=auth)` ensures that only the row
corresponding to the current user is accessible when responding to a
request. This is often nicer than trying to remember to filter the
data in every route, and lowers the risk of accidentally leaking data.
- In the `auth_redirect` route, we store the user ID in the session and
create a new row in the `user_counts` table if it doesn’t already
exist.
You can find more heavily-commented version of this code in the [oauth
directory in
fasthtml-example](https://github.com/AnswerDotAI/fasthtml-example/tree/main/oauth_example),
along with an even more minimal example. More examples may be added in
the future.
### Revoking Tokens (Google)
When the user in the example above logs out, we remove their user ID
from the session. However, the user is still logged in to GitHub. If
they click ‘Login with GitHub’ again, they’ll be redirected back to our
site without having to log in again. This is because GitHub remembers
that they’ve already granted our app permission to access their account.
Most of the time this is convenient, but for testing or security
purposes you may want a way to revoke this permission.
As a user, you can usually revoke access to an app from the provider’s
website (for example, <https://github.com/settings/applications>). But
as a developer, you can also revoke access programmatically - at least
with some providers. This requires keeping track of the access token
(stored in `client.token["access_token"]` after you call `retr_info`),
and sending a request to the provider’s revoke URL:
``` python
auth_revoke_url = "https://accounts.google.com/o/oauth2/revoke"
def revoke_token(token):
response = requests.post(auth_revoke_url, params={"token": token})
return response.status_code == 200 # True if successful
```
Not all proivders support token revocation, and it is not built into
FastHTML clients at the moment.
### Using State (Hugging Face)
Imagine a user (not logged in) comes to your AI image editing site,
starts testing things out, and then realizes they need to sign in before
they can click “Run (Pro)” on the edit they’re working on. They click
“Sign in with Hugging Face”, log in, and are redirected back to your
site. But now they’ve lost their in-progress edit and are left just
looking at the homepage! This is an example of a case where you might
want to keep track of some additional state. Another strong use case for
being able to pass some uniqie state through the OAuth flow is to
prevent something called a [CSRF
attack](https://en.wikipedia.org/wiki/Cross-site_request_forgery). To
add a state string to the OAuth flow, you can use
`client.login_link_with_state(state)` instead of `client.login_link()`,
like so:
``` python
# in login page:
link = A('Login with GitHub', href=client.login_link_with_state(state='current_prompt: add a unicorn'))
# in auth_redirect:
@app.get('/auth_redirect')
def auth_redirect(code:str, session, state:str=None):
print(f"state: {state}") # Use as needed
...
```
The state string is passed through the OAuth flow and back to your site.
### A Work in Progress
This page (and OAuth support in FastHTML) is a work in progress.
Questions, PRs, and feedback are welcome!
| md |
FastHTML Docs | explains/routes.html.md | # Routes
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
Behaviour in FastHTML apps is defined by routes. The syntax is largely
the same as the wonderful [FastAPI](https://fastapi.tiangolo.com/)
(which is what you should be using instead of this if you’re creating a
JSON service. FastHTML is mainly for making HTML web apps, not APIs).
<div>
> **Unfinished**
>
> We haven’t yet written complete documentation of all of FastHTML’s
> routing features – until we add that, the best place to see all the
> available functionality is to look over [the
> tests](../api/core.html#tests)
</div>
Note that you need to include the types of your parameters, so that
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml)
knows what to pass to your function. Here, we’re just expecting a
string:
``` python
from fasthtml.common import *
```
``` python
app = FastHTML()
@app.get('/user/{nm}')
def get_nm(nm:str): return f"Good day to you, {nm}!"
```
Normally you’d save this into a file such as main.py, and then run it in
`uvicorn` using:
uvicorn main:app
However, for testing, we can use Starlette’s `TestClient` to try it out:
``` python
from starlette.testclient import TestClient
```
``` python
client = TestClient(app)
r = client.get('/user/Jeremy')
r
```
<Response [200 OK]>
TestClient uses `httpx` behind the scenes, so it returns a
`httpx.Response`, which has a `text` attribute with our response body:
``` python
r.text
```
'Good day to you, Jeremy!'
In the previous example, the function name (`get_nm`) didn’t actually
matter – we could have just called it `_`, for instance, since we never
actually call it directly. It’s just called through HTTP. In fact, we
often do call our functions `_` when using this style of route, since
that’s one less thing we have to worry about, naming.
An alternative approach to creating a route is to use `app.route`
instead, in which case, you make the function name the HTTP method you
want. Since this is such a common pattern, you might like to give a
shorter name to `app.route` – we normally use `rt`:
``` python
rt = app.route
@rt('/')
def post(): return "Going postal!"
client.post('/').text
```
'Going postal!'
### Route-specific functionality
FastHTML supports custom decorators for adding specific functionality to
routes. This allows you to implement authentication, authorization,
middleware, or other custom behaviors for individual routes.
Here’s an example of a basic authentication decorator:
``` python
from functools import wraps
def basic_auth(f):
@wraps(f)
async def wrapper(req, *args, **kwargs):
token = req.headers.get("Authorization")
if token == 'abc123':
return await f(req, *args, **kwargs)
return Response('Not Authorized', status_code=401)
return wrapper
@app.get("/protected")
@basic_auth
async def protected(req):
return "Protected Content"
client.get('/protected', headers={'Authorization': 'abc123'}).text
```
'Protected Content'
The decorator intercepts the request before the route function executes.
If the decorator allows the request to proceed, it calls the original
route function, passing along the request and any other arguments.
One of the key advantages of this approach is the ability to apply
different behaviors to different routes. You can also stack multiple
decorators on a single route for combined functionality.
``` python
def app_beforeware():
print('App level beforeware')
app = FastHTML(before=Beforeware(app_beforeware))
client = TestClient(app)
def route_beforeware(f):
@wraps(f)
async def decorator(*args, **kwargs):
print('Route level beforeware')
return await f(*args, **kwargs)
return decorator
def second_route_beforeware(f):
@wraps(f)
async def decorator(*args, **kwargs):
print('Second route level beforeware')
return await f(*args, **kwargs)
return decorator
@app.get("/users")
@route_beforeware
@second_route_beforeware
async def users():
return "Users Page"
client.get('/users').text
```
App level beforeware
Route level beforeware
Second route level beforeware
'Users Page'
This flexiblity allows for granular control over route behaviour,
enabling you to tailor each endpoint’s functionality as needed. While
app-level beforeware remains useful for global operations, decorators
provide a powerful tool for route-specific customization.
## Combining Routes
Sometimes a FastHTML project can grow so weildy that putting all the
routes into `main.py` becomes unweildy. Or, we install a FastHTML- or
Starlette-based package that requires us to add routes.
First let’s create a `books.py` module, that represents all the
user-related views:
``` python
# books.py
books_app, rt = fast_app()
books = ['A Guide to FastHTML', 'FastHTML Cookbook', 'FastHTML in 24 Hours']
@rt("/", name="list")
def get():
return Titled("Books", *[P(book) for book in books])
```
Let’s mount it in our main module:
``` python
from books import app as books_app
app, rt = fast_app(routes=[Mount("/books", books_app, name="books")])
@rt("/")
def get():
return Titled("Dashboard",
P(A(href="/books")("Books")),
Hr(),
P(A(link=uri("books:list"))("Books")),
)
serve()
```
Line 3
We use `starlette.Mount` to add the route to our routes list. We provide
the name of `books` to make discovery and management of the links
easier. More on that in items 2 and 3 of this annotations list
Line 8
This example link to the books list view is hand-crafted. Obvious in
purpose, it makes changing link patterns in the future harder
Line 10
This example link uses the named URL route for the books. The advantage
of this approach is it makes management of large numbers of link items
easier.
| md |
FastHTML Docs | ref/handlers.html.md | # Handling handlers
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
``` python
from fasthtml.common import *
from collections import namedtuple
from typing import TypedDict
from datetime import datetime
import json,time
```
``` python
app = FastHTML()
```
The
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml)
class is the main application class for FastHTML apps.
``` python
rt = app.route
```
`app.route` is used to register route handlers. It is a decorator, which
means we place it before a function that is used as a handler. Because
it’s used frequently in most FastHTML applications, we often alias it as
`rt`, as we do here.
## Basic Route Handling
``` python
@rt("/hi")
def get(): return 'Hi there'
```
Handler functions can return strings directly. These strings are sent as
the response body to the client.
``` python
cli = Client(app)
```
[`Client`](https://AnswerDotAI.github.io/fasthtml/api/core.html#client)
is a test client for FastHTML applications. It allows you to simulate
requests to your app without running a server.
``` python
cli.get('/hi').text
```
'Hi there'
The `get` method on a
[`Client`](https://AnswerDotAI.github.io/fasthtml/api/core.html#client)
instance simulates GET requests to the app. It returns a response object
that has a `.text` attribute, which you can use to access the body of
the response. It calls `httpx.get` internally – all httpx HTTP verbs are
supported.
``` python
@rt("/hi")
def post(): return 'Postal'
cli.post('/hi').text
```
'Postal'
Handler functions can be defined for different HTTP methods on the same
route. Here, we define a `post` handler for the `/hi` route. The
[`Client`](https://AnswerDotAI.github.io/fasthtml/api/core.html#client)
instance can simulate different HTTP methods, including POST requests.
## Request and Response Objects
``` python
@app.get("/hostie")
def show_host(req): return req.headers['host']
cli.get('/hostie').text
```
'testserver'
Handler functions can accept a `req` (or `request`) parameter, which
represents the incoming request. This object contains information about
the request, including headers. In this example, we return the `host`
header from the request. The test client uses ‘testserver’ as the
default host.
In this example, we use `@app.get("/hostie")` instead of
`@rt("/hostie")`. The `@app.get()` decorator explicitly specifies the
HTTP method (GET) for the route, while `@rt()` by default handles both
GET and POST requests.
``` python
@rt
def yoyo(): return 'a yoyo'
cli.post('/yoyo').text
```
'a yoyo'
If the `@rt` decorator is used without arguments, it uses the function
name as the route path. Here, the `yoyo` function becomes the handler
for the `/yoyo` route. This handler responds to GET and POST methods,
since a specific method wasn’t provided.
``` python
@rt
def ft1(): return Html(Div('Text.'))
print(cli.get('/ft1').text)
```
<html>
<div>Text.</div>
</html>
Handler functions can return
[`FT`](https://docs.fastht.ml/explains/explaining_xt_components.html)
objects, which are automatically converted to HTML strings. The `FT`
class can take other `FT` components as arguments, such as `Div`. This
allows for easy composition of HTML elements in your responses.
``` python
@app.get
def autopost(): return Html(Div('Text.', hx_post=yoyo.rt()))
print(cli.get('/autopost').text)
```
<html>
<div hx-post="/yoyo">Text.</div>
</html>
The `rt` decorator modifies the `yoyo` function by adding an `rt()`
method. This method returns the route path associated with the handler.
It’s a convenient way to reference the route of a handler function
dynamically.
In the example, `yoyo.rt()` is used as the value for `hx_post`. This
means when the div is clicked, it will trigger an HTMX POST request to
the route of the `yoyo` handler. This approach allows for flexible, DRY
code by avoiding hardcoded route strings and automatically updating if
the route changes.
This pattern is particularly useful in larger applications where routes
might change, or when building reusable components that need to
reference their own routes dynamically.
``` python
@app.get
def autoget(): return Html(Body(Div('Text.', cls='px-2', hx_post=show_host.rt(a='b'))))
print(cli.get('/autoget').text)
```
<html>
<body>
<div hx-post="/hostie?a=b" class="px-2">Text.</div>
</body>
</html>
The `rt()` method of handler functions can also accept parameters. When
called with parameters, it returns the route path with a query string
appended. In this example, `show_host.rt(a='b')` generates the path
`/hostie?a=b`.
The `Body` component is used here to demonstrate nesting of FT
components. `Div` is nested inside `Body`, showcasing how you can create
more complex HTML structures.
The `cls` parameter is used to add a CSS class to the `Div`. This
translates to the `class` attribute in the rendered HTML. (`class` can’t
be used as a parameter name directly in Python since it’s a reserved
word.)
``` python
@rt('/ft2')
def get(): return Title('Foo'),H1('bar')
print(cli.get('/ft2').text)
```
<!doctype html>
<html>
<head>
<title>Foo</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, viewport-fit=cover">
<script src="https://unpkg.com/htmx.org@next/dist/htmx.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/fasthtml-js@main/fasthtml.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/surreal@main/surreal.js"></script>
<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>
</head>
<body>
<h1>bar</h1>
</body>
</html>
Handler functions can return multiple `FT` objects as a tuple. The first
item is treated as the `Title`, and the rest are added to the `Body`.
When the request is not an HTMX request, FastHTML automatically adds
necessary HTML boilerplate, including default `head` content with
required scripts.
When using `app.route` (or `rt`), if the function name matches an HTTP
verb (e.g., `get`, `post`, `put`, `delete`), that HTTP method is
automatically used for the route. In this case, a path must be
explicitly provided as an argument to the decorator.
``` python
hxhdr = {'headers':{'hx-request':"1"}}
print(cli.get('/ft2', **hxhdr).text)
```
<title>Foo</title>
<h1>bar</h1>
For HTMX requests (indicated by the `hx-request` header), FastHTML
returns only the specified components without the full HTML structure.
This allows for efficient partial page updates in HTMX applications.
``` python
@rt('/ft3')
def get(): return H1('bar')
print(cli.get('/ft3', **hxhdr).text)
```
<h1>bar</h1>
When a handler function returns a single `FT` object for an HTMX
request, it’s rendered as a single HTML partial.
``` python
@rt('/ft4')
def get(): return Html(Head(Title('hi')), Body(P('there')))
print(cli.get('/ft4').text)
```
<html>
<head>
<title>hi</title>
</head>
<body>
<p>there</p>
</body>
</html>
Handler functions can return a complete `Html` structure, including
`Head` and `Body` components. When a full HTML structure is returned,
FastHTML doesn’t add any additional boilerplate. This gives you full
control over the HTML output when needed.
``` python
@rt
def index(): return "welcome!"
print(cli.get('/').text)
```
welcome!
The `index` function is a special handler in FastHTML. When defined
without arguments to the `@rt` decorator, it automatically becomes the
handler for the root path (`'/'`). This is a convenient way to define
the main page or entry point of your application.
## Path and Query Parameters
``` python
@rt('/user/{nm}', name='gday')
def get(nm:str=''): return f"Good day to you, {nm}!"
cli.get('/user/Alexis').text
```
'Good day to you, Alexis!'
Handler functions can use path parameters, defined using curly braces in
the route – this is implemented by Starlette directly, so all Starlette
path parameters can be used. These parameters are passed as arguments to
the function.
The `name` parameter in the decorator allows you to give the route a
name, which can be used for URL generation.
In this example, `{nm}` in the route becomes the `nm` parameter in the
function. The function uses this parameter to create a personalized
greeting.
``` python
@app.get
def autolink(): return Html(Div('Text.', link=uri('gday', nm='Alexis')))
print(cli.get('/autolink').text)
```
<html>
<div href="/user/Alexis">Text.</div>
</html>
The [`uri`](https://AnswerDotAI.github.io/fasthtml/api/core.html#uri)
function is used to generate URLs for named routes. It takes the route
name as its first argument, followed by any path or query parameters
needed for that route.
In this example, `uri('gday', nm='Alexis')` generates the URL for the
route named ‘gday’ (which we defined earlier as ‘/user/{nm}’), with
‘Alexis’ as the value for the ‘nm’ parameter.
The `link` parameter in FT components sets the `href` attribute of the
rendered HTML element. By using `uri()`, we can dynamically generate
correct URLs even if the underlying route structure changes.
This approach promotes maintainable code by centralizing route
definitions and avoiding hardcoded URLs throughout the application.
``` python
@rt('/link')
def get(req): return f"{req.url_for('gday', nm='Alexis')}; {req.url_for('show_host')}"
cli.get('/link').text
```
'http://testserver/user/Alexis; http://testserver/hostie'
The `url_for` method of the request object can be used to generate URLs
for named routes. It takes the route name as its first argument,
followed by any path parameters needed for that route.
In this example, `req.url_for('gday', nm='Alexis')` generates the full
URL for the route named ‘gday’, including the scheme and host.
Similarly, `req.url_for('show_host')` generates the URL for the
‘show_host’ route.
This method is particularly useful when you need to generate absolute
URLs, such as for email links or API responses. It ensures that the
correct host and scheme are included, even if the application is
accessed through different domains or protocols.
``` python
app.url_path_for('gday', nm='Jeremy')
```
'/user/Jeremy'
The `url_path_for` method of the application can be used to generate URL
paths for named routes. Unlike `url_for`, it returns only the path
component of the URL, without the scheme or host.
In this example, `app.url_path_for('gday', nm='Jeremy')` generates the
path ‘/user/Jeremy’ for the route named ‘gday’.
This method is useful when you need relative URLs or just the path
component, such as for internal links or when constructing URLs in a
host-agnostic manner.
``` python
@rt('/oops')
def get(nope): return nope
r = cli.get('/oops?nope=1')
print(r)
r.text
```
<Response [200 OK]>
/Users/jhoward/Documents/GitHub/fasthtml/fasthtml/core.py:175: UserWarning: `nope has no type annotation and is not a recognised special name, so is ignored.
if arg!='resp': warn(f"`{arg} has no type annotation and is not a recognised special name, so is ignored.")
''
Handler functions can include parameters, but they must be
type-annotated or have special names (like `req`) to be recognized. In
this example, the `nope` parameter is not annotated, so it’s ignored,
resulting in a warning.
When a parameter is ignored, it doesn’t receive the value from the query
string. This can lead to unexpected behavior, as the function attempts
to return `nope`, which is undefined.
The `cli.get('/oops?nope=1')` call succeeds with a 200 OK status because
the handler doesn’t raise an exception, but it returns an empty
response, rather than the intended value.
To fix this, you should either add a type annotation to the parameter
(e.g., `def get(nope: str):`) or use a recognized special name like
`req`.
``` python
@rt('/html/{idx}')
def get(idx:int): return Body(H4(f'Next is {idx+1}.'))
print(cli.get('/html/1', **hxhdr).text)
```
<body>
<h4>Next is 2.</h4>
</body>
Path parameters can be type-annotated, and FastHTML will automatically
convert them to the specified type if possible. In this example, `idx`
is annotated as `int`, so it’s converted from the string in the URL to
an integer.
``` python
reg_re_param("imgext", "ico|gif|jpg|jpeg|webm")
@rt(r'/static/{path:path}{fn}.{ext:imgext}')
def get(fn:str, path:str, ext:str): return f"Getting {fn}.{ext} from /{path}"
print(cli.get('/static/foo/jph.ico').text)
```
Getting jph.ico from /foo/
The
[`reg_re_param`](https://AnswerDotAI.github.io/fasthtml/api/core.html#reg_re_param)
function is used to register custom path parameter types using regular
expressions. Here, we define a new path parameter type called “imgext”
that matches common image file extensions.
Handler functions can use complex path patterns with multiple parameters
and custom types. In this example, the route pattern
`r'/static/{path:path}{fn}.{ext:imgext}'` uses three path parameters:
1. `path`: A Starlette built-in type that matches any path segments
2. `fn`: The filename without extension
3. `ext`: Our custom “imgext” type that matches specific image
extensions
``` python
ModelName = str_enum('ModelName', "alexnet", "resnet", "lenet")
@rt("/models/{nm}")
def get(nm:ModelName): return nm
print(cli.get('/models/alexnet').text)
```
alexnet
We define `ModelName` as an enum with three possible values: “alexnet”,
“resnet”, and “lenet”. Handler functions can use these enum types as
parameter annotations. In this example, the `nm` parameter is annotated
with `ModelName`, which ensures that only valid model names are
accepted.
When a request is made with a valid model name, the handler function
returns that name. This pattern is useful for creating type-safe APIs
with a predefined set of valid values.
``` python
@rt("/files/{path}")
async def get(path: Path): return path.with_suffix('.txt')
print(cli.get('/files/foo').text)
```
foo.txt
Handler functions can use
[`Path`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#path)
objects as parameter types. The
[`Path`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#path) type
is from Python’s standard library `pathlib` module, which provides an
object-oriented interface for working with file paths. In this example,
the `path` parameter is annotated with
[`Path`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#path), so
FastHTML automatically converts the string from the URL to a
[`Path`](https://AnswerDotAI.github.io/fasthtml/api/svg.html#path)
object.
This approach is particularly useful when working with file-related
routes, as it provides a convenient and platform-independent way to
handle file paths.
``` python
fake_db = [{"name": "Foo"}, {"name": "Bar"}]
@rt("/items/")
def get(idx:int|None = 0): return fake_db[idx]
print(cli.get('/items/?idx=1').text)
```
{"name":"Bar"}
Handler functions can use query parameters, which are automatically
parsed from the URL. In this example, `idx` is a query parameter with a
default value of 0. It’s annotated as `int|None`, allowing it to be
either an integer or None.
The function uses this parameter to index into a fake database
(`fake_db`). When a request is made with a valid `idx` query parameter,
the handler returns the corresponding item from the database.
``` python
print(cli.get('/items/').text)
```
{"name":"Foo"}
When no `idx` query parameter is provided, the handler function uses the
default value of 0. This results in returning the first item from the
`fake_db` list, which is `{"name":"Foo"}`.
This behavior demonstrates how default values for query parameters work
in FastHTML. They allow the API to have a sensible default behavior when
optional parameters are not provided.
``` python
print(cli.get('/items/?idx=g'))
```
<Response [404 Not Found]>
When an invalid value is provided for a typed query parameter, FastHTML
returns a 404 Not Found response. In this example, ‘g’ is not a valid
integer for the `idx` parameter, so the request fails with a 404 status.
This behavior ensures type safety and prevents invalid inputs from
reaching the handler function.
``` python
@app.get("/booly/")
def _(coming:bool=True): return 'Coming' if coming else 'Not coming'
print(cli.get('/booly/?coming=true').text)
print(cli.get('/booly/?coming=no').text)
```
Coming
Not coming
Handler functions can use boolean query parameters. In this example,
`coming` is a boolean parameter with a default value of `True`. FastHTML
automatically converts string values like ‘true’, ‘false’, ‘1’, ‘0’,
‘on’, ‘off’, ‘yes’, and ‘no’ to their corresponding boolean values.
The underscore `_` is used as the function name in this example to
indicate that the function’s name is not important or won’t be
referenced elsewhere. This is a common Python convention for throwaway
or unused variables, and it works here because FastHTML uses the route
decorator parameter, when provided, to determine the URL path, not the
function name. By default, both `get` and `post` methods can be used in
routes that don’t specify an http method (by either using `app.get`,
`def get`, or the `methods` parameter to `app.route`).
``` python
@app.get("/datie/")
def _(d:parsed_date): return d
date_str = "17th of May, 2024, 2p"
print(cli.get(f'/datie/?d={date_str}').text)
```
2024-05-17 14:00:00
Handler functions can use `date` objects as parameter types. FastHTML
uses `dateutil.parser` library to automatically parse a wide variety of
date string formats into `date` objects.
``` python
@app.get("/ua")
async def _(user_agent:str): return user_agent
print(cli.get('/ua', headers={'User-Agent':'FastHTML'}).text)
```
FastHTML
Handler functions can access HTTP headers by using parameter names that
match the header names. In this example, `user_agent` is used as a
parameter name, which automatically captures the value of the
‘User-Agent’ header from the request.
The
[`Client`](https://AnswerDotAI.github.io/fasthtml/api/core.html#client)
instance allows setting custom headers for test requests. Here, we set
the ‘User-Agent’ header to ‘FastHTML’ in the test request.
``` python
@app.get("/hxtest")
def _(htmx): return htmx.request
print(cli.get('/hxtest', headers={'HX-Request':'1'}).text)
@app.get("/hxtest2")
def _(foo:HtmxHeaders, req): return foo.request
print(cli.get('/hxtest2', headers={'HX-Request':'1'}).text)
```
1
1
Handler functions can access HTMX-specific headers using either the
special `htmx` parameter name, or a parameter annotated with
[`HtmxHeaders`](https://AnswerDotAI.github.io/fasthtml/api/core.html#htmxheaders).
Both approaches provide access to HTMX-related information.
In these examples, the `htmx.request` attribute returns the value of the
‘HX-Request’ header.
``` python
app.chk = 'foo'
@app.get("/app")
def _(app): return app.chk
print(cli.get('/app').text)
```
foo
Handler functions can access the
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml)
application instance using the special `app` parameter name. This allows
handlers to access application-level attributes and methods.
In this example, we set a custom attribute `chk` on the application
instance. The handler function then uses the `app` parameter to access
this attribute and return its value.
``` python
@app.get("/app2")
def _(foo:FastHTML): return foo.chk,HttpHeader("mykey", "myval")
r = cli.get('/app2', **hxhdr)
print(r.text)
print(r.headers)
```
foo
Headers({'mykey': 'myval', 'content-length': '4', 'content-type': 'text/html; charset=utf-8'})
Handler functions can access the
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml)
application instance using a parameter annotated with
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml).
This allows handlers to access application-level attributes and methods,
just like using the special `app` parameter name.
Handlers can return tuples containing both content and
[`HttpHeader`](https://AnswerDotAI.github.io/fasthtml/api/core.html#httpheader)
objects.
[`HttpHeader`](https://AnswerDotAI.github.io/fasthtml/api/core.html#httpheader)
allows setting custom HTTP headers in the response.
In this example:
- We define a handler that returns both the `chk` attribute from the
application and a custom header.
- The `HttpHeader("mykey", "myval")` sets a custom header in the
response.
- We use the test client to make a request and examine both the response
text and headers.
- The response includes the custom header “mykey” along with standard
headers like content-length and content-type.
``` python
@app.get("/app3")
def _(foo:FastHTML): return HtmxResponseHeaders(location="http://example.org")
r = cli.get('/app3')
print(r.headers)
```
Headers({'hx-location': 'http://example.org', 'content-length': '0', 'content-type': 'text/html; charset=utf-8'})
Handler functions can return
[`HtmxResponseHeaders`](https://AnswerDotAI.github.io/fasthtml/api/core.html#htmxresponseheaders)
objects to set HTMX-specific response headers. This is useful for
HTMX-specific behaviors like client-side redirects.
In this example we define a handler that returns an
[`HtmxResponseHeaders`](https://AnswerDotAI.github.io/fasthtml/api/core.html#htmxresponseheaders)
object with a `location` parameter, which sets the `HX-Location` header
in the response. HTMX uses this for client-side redirects.
``` python
@app.get("/app4")
def _(foo:FastHTML): return Redirect("http://example.org")
cli.get('/app4', follow_redirects=False)
```
<Response [303 See Other]>
Handler functions can return
[`Redirect`](https://AnswerDotAI.github.io/fasthtml/api/core.html#redirect)
objects to perform HTTP redirects. This is useful for redirecting users
to different pages or external URLs.
In this example:
- We define a handler that returns a
[`Redirect`](https://AnswerDotAI.github.io/fasthtml/api/core.html#redirect)
object with the URL “http://example.org”.
- The `cli.get('/app4', follow_redirects=False)` call simulates a GET
request to the ‘/app4’ route without following redirects.
- The response has a 303 See Other status code, indicating a redirect.
The `follow_redirects=False` parameter is used to prevent the test
client from automatically following the redirect, allowing us to inspect
the redirect response itself.
``` python
Redirect.__response__
```
<function fasthtml.core.Redirect.__response__(self, req)>
The
[`Redirect`](https://AnswerDotAI.github.io/fasthtml/api/core.html#redirect)
class in FastHTML implements a `__response__` method, which is a special
method recognized by the framework. When a handler returns a
[`Redirect`](https://AnswerDotAI.github.io/fasthtml/api/core.html#redirect)
object, FastHTML internally calls this `__response__` method to replace
the original response.
The `__response__` method takes a `req` parameter, which represents the
incoming request. This allows the method to access request information
if needed when constructing the redirect response.
``` python
@rt
def meta():
return ((Title('hi'),H1('hi')),
(Meta(property='image'), Meta(property='site_name')))
print(cli.post('/meta').text)
```
<!doctype html>
<html>
<head>
<title>hi</title>
<meta property="image">
<meta property="site_name">
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, viewport-fit=cover">
<script src="https://unpkg.com/htmx.org@next/dist/htmx.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/fasthtml-js@main/fasthtml.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/surreal@main/surreal.js"></script>
<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>
</head>
<body>
<h1>hi</h1>
</body>
</html>
FastHTML automatically identifies elements typically placed in the
`<head>` (like `Title` and `Meta`) and positions them accordingly, while
other elements go in the `<body>`.
In this example: - `(Title('hi'), H1('hi'))` defines the title and main
heading. The title is placed in the head, and the H1 in the body. -
`(Meta(property='image'), Meta(property='site_name'))` defines two meta
tags, which are both placed in the head.
## Form Data and JSON Handling
``` python
@app.post('/profile/me')
def profile_update(username: str): return username
print(cli.post('/profile/me', data={'username' : 'Alexis'}).text)
r = cli.post('/profile/me', data={})
print(r.text)
r
```
Alexis
Missing required field: username
<Response [400 Bad Request]>
Handler functions can accept form data parameters, without needing to
manually extract it from the request. In this example, `username` is
expected to be sent as form data.
If required form data is missing, FastHTML automatically returns a 400
Bad Request response with an error message.
The `data` parameter in the `cli.post()` method simulates sending form
data in the request.
``` python
@app.post('/pet/dog')
def pet_dog(dogname: str = None): return dogname or 'unknown name'
print(cli.post('/pet/dog', data={}).text)
```
unknown name
Handlers can have optional form data parameters with default values. In
this example, `dogname` is an optional parameter with a default value of
`None`.
Here, if the form data doesn’t include the `dogname` field, the function
uses the default value. The function returns either the provided
`dogname` or ‘unknown name’ if `dogname` is `None`.
``` python
@dataclass
class Bodie: a:int;b:str
@rt("/bodie/{nm}")
def post(nm:str, data:Bodie):
res = asdict(data)
res['nm'] = nm
return res
print(cli.post('/bodie/me', data=dict(a=1, b='foo', nm='me')).text)
```
{"a":1,"b":"foo","nm":"me"}
You can use dataclasses to define structured form data. In this example,
`Bodie` is a dataclass with `a` (int) and `b` (str) fields.
FastHTML automatically converts the incoming form data to a `Bodie`
instance where attribute names match parameter names. Other form data
elements are matched with parameters with the same names (in this case,
`nm`).
Handler functions can return dictionaries, which FastHTML automatically
JSON-encodes.
``` python
@app.post("/bodied/")
def bodied(data:dict): return data
d = dict(a=1, b='foo')
print(cli.post('/bodied/', data=d).text)
```
{"a":"1","b":"foo"}
`dict` parameters capture all form data as a dictionary. In this
example, the `data` parameter is annotated with `dict`, so FastHTML
automatically converts all incoming form data into a dictionary.
Note that when form data is converted to a dictionary, all values become
strings, even if they were originally numbers. This is why the ‘a’ key
in the response has a string value “1” instead of the integer 1.
``` python
nt = namedtuple('Bodient', ['a','b'])
@app.post("/bodient/")
def bodient(data:nt): return asdict(data)
print(cli.post('/bodient/', data=d).text)
```
{"a":"1","b":"foo"}
Handler functions can use named tuples to define structured form data.
In this example, `Bodient` is a named tuple with `a` and `b` fields.
FastHTML automatically converts the incoming form data to a `Bodient`
instance where field names match parameter names. As with the previous
example, all form data values are converted to strings in the process.
``` python
class BodieTD(TypedDict): a:int;b:str='foo'
@app.post("/bodietd/")
def bodient(data:BodieTD): return data
print(cli.post('/bodietd/', data=d).text)
```
{"a":1,"b":"foo"}
You can use `TypedDict` to define structured form data with type hints.
In this example, `BodieTD` is a `TypedDict` with `a` (int) and `b` (str)
fields, where `b` has a default value of ‘foo’.
FastHTML automatically converts the incoming form data to a `BodieTD`
instance where keys match the defined fields. Unlike with regular
dictionaries or named tuples, FastHTML respects the type hints in
`TypedDict`, converting values to the specified types when possible
(e.g., converting ‘1’ to the integer 1 for the ‘a’ field).
``` python
class Bodie2:
a:int|None; b:str
def __init__(self, a, b='foo'): store_attr()
@app.post("/bodie2/")
def bodie(d:Bodie2): return f"a: {d.a}; b: {d.b}"
print(cli.post('/bodie2/', data={'a':1}).text)
```
a: 1; b: foo
Custom classes can be used to define structured form data. Here,
`Bodie2` is a custom class with `a` (int|None) and `b` (str) attributes,
where `b` has a default value of ‘foo’. The `store_attr()` function
(from fastcore) automatically assigns constructor parameters to instance
attributes.
FastHTML automatically converts the incoming form data to a `Bodie2`
instance, matching form fields to constructor parameters. It respects
type hints and default values.
``` python
@app.post("/b")
def index(it: Bodie): return Titled("It worked!", P(f"{it.a}, {it.b}"))
s = json.dumps({"b": "Lorem", "a": 15})
print(cli.post('/b', headers={"Content-Type": "application/json", 'hx-request':"1"}, data=s).text)
```
<title>It worked!</title>
<main class="container">
<h1>It worked!</h1>
<p>15, Lorem</p>
</main>
Handler functions can accept JSON data as input, which is automatically
parsed into the specified type. In this example, `it` is of type
`Bodie`, and FastHTML converts the incoming JSON data to a `Bodie`
instance.
The
[`Titled`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#titled)
component is used to create a page with a title and main content. It
automatically generates an `<h1>` with the provided title, wraps the
content in a `<main>` tag with a “container” class, and adds a `title`
to the head.
When making a request with JSON data: - Set the “Content-Type” header to
“application/json” - Provide the JSON data as a string in the `data`
parameter of the request
## Cookies, Sessions, File Uploads, and more
``` python
@rt("/setcookie")
def get(): return cookie('now', datetime.now())
@rt("/getcookie")
def get(now:parsed_date): return f'Cookie was set at time {now.time()}'
print(cli.get('/setcookie').text)
time.sleep(0.01)
cli.get('/getcookie').text
```
'Cookie was set at time 16:53:08.485345'
Handler functions can set and retrieve cookies. In this example:
- The `/setcookie` route sets a cookie named ‘now’ with the current
datetime.
- The `/getcookie` route retrieves the ‘now’ cookie and returns its
value.
The `cookie()` function is used to create a cookie response. FastHTML
automatically converts the datetime object to a string when setting the
cookie, and parses it back to a date object when retrieving it.
``` python
cookie('now', datetime.now())
```
HttpHeader(k='set-cookie', v='now="2024-09-07 16:54:05.275757"; Path=/; SameSite=lax')
The `cookie()` function returns an
[`HttpHeader`](https://AnswerDotAI.github.io/fasthtml/api/core.html#httpheader)
object with the ‘set-cookie’ key. You can return it in a tuple along
with `FT` elements, along with anything else FastHTML supports in
responses.
``` python
app = FastHTML(secret_key='soopersecret')
cli = Client(app)
rt = app.route
```
``` python
@rt("/setsess")
def get(sess, foo:str=''):
now = datetime.now()
sess['auth'] = str(now)
return f'Set to {now}'
@rt("/getsess")
def get(sess): return f'Session time: {sess["auth"]}'
print(cli.get('/setsess').text)
time.sleep(0.01)
cli.get('/getsess').text
```
Set to 2024-09-07 16:56:20.445950
'Session time: 2024-09-07 16:56:20.445950'
Sessions store and retrieve data across requests. To use sessions, you
should to initialize the FastHTML application with a `secret_key`. This
is used to cryptographically sign the cookie used by the session.
The `sess` parameter in handler functions provides access to the session
data. You can set and get session variables using dictionary-style
access.
``` python
@rt("/upload")
async def post(uf:UploadFile): return (await uf.read()).decode()
with open('../../CHANGELOG.md', 'rb') as f:
print(cli.post('/upload', files={'uf':f}, data={'msg':'Hello'}).text[:15])
```
# Release notes
Handler functions can accept file uploads using Starlette’s `UploadFile`
type. In this example:
- The `/upload` route accepts a file upload named `uf`.
- The `UploadFile` object provides an asynchronous `read()` method to
access the file contents.
- We use `await` to read the file content asynchronously and decode it
to a string.
We added `async` to the handler function because it uses `await` to read
the file content asynchronously. In Python, any function that uses
`await` must be declared as `async`. This allows the function to be run
asynchronously, potentially improving performance by not blocking other
operations while waiting for the file to be read.
``` python
app.static_route('.md', static_path='../..')
print(cli.get('/README.md').text[:10])
```
# FastHTML
The `static_route` method of the FastHTML application allows serving
static files with specified extensions from a given directory. In this
example:
- `.md` files are served from the `../..` directory (two levels up from
the current directory).
- Accessing `/README.md` returns the contents of the README.md file from
that directory.
``` python
help(app.static_route_exts)
```
Help on method static_route_exts in module fasthtml.core:
static_route_exts(prefix='/', static_path='.', exts='static') method of fasthtml.core.FastHTML instance
Add a static route at URL path `prefix` with files from `static_path` and `exts` defined by `reg_re_param()`
``` python
app.static_route_exts()
print(cli.get('/README.txt').text[:50])
```
These are the source notebooks for FastHTML.
The `static_route_exts` method of the FastHTML application allows
serving static files with specified extensions from a given directory.
By default:
- It serves files from the current directory (‘.’).
- It uses the ‘static’ regex, which includes common static file
extensions like ‘ico’, ‘gif’, ‘jpg’, ‘css’, ‘js’, etc.
- The URL prefix is set to ‘/’.
The ‘static’ regex is defined by FastHTML using this code:
``` python
reg_re_param("static", "ico|gif|jpg|jpeg|webm|css|js|woff|png|svg|mp4|webp|ttf|otf|eot|woff2|txt|html|map")
```
``` python
@rt("/form-submit/{list_id}")
def options(list_id: str):
headers = {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'POST',
'Access-Control-Allow-Headers': '*',
}
return Response(status_code=200, headers=headers)
print(cli.options('/form-submit/2').headers)
```
Headers({'access-control-allow-origin': '*', 'access-control-allow-methods': 'POST', 'access-control-allow-headers': '*', 'content-length': '0', 'set-cookie': 'session_=eyJhdXRoIjogIjIwMjQtMDktMDcgMTY6NTY6MjAuNDQ1OTUwIiwgIm5hbWUiOiAiMiJ9.Ztv60A.N0b4z5rNg6GT3xCFc8X4DqwcNjQ; path=/; Max-Age=31536000; httponly; samesite=lax'})
FastHTML handlers can handle OPTIONS requests and set custom headers. In
this example:
- The `/form-submit/{list_id}` route handles OPTIONS requests.
- Custom headers are set to allow cross-origin requests (CORS).
- The function returns a Starlette `Response` object with a 200 status
code and the custom headers.
You can return any Starlette Response type from a handler function,
giving you full control over the response when needed.
``` python
def _not_found(req, exc): return Div('nope')
app = FastHTML(exception_handlers={404:_not_found})
cli = Client(app)
rt = app.route
r = cli.get('/')
print(r.text)
```
<!doctype html>
<html>
<head>
<title>FastHTML page</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, viewport-fit=cover">
<script src="https://unpkg.com/htmx.org@next/dist/htmx.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/fasthtml-js@main/fasthtml.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/surreal@main/surreal.js"></script>
<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>
</head>
<body>
<div>nope</div>
</body>
</html>
FastHTML allows you to define custom exception handlers. In this example
we defined a custom 404 (Not Found) handler function `_not_found`, which
returns a `Div` component with the text ‘nope’.
FastHTML includes the full HTML structure, since we returned an HTML
partial.
| md |
FastHTML Docs | ref/live_reload.html.md | # Live Reloading
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
When building your app it can be useful to view your changes in a web
browser as you make them. FastHTML supports live reloading which means
that it watches for any changes to your code and automatically refreshes
the webpage in your browser.
To enable live reloading simply replace
[`FastHTML`](https://AnswerDotAI.github.io/fasthtml/api/core.html#fasthtml)
in your app with `FastHTMLWithLiveReload`.
``` python
from fasthtml.common import *
app = FastHTMLWithLiveReload()
```
Then in your terminal run `uvicorn` with reloading enabled.
uvicorn main:app --reload
**⚠️ Gotchas** - A reload is only triggered when you save your
changes. - `FastHTMLWithLiveReload` should only be used during
development. - If your app spans multiple directories you might need to
use the `--reload-dir` flag to watch all files in each directory. See
the uvicorn [docs](https://www.uvicorn.org/settings/#development) for
more info.
## Live reloading with [`fast_app`](https://AnswerDotAI.github.io/fasthtml/api/fastapp.html#fast_app)
In development the
[`fast_app`](https://AnswerDotAI.github.io/fasthtml/api/fastapp.html#fast_app)
function provides the same functionality. It instantiates the
`FastHTMLWithLiveReload` class if you pass `live=True`:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app, rt = fast_app(live=True)
serve()
```
</div>
Line 3
`fast_app()` instantiates the `FastHTMLWithLiveReload` class.
Line 5
`serve()` is a wrapper around a `uvicorn` call.
To run `main.py` in live reload mode, just do `python main.py`. We
recommend turning off live reload when deploying your app to production.
| md |
FastHTML Docs | tutorials/by_example.html.md | # FastHTML By Example
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
This tutorial provides an alternate introduction to FastHTML by building
out example applications. We also illustrate how to use FastHTML
foundations to create custom web apps. Finally, this document serves as
minimal context for a LLM to turn it into a FastHTML assistant.
Let’s get started.
## FastHTML Basics
FastHTML is *just Python*. You can install it with
`pip install python-fasthtml`. Extensions/components built for it can
likewise be distributed via PyPI or as simple Python files.
The core usage of FastHTML is to define routes, and then to define what
to do at each route. This is similar to the
[FastAPI](https://fastapi.tiangolo.com/) web framework (in fact we
implemented much of the functionality to match the FastAPI usage
examples), but where FastAPI focuses on returning JSON data to build
APIs, FastHTML focuses on returning HTML data.
Here’s a simple FastHTML app that returns a “Hello, World” message:
``` python
from fasthtml.common import FastHTML, serve
app = FastHTML()
@app.get("/")
def home():
return "<h1>Hello, World</h1>"
serve()
```
To run this app, place it in a file, say `app.py`, and then run it with
`python app.py`.
INFO: Will watch for changes in these directories: ['/home/jonathan/fasthtml-example']
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
INFO: Started reloader process [871942] using WatchFiles
INFO: Started server process [871945]
INFO: Waiting for application startup.
INFO: Application startup complete.
If you navigate to <http://127.0.0.1:8000> in a browser, you’ll see your
“Hello, World”. If you edit the `app.py` file and save it, the server
will reload and you’ll see the updated message when you refresh the page
in your browser.
<div>
> **Live Reloading**
>
> You can also enable [live reloading](../ref/live_reload.ipynb) so you
> don’t have to manually refresh your browser while editing it.
</div>
## Constructing HTML
Notice we wrote some HTML in the previous example. We don’t want to do
that! Some web frameworks require that you learn HTML, CSS, JavaScript
AND some templating language AND python. We want to do as much as
possible with just one language. Fortunately, the Python module
[fastcore.xml](https://fastcore.fast.ai/xml.html) has all we need for
constructing HTML from Python, and FastHTML includes all the tags you
need to get started. For example:
``` python
from fasthtml.common import *
page = Html(
Head(Title('Some page')),
Body(Div('Some text, ', A('A link', href='https://example.com'), Img(src="https://placehold.co/200"), cls='myclass')))
print(to_xml(page))
```
<!doctype html></!doctype>
<html>
<head>
<title>Some page</title>
</head>
<body>
<div class="myclass">
Some text,
<a href="https://example.com">A link</a>
<img src="https://placehold.co/200">
</div>
</body>
</html>
``` python
show(page)
```
<!doctype html></!doctype>
<html>
<head>
<title>Some page</title>
</head>
<body>
<div class="myclass">
Some text,
<a href="https://example.com">A link</a>
<img src="https://placehold.co/200">
</div>
</body>
</html>
If that `import *` worries you, you can always import only the tags you
need.
FastHTML is smart enough to know about fastcore.xml, and so you don’t
need to use the `to_xml` function to convert your FT objects to HTML.
You can just return them as you would any other Python object. For
example, if we modify our previous example to use fastcore.xml, we can
return an FT object directly:
``` python
from fasthtml.common import *
app = FastHTML()
@app.get("/")
def home():
page = Html(
Head(Title('Some page')),
Body(Div('Some text, ', A('A link', href='https://example.com'), Img(src="https://placehold.co/200"), cls='myclass')))
return page
serve()
```
This will render the HTML in the browser.
For debugging, you can right-click on the rendered HTML in the browser
and select “Inspect” to see the underlying HTML that was generated.
There you’ll also find the ‘network’ tab, which shows you the requests
that were made to render the page. Refresh and look for the request to
`127.0.0.1` - and you’ll see it’s just a `GET` request to `/`, and the
response body is the HTML you just returned.
You can also use Starlette’s `TestClient` to try it out in a notebook:
``` python
from starlette.testclient import TestClient
client = TestClient(app)
r = client.get("/")
print(r.text)
```
<html>
<head><title>Some page</title>
</head>
<body><div class="myclass">
Some text,
<a href="https://example.com">A link</a>
<img src="https://placehold.co/200">
</div>
</body>
</html>
FastHTML wraps things in an Html tag if you don’t do it yourself (unless
the request comes from htmx, in which case you get the element
directly). See [FT objects and HTML](#ft-objects-and-html) for more on
creating custom components or adding HTML rendering to existing Python
objects. To give the page a non-default title, return a Title before
your main content:
``` python
app = FastHTML()
@app.get("/")
def home():
return Title("Page Demo"), Div(H1('Hello, World'), P('Some text'), P('Some more text'))
client = TestClient(app)
print(client.get("/").text)
```
<!doctype html></!doctype>
<html>
<head>
<title>Page Demo</title>
<meta charset="utf-8"></meta>
<meta name="viewport" content="width=device-width, initial-scale=1, viewport-fit=cover"></meta>
<script src="https://unpkg.com/htmx.org@next/dist/htmx.min.js"></script>
<script src="https://cdn.jsdelivr.net/gh/answerdotai/[email protected]/surreal.js"></script>
<script src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js"></script>
</head>
<body>
<div>
<h1>Hello, World</h1>
<p>Some text</p>
<p>Some more text</p>
</div>
</body>
</html>
We’ll use this pattern often in the examples to follow.
## Defining Routes
The HTTP protocol defines a number of methods (‘verbs’) to send requests
to a server. The most common are GET, POST, PUT, DELETE, and HEAD. We
saw ‘GET’ in action before - when you navigate to a URL, you’re making a
GET request to that URL. We can do different things on a route for
different HTTP methods. For example:
``` python
@app.route("/", methods='get')
def home():
return H1('Hello, World')
@app.route("/", methods=['post', 'put'])
def post_or_put():
return "got a POST or PUT request"
```
This says that when someone navigates to the root URL “/” (i.e. sends a
GET request), they will see the big “Hello, World” heading. When someone
submits a POST or PUT request to the same URL, the server should return
the string “got a post or put request”.
<div>
> **Test the POST request**
>
> You can test the POST request with
> `curl -X POST http://127.0.0.1:8000 -d "some data"`. This sends some
> data to the server, you should see the response “got a post or put
> request” printed in the terminal.
</div>
There are a few other ways you can specify the route+method - FastHTML
has `.get`, `.post`, etc. as shorthand for
`route(..., methods=['get'])`, etc.
``` python
@app.get("/")
def my_function():
return "Hello World from a GET request"
```
Or you can use the `@rt` decorator without a method but specify the
method with the name of the function. For example:
``` python
rt = app.route
@rt("/")
def post():
return "Hello World from a POST request"
```
``` python
client.post("/").text
```
'Hello World from a POST request'
You’re welcome to pick whichever style you prefer. Using routes lets you
show different content on different pages - ‘/home’, ‘/about’ and so on.
You can also respond differently to different kinds of requests to the
same route, as shown above. You can also pass data via the route:
<div class="panel-tabset">
## `@app.get`
``` python
@app.get("/greet/{nm}")
def greet(nm:str):
return f"Good day to you, {nm}!"
client.get("/greet/Dave").text
```
'Good day to you, Dave!'
## `@rt`
``` python
@rt("/greet/{nm}")
def get(nm:str):
return f"Good day to you, {nm}!"
client.get("/greet/Dave").text
```
'Good day to you, Dave!'
</div>
More on this in the [More on Routing and Request
Parameters](#more-on-routing-and-request-parameters) section, which goes
deeper into the different ways to get information from a request.
## Styling Basics
Plain HTML probably isn’t quite what you imagine when you visualize your
beautiful web app. CSS is the go-to language for styling HTML. But
again, we don’t want to learn extra languages unless we absolutely have
to! Fortunately, there are ways to get much more visually appealing
sites by relying on the hard work of others, using existing CSS
libraries. One of our favourites is [PicoCSS](https://picocss.com/). A
common way to add CSS files to web pages is to use a
[`<link>`](https://www.w3schools.com/tags/tag_link.asp) tag inside your
[HTML header](https://www.w3schools.com/tags/tag_header.asp), like this:
``` html
<header>
...
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@latest/css/pico.min.css">
</header>
```
For convenience, FastHTML already defines a Pico component for you with
`picolink`:
``` python
print(to_xml(picolink))
```
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@latest/css/pico.min.css">
<style>:root { --pico-font-size: 100%; }</style>
<div>
> **Note**
>
> `picolink` also includes a `<style>` tag, as we found that setting the
> font-size to 100% to be a good default. We show you how to override
> this below.
</div>
Since we typically want CSS styling on all pages of our app, FastHTML
lets you define a shared HTML header with the `hdrs` argument as shown
below:
``` python
from fasthtml.common import *
css = Style(':root {--pico-font-size:90%,--pico-font-family: Pacifico, cursive;}')
app = FastHTML(hdrs=(picolink, css))
@app.route("/")
def get():
return (Title("Hello World"),
Main(H1('Hello, World'), cls="container"))
```
Line 2
Custom styling to override the pico defaults
Line 3
Define shared headers for all pages
Line 8
As per the [pico docs](https://picocss.com/docs), we put all of our
content inside a `<main>` tag with a class of `container`:
<div>
> **Returning Tuples**
>
> We’re returning a tuple here (a title and the main page). Returning a
> tuple, list, `FT` object, or an object with a `__ft__` method tells
> FastHTML to turn the main body into a full HTML page that includes the
> headers (including the pico link and our custom css) which we passed
> in. This only occurs if the request isn’t from HTMX (for HTMX requests
> we need only return the rendered components).
</div>
You can check out the Pico [examples](https://picocss.com/examples) page
to see how different elements will look. If everything is working, the
page should now render nice text with our custom font, and it should
respect the user’s light/dark mode preferences too.
If you want to [override the default
styles](https://picocss.com/docs/css-variables) or add more custom CSS,
you can do so by adding a `<style>` tag to the headers as shown above.
So you are allowed to write CSS to your heart’s content - we just want
to make sure you don’t necessarily have to! Later on we’ll see examples
using other component libraries and tailwind css to do more fancy
styling things, along with tips to get an LLM to write all those fiddly
bits so you don’t have to.
## Web Page -\> Web App
Showing content is all well and good, but we typically expect a bit more
*interactivity* from something calling itself a web app! So, let’s add a
few different pages, and use a form to let users add messages to a list:
``` python
app = FastHTML()
messages = ["This is a message, which will get rendered as a paragraph"]
@app.get("/")
def home():
return Main(H1('Messages'),
*[P(msg) for msg in messages],
A("Link to Page 2 (to add messages)", href="/page2"))
@app.get("/page2")
def page2():
return Main(P("Add a message with the form below:"),
Form(Input(type="text", name="data"),
Button("Submit"),
action="/", method="post"))
@app.post("/")
def add_message(data:str):
messages.append(data)
return home()
```
We re-render the entire homepage to show the newly added message. This
is fine, but modern web apps often don’t re-render the entire page, they
just update a part of the page. In fact even very complicated
applications are often implemented as ‘Single Page Apps’ (SPAs). This is
where HTMX comes in.
## HTMX
[HTMX](https://htmx.org/) addresses some key limitations of HTML. In
vanilla HTML, links can trigger a GET request to show a new page, and
forms can send requests containing data to the server. A lot of ‘Web
1.0’ design revolved around ways to use these to do everything we
wanted. But why should only *some* elements be allowed to trigger
requests? And why should we refresh the *entire page* with the result
each time one does? HTMX extends HTML to allow us to trigger requests
from *any* element on all kinds of events, and to update a part of the
page without refreshing the entire page. It’s a powerful tool for
building modern web apps.
It does this by adding attributes to HTML tags to make them do things.
For example, here’s a page with a counter and a button that increments
it:
``` python
app = FastHTML()
count = 0
@app.get("/")
def home():
return Title("Count Demo"), Main(
H1("Count Demo"),
P(f"Count is set to {count}", id="count"),
Button("Increment", hx_post="/increment", hx_target="#count", hx_swap="innerHTML")
)
@app.post("/increment")
def increment():
print("incrementing")
global count
count += 1
return f"Count is set to {count}"
```
The button triggers a POST request to `/increment` (since we set
`hx_post="/increment"`), which increments the count and returns the new
count. The `hx_target` attribute tells HTMX where to put the result. If
no target is specified it replaces the element that triggered the
request. The `hx_swap` attribute specifies how it adds the result to the
page. Useful options are:
- *`innerHTML`*: Replace the target element’s content with the result.
- *`outerHTML`*: Replace the target element with the result.
- *`beforebegin`*: Insert the result before the target element.
- *`beforeend`*: Insert the result inside the target element, after its
last child.
- *`afterbegin`*: Insert the result inside the target element, before
its first child.
- *`afterend`*: Insert the result after the target element.
You can also use an hx_swap of `delete` to delete the target element
regardless of response, or of `none` to do nothing.
By default, requests are triggered by the “natural” event of an
element - click in the case of a button (and most other elements). You
can also specify different triggers, along with various modifiers - see
the [HTMX docs](https://htmx.org/docs/#triggers) for more.
This pattern of having elements trigger requests that modify or replace
other elements is a key part of the HTMX philosophy. It takes a little
getting used to, but once mastered it is extremely powerful.
### Replacing Elements Besides the Target
Sometimes having a single target is not enough, and we’d like to specify
some additional elements to update or remove. In these cases, returning
elements with an id that matches the element to be replaced and
`hx_swap_oob='true'` will replace those elements too. We’ll use this in
the next example to clear an input field when we submit a form.
## Full Example \#1 - ToDo App
The canonical demo web app! A TODO list. Rather than create yet another
variant for this tutorial, we recommend starting with this video
tutorial from Jeremy:
<https://www.youtube.com/embed/Auqrm7WFc0I>
<figure>
<img src="by_example_files/figure-commonmark/cell-53-1-image.png"
alt="image.png" />
<figcaption aria-hidden="true">image.png</figcaption>
</figure>
We’ve made a number of variants of this app - so in addition to the
version shown in the video you can browse
[this](https://github.com/AnswerDotAI/fasthtml-tut) series of examples
with increasing complexity, the heavily-commented [“idiomatic” version
here](https://github.com/AnswerDotAI/fasthtml/blob/main/examples/adv_app.py),
and the
[example](https://github.com/AnswerDotAI/fasthtml-example/tree/main/01_todo_app)
linked from the [FastHTML homepage](https://fastht.ml/).
## Full Example \#2 - Image Generation App
Let’s create an image generation app. We’d like to wrap a text-to-image
model in a nice UI, where the user can type in a prompt and see a
generated image appear. We’ll use a model hosted by
[Replicate](https://replicate.com) to actually generate the images.
Let’s start with the homepage, with a form to submit prompts and a div
to hold the generated images:
``` python
# Main page
@app.get("/")
def get():
inp = Input(id="new-prompt", name="prompt", placeholder="Enter a prompt")
add = Form(Group(inp, Button("Generate")), hx_post="/", target_id='gen-list', hx_swap="afterbegin")
gen_list = Div(id='gen-list')
return Title('Image Generation Demo'), Main(H1('Magic Image Generation'), add, gen_list, cls='container')
```
Submitting the form will trigger a POST request to `/`, so next we need
to generate an image and add it to the list. One problem: generating
images is slow! We’ll start the generation in a separate thread, but
this now surfaces a different problem: we want to update the UI right
away, but our image will only be ready a few seconds later. This is a
common pattern - think about how often you see a loading spinner online.
We need a way to return a temporary bit of UI which will eventually be
replaced by the final image. Here’s how we might do this:
``` python
def generation_preview(id):
if os.path.exists(f"gens/{id}.png"):
return Div(Img(src=f"/gens/{id}.png"), id=f'gen-{id}')
else:
return Div("Generating...", id=f'gen-{id}',
hx_post=f"/generations/{id}",
hx_trigger='every 1s', hx_swap='outerHTML')
@app.post("/generations/{id}")
def get(id:int): return generation_preview(id)
@app.post("/")
def post(prompt:str):
id = len(generations)
generate_and_save(prompt, id)
generations.append(prompt)
clear_input = Input(id="new-prompt", name="prompt", placeholder="Enter a prompt", hx_swap_oob='true')
return generation_preview(id), clear_input
@threaded
def generate_and_save(prompt, id): ...
```
The form sends the prompt to the `/` route, which starts the generation
in a separate thread then returns two things:
- A generation preview element that will be added to the top of the
`gen-list` div (since that is the target_id of the form which
triggered the request)
- An input field that will replace the form’s input field (that has the
same id), using the hx_swap_oob=‘true’ trick. This clears the prompt
field so the user can type another prompt.
The generation preview first returns a temporary “Generating…” message,
which polls the `/generations/{id}` route every second. This is done by
setting hx_post to the route and hx_trigger to ‘every 1s’. The
`/generations/{id}` route returns the preview element every second until
the image is ready, at which point it returns the final image. Since the
final image replaces the temporary one (hx_swap=‘outerHTML’), the
polling stops running and the generation preview is now complete.
This works nicely - the user can submit several prompts without having
to wait for the first one to generate, and as the images become
available they are added to the list. You can see the full code of this
version
[here](https://github.com/AnswerDotAI/fasthtml-example/blob/main/image_app_simple/draft1.py).
### Again, with Style
The app is functional, but can be improved. The [next
version](https://github.com/AnswerDotAI/fasthtml-example/blob/main/image_app_simple/main.py)
adds more stylish generation previews, lays out the images in a grid
layout that is responsive to different screen sizes, and adds a database
to track generations and make them persistent. The database part is very
similar to the todo list example, so let’s just quickly look at how we
add the nice grid layout. This is what the result looks like:
<figure>
<img src="by_example_files/figure-commonmark/cell-58-1-image.png"
alt="image.png" />
<figcaption aria-hidden="true">image.png</figcaption>
</figure>
Step one was looking around for existing components. The Pico CSS
library we’ve been using has a rudimentary grid but recommends using an
alternative layout system. One of the options listed was
[Flexbox](http://flexboxgrid.com/).
To use Flexbox you create a “row” with one or more elements. You can
specify how wide things should be with a specific syntax in the class
name. For example, `col-xs-12` means a box that will take up 12 columns
(out of 12 total) of the row on extra small screens, `col-sm-6` means a
column that will take up 6 columns of the row on small screens, and so
on. So if you want four columns on large screens you would use
`col-lg-3` for each item (i.e. each item is using 3 columns out of 12).
``` html
<div class="row">
<div class="col-xs-12">
<div class="box">This takes up the full width</div>
</div>
</div>
```
This was non-intuitive to me. Thankfully ChatGPT et al know web stuff
quite well, and we can also experiment in a notebook to test things out:
``` python
grid = Html(
Link(rel="stylesheet", href="https://cdnjs.cloudflare.com/ajax/libs/flexboxgrid/6.3.1/flexboxgrid.min.css", type="text/css"),
Div(
Div(Div("This takes up the full width", cls="box", style="background-color: #800000;"), cls="col-xs-12"),
Div(Div("This takes up half", cls="box", style="background-color: #008000;"), cls="col-xs-6"),
Div(Div("This takes up half", cls="box", style="background-color: #0000B0;"), cls="col-xs-6"),
cls="row", style="color: #fff;"
)
)
show(grid)
```
<!doctype html></!doctype>
<html>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/flexboxgrid/6.3.1/flexboxgrid.min.css" type="text/css">
<div class="row" style="color: #fff;">
<div class="col-xs-12">
<div class="box" style="background-color: #800000;">This takes up the full width</div>
</div>
<div class="col-xs-6">
<div class="box" style="background-color: #008000;">This takes up half</div>
</div>
<div class="col-xs-6">
<div class="box" style="background-color: #0000B0;">This takes up half</div>
</div>
</div>
</html>
Aside: when in doubt with CSS stuff, add a background color or a border
so you can see what’s happening!
Translating this into our app, we have a new homepage with a
`div (class="row")` to store the generated images / previews, and a
`generation_preview` function that returns boxes with the appropriate
classes and styles to make them appear in the grid. I chose a layout
with different numbers of columns for different screen sizes, but you
could also *just* specify the `col-xs` class if you wanted the same
layout on all devices.
``` python
gridlink = Link(rel="stylesheet", href="https://cdnjs.cloudflare.com/ajax/libs/flexboxgrid/6.3.1/flexboxgrid.min.css", type="text/css")
app = FastHTML(hdrs=(picolink, gridlink))
# Main page
@app.get("/")
def get():
inp = Input(id="new-prompt", name="prompt", placeholder="Enter a prompt")
add = Form(Group(inp, Button("Generate")), hx_post="/", target_id='gen-list', hx_swap="afterbegin")
gen_containers = [generation_preview(g) for g in gens(limit=10)] # Start with last 10
gen_list = Div(*gen_containers[::-1], id='gen-list', cls="row") # flexbox container: class = row
return Title('Image Generation Demo'), Main(H1('Magic Image Generation'), add, gen_list, cls='container')
# Show the image (if available) and prompt for a generation
def generation_preview(g):
grid_cls = "box col-xs-12 col-sm-6 col-md-4 col-lg-3"
image_path = f"{g.folder}/{g.id}.png"
if os.path.exists(image_path):
return Div(Card(
Img(src=image_path, alt="Card image", cls="card-img-top"),
Div(P(B("Prompt: "), g.prompt, cls="card-text"),cls="card-body"),
), id=f'gen-{g.id}', cls=grid_cls)
return Div(f"Generating gen {g.id} with prompt {g.prompt}",
id=f'gen-{g.id}', hx_get=f"/gens/{g.id}",
hx_trigger="every 2s", hx_swap="outerHTML", cls=grid_cls)
```
You can see the final result in
[main.py](https://github.com/AnswerDotAI/fasthtml-example/blob/main/image_app_simple/main.py)
in the `image_app_simple` example directory, along with info on
deploying it (tl;dr don’t!). We’ve also deployed a version that only
shows *your* generations (tied to browser session) and has a credit
system to save our bank accounts. You can access that
[here](https://image-gen-public-credit-pool.replit.app/). Now for the
next question: how do we keep track of different users?
### Again, with Sessions
At the moment everyone sees all images! How do we keep some sort of
unique identifier tied to a user? Before going all the way to setting up
users, login pages etc., let’s look at a way to at least limit
generations to the user’s *session*. You could do this manually with
cookies. For convenience and security, fasthtml (via Starlette) has a
special mechanism for storing small amounts of data in the user’s
browser via the `session` argument to your route. This acts like a
dictionary and you can set and get values from it. For example, here we
look for a `session_id` key, and if it doesn’t exist we generate a new
one:
``` python
@app.get("/")
def get(session):
if 'session_id' not in session: session['session_id'] = str(uuid.uuid4())
return H1(f"Session ID: {session['session_id']}")
```
Refresh the page a few times - you’ll notice that the session ID remains
the same. If you clear your browsing data, you’ll get a new session ID.
And if you load the page in a different browser (but not a different
tab), you’ll get a new session ID. This will persist within the current
browser, letting us use it as a key for our generations. As a bonus,
someone can’t spoof this session id by passing it in another way (for
example, sending a query parameter). Behind the scenes, the data *is*
stored in a browser cookie but it is signed with a secret key that stops
the user or anyone nefarious from being able to tamper with it. The
cookie is decoded back into a dictionary by something called a
middleware function, which we won’t cover here. All you need to know is
that we can use this to store bits of state in the user’s browser.
In the image app example, we can add a `session_id` column to our
database, and modify our homepage like so:
``` python
@app.get("/")
def get(session):
if 'session_id' not in session: session['session_id'] = str(uuid.uuid4())
inp = Input(id="new-prompt", name="prompt", placeholder="Enter a prompt")
add = Form(Group(inp, Button("Generate")), hx_post="/", target_id='gen-list', hx_swap="afterbegin")
gen_containers = [generation_preview(g) for g in gens(limit=10, where=f"session_id == '{session['session_id']}'")]
...
```
So we check if the session id exists in the session, add one if not, and
then limit the generations shown to only those tied to this session id.
We filter the database with a where clause - see \[TODO link Jeremy’s
example for a more reliable way to do this\]. The only other change we
need to make is to store the session id in the database when a
generation is made. You can check out this version
[here](https://github.com/AnswerDotAI/fasthtml-example/blob/main/image_app_session_credits/session.py).
You could instead write this app without relying on a database at all -
simply storing the filenames of the generated images in the session, for
example. But this more general approach of linking some kind of unique
session identifier to users or data in our tables is a useful general
pattern for more complex examples.
### Again, with Credits!
Generating images with replicate costs money. So next let’s add a pool
of credits that get used up whenever anyone generates an image. To
recover our lost funds, we’ll also set up a payment system so that
generous users can buy more credits for everyone. You could modify this
to let users buy credits tied to their session ID, but at that point you
risk having angry customers losing their money after wiping their
browser history, and should consider setting up proper account
management :)
Taking payments with Stripe is intimidating but very doable. [Here’s a
tutorial](https://testdriven.io/blog/flask-stripe-tutorial/) that shows
the general principle using Flask. As with other popular tasks in the
web-dev world, ChatGPT knows a lot about Stripe - but you should
exercise extra caution when writing code that handles money!
For the [finished
example](https://github.com/AnswerDotAI/fasthtml-example/blob/main/image_app_session_credits/main.py)
we add the bare minimum:
- A way to create a Stripe checkout session and redirect the user to the
session URL
- ‘Success’ and ‘Cancel’ routes to handle the result of the checkout
- A route that listens for a webhook from Stripe to update the number of
credits when a payment is made.
In a typical application you’ll want to keep track of which users make
payments, catch other kinds of stripe events and so on. This example is
more a ‘this is possible, do your own research’ than ‘this is how you do
it’. But hopefully it does illustrate the key idea: there is no magic
here. Stripe (and many other technologies) relies on sending users to
different routes and shuttling data back and forth in requests. And we
know how to do that!
## More on Routing and Request Parameters
There are a number of ways information can be passed to the server. When
you specify arguments to a route, FastHTML will search the request for
values with the same name, and convert them to the correct type. In
order, it searches
- The path parameters
- The query parameters
- The cookies
- The headers
- The session
- Form data
There are also a few special arguments
- `request` (or any prefix like `req`): gets the raw Starlette `Request`
object
- `session` (or any prefix like `sess`): gets the session object
- `auth`
- `htmx`
- `app`
In this section let’s quickly look at some of these in action.
``` python
app = FastHTML()
cli = TestClient(app)
```
Part of the route (path parameters):
``` python
@app.get('/user/{nm}')
def _(nm:str): return f"Good day to you, {nm}!"
cli.get('/user/jph').text
```
'Good day to you, jph!'
Matching with a regex:
``` python
reg_re_param("imgext", "ico|gif|jpg|jpeg|webm")
@app.get(r'/static/{path:path}{fn}.{ext:imgext}')
def get_img(fn:str, path:str, ext:str): return f"Getting {fn}.{ext} from /{path}"
cli.get('/static/foo/jph.ico').text
```
'Getting jph.ico from /foo/'
Using an enum (try using a string that isn’t in the enum):
``` python
ModelName = str_enum('ModelName', "alexnet", "resnet", "lenet")
@app.get("/models/{nm}")
def model(nm:ModelName): return nm
print(cli.get('/models/alexnet').text)
```
alexnet
Casting to a Path:
``` python
@app.get("/files/{path}")
def txt(path: Path): return path.with_suffix('.txt')
print(cli.get('/files/foo').text)
```
foo.txt
An integer with a default value:
``` python
fake_db = [{"name": "Foo"}, {"name": "Bar"}]
@app.get("/items/")
def read_item(idx:int|None = 0): return fake_db[idx]
print(cli.get('/items/?idx=1').text)
```
{"name":"Bar"}
``` python
print(cli.get('/items/').text)
```
{"name":"Foo"}
Boolean values (takes anything “truthy” or “falsy”):
``` python
@app.get("/booly/")
def booly(coming:bool=True): return 'Coming' if coming else 'Not coming'
print(cli.get('/booly/?coming=true').text)
```
Coming
``` python
print(cli.get('/booly/?coming=no').text)
```
Not coming
Getting dates:
``` python
@app.get("/datie/")
def datie(d:parsed_date): return d
date_str = "17th of May, 2024, 2p"
print(cli.get(f'/datie/?d={date_str}').text)
```
2024-05-17 14:00:00
Matching a dataclass:
``` python
from dataclasses import dataclass, asdict
@dataclass
class Bodie:
a:int;b:str
@app.route("/bodie/{nm}")
def post(nm:str, data:Bodie):
res = asdict(data)
res['nm'] = nm
return res
cli.post('/bodie/me', data=dict(a=1, b='foo')).text
```
'{"a":1,"b":"foo","nm":"me"}'
### Cookies
Cookies can be set via a Starlette Response object, and can be read back
by specifying the name:
``` python
from datetime import datetime
@app.get("/setcookie")
def setc(req):
now = datetime.now()
res = Response(f'Set to {now}')
res.set_cookie('now', str(now))
return res
cli.get('/setcookie').text
```
'Set to 2024-07-20 23:14:54.364793'
``` python
@app.get("/getcookie")
def getc(now:parsed_date): return f'Cookie was set at time {now.time()}'
cli.get('/getcookie').text
```
'Cookie was set at time 23:14:54.364793'
### User Agent and HX-Request
An argument of `user_agent` will match the header `User-Agent`. This
holds for special headers like `HX-Request` (used by HTMX to signal when
a request comes from an HTMX request) - the general pattern is that “-”
is replaced with “\_” and strings are turned to lowercase.
``` python
@app.get("/ua")
async def ua(user_agent:str): return user_agent
cli.get('/ua', headers={'User-Agent':'FastHTML'}).text
```
'FastHTML'
``` python
@app.get("/hxtest")
def hxtest(htmx): return htmx.request
cli.get('/hxtest', headers={'HX-Request':'1'}).text
```
'1'
### Starlette Requests
If you add an argument called `request`(or any prefix of that, for
example `req`) it will be populated with the Starlette `Request` object.
This is useful if you want to do your own processing manually. For
example, although FastHTML will parse forms for you, you could instead
get form data like so:
``` python
@app.get("/form")
async def form(request:Request):
form_data = await request.form()
a = form_data.get('a')
```
See the [Starlette docs](https://starlette.io/docs/) for more
information on the `Request` object.
### Starlette Responses
You can return a Starlette Response object from a route to control the
response. For example:
``` python
@app.get("/redirect")
def redirect():
return RedirectResponse(url="/")
```
We used this to set cookies in the previous example. See the [Starlette
docs](https://starlette.io/docs/) for more information on the `Response`
object.
### Static Files
We often want to serve static files like images. This is easily done!
For common file types (images, CSS etc) we can create a route that
returns a Starlette `FileResponse` like so:
``` python
# For images, CSS, etc.
@app.get("/{fname:path}.{ext:static}")
def static(fname: str, ext: str):
return FileResponse(f'{fname}.{ext}')
```
You can customize it to suit your needs (for example, only serving files
in a certain directory). You’ll notice some variant of this route in all
our complete examples - even for apps with no static files the browser
will typically request a `/favicon.ico` file, for example, and as the
astute among you will have noticed this has sparked a bit of competition
between Johno and Jeremy regarding which country flag should serve as
the default!
### WebSockets
For certain applications such as multiplayer games, websockets can be a
powerful feature. Luckily HTMX and FastHTML has you covered! Simply
specify that you wish to include the websocket header extension from
HTMX:
``` python
app = FastHTML(ws_hdr=True)
rt = app.route
```
With that, you are now able to specify the different websocket specific
HTMX goodies. For example, say we have a website we want to setup a
websocket, you can simply:
``` python
def mk_inp(): return Input(id='msg')
@rt('/')
async def get(request):
cts = Div(
Div(id='notifications'),
Form(mk_inp(), id='form', ws_send=True),
hx_ext='ws', ws_connect='/ws')
return Titled('Websocket Test', cts)
```
And this will setup a connection on the route `/ws` along with a form
that will send a message to the websocket whenever the form is
submitted. Let’s go ahead and handle this route:
``` python
@app.ws('/ws')
async def ws(msg:str, send):
await send(Div('Hello ' + msg, id="notifications"))
await sleep(2)
return Div('Goodbye ' + msg, id="notifications"), mk_inp()
```
One thing you might have noticed is a lack of target id for our
websocket trigger for swapping HTML content. This is because HTMX always
swaps content with websockets with Out of Band Swaps. Therefore, HTMX
will look for the id in the returned HTML content from the server for
determining what to swap. To send stuff to the client, you can either
use the `send` parameter or simply return the content or both!
Now, sometimes you might want to perform actions when a client connects
or disconnects such as add or remove a user from a player queue. To hook
into these events, you can pass your connection or disconnection
function to the `app.ws` decorator:
``` python
async def on_connect(send):
print('Connected!')
await send(Div('Hello, you have connected', id="notifications"))
async def on_disconnect(ws):
print('Disconnected!')
@app.ws('/ws', conn=on_connect, disconn=on_disconnect)
async def ws(msg:str, send):
await send(Div('Hello ' + msg, id="notifications"))
await sleep(2)
return Div('Goodbye ' + msg, id="notifications"), mk_inp()
```
## Full Example \#3 - Chatbot Example with DaisyUI Components
Let’s go back to the topic of adding components or styling beyond the
simple PicoCSS examples so far. How might we adopt a component or
framework? In this example, let’s build a chatbot UI leveraging the
[DaisyUI chat bubble](https://daisyui.com/components/chat/). The final
result will look like this:
<figure>
<img src="by_example_files/figure-commonmark/cell-101-1-image.png"
alt="image.png" />
<figcaption aria-hidden="true">image.png</figcaption>
</figure>
At first glance, DaisyUI’s chat component looks quite intimidating. The
examples look like this:
``` html
<div class="chat chat-start">
<div class="chat-image avatar">
<div class="w-10 rounded-full">
<img alt="Tailwind CSS chat bubble component" src="https://img.daisyui.com/images/stock/photo-1534528741775-53994a69daeb.jpg" />
</div>
</div>
<div class="chat-header">
Obi-Wan Kenobi
<time class="text-xs opacity-50">12:45</time>
</div>
<div class="chat-bubble">You were the Chosen One!</div>
<div class="chat-footer opacity-50">
Delivered
</div>
</div>
<div class="chat chat-end">
<div class="chat-image avatar">
<div class="w-10 rounded-full">
<img alt="Tailwind CSS chat bubble component" src="https://img.daisyui.com/images/stock/photo-1534528741775-53994a69daeb.jpg" />
</div>
</div>
<div class="chat-header">
Anakin
<time class="text-xs opacity-50">12:46</time>
</div>
<div class="chat-bubble">I hate you!</div>
<div class="chat-footer opacity-50">
Seen at 12:46
</div>
</div>
```
We have several things going for us however.
- ChatGPT knows DaisyUI and Tailwind (DaisyUI is a Tailwind component
library)
- We can build things up piece by piece with AI standing by to help.
<https://h2x.answer.ai/> is a tool that can convert HTML to FT
(fastcore.xml) and back, which is useful for getting a quick starting
point when you have an HTML example to start from.
We can strip out some unnecessary bits and try to get the simplest
possible example working in a notebook first:
``` python
# Loading tailwind and daisyui
headers = (Script(src="https://cdn.tailwindcss.com"),
Link(rel="stylesheet", href="https://cdn.jsdelivr.net/npm/[email protected]/dist/full.min.css"))
# Displaying a single message
d = Div(
Div("Chat header here", cls="chat-header"),
Div("My message goes here", cls="chat-bubble chat-bubble-primary"),
cls="chat chat-start"
)
# show(Html(*headers, d)) # uncomment to view
```
Now we can extend this to render multiple messages, with the message
being on the left (`chat-start`) or right (`chat-end`) depending on the
role. While we’re at it, we can also change the color
(`chat-bubble-primary`) of the message and put them all in a `chat-box`
div:
``` python
messages = [
{"role":"user", "content":"Hello"},
{"role":"assistant", "content":"Hi, how can I assist you?"}
]
def ChatMessage(msg):
return Div(
Div(msg['role'], cls="chat-header"),
Div(msg['content'], cls=f"chat-bubble chat-bubble-{'primary' if msg['role'] == 'user' else 'secondary'}"),
cls=f"chat chat-{'end' if msg['role'] == 'user' else 'start'}")
chatbox = Div(*[ChatMessage(msg) for msg in messages], cls="chat-box", id="chatlist")
# show(Html(*headers, chatbox)) # Uncomment to view
```
Next, it was back to the ChatGPT to tweak the chat box so it wouldn’t
grow as messages were added. I asked:
"I have something like this (it's working now)
[code]
The messages are added to this div so it grows over time.
Is there a way I can set it's height to always be 80% of the total window height with a scroll bar if needed?"
Based on this query GPT4o helpfully shared that “This can be achieved
using Tailwind CSS utility classes. Specifically, you can use h-\[80vh\]
to set the height to 80% of the viewport height, and overflow-y-auto to
add a vertical scroll bar when needed.”
To put it another way: none of the CSS classes in the following example
were written by a human, and what edits I did make were informed by
advice from the AI that made it relatively painless!
The actual chat functionality of the app is based on our
[claudette](https://claudette.answer.ai/) library. As with the image
example, we face a potential hiccup in that getting a response from an
LLM is slow. We need a way to have the user message added to the UI
immediately, and then have the response added once it’s available. We
could do something similar to the image generation example above, or use
websockets. Check out the [full
example](https://github.com/AnswerDotAI/fasthtml-example/tree/main/02_chatbot)
for implementations of both, along with further details.
## Full Example \#4 - Multiplayer Game of Life Example with Websockets
Let’s see how we can implement a collaborative website using Websockets
in FastHTML. To showcase this, we will use the famous [Conway’s Game of
Life](https://en.wikipedia.org/wiki/Conway's_Game_of_Life), which is a
game that takes place in a grid world. Each cell in the grid can be
either alive or dead. The cell’s state is initially given by a user
before the game is started and then evolves through the iteration of the
grid world once the clock starts. Whether a cell’s state will change
from the previous state depends on simple rules based on its neighboring
cells’ states. Here is the standard Game of Life logic implemented in
Python courtesy of ChatGPT:
``` python
grid = [[0 for _ in range(20)] for _ in range(20)]
def update_grid(grid: list[list[int]]) -> list[list[int]]:
new_grid = [[0 for _ in range(20)] for _ in range(20)]
def count_neighbors(x, y):
directions = [(-1, -1), (-1, 0), (-1, 1), (0, -1), (0, 1), (1, -1), (1, 0), (1, 1)]
count = 0
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < len(grid) and 0 <= ny < len(grid[0]): count += grid[nx][ny]
return count
for i in range(len(grid)):
for j in range(len(grid[0])):
neighbors = count_neighbors(i, j)
if grid[i][j] == 1:
if neighbors < 2 or neighbors > 3: new_grid[i][j] = 0
else: new_grid[i][j] = 1
elif neighbors == 3: new_grid[i][j] = 1
return new_grid
```
This would be a very dull game if we were to run it, since the initial
state of everything would remain dead. Therefore, we need a way of
letting the user give an initial state before starting the game.
FastHTML to the rescue!
``` python
def Grid():
cells = []
for y, row in enumerate(game_state['grid']):
for x, cell in enumerate(row):
cell_class = 'alive' if cell else 'dead'
cell = Div(cls=f'cell {cell_class}', hx_put='/update', hx_vals={'x': x, 'y': y}, hx_swap='none', hx_target='#gol', hx_trigger='click')
cells.append(cell)
return Div(*cells, id='grid')
@rt('/update')
async def put(x: int, y: int):
grid[y][x] = 1 if grid[y][x] == 0 else 0
```
Above is a component for representing the game’s state that the user can
interact with and update on the server using cool HTMX features such as
`hx_vals` for determining which cell was clicked to make it dead or
alive. Now, you probably noticed that the HTTP request in this case is a
PUT request, which does not return anything and this means our client’s
view of the grid world and the server’s game state will immediately
become out of sync :(. We could of course just return a new Grid
component with the updated state, but that would only work for a single
client, if we had more, they quickly get out of sync with each other and
the server. Now Websockets to the rescue!
Websockets are a way for the server to keep a persistent connection with
clients and send data to the client without explicitly being requested
for information, which is not possible with HTTP. Luckily FastHTML and
HTMX work well with Websockets. Simply state you wish to use websockets
for your app and define a websocket route:
``` python
...
app = FastHTML(hdrs=(picolink, gridlink, css, htmx_ws), ws_hdr=True)
player_queue = []
async def update_players():
for i, player in enumerate(player_queue):
try: await player(Grid())
except: player_queue.pop(i)
async def on_connect(send): player_queue.append(send)
async def on_disconnect(send): await update_players()
@app.ws('/gol', conn=on_connect, disconn=on_disconnect)
async def ws(msg:str, send): pass
def Home(): return Title('Game of Life'), Main(gol, Div(Grid(), id='gol', cls='row center-xs'), hx_ext="ws", ws_connect="/gol")
@rt('/update')
async def put(x: int, y: int):
grid[y][x] = 1 if grid[y][x] == 0 else 0
await update_players()
...
```
Here we simply keep track of all the players that have connected or
disconnected to our site and when an update occurs, we send updates to
all the players still connected via websockets. Via HTMX, you are still
simply exchanging HTML from the server to the client and will swap in
the content based on how you setup your `hx_swap` attribute. There is
only one difference, that being all swaps are OOB. You can find more
information on the HTMX websocket extension documentation page
[here](https://github.com/bigskysoftware/htmx-extensions/blob/main/src/ws/README.md).
You can find a full fledge hosted example of this app
[here](https://game-of-life-production-ed7f.up.railway.app/).
## FT objects and HTML
These FT objects create a ‘FastTag’ structure \[tag,children,attrs\] for
`to_xml()`. When we call `Div(...)`, the elements we pass in are the
children. Attributes are passed in as keywords. `class` and `for` are
special words in python, so we use `cls`, `klass` or `_class` instead of
`class` and `fr` or `_for` instead of `for`. Note these objects are just
3-element lists - you can create custom ones too as long as they’re also
3-element lists. Alternately, leaf nodes can be strings instead (which
is why you can do `Div('some text')`). If you pass something that isn’t
a 3-element list or a string, it will be converted to a string using
str()… unless (our final trick) you define a `__ft__` method that will
run before str(), so you can render things a custom way.
For example, here’s one way we could make a custom class that can be
rendered into HTML:
``` python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __ft__(self):
return ['div', [f'{self.name} is {self.age} years old.'], {}]
p = Person('Jonathan', 28)
print(to_xml(Div(p, "more text", cls="container")))
```
<div class="container">
<div>Jonathan is 28 years old.</div>
more text
</div>
In the examples, you’ll see we often patch in `__ft__` methods to
existing classes to control how they’re rendered. For example, if Person
didn’t have a `__ft__` method or we wanted to override it, we could add
a new one like this:
``` python
from fastcore.all import patch
@patch
def __ft__(self:Person):
return Div("Person info:", Ul(Li("Name:",self.name), Li("Age:", self.age)))
show(p)
```
<div>
Person info:
<ul>
<li>
Name:
Jonathan
</li>
<li>
Age:
28
</li>
</ul>
</div>
Some tags from fastcore.xml are overwritten by fasthtml.core and a few
are further extended by fasthtml.xtend using this method. Over time, we
hope to see others developing custom components too, giving us a larger
and larger ecosystem of reusable components.
## Custom Scripts and Styling
There are many popular JavaScript and CSS libraries that can be used via
a simple
[`Script`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#script)
or
[`Style`](https://AnswerDotAI.github.io/fasthtml/api/xtend.html#style)
tag. But in some cases you will need to write more custom code.
FastHTML’s
[js.py](https://github.com/AnswerDotAI/fasthtml/blob/main/fasthtml/js.py)
contains a few examples that may be useful as reference.
For example, to use the [marked.js](https://marked.js.org/) library to
render markdown in a div, including in components added after the page
has loaded via htmx, we do something like this:
``` javascript
import { marked } from "https://cdn.jsdelivr.net/npm/marked/lib/marked.esm.js";
import { proc_htmx} from "https://cdn.jsdelivr.net/gh/answerdotai/fasthtml-js/fasthtml.js";
proc_htmx('%s', e => e.innerHTML = marked.parse(e.textContent));
```
`proc_htmx` is a shortcut that we wrote to apply a function to elements
matching a selector, including the element that triggered the event.
Here’s the code for reference:
``` javascript
export function proc_htmx(sel, func) {
htmx.onLoad(elt => {
const elements = htmx.findAll(elt, sel);
if (elt.matches(sel)) elements.unshift(elt)
elements.forEach(func);
});
}
```
The [AI Pictionary
example](https://github.com/AnswerDotAI/fasthtml-example/tree/main/ai_pictionary)
uses a larger chunk of custom JavaScript to handle the drawing canvas.
It’s a good example of the type of application where running code on the
client side makes the most sense, but still shows how you can integrate
it with FastHTML on the server side to add functionality (like the AI
responses) easily.
Adding styling with custom CSS and libraries such as tailwind is done
the same way we add custom JavaScript. The [doodle
example](https://github.com/AnswerDotAI/fasthtml-example/tree/main/doodle)
uses [Doodle.CSS](https://github.com/chr15m/DoodleCSS) to style the page
in a quirky way.
## Deploying Your App
We can deploy FastHTML almost anywhere you can deploy python apps. We’ve
tested Railway, Replit,
[HuggingFace](https://github.com/AnswerDotAI/fasthtml-hf), and
[PythonAnywhere](https://github.com/AnswerDotAI/fasthtml-example/blob/main/deploying-to-pythonanywhere.md).
### Railway
1. [Install the Railway CLI](https://docs.railway.app/guides/cli) and
sign up for an account.
2. Set up a folder with our app as `main.py`
3. In the folder, run `railway login`.
4. Use the `fh_railway_deploy` script to deploy our project:
``` bash
fh_railway_deploy MY_APP_NAME
```
What the script does for us:
4. Do we have an existing railway project?
- Yes: Link the project folder to our existing Railway project.
- No: Create a new Railway project.
5. Deploy the project. We’ll see the logs as the service is built and
run!
6. Fetches and displays the URL of our app.
7. By default, mounts a `/app/data` folder on the cloud to our app’s
root folder. The app is run in `/app` by default, so from our app
anything we store in `/data` will persist across restarts.
A final note about Railway: We can add secrets like API keys that can be
accessed as environment variables from our apps via
[‘Variables’](https://docs.railway.app/guides/variables). For example,
for the image app (TODO link), we can add a `REPLICATE_API_KEY`
variable, and then in `main.py` we can access it as
`os.environ['REPLICATE_API_KEY']`.
### Replit
Fork [this repl](https://replit.com/@johnowhitaker/FastHTML-Example) for
a minimal example you can edit to your heart’s content. `.replit` has
been edited to add the right run command
(`run = ["uvicorn", "main:app", "--reload"]`) and to set up the ports
correctly. FastHTML was installed with `poetry add python-fasthtml`, you
can add additional packages as needed in the same way. Running the app
in Replit will show you a webview, but you may need to open in a new tab
for all features (such as cookies) to work. When you’re ready, you can
deploy your app by clicking the ‘Deploy’ button. You pay for usage - for
an app that is mostly idle the cost is usually a few cents per month.
You can store secrets like API keys via the ‘Secrets’ tab in the Replit
project settings.
### HuggingFace
Follow the instructions in [this
repository](https://github.com/AnswerDotAI/fasthtml-hf) to deploy to
HuggingFace spaces.
## Where Next?
We’ve covered a lot of ground here! Hopefully this has given you plenty
to work with in building your own FastHTML apps. If you have any
questions, feel free to ask in the \#fasthtml Discord channel (in the
fastai Discord community). You can look through the other examples in
the [fasthtml-example
repository](https://github.com/AnswerDotAI/fasthtml-example) for more
ideas, and keep an eye on Jeremy’s [YouTube
channel](https://www.youtube.com/@howardjeremyp) where we’ll be
releasing a number of “dev chats” related to FastHTML in the near
future.
| md |
FastHTML Docs | tutorials/e2e.html.md | # JS App Walkthrough
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
## Installation
You’ll need the following software to complete the tutorial, read on for
specific installation instructions:
1. Python
2. A Python package manager such as pip (which normally comes with
Python) or uv
3. FastHTML
4. Web browser
5. Railway.app account
If you haven’t worked with Python before, we recommend getting started
with [Miniconda](https://docs.anaconda.com/miniconda/).
Note that you will only need to follow the steps in the installation
section once per environment. If you create a new repo, you won’t need
to redo these.
### Install FastHTML
For Mac, Windows and Linux, enter:
``` sh
pip install python-fasthtml
```
## First steps
By the end of this section you’ll have your own FastHTML website with
tests deployed to railway.app.
### Create a hello world
Create a new folder to organize all the files for your project. Inside
this folder, create a file called `main.py` and add the following code
to it:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
app = FastHTML()
rt = app.route
@rt('/')
def get():
return 'Hello, world!'
serve()
```
</div>
Finally, run `python main.py` in your terminal and open your browser to
the ‘Link’ that appears.
### QuickDraw: A FastHTML Adventure 🎨✨
The end result of this tutorial will be QuickDraw, a real-time
collaborative drawing app using FastHTML. Here is what the final site
will look like:
<figure>
<img src="imgs/quickdraw.png" alt="QuickDraw" />
<figcaption aria-hidden="true">QuickDraw</figcaption>
</figure>
#### Drawing Rooms
Drawing rooms are the core concept of our application. Each room
represents a separate drawing space where a user can let their inner
Picasso shine. Here’s a detailed breakdown:
1. Room Creation and Storage
<div class="code-with-filename">
**main.py**
``` python
db = database('data/drawapp.db')
rooms = db.t.rooms
if rooms not in db.t:
rooms.create(id=int, name=str, created_at=str, pk='id')
Room = rooms.dataclass()
@patch
def __ft__(self:Room):
return Li(A(self.name, href=f"/rooms/{self.id}"))
```
</div>
Or you can use our
[`fast_app`](https://AnswerDotAI.github.io/fasthtml/api/fastapp.html#fast_app)
function to create a FastHTML app with a SQLite database and dataclass
in one line:
<div class="code-with-filename">
**main.py**
``` python
def render(room):
return Li(A(room.name, href=f"/rooms/{room.id}"))
app,rt,rooms,Room = fast_app('data/drawapp.db', render=render, id=int, name=str, created_at=str, pk='id')
```
</div>
We are specifying a render function to convert our dataclass into HTML,
which is the same as extending the `__ft__` method from the `patch`
decorator we used before. We will use this method for the rest of the
tutorial since it is a lot cleaner and easier to read.
- We’re using a SQLite database (via FastLite) to store our rooms.
- Each room has an id (integer), a name (string), and a created_at
timestamp (string).
- The Room dataclass is automatically generated based on this structure.
2. Creating a room
<div class="code-with-filename">
**main.py**
``` python
@rt("/")
def get():
# The 'Input' id defaults to the same as the name, so you can omit it if you wish
create_room = Form(Input(id="name", name="name", placeholder="New Room Name"),
Button("Create Room"),
hx_post="/rooms", hx_target="#rooms-list", hx_swap="afterbegin")
rooms_list = Ul(*rooms(order_by='id DESC'), id='rooms-list')
return Titled("DrawCollab",
H1("DrawCollab"),
create_room, rooms_list)
@rt("/rooms")
async def post(room:Room):
room.created_at = datetime.now().isoformat()
return rooms.insert(room)
```
</div>
- When a user submits the “Create Room” form, this route is called.
- It creates a new Room object, sets the creation time, and inserts it
into the database.
- It returns an HTML list item with a link to the new room, which is
dynamically added to the room list on the homepage thanks to HTMX.
3. Let’s give our rooms shape
<div class="code-with-filename">
**main.py**
``` python
@rt("/rooms/{id}")
async def get(id:int):
room = rooms[id]
return Titled(f"Room: {room.name}", H1(f"Welcome to {room.name}"), A(Button("Leave Room"), href="/"))
```
</div>
- This route renders the interface for a specific room.
- It fetches the room from the database and renders a title, heading,
and paragraph.
Here is the full code so far:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
from datetime import datetime
def render(room):
return Li(A(room.name, href=f"/rooms/{room.id}"))
app,rt,rooms,Room = fast_app('data/drawapp.db', render=render, id=int, name=str, created_at=str, pk='id')
@rt("/")
def get():
create_room = Form(Input(id="name", name="name", placeholder="New Room Name"),
Button("Create Room"),
hx_post="/rooms", hx_target="#rooms-list", hx_swap="afterbegin")
rooms_list = Ul(*rooms(order_by='id DESC'), id='rooms-list')
return Titled("DrawCollab", create_room, rooms_list)
@rt("/rooms")
async def post(room:Room):
room.created_at = datetime.now().isoformat()
return rooms.insert(room)
@rt("/rooms/{id}")
async def get(id:int):
room = rooms[id]
return Titled(f"Room: {room.name}", H1(f"Welcome to {room.name}"), A(Button("Leave Room"), href="/"))
serve()
```
</div>
Now run `python main.py` in your terminal and open your browser to the
‘Link’ that appears. You should see a page with a form to create a new
room and a list of existing rooms.
#### The Canvas - Let’s Get Drawing! 🖌️
Time to add the actual drawing functionality. We’ll use Fabric.js for
this:
<div class="code-with-filename">
**main.py**
``` python
# ... (keep the previous imports and database setup)
@rt("/rooms/{id}")
async def get(id:int):
room = rooms[id]
canvas = Canvas(id="canvas", width="800", height="600")
color_picker = Input(type="color", id="color-picker", value="#3CDD8C")
brush_size = Input(type="range", id="brush-size", min="1", max="50", value="10")
js = """
var canvas = new fabric.Canvas('canvas');
canvas.isDrawingMode = true;
canvas.freeDrawingBrush.color = '#3CDD8C';
canvas.freeDrawingBrush.width = 10;
document.getElementById('color-picker').onchange = function() {
canvas.freeDrawingBrush.color = this.value;
};
document.getElementById('brush-size').oninput = function() {
canvas.freeDrawingBrush.width = parseInt(this.value, 10);
};
"""
return Titled(f"Room: {room.name}",
canvas,
Div(color_picker, brush_size),
Script(src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/5.3.1/fabric.min.js"),
Script(js))
# ... (keep the serve() part)
```
</div>
Now we’ve got a drawing canvas! FastHTML makes it easy to include
external libraries and add custom JavaScript.
#### Saving and Loading Canvases 💾
Now that we have a working drawing canvas, let’s add the ability to save
and load drawings. We’ll modify our database schema to include a
`canvas_data` field, and add new routes for saving and loading canvas
data. Here’s how we’ll update our code:
1. Modify the database schema:
<div class="code-with-filename">
**main.py**
``` python
app,rt,rooms,Room = fast_app('data/drawapp.db', render=render, id=int, name=str, created_at=str, canvas_data=str, pk='id')
```
</div>
2. Add a save button that grabs the canvas’ state and sends it to the
server:
<div class="code-with-filename">
**main.py**
``` python
@rt("/rooms/{id}")
async def get(id:int):
room = rooms[id]
canvas = Canvas(id="canvas", width="800", height="600")
color_picker = Input(type="color", id="color-picker", value="#3CDD8C")
brush_size = Input(type="range", id="brush-size", min="1", max="50", value="10")
save_button = Button("Save Canvas", id="save-canvas", hx_post=f"/rooms/{id}/save", hx_vals="js:{canvas_data: JSON.stringify(canvas.toJSON())}")
# ... (rest of the function remains the same)
```
</div>
3. Add routes for saving and loading canvas data:
<div class="code-with-filename">
**main.py**
``` python
@rt("/rooms/{id}/save")
async def post(id:int, canvas_data:str):
rooms.update({'canvas_data': canvas_data}, id)
return "Canvas saved successfully"
@rt("/rooms/{id}/load")
async def get(id:int):
room = rooms[id]
return room.canvas_data if room.canvas_data else "{}"
```
</div>
4. Update the JavaScript to load existing canvas data:
<div class="code-with-filename">
**main.py**
``` javascript
js = f"""
var canvas = new fabric.Canvas('canvas');
canvas.isDrawingMode = true;
canvas.freeDrawingBrush.color = '#3CDD8C';
canvas.freeDrawingBrush.width = 10;
// Load existing canvas data
fetch(`/rooms/{id}/load`)
.then(response => response.json())
.then(data => {{
if (data && Object.keys(data).length > 0) {{
canvas.loadFromJSON(data, canvas.renderAll.bind(canvas));
}}
}});
// ... (rest of the JavaScript remains the same)
"""
```
</div>
With these changes, users can now save their drawings and load them when
they return to the room. The canvas data is stored as a JSON string in
the database, allowing for easy serialization and deserialization. Try
it out! Create a new room, make a drawing, save it, and then reload the
page. You should see your drawing reappear, ready for further editing.
Here is the completed code:
<div class="code-with-filename">
**main.py**
``` python
from fasthtml.common import *
from datetime import datetime
def render(room):
return Li(A(room.name, href=f"/rooms/{room.id}"))
app,rt,rooms,Room = fast_app('data/drawapp.db', render=render, id=int, name=str, created_at=str, canvas_data=str, pk='id')
@rt("/")
def get():
create_room = Form(Input(id="name", name="name", placeholder="New Room Name"),
Button("Create Room"),
hx_post="/rooms", hx_target="#rooms-list", hx_swap="afterbegin")
rooms_list = Ul(*rooms(order_by='id DESC'), id='rooms-list')
return Titled("QuickDraw",
create_room, rooms_list)
@rt("/rooms")
async def post(room:Room):
room.created_at = datetime.now().isoformat()
return rooms.insert(room)
@rt("/rooms/{id}")
async def get(id:int):
room = rooms[id]
canvas = Canvas(id="canvas", width="800", height="600")
color_picker = Input(type="color", id="color-picker", value="#000000")
brush_size = Input(type="range", id="brush-size", min="1", max="50", value="10")
save_button = Button("Save Canvas", id="save-canvas", hx_post=f"/rooms/{id}/save", hx_vals="js:{canvas_data: JSON.stringify(canvas.toJSON())}")
js = f"""
var canvas = new fabric.Canvas('canvas');
canvas.isDrawingMode = true;
canvas.freeDrawingBrush.color = '#000000';
canvas.freeDrawingBrush.width = 10;
// Load existing canvas data
fetch(`/rooms/{id}/load`)
.then(response => response.json())
.then(data => {{
if (data && Object.keys(data).length > 0) {{
canvas.loadFromJSON(data, canvas.renderAll.bind(canvas));
}}
}});
document.getElementById('color-picker').onchange = function() {{
canvas.freeDrawingBrush.color = this.value;
}};
document.getElementById('brush-size').oninput = function() {{
canvas.freeDrawingBrush.width = parseInt(this.value, 10);
}};
"""
return Titled(f"Room: {room.name}",
A(Button("Leave Room"), href="/"),
canvas,
Div(color_picker, brush_size, save_button),
Script(src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/5.3.1/fabric.min.js"),
Script(js))
@rt("/rooms/{id}/save")
async def post(id:int, canvas_data:str):
rooms.update({'canvas_data': canvas_data}, id)
return "Canvas saved successfully"
@rt("/rooms/{id}/load")
async def get(id:int):
room = rooms[id]
return room.canvas_data if room.canvas_data else "{}"
serve()
```
</div>
### Deploying to Railway
You can deploy your website to a number of hosting providers, for this
tutorial we’ll be using Railway. To get started, make sure you create an
[account](https://railway.app/) and install the [Railway
CLI](https://docs.railway.app/guides/cli). Once installed, make sure to
run `railway login` to log in to your account.
To make deploying your website as easy as possible, FastHTMl comes with
a built in CLI tool that will handle most of the deployment process for
you. To deploy your website, run the following command in your terminal
in the root directory of your project:
``` sh
fh_railway_deploy quickdraw
```
<div>
> **Note**
>
> Your app must be located in a `main.py` file for this to work.
</div>
### Conclusion: You’re a FastHTML Artist Now! 🎨🚀
Congratulations! You’ve just built a sleek, interactive web application
using FastHTML. Let’s recap what we’ve learned:
1. FastHTML allows you to create dynamic web apps with minimal code.
2. We used FastHTML’s routing system to handle different pages and
actions.
3. We integrated with a SQLite database to store room information and
canvas data.
4. We utilized Fabric.js to create an interactive drawing canvas.
5. We implemented features like color picking, brush size adjustment,
and canvas saving.
6. We used HTMX for seamless, partial page updates without full
reloads.
7. We learned how to deploy our FastHTML application to Railway for
easy hosting.
You’ve taken your first steps into the world of FastHTML development.
From here, the possibilities are endless! You could enhance the drawing
app further by adding features like:
- Implementing different drawing tools (e.g., shapes, text)
- Adding user authentication
- Creating a gallery of saved drawings
- Implementing real-time collaborative drawing using WebSockets
Whatever you choose to build next, FastHTML has got your back. Now go
forth and create something awesome! Happy coding! 🖼️🚀
| md |
FastHTML Docs | tutorials/tutorial_for_web_devs.html.md | # BYO Blog
<!-- WARNING: THIS FILE WAS AUTOGENERATED! DO NOT EDIT! -->
<div>
> **Caution**
>
> This document is a work in progress.
</div>
In this tutorial we’re going to write a blog by example. Blogs are a
good way to learn a web framework as they start simple yet can get
surprisingly sophistated. The [wikipedia definition of a
blog](https://en.wikipedia.org/wiki/Blog) is “an informational website
consisting of discrete, often informal diary-style text entries (posts)
informal diary-style text entries (posts)”, which means we need to
provide these basic features:
- A list of articles
- A means to create/edit/delete the articles
- An attractive but accessible layout
We’ll also add in these features, so the blog can become a working site:
- RSS feed
- Pages independent of the list of articles (about and contact come to
mind)
- Import and Export of articles
- Tagging and categorization of data
- Deployment
- Ability to scale for large volumes of readers
## How to best use this tutorial
We could copy/paste every code example in sequence and have a finished
blog at the end. However, it’s debatable how much we will learn through
the copy/paste method. We’re not saying its impossible to learn through
copy/paste, we’re just saying it’s not that of an efficient way to
learn. It’s analogous to learning how to play a musical instrument or
sport or video game by watching other people do it - you can learn some
but its not the same as doing.
A better approach is to type out every line of code in this tutorial.
This forces us to run the code through our brains, giving us actual
practice in how to write FastHTML and Pythoncode and forcing us to debug
our own mistakes. In some cases we’ll repeat similar tasks - a key
component in achieving mastery in anything. Coming back to the
instrument/sport/video game analogy, it’s exactly like actually
practicing an instrument, sport, or video game. Through practice and
repetition we eventually achieve mastery.
## Installing FastHTML
FastHTML is *just Python*. Installation is often done with pip:
``` shellscript
pip install python-fasthtml
```
## A minimal FastHTML app
First, create the directory for our project using Python’s
[pathlib](https://docs.python.org/3/library/pathlib.html) module:
``` python
import pathlib
pathlib.Path('blog-system').mkdir()
```
Now that we have our directory, let’s create a minimal FastHTML site in
it.
<div class="code-with-filename">
**blog-system/minimal.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/")
def get():
return Titled("FastHTML", P("Let's do this!"))
serve()
```
</div>
Run that with `python minimal.py` and you should get something like
this:
``` shellscript
python minimal.py
Link: http://localhost:5001
INFO: Will watch for changes in these directories: ['/Users/pydanny/projects/blog-system']
INFO: Uvicorn running on http://0.0.0.0:5001 (Press CTRL+C to quit)
INFO: Started reloader process [46572] using WatchFiles
INFO: Started server process [46576]
INFO: Waiting for application startup.
INFO: Application startup complete.
```
Confirm FastHTML is running by opening your web browser to
[127.0.0.1:5001](http://127.0.0.1:5001). You should see something like
the image below:

<div>
> **What about the `import *`?**
>
> For those worried about the use of `import *` rather than a PEP8-style
> declared namespace, understand that `__all__` is defined in FastHTML’s
> common module. That means that only the symbols (functions, classes,
> and other things) the framework wants us to have will be brought into
> our own code via `import *`. Read [importing from a
> package](https://docs.python.org/3/tutorial/modules.html#importing-from-a-package))
> for more information.
>
> Nevertheless, if we want to use a defined namespace we can do so.
> Here’s an example:
>
> ``` python
> from fasthtml import common as fh
>
>
> app, rt = fh.fast_app()
>
> @rt("/")
> def get():
> return fh.Titled("FastHTML", fh.P("Let's do this!"))
>
> fh.serve()
> ```
</div>
## Looking more closely at our app
Let’s look more closely at our application. Every line is packed with
powerful features of FastHTML:
<div class="code-with-filename">
**blog-system/minimal.py**
``` python
from fasthtml.common import *
app, rt = fast_app()
@rt("/")
def get():
return Titled("FastHTML", P("Let's do this!"))
serve()
```
</div>
Line 1
The top level namespace of Fast HTML (fasthtml.common) contains
everything we need from FastHTML to build applications. A
carefully-curated set of FastHTML functions and other Python objects is
brought into our global namespace for convenience.
Line 3
We instantiate a FastHTML app with the `fast_app()` utility function.
This provides a number of really useful defaults that we’ll modify or
take advantage of later in the tutorial.
Line 5
We use the `rt()` decorator to tell FastHTML what to return when a user
visits `/` in their browser.
Line 6
We connect this route to HTTP GET requests by defining a view function
called `get()`.
Line 7
A tree of Python function calls that return all the HTML required to
write a properly formed web page. You’ll soon see the power of this
approach.
Line 9
The `serve()` utility configures and runs FastHTML using a library
called `uvicorn`. Any changes to this module will be reloaded into the
browser.
## Adding dynamic content to our minimal app
Our page is great, but we’ll make it better. Let’s add a randomized list
of letters to the page. Every time the page reloads, a new list of
varying length will be generated.
<div class="code-with-filename">
**blog-system/random_letters.py**
``` python
from fasthtml.common import *
import string, random
app, rt = fast_app()
@rt("/")
def get():
letters = random.choices(string.ascii_uppercase, k=random.randint(5, 20))
items = [Li(c) for c in letters]
return Titled("Random lists of letters",
Ul(*items)
)
serve()
```
</div>
Line 2
The `string` and `random` libraries are part of Python’s standard
library
Line 8
We use these libraries to generate a random length list of random
letters called `letters`
Line 9
Using `letters` as the base we use list comprehension to generate a list
of `Li` ft display components, each with their own letter and save that
to the variable `items`
Line 11
Inside a call to the `Ul()` ft component we use Python’s `*args` special
syntax on the `items` variable. Therefore `*list` is treated not as one
argument but rather a set of them.
When this is run, it will generate something like this with a different
random list of letters for each page load:

## Storing the articles
The most basic component of a blog is a series of articles sorted by
date authored. Rather than a database we’re going to use our computer’s
harddrive to store a set of markdown files in a directory within our
blog called `posts`. First, let’s create the directory and some test
files we can use to search for:
``` python
from fastcore.utils import *
```
``` python
# Create some dummy posts
posts = Path("posts")
posts.mkdir(exist_ok=True)
for i in range(10): (posts/f"article_{i}.md").write_text(f"This is article {i}")
```
Searching for these files can be done with pathlib.
``` python
import pathlib
posts.ls()
```
(#10) [Path('posts/article_5.md'),Path('posts/article_1.md'),Path('posts/article_0.md'),Path('posts/article_4.md'),Path('posts/article_3.md'),Path('posts/article_7.md'),Path('posts/article_6.md'),Path('posts/article_2.md'),Path('posts/article_9.md'),Path('posts/article_8.md')]
<div>
> **Tip**
>
> Python’s [pathlib](https://docs.python.org/3/library/pathlib.html)
> library is quite useful and makes file search and manipulation much
> easier. There’s many uses for it and is compatible across operating
> systems.
</div>
## Creating the blog home page
We now have enough tools that we can create the home page. Let’s create
a new Python file and write out our simple view to list the articles in
our blog.
<div class="code-with-filename">
**blog-system/main.py**
``` python
from fasthtml.common import *
import pathlib
app, rt = fast_app()
@rt("/")
def get():
fnames = pathlib.Path("posts").rglob("*.md")
items = [Li(A(fname, href=fname)) for fname in fnames]
return Titled("My Blog",
Ul(*items)
)
serve()
```
</div>
``` python
for p in posts.ls(): p.unlink()
```
| md |
HTMX Book Summary | https://gist.githubusercontent.com/jph00/4ad7d35ad79013aded41b5ba535a12a3/raw/f677ec873cedb23ccd81fd4f24ba3b903746e26a/hypermedia-applications.summ.md | # Hypermedia Systems: book summary
This is a summary of "Hypermedia Systems" by Carson Gross, Adam Stepinski, Deniz Akşimşek. Summary created by Jeremy Howard with AI help.
- [Website for the book](https://hypermedia.systems/)
- The revolutionary ideas that empowered the Web
- A simpler approach to building applications on the Web and beyond with htmx and Hyperview
- Enhancing web applications without using SPA frameworks
## 1: Hypermedia: A Reintroduction
Hypermedia:
- Non-linear media with branching
- Hypermedia controls enable interactions, often with remote servers
- Example: Hyperlinks in HTML
Brief History:
- 1945: Vannevar Bush's Memex concept
- 1963: Ted Nelson coins "hypertext" and "hypermedia"
- 1968: Douglas Engelbart's "Mother of All Demos"
- 1990: Tim Berners-Lee creates first website
- 2000: Roy Fielding's REST dissertation
HTML as Hypermedia:
1. Anchor tags:
```html
<a href="https://hypermedia.systems/">Hypermedia Systems</a>
```
2. Form tags:
```html
<form action="/signup" method="post">
<input type="text" name="email" placeholder="Enter Email To Sign Up">
<button>Sign Up</button>
</form>
```
Non-Hypermedia Approach:
- JSON Data APIs
- Example:
```javascript
fetch('/api/v1/contacts/1')
.then(response => response.json())
.then(data => updateUI(data))
```
Single Page Applications (SPAs):
- Use JavaScript for UI updates
- Communicate with server via JSON APIs
- Example frameworks: React, Angular, Vue.js
Hypermedia Advantages:
- Simplicity
- Tolerance to content and API changes
- Leverages browser features (e.g., caching)
Hypermedia-Oriented Libraries:
- Enhance HTML as hypermedia
- Example: htmx
```html
<button hx-get="/contacts/1" hx-target="#contact-ui">
Fetch Contact
</button>
```
When to Use Hypermedia:
- Websites with moderate interactivity needs
- Server-side focused applications
- CRUD operations
When Not to Use Hypermedia:
- Highly dynamic UIs (e.g., spreadsheet applications)
- Applications requiring frequent, complex updates
HTML Notes:
- Avoid `<div>` soup
- Use semantic HTML elements
- Example of poor practice:
```html
<div class="bg-accent padding-4 rounded-2" onclick="doStuff()">
Do stuff
</div>
```
- Improved version:
```html
<button class="bg-accent padding-4 rounded-2" onclick="doStuff()">
Do stuff
</button>
```
## 2. Components Of A Hypermedia System
Components of a Hypermedia System:
1. Hypermedia:
- HTML as primary example
- Hypermedia controls: anchors and forms
- URLs: `[scheme]://[userinfo]@[host]:[port][path]?[query]#[fragment]`
Example: `https://hypermedia.systems/book/contents/`
2. Hypermedia Protocols:
- HTTP methods: GET, POST, PUT, PATCH, DELETE
- HTTP response codes with examples:
200 OK: Successful request
301 Moved Permanently: Resource has new permanent URL
404 Not Found: Resource doesn't exist
500 Internal Server Error: Server-side error
- Caching: `Cache-Control` and `Vary` headers
3. Hypermedia Servers:
- Can be built in any programming language
- Flexibility in choosing backend technology (HOWL stack)
4. Hypermedia Clients:
- Web browsers as primary example
- Importance of uniform interface
REST Constraints:
1. Client-Server Architecture
2. Statelessness:
- Every request contains all necessary information
- Session cookies as a common violation
3. Caching:
- Supported through HTTP headers
4. Uniform Interface:
a. Identification of resources (URLs)
b. Manipulation through representations
c. Self-descriptive messages
d. Hypermedia As The Engine of Application State (HATEOAS)
HATEOAS example showing adaptation to state changes:
```html
<!-- Active contact -->
<div>
<h1>Joe Smith</h1>
<div>Status: Active</div>
<a href="/contacts/42/archive">Archive</a>
</div>
<!-- Archived contact -->
<div>
<h1>Joe Smith</h1>
<div>Status: Archived</div>
<a href="/contacts/42/unarchive">Unarchive</a>
</div>
```
5. Layered System:
- Allows intermediaries like CDNs
6. Code-On-Demand (optional):
- Allows client-side scripting
Advantages of Hypermedia-Driven Applications:
- Reduced API versioning issues
- Improved system adaptability
- Self-documenting interfaces
Limitations:
- May not be ideal for applications requiring extensive client-side interactivity
HTML Notes:
- Use semantic elements carefully (e.g., `<section>`, `<article>`, `<nav>`)
- Refer to HTML specification for proper usage
- Sometimes `<div>` is appropriate
Practical implications of REST constraints:
1. Statelessness: Improves scalability but can complicate user sessions.
2. Caching: Reduces server load and improves response times.
3. Uniform Interface: Simplifies client-server interaction but may increase payload size.
Hypermedia vs JSON-based APIs:
1. Flexibility: Hypermedia adapts to changes without client updates; JSON APIs often require versioning.
2. Discoverability: Hypermedia exposes available actions; JSON requires separate documentation.
3. Payload size: Hypermedia typically larger due to including UI elements.
Examples where hypermedia might not be ideal:
1. Real-time applications (e.g., chat systems) requiring frequent, small updates.
2. Complex data visualization tools needing extensive client-side processing.
3. Offline-first mobile apps that require local data manipulation.
## 3. A Web 1.0 Application
To start our journey into Hypermedia-Driven Applications, we consider a simple contact management web application called Contact.app. Contact.app is a web 1.0-style contact management application built with Python, Flask, and Jinja2 templates. It implements CRUD operations for contacts using a RESTful architecture.
Key components:
1. Flask routes:
- GET /contacts: List all contacts or search
- GET /contacts/new: New contact form
- POST /contacts/new: Create new contact
- GET /contacts/<id>: View contact details
- GET /contacts/<id>/edit: Edit contact form
- POST /contacts/<id>/edit: Update contact
- POST /contacts/<id>/delete: Delete contact
2. Jinja2 templates:
- index.html: Contact list and search form
- new.html: New contact form
- show.html: Contact details
- edit.html: Edit contact form
3. Contact model (implementation details omitted)
The application uses the Post/Redirect/Get pattern for form submissions to prevent duplicate submissions on page refresh.
Example route handler for creating a new contact:
```python
@app.route("/contacts/new", methods=['POST'])
def contacts_new():
c = Contact(
None,
request.form['first_name'],
request.form['last_name'],
request.form['phone'],
request.form['email'])
if c.save():
flash("Created New Contact!")
return redirect("/contacts")
else:
return render_template("new.html", contact=c)
```
This handler creates a new Contact object, attempts to save it, and either redirects to the contact list with a success message or re-renders the form with error messages.
Example template snippet (edit.html):
```html
<form action="/contacts/{{ contact.id }}/edit" method="post">
<fieldset>
<legend>Contact Values</legend>
<p>
<label for="email">Email</label>
<input name="email" id="email" type="text"
placeholder="Email" value="{{ contact.email }}">
<span class="error">{{ contact.errors['email'] }}</span>
</p>
<p>
<label for="first_name">First Name</label>
<input name="first_name" id="first_name" type="text"
placeholder="First Name" value="{{ contact.first }}">
<span class="error">{{ contact.errors['first'] }}</span>
</p>
<!-- Other fields omitted -->
<button>Save</button>
</fieldset>
</form>
<form action="/contacts/{{ contact.id }}/delete" method="post">
<button>Delete Contact</button>
</form>
```
This template renders a form for editing a contact, including error message display. It also includes a separate form for deleting the contact.
The application demonstrates RESTful principles and HATEOAS through hypermedia exchanges. Each response contains the necessary links and forms for the client to navigate and interact with the application, without requiring prior knowledge of the API structure.
While functional, the application lacks modern interactivity. Every action results in a full page reload, which can feel clunky to users accustomed to more dynamic interfaces. This sets the stage for improvement using htmx in subsequent chapters, which will enhance the user experience while maintaining the hypermedia-driven architecture.
Don't overuse of generic elements like `<div>` and `<span>`, which can lead to "div soup". Instead, the use of semantic HTML is encouraged for better accessibility, readability, and maintainability. For example, using `<article>`, `<nav>`, or `<section>` tags where appropriate, rather than generic `<div>` tags.
By using HTML as the primary representation, the application inherently follows REST principles without the need for complex API versioning or extensive client-side logic to interpret application state.
## 4. Extending HTML As Hypermedia
Htmx extends HTML as a hypermedia, addressing limitations of traditional HTML:
1. Any element can make HTTP requests
2. Any event can trigger requests
3. All HTTP methods are available
4. Content can be replaced anywhere on the page
Core htmx attributes:
- `hx-get`, `hx-post`, `hx-put`, `hx-patch`, `hx-delete`: Issue HTTP requests
- `hx-target`: Specify where to place the response
- `hx-swap`: Define how to swap in new content
- `hx-trigger`: Specify events that trigger requests
Htmx expects HTML responses, not JSON. This allows for partial content updates without full page reloads.
Passing request parameters:
1. Enclosing forms: Values from ancestor form elements are included
2. `hx-include`: Specify inputs to include using CSS selectors
3. `hx-vals`: Include static or dynamic values
Example:
```html
<button hx-get="/contacts" hx-target="#main" hx-swap="outerHTML"
hx-trigger="click, keyup[ctrlKey && key == 'l'] from:body">
Get The Contacts
</button>
```
This button loads contacts when clicked or when Ctrl+L is pressed anywhere on the page.
Browser history support:
- `hx-push-url`: Create history entries for htmx requests
Example of including values:
```html
<button hx-get="/contacts" hx-vals='{"state":"MT"}'>
Get The Contacts In Montana
</button>
```
Dynamic values can be included using the `js:` prefix:
```html
<button hx-get="/contacts"
hx-vals='js:{"state":getCurrentState()}'>
Get The Contacts In The Selected State
</button>
```
Htmx enhances HTML's capabilities while maintaining simplicity and declarative nature. It allows for more interactive web applications without abandoning the hypermedia model, bridging the gap between traditional web applications and Single Page Applications.
When using htmx with history support, handle both partial and full-page responses for refreshed pages. This can be done using HTTP headers, a topic covered later.
HTML quality is important. Avoid incorrect HTML and use semantic elements when possible. However, maintaining high-quality markup in large-scale, internationalized websites can be challenging due to the separation of content authors and developers, and differing stylistic conventions between languages.
## 5. Htmx Patterns
Installing htmx: Download from unpkg.com and save to static/js/htmx.js, or use the CDN:
```html
<script src="https://unpkg.com/htmx.org@next/dist/htmx.min.js"></script>
```
AJAX-ifying the application: Use `hx-boost="true"` on the body tag to convert all links and forms to AJAX-powered controls:
```html
<body hx-boost="true">
```
Boosted links: Convert normal links to AJAX requests, replacing only the body content. Example:
```html
<a href="/contacts">Contacts</a>
```
This link now uses AJAX, avoiding full page reloads.
Boosted forms: Similar to boosted links, forms use AJAX requests instead of full page loads. No changes needed to HTML.
Attribute inheritance: Place `hx-boost` on a parent element to apply to all children:
```html
<div hx-boost="true">
<a href="/contacts">Contacts</a>
<a href="/settings">Settings</a>
</div>
```
Progressive enhancement: Boosted elements work without JavaScript, falling back to normal behavior. No additional code needed.
Deleting contacts with HTTP DELETE: Use `hx-delete` attribute on a button to issue DELETE requests:
```html
<button hx-delete="/contacts/{{ contact.id }}">Delete Contact</button>
```
Updating server-side code: Modify route to handle DELETE requests:
```python
@app.route("/contacts/<contact_id>", methods=["DELETE"])
def contacts_delete(contact_id=0):
# Delete contact logic here
```
Response code gotcha: Use 303 redirect to ensure proper GET request after deletion:
```python
return redirect("/contacts", 303)
```
Targeting the right element: Use `hx-target` to specify where to place the response:
```html
<button hx-delete="/contacts/{{ contact.id }}" hx-target="body">Delete Contact</button>
```
Updating location bar URL: Add `hx-push-url="true"` to update browser history:
```html
<button hx-delete="/contacts/{{ contact.id }}" hx-target="body" hx-push-url="true">Delete Contact</button>
```
Confirmation dialog: Use `hx-confirm` for delete operations:
```html
<button hx-delete="/contacts/{{ contact.id }}" hx-confirm="Are you sure?">Delete Contact</button>
```
Validating contact emails: Implement inline validation using htmx:
```html
<input name="email" type="email"
hx-get="/contacts/{{ contact.id }}/email"
hx-target="next .error">
<span class="error"></span>
```
Updating input type: Change input type to "email" for basic browser validation:
```html
<input name="email" type="email">
```
Inline validation: Use `hx-get` to trigger server-side validation on input change (see example above).
Validating emails server-side: Create endpoint to check email uniqueness:
```python
@app.route("/contacts/<contact_id>/email", methods=["GET"])
def contacts_email_get(contact_id=0):
# Email validation logic here
```
Improving user experience: Validate email as user types using `keyup` event:
```html
<input name="email" type="email"
hx-get="/contacts/{{ contact.id }}/email"
hx-target="next .error"
hx-trigger="change, keyup">
```
Debouncing validation requests: Add delay to avoid excessive requests during typing:
```html
<input name="email" type="email"
hx-get="/contacts/{{ contact.id }}/email"
hx-target="next .error"
hx-trigger="change, keyup delay:200ms">
```
Ignoring non-mutating keys: Use `changed` modifier to only trigger on value changes:
```html
<input name="email" type="email"
hx-get="/contacts/{{ contact.id }}/email"
hx-target="next .error"
hx-trigger="change, keyup delay:200ms changed">
```
Paging: Implement basic paging for contact list:
```html
<a href="/contacts?page={{ page + 1 }}">Next</a>
```
Click to load: Create a button to load more contacts inline:
```html
<button hx-get="/contacts?page={{ page + 1 }}"
hx-target="closest tr"
hx-swap="outerHTML"
hx-select="tbody > tr">
Load More
</button>
```
Infinite scroll: Use `hx-trigger="revealed"` to load more contacts automatically:
```html
<span hx-get="/contacts?page={{ page + 1 }}"
hx-trigger="revealed"
hx-target="closest tr"
hx-swap="outerHTML"
hx-select="tbody > tr">
Loading More...
</span>
```
HTML notes on modals: Use modals cautiously, as they can complicate hypermedia approach. Consider inline editing or separate pages instead.
Caution with "display: none": Be aware of accessibility implications when hiding elements. It removes elements from the accessibility tree and keyboard focus.
Visually hidden class: Use a utility class to hide elements visually while maintaining accessibility:
```css
.vh {
clip: rect(0 0 0 0);
clip-path: inset(50%);
block-size: 1px;
inline-size: 1px;
overflow: hidden;
white-space: nowrap;
}
```
## 6. More Htmx Patterns
Active Search:
- Implement search-as-you-type using `hx-get`, `hx-trigger`, and `hx-target`.
- Use `hx-trigger="search, keyup delay:200ms changed"` for debouncing.
- Target specific elements with `hx-target="tbody"`.
- Use HTTP headers like `HX-Trigger` for server-side logic.
- Factor templates for reusability (e.g., `rows.html`).
- Update URL with `hx-push-url="true"`.
- Add request indicators using `hx-indicator` attribute.
Example:
```html
<input id="search" type="search" name="q"
hx-get="/contacts"
hx-trigger="search, keyup delay:200ms changed"
hx-target="tbody"
hx-push-url="true"
hx-indicator="#spinner">
<img id="spinner" class="htmx-indicator" src="/static/img/spinning-circles.svg">
```
Lazy Loading:
- Defer expensive operations using `hx-get` and `hx-trigger="load"`.
- Example: Lazy loading contact count.
```html
<span hx-get="/contacts/count" hx-trigger="load">
<img class="htmx-indicator" src="/static/img/spinning-circles.svg"/>
</span>
```
- Use internal indicators for one-time requests.
- Implement truly lazy loading with `hx-trigger="revealed"`.
Inline Delete:
- Add delete functionality to contact rows.
- Use `hx-delete`, `hx-confirm`, and `hx-target` attributes.
- Differentiate between delete button and inline delete using `HX-Trigger` header.
- Implement fade-out effect using CSS transitions and `htmx-swapping` class.
- Adjust swap timing with `hx-swap="outerHTML swap:1s"`.
Example:
```html
<a href="#" hx-delete="/contacts/{{ contact.id }}"
hx-swap="outerHTML swap:1s"
hx-confirm="Are you sure you want to delete this contact?"
hx-target="closest tr">Delete</a>
```
CSS for fade-out:
```css
tr.htmx-swapping {
opacity: 0;
transition: opacity 1s ease-out;
}
```
Bulk Delete:
- Add checkboxes to rows for selection.
- Create a "Delete Selected Contacts" button with `hx-delete="/contacts"`.
- Enclose table in a form to include selected contact IDs.
- Update server-side code to handle multiple deletions.
Example:
```html
<form>
<table>
<!-- Table content -->
</table>
<button
hx-delete="/contacts"
hx-confirm="Are you sure you want to delete these contacts?"
hx-target="body">
Delete Selected Contacts
</button>
</form>
```
Server-side code:
```python
@app.route("/contacts/", methods=["DELETE"])
def contacts_delete_all():
contact_ids = [int(id) for id in request.form.getlist("selected_contact_ids")]
for contact_id in contact_ids:
contact = Contact.find(contact_id)
contact.delete()
flash("Deleted Contacts!")
contacts_set = Contact.all()
return render_template("index.html", contacts=contacts_set)
```
## 7. A Dynamic Archive UI
(This chapter is not included)
## 8. Tricks Of The Htmx Masters
Htmx Attributes:
- `hx-swap`: Controls content swapping. Options include `innerHTML`, `outerHTML`, `beforebegin`, `afterend`.
- Modifiers: `settle`, `show`, `scroll`, `focus-scroll`.
Example:
```html
<button hx-get="/contacts" hx-target="#content-div"
hx-swap="innerHTML show:body:top">
Get Contacts
</button>
```
- `hx-trigger`: Specifies events triggering requests. Modifiers: `delay`, `changed`, `once`, `throttle`, `from`, `target`, `consume`, `queue`.
- Trigger filters: Use JavaScript expressions in square brackets.
Example:
```html
<button hx-get="/contacts"
hx-trigger="click[contactRetrievalEnabled()]">
Get Contacts
</button>
```
- Synthetic events: `load`, `revealed`, `intersect`.
Other Attributes:
- `hx-push-url`: Updates navigation bar URL.
- `hx-preserve`: Keeps original content between requests.
- `hx-sync`: Synchronizes requests between elements.
- `hx-disable`: Disables htmx behavior.
Events:
- Htmx-generated events: `htmx:load`, `htmx:configRequest`, `htmx:afterRequest`, `htmx:abort`.
- Using `htmx:configRequest`:
```javascript
document.body.addEventListener("htmx:configRequest", configEvent => {
configEvent.detail.headers['X-SPECIAL-TOKEN'] = localStorage['special-token'];
})
```
- Canceling requests with `htmx:abort`:
```html
<button id="contacts-btn" hx-get="/contacts" hx-target="body">
Get Contacts
</button>
<button onclick="document.getElementById('contacts-btn')
.dispatchEvent(new Event('htmx:abort'))">
Cancel
</button>
```
- Server-generated events using `HX-Trigger` header.
HTTP Requests & Responses:
- Headers: `HX-Location`, `HX-Push-Url`, `HX-Refresh`, `HX-Retarget`.
- Special response codes: 204 (No Content), 286 (Stop Polling).
- Customizing response handling:
```javascript
document.body.addEventListener('htmx:beforeSwap', evt => {
if (evt.detail.xhr.status === 404) {
showNotFoundError();
}
});
```
Updating Other Content:
1. Expanding selection
2. Out of Band Swaps using `hx-swap-oob`
Example:
```html
<table id="contacts-table" hx-swap-oob="true">
<!-- Updated table content -->
</table>
```
3. Events (server-triggered)
Debugging:
- `htmx.logAll()`: Logs all internal htmx events.
- Chrome's `monitorEvents()`: Monitors all events on an element.
```javascript
monitorEvents(document.getElementById("some-element"));
```
Security Considerations:
- Use `hx-disable` to ignore htmx attributes in user-generated content.
- Content Security Policies (CSP): Htmx works with `eval()` disabled, except for event filters.
Configuring:
- Use `meta` tag for configuration:
```html
<meta name="htmx-config" content='{"defaultSwapStyle":"outerHTML"}'>
```
HTML Notes: Semantic HTML:
- Focus on writing conformant HTML rather than guessing at "semantic" meanings.
- Use elements as outlined in the HTML specification.
- Consider the needs of browsers, assistive technologies, and search engines.
## 9. Client Side Scripting
Scripting in Hypermedia-Driven Applications:
1. Is Scripting Allowed?
- Scripting enhances HTML websites and creates full-fledged client-side applications.
- Goal: Less code, more readable and hypermedia-friendly code.
2. Scripting for Hypermedia:
- Constraints:
a. Main data format must be hypermedia.
b. Minimize client-side state outside the DOM.
- Focus on interaction design, not business or presentation logic.
3. Scripting Tools:
- VanillaJS: Plain JavaScript without frameworks.
- Alpine.js: JavaScript library for adding behavior directly in HTML.
- _hyperscript: Non-JavaScript scripting language created alongside htmx.
4. Vanilla JavaScript:
- Simple counter example:
```html
<section class="counter">
<output id="my-output">0</output>
<button onclick="document.querySelector('#my-output').textContent++">
Increment
</button>
</section>
```
- RSJS (Reasonable System for JavaScript Structure):
```html
<section class="counter" data-counter>
<output data-counter-output>0</output>
<button data-counter-increment>Increment</button>
</section>
```
```javascript
document.querySelectorAll("[data-counter]").forEach(el => {
const output = el.querySelector("[data-counter-output]"),
increment = el.querySelector("[data-counter-increment]");
increment.addEventListener("click", e => output.textContent++);
});
```
5. Alpine.js:
- Counter example:
```html
<div x-data="{ count: 0 }">
<output x-text="count"></output>
<button x-on:click="count++">Increment</button>
</div>
```
- Bulk action toolbar:
```html
<form x-data="{ selected: [] }">
<template x-if="selected.length > 0">
<div class="tool-bar">
<span x-text="selected.length"></span> contacts selected
<button @click="selected = []">Cancel</button>
<button @click="confirm(`Delete ${selected.length} contacts?`) &&
htmx.ajax('DELETE', '/contacts',
{ source: $root, target: document.body })">
Delete
</button>
</div>
</template>
</form>
```
6. _hyperscript:
- Counter example:
```html
<div class="counter">
<output>0</output>
<button _="on click
increment the textContent of the previous <output/>">
Increment
</button>
</div>
```
- Keyboard shortcut:
```html
<input id="search" name="q" type="search" placeholder="Search Contacts"
_="on keydown[altKey and code is 'KeyS'] from the window
focus() me">
```
7. Off-the-Shelf Components:
- Integration options:
a. Callbacks:
```html
<button @click="Swal.fire({
title: 'Delete these contacts?',
showCancelButton: true,
confirmButtonText: 'Delete'
}).then((result) => {
if (result.isConfirmed) htmx.ajax('DELETE', '/contacts',
{ source: $root, target: document.body })
});">Delete</button>
```
b. Events:
```javascript
function sweetConfirm(elt, config) {
Swal.fire(config)
.then((result) => {
if (result.isConfirmed) {
elt.dispatchEvent(new Event('confirmed'));
}
});
}
```
```html
<button hx-delete="/contacts" hx-target="body" hx-trigger="confirmed"
@click="sweetConfirm($el, {
title: 'Delete these contacts?',
showCancelButton: true,
confirmButtonText: 'Delete'
})">Delete</button>
```
8. Pragmatic Scripting:
- Choose the right tool for the job.
- Avoid JSON data APIs for server communication.
- Minimize state outside the DOM.
- Favor events over hard-coded callbacks.
9. HTML Notes:
- HTML is suitable for both documents and applications.
- Hypermedia allows for interactive controls within documents.
- HTML's interactive capabilities need further development.
## 10. JSON Data APIs
Hypermedia APIs & JSON Data APIs:
1. Differences:
- Hypermedia API: Flexible, no versioning needed, specific to application needs.
- JSON Data API: Stable, versioned, rate-limited, general-purpose.
2. Adding JSON Data API to Contact.app:
- Root URL: `/api/v1/`
- Listing contacts:
```python
@app.route("/api/v1/contacts", methods=["GET"])
def json_contacts():
contacts_set = Contact.all()
contacts_dicts = [c.__dict__ for c in contacts_set]
return {"contacts": contacts_dicts}
```
3. Adding contacts:
```python
@app.route("/api/v1/contacts", methods=["POST"])
def json_contacts_new():
c = Contact(None,
request.form.get('first_name'),
request.form.get('last_name'),
request.form.get('phone'),
request.form.get('email'))
if c.save():
return c.__dict__
else:
return {"errors": c.errors}, 400
```
4. Viewing contact details:
```python
@app.route("/api/v1/contacts/<contact_id>", methods=["GET"])
def json_contacts_view(contact_id=0):
contact = Contact.find(contact_id)
return contact.__dict__
```
5. Updating contacts:
```python
@app.route("/api/v1/contacts/<contact_id>", methods=["PUT"])
def json_contacts_edit(contact_id):
c = Contact.find(contact_id)
c.update(
request.form['first_name'],
request.form['last_name'],
request.form['phone'],
request.form['email'])
if c.save():
return c.__dict__
else:
return {"errors": c.errors}, 400
```
6. Deleting contacts:
```python
@app.route("/api/v1/contacts/<contact_id>", methods=["DELETE"])
def json_contacts_delete(contact_id=0):
contact = Contact.find(contact_id)
contact.delete()
return jsonify({"success": True})
```
7. Additional considerations:
- Rate limiting
- Authentication (token-based for JSON API, session cookies for web app)
- Pagination
- Proper error handling (e.g., 404 responses)
8. API "Shape" differences:
- JSON API: Fewer endpoints, no need for UI-specific routes
- Hypermedia API: More flexible, can change without breaking clients
9. Model View Controller (MVC) Paradigm:
- Model: Domain logic
- View: HTML representation
- Controller: Thin layer connecting HTTP requests to model
10. HTML Notes - Microformats:
- Embed machine-readable data in HTML using classes
- Example:
```html
<a class="h-card" href="https://john.example">
<img src="john.jpg" alt=""> John Doe
</a>
```
- Parsed result:
```json
{
"type": ["h-card"],
"properties": {
"name": ["John Doe"],
"photo": ["john.jpg"],
"url": ["https://john.example"]
}
}
```
- Useful for cross-website systems like IndieWeb
| md |
FastHTML Source | fasthtml/authmw.py | import base64, binascii, re
from fasthtml.core import *
from fasthtml.starlette import *
from typing import Mapping
from hmac import compare_digest
auth_hdrs = {'WWW-Authenticate': 'Basic realm="login"'}
class BasicAuthMiddleware(MiddlewareBase):
def __init__(self, app, cb, skip=None): self.app,self.cb,self.skip = app,cb,skip or []
async def _resp(self, scope, receive, send, resp):
await (send({"type": "websocket.close", "code": 1000}) if scope["type"]=="websocket" else resp(scope, receive, send))
async def __call__(self, scope, receive, send) -> None:
conn = await super().__call__(scope, receive, send)
if not conn: return
request = Request(scope, receive)
if not any(re.match(o+'$', request.url.path) for o in self.skip):
res = await self.authenticate(conn)
if not res: res = Response('not authenticated', status_code=401, headers=auth_hdrs)
if isinstance(res, Response): return await self._resp(scope, receive, send, res)
scope["auth"] = res
await self.app(scope, receive, send)
async def authenticate(self, conn):
if "Authorization" not in conn.headers: return
auth = conn.headers["Authorization"]
try:
scheme, credentials = auth.split()
if scheme.lower() != 'basic': return
decoded = base64.b64decode(credentials).decode("ascii")
except (ValueError, UnicodeDecodeError, binascii.Error) as exc: raise AuthenticationError('Invalid credentials')
user, _, pwd = decoded.partition(":")
if self.cb(user,pwd): return user
def user_pwd_auth(lookup=None, skip=None, **kwargs):
if isinstance(lookup,Mapping): kwargs = lookup | kwargs
def cb(u,p):
if u=='logout' or not u or not p: return
if callable(lookup): return lookup(u,p)
return compare_digest(kwargs.get(u,'').encode("utf-8"), p.encode("utf-8"))
return Middleware(BasicAuthMiddleware, cb=cb, skip=skip)
def basic_logout(request):
return f'{request.url.scheme}://logout:logout@{request.headers["host"]}/'
| py |
FastHTML Source | fasthtml/basics.py | from .core import *
from .components import *
from .xtend import *
| py |
FastHTML Source | fasthtml/cli.py | # AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/09_cli.ipynb.
# %% auto 0
__all__ = ['railway_link', 'railway_deploy']
# %% ../nbs/api/09_cli.ipynb
from fastcore.utils import *
from fastcore.script import call_parse, bool_arg
from subprocess import check_output, run
import json
# %% ../nbs/api/09_cli.ipynb
@call_parse
def railway_link():
"Link the current directory to the current project's Railway service"
j = json.loads(check_output("railway status --json".split()))
prj = j['id']
idxpath = 'edges', 0, 'node', 'id'
env = nested_idx(j, 'environments', *idxpath)
svc = nested_idx(j, 'services', *idxpath)
cmd = f"railway link -e {env} -p {prj} -s {svc}"
res = check_output(cmd.split())
# %% ../nbs/api/09_cli.ipynb
def _run(a, **kw):
print('#', ' '.join(a))
run(a)
# %% ../nbs/api/09_cli.ipynb
@call_parse
def railway_deploy(
name:str, # The project name to deploy
mount:bool_arg=True # Create a mounted volume at /app/data?
):
"""Deploy a FastHTML app to Railway"""
nm,ver = check_output("railway --version".split()).decode().split()
assert nm=='railwayapp', f'Unexpected railway version string: {nm}'
if ver2tuple(ver)<(3,8): return print("Please update your railway CLI version to 3.8 or higher")
cp = run("railway status --json".split(), capture_output=True)
if not cp.returncode:
print("Checking deployed projects...")
project_name = json.loads(cp.stdout.decode()).get('name')
if project_name == name: return print("This project is already deployed. Run `railway open`.")
reqs = Path('requirements.txt')
if not reqs.exists(): reqs.write_text('python-fasthtml')
_run(f"railway init -n {name}".split())
_run(f"railway up -c".split())
_run(f"railway domain".split())
railway_link.__wrapped__()
if mount: _run(f"railway volume add -m /app/data".split())
_run(f"railway up -c".split())
| py |
FastHTML Source | fasthtml/common.py | import uvicorn
from dataclasses import dataclass
from .starlette import *
from fastcore.utils import *
from fastcore.xml import *
from sqlite_minutils import Database
from fastlite import *
from .basics import *
from .pico import *
from .authmw import *
from .live_reload import *
from .toaster import *
from .js import *
from .fastapp import *
| py |
FastHTML Source | fasthtml/components.py | """`ft_html` and `ft_hx` functions to add some conveniences to `ft`, along with a full set of basic HTML components, and functions to work with forms and `FT` conversion"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/01_components.ipynb.
# %% auto 0
__all__ = ['named', 'html_attrs', 'hx_attrs', 'show', 'attrmap_x', 'ft_html', 'ft_hx', 'File', 'fill_form', 'fill_dataclass',
'find_inputs', 'html2ft', 'sse_message', 'A', 'Abbr', 'Address', 'Area', 'Article', 'Aside', 'Audio', 'B',
'Base', 'Bdi', 'Bdo', 'Blockquote', 'Body', 'Br', 'Button', 'Canvas', 'Caption', 'Cite', 'Code', 'Col',
'Colgroup', 'Data', 'Datalist', 'Dd', 'Del', 'Details', 'Dfn', 'Dialog', 'Div', 'Dl', 'Dt', 'Em', 'Embed',
'Fencedframe', 'Fieldset', 'Figcaption', 'Figure', 'Footer', 'Form', 'H1', 'H2', 'H3', 'H4', 'H5', 'H6',
'Head', 'Header', 'Hgroup', 'Hr', 'Html', 'I', 'Iframe', 'Img', 'Input', 'Ins', 'Kbd', 'Label', 'Legend',
'Li', 'Link', 'Main', 'Map', 'Mark', 'Menu', 'Meta', 'Meter', 'Nav', 'Noscript', 'Object', 'Ol', 'Optgroup',
'Option', 'Output', 'P', 'Picture', 'PortalExperimental', 'Pre', 'Progress', 'Q', 'Rp', 'Rt', 'Ruby', 'S',
'Samp', 'Script', 'Search', 'Section', 'Select', 'Slot', 'Small', 'Source', 'Span', 'Strong', 'Style', 'Sub',
'Summary', 'Sup', 'Table', 'Tbody', 'Td', 'Template', 'Textarea', 'Tfoot', 'Th', 'Thead', 'Time', 'Title',
'Tr', 'Track', 'U', 'Ul', 'Var', 'Video', 'Wbr']
# %% ../nbs/api/01_components.ipynb
from dataclasses import dataclass, asdict, is_dataclass, make_dataclass, replace, astuple, MISSING
from bs4 import BeautifulSoup, Comment
from fastcore.utils import *
from fastcore.xml import *
from fastcore.meta import use_kwargs, delegates
from fastcore.test import *
from .core import fh_cfg
import types, json
try: from IPython import display
except ImportError: display=None
# %% ../nbs/api/01_components.ipynb
def show(ft,*rest):
"Renders FT Components into HTML within a Jupyter notebook."
if rest: ft = (ft,)+rest
return display.HTML(to_xml(ft))
# %% ../nbs/api/01_components.ipynb
named = set('a button form frame iframe img input map meta object param select textarea'.split())
html_attrs = 'id cls title style accesskey contenteditable dir draggable enterkeyhint hidden inert inputmode lang popover spellcheck tabindex translate'.split()
hx_attrs = 'get post put delete patch trigger target swap swap_oob include select select_oob indicator push_url confirm disable replace_url vals disabled_elt ext headers history history_elt indicator inherit params preserve prompt replace_url request sync validate'
hx_attrs = html_attrs + [f'hx_{o}' for o in hx_attrs.split()]
# %% ../nbs/api/01_components.ipynb
def attrmap_x(o):
if o.startswith('_at_'): o = '@'+o[4:]
return attrmap(o)
# %% ../nbs/api/01_components.ipynb
fh_cfg['attrmap']=attrmap_x
fh_cfg['valmap' ]=valmap
# %% ../nbs/api/01_components.ipynb
def ft_html(tag: str, *c, id=None, cls=None, title=None, style=None, attrmap=None, valmap=None, ft_cls=FT, **kwargs):
ds,c = partition(c, risinstance(dict))
for d in ds: kwargs = {**kwargs, **d}
if attrmap is None: attrmap=fh_cfg.attrmap
if valmap is None: valmap =fh_cfg.valmap
kwargs['id'],kwargs['cls'],kwargs['title'],kwargs['style'] = id,cls,title,style
tag,c,kw = ft(tag, *c, attrmap=attrmap, valmap=valmap, **kwargs).list
if tag in named and 'id' in kw and 'name' not in kw: kw['name'] = kw['id']
return ft_cls(tag,c,kw, void_=tag in voids)
# %% ../nbs/api/01_components.ipynb
@use_kwargs(hx_attrs, keep=True)
def ft_hx(tag: str, *c, target_id=None, hx_vals=None, **kwargs):
if hx_vals: kwargs['hx_vals'] = json.dumps(hx_vals) if isinstance (hx_vals,dict) else hx_vals
if target_id: kwargs['hx_target'] = '#'+target_id
return ft_html(tag, *c, **kwargs)
# %% ../nbs/api/01_components.ipynb
_g = globals()
_all_ = [
'A', 'Abbr', 'Address', 'Area', 'Article', 'Aside', 'Audio', 'B', 'Base', 'Bdi', 'Bdo', 'Blockquote', 'Body', 'Br',
'Button', 'Canvas', 'Caption', 'Cite', 'Code', 'Col', 'Colgroup', 'Data', 'Datalist', 'Dd', 'Del', 'Details', 'Dfn',
'Dialog', 'Div', 'Dl', 'Dt', 'Em', 'Embed', 'Fencedframe', 'Fieldset', 'Figcaption', 'Figure', 'Footer', 'Form',
'H1', 'H2', 'H3', 'H4', 'H5', 'H6', 'Head', 'Header',
'Hgroup', 'Hr', 'Html', 'I', 'Iframe', 'Img', 'Input', 'Ins', 'Kbd', 'Label', 'Legend', 'Li',
'Link', 'Main', 'Map', 'Mark', 'Menu', 'Meta', 'Meter', 'Nav', 'Noscript', 'Object', 'Ol', 'Optgroup', 'Option', 'Output',
'P', 'Picture', 'PortalExperimental', 'Pre', 'Progress', 'Q', 'Rp', 'Rt', 'Ruby', 'S', 'Samp', 'Script', 'Search',
'Section', 'Select', 'Slot', 'Small', 'Source', 'Span', 'Strong', 'Style', 'Sub', 'Summary', 'Sup', 'Table', 'Tbody',
'Td', 'Template', 'Textarea', 'Tfoot', 'Th', 'Thead', 'Time', 'Title', 'Tr', 'Track', 'U', 'Ul', 'Var', 'Video', 'Wbr']
for o in _all_: _g[o] = partial(ft_hx, o.lower())
# %% ../nbs/api/01_components.ipynb
def File(fname):
"Use the unescaped text in file `fname` directly"
return NotStr(Path(fname).read_text())
# %% ../nbs/api/01_components.ipynb
def _fill_item(item, obj):
if not isinstance(item,FT): return item
tag,cs,attr = item.list
if isinstance(cs,tuple): cs = tuple(_fill_item(o, obj) for o in cs)
name = attr.get('name', None)
val = None if name is None else obj.get(name, None)
if val is not None and not 'skip' in attr:
if tag=='input':
if attr.get('type', '') == 'checkbox':
if val: attr['checked'] = '1'
else: attr.pop('checked', '')
elif attr.get('type', '') == 'radio':
if val and val == attr['value']: attr['checked'] = '1'
else: attr.pop('checked', '')
else: attr['value'] = val
if tag=='textarea': cs=(val,)
if tag == 'select':
option = next((o for o in cs if o.tag=='option' and o.get('value')==val), None)
if option: option.selected = '1'
return FT(tag,cs,attr,void_=item.void_)
# %% ../nbs/api/01_components.ipynb
def fill_form(form:FT, obj)->FT:
"Fills named items in `form` using attributes in `obj`"
if is_dataclass(obj): obj = asdict(obj)
elif not isinstance(obj,dict): obj = obj.__dict__
return _fill_item(form, obj)
# %% ../nbs/api/01_components.ipynb
def fill_dataclass(src, dest):
"Modifies dataclass in-place and returns it"
for nm,val in asdict(src).items(): setattr(dest, nm, val)
return dest
# %% ../nbs/api/01_components.ipynb
def find_inputs(e, tags='input', **kw):
"Recursively find all elements in `e` with `tags` and attrs matching `kw`"
if not isinstance(e, (list,tuple,FT)): return []
inputs = []
if isinstance(tags,str): tags = [tags]
elif tags is None: tags = []
cs = e
if isinstance(e, FT):
tag,cs,attr = e.list
if tag in tags and kw.items()<=attr.items(): inputs.append(e)
for o in cs: inputs += find_inputs(o, tags, **kw)
return inputs
# %% ../nbs/api/01_components.ipynb
def __getattr__(tag):
if tag.startswith('_') or tag[0].islower(): raise AttributeError
tag = tag.replace("_", "-")
def _f(*c, target_id=None, **kwargs): return ft_hx(tag, *c, target_id=target_id, **kwargs)
return _f
# %% ../nbs/api/01_components.ipynb
_re_h2x_attr_key = re.compile(r'^[A-Za-z_-][\w-]*$')
def html2ft(html, attr1st=False):
"""Convert HTML to an `ft` expression"""
rev_map = {'class': 'cls', 'for': 'fr'}
def _parse(elm, lvl=0, indent=4):
if isinstance(elm, str): return repr(elm.strip()) if elm.strip() else ''
if isinstance(elm, list): return '\n'.join(_parse(o, lvl) for o in elm)
tag_name = elm.name.capitalize().replace("-", "_")
if tag_name=='[document]': return _parse(list(elm.children), lvl)
cts = elm.contents
cs = [repr(c.strip()) if isinstance(c, str) else _parse(c, lvl+1)
for c in cts if str(c).strip()]
attrs = []
for key, value in sorted(elm.attrs.items(), key=lambda x: x[0]=='class'):
if isinstance(value,(tuple,list)): value = " ".join(value)
key = rev_map.get(key, key)
attrs.append(f'{key.replace("-", "_")}={value!r}'
if _re_h2x_attr_key.match(key) else f'**{{{key!r}:{value!r}}}')
spc = " "*lvl*indent
onlychild = not cts or (len(cts)==1 and isinstance(cts[0],str))
j = ', ' if onlychild else f',\n{spc}'
inner = j.join(filter(None, cs+attrs))
if onlychild: return f'{tag_name}({inner})'
if not attr1st or not attrs: return f'{tag_name}(\n{spc}{inner}\n{" "*(lvl-1)*indent})'
inner_cs = j.join(filter(None, cs))
inner_attrs = ', '.join(filter(None, attrs))
return f'{tag_name}({inner_attrs})(\n{spc}{inner_cs}\n{" "*(lvl-1)*indent})'
soup = BeautifulSoup(html.strip(), 'html.parser')
for c in soup.find_all(string=risinstance(Comment)): c.extract()
return _parse(soup, 1)
# %% ../nbs/api/01_components.ipynb
def sse_message(elm, event='message'):
"Convert element `elm` into a format suitable for SSE streaming"
data = '\n'.join(f'data: {o}' for o in to_xml(elm).splitlines())
return f'event: {event}\n{data}\n\n'
| py |
FastHTML Source | fasthtml/core.py | """The `FastHTML` subclass of `Starlette`, along with the `RouterX` and `RouteX` classes it automatically uses."""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/00_core.ipynb.
# %% auto 0
__all__ = ['empty', 'htmx_hdrs', 'fh_cfg', 'htmx_resps', 'htmxsrc', 'htmxwssrc', 'fhjsscr', 'htmxctsrc', 'surrsrc', 'scopesrc',
'viewport', 'charset', 'all_meths', 'parsed_date', 'snake2hyphens', 'HtmxHeaders', 'str2int', 'str2date',
'HttpHeader', 'HtmxResponseHeaders', 'form2dict', 'parse_form', 'flat_xt', 'Beforeware', 'EventStream',
'signal_shutdown', 'WS_RouteX', 'uri', 'decode_uri', 'flat_tuple', 'Redirect', 'RouteX', 'RouterX',
'get_key', 'FastHTML', 'serve', 'Client', 'cookie', 'reg_re_param', 'MiddlewareBase', 'FtResponse']
# %% ../nbs/api/00_core.ipynb
import json,uuid,inspect,types,uvicorn,signal,asyncio,threading
from fastcore.utils import *
from fastcore.xml import *
from fastcore.meta import use_kwargs_dict
from types import UnionType, SimpleNamespace as ns, GenericAlias
from typing import Optional, get_type_hints, get_args, get_origin, Union, Mapping, TypedDict, List, Any
from datetime import datetime,date
from dataclasses import dataclass,fields
from collections import namedtuple
from inspect import isfunction,ismethod,Parameter,get_annotations
from functools import wraps, partialmethod, update_wrapper
from http import cookies
from urllib.parse import urlencode, parse_qs, quote, unquote
from copy import copy,deepcopy
from warnings import warn
from dateutil import parser as dtparse
from httpx import ASGITransport, AsyncClient
from anyio import from_thread
from .starlette import *
empty = Parameter.empty
# %% ../nbs/api/00_core.ipynb
def _sig(f): return signature_ex(f, True)
# %% ../nbs/api/00_core.ipynb
def parsed_date(s:str):
"Convert `s` to a datetime"
return dtparse.parse(s)
# %% ../nbs/api/00_core.ipynb
def snake2hyphens(s:str):
"Convert `s` from snake case to hyphenated and capitalised"
s = snake2camel(s)
return camel2words(s, '-')
# %% ../nbs/api/00_core.ipynb
htmx_hdrs = dict(
boosted="HX-Boosted",
current_url="HX-Current-URL",
history_restore_request="HX-History-Restore-Request",
prompt="HX-Prompt",
request="HX-Request",
target="HX-Target",
trigger_name="HX-Trigger-Name",
trigger="HX-Trigger")
@dataclass
class HtmxHeaders:
boosted:str|None=None; current_url:str|None=None; history_restore_request:str|None=None; prompt:str|None=None
request:str|None=None; target:str|None=None; trigger_name:str|None=None; trigger:str|None=None
def __bool__(self): return any(hasattr(self,o) for o in htmx_hdrs)
def _get_htmx(h):
res = {k:h.get(v.lower(), None) for k,v in htmx_hdrs.items()}
return HtmxHeaders(**res)
# %% ../nbs/api/00_core.ipynb
def str2int(s)->int:
"Convert `s` to an `int`"
s = s.lower()
if s=='on': return 1
if s=='none': return 0
return 0 if not s else int(s)
# %% ../nbs/api/00_core.ipynb
def _mk_list(t, v): return [t(o) for o in v]
# %% ../nbs/api/00_core.ipynb
fh_cfg = AttrDict(indent=True)
# %% ../nbs/api/00_core.ipynb
def str2date(s:str)->date:
"`date.fromisoformat` with empty string handling"
return date.fromisoformat(s) if s else None
# %% ../nbs/api/00_core.ipynb
def _fix_anno(t):
"Create appropriate callable type for casting a `str` to type `t` (or first type in `t` if union)"
origin = get_origin(t)
if origin is Union or origin is UnionType or origin in (list,List):
t = first(o for o in get_args(t) if o!=type(None))
d = {bool: str2bool, int: str2int, date: str2date}
res = d.get(t, t)
if origin in (list,List): return partial(_mk_list, res)
return lambda o: res(o[-1]) if isinstance(o,(list,tuple)) else res(o)
# %% ../nbs/api/00_core.ipynb
def _form_arg(k, v, d):
"Get type by accessing key `k` from `d`, and use to cast `v`"
if v is None: return
if not isinstance(v, (str,list,tuple)): return v
# This is the type we want to cast `v` to
anno = d.get(k, None)
if not anno: return v
return _fix_anno(anno)(v)
# %% ../nbs/api/00_core.ipynb
@dataclass
class HttpHeader: k:str;v:str
# %% ../nbs/api/00_core.ipynb
def _to_htmx_header(s):
return 'HX-' + s.replace('_', '-').title()
htmx_resps = dict(location=None, push_url=None, redirect=None, refresh=None, replace_url=None,
reswap=None, retarget=None, reselect=None, trigger=None, trigger_after_settle=None, trigger_after_swap=None)
# %% ../nbs/api/00_core.ipynb
@use_kwargs_dict(**htmx_resps)
def HtmxResponseHeaders(**kwargs):
"HTMX response headers"
res = tuple(HttpHeader(_to_htmx_header(k), v) for k,v in kwargs.items())
return res[0] if len(res)==1 else res
# %% ../nbs/api/00_core.ipynb
def _annotations(anno):
"Same as `get_annotations`, but also works on namedtuples"
if is_namedtuple(anno): return {o:str for o in anno._fields}
return get_annotations(anno)
# %% ../nbs/api/00_core.ipynb
def _is_body(anno): return issubclass(anno, (dict,ns)) or _annotations(anno)
# %% ../nbs/api/00_core.ipynb
def _formitem(form, k):
"Return single item `k` from `form` if len 1, otherwise return list"
o = form.getlist(k)
return o[0] if len(o) == 1 else o if o else None
# %% ../nbs/api/00_core.ipynb
def form2dict(form: FormData) -> dict:
"Convert starlette form data to a dict"
return {k: _formitem(form, k) for k in form}
# %% ../nbs/api/00_core.ipynb
async def parse_form(req: Request) -> FormData:
"Starlette errors on empty multipart forms, so this checks for that situation"
ctype = req.headers.get("Content-Type", "")
if not ctype.startswith("multipart/form-data"): return await req.form()
try: boundary = ctype.split("boundary=")[1].strip()
except IndexError: raise HTTPException(400, "Invalid form-data: no boundary")
min_len = len(boundary) + 6
clen = int(req.headers.get("Content-Length", "0"))
if clen <= min_len: return FormData()
return await req.form()
# %% ../nbs/api/00_core.ipynb
async def _from_body(req, p):
anno = p.annotation
# Get the fields and types of type `anno`, if available
d = _annotations(anno)
if req.headers.get('content-type', None)=='application/json': data = await req.json()
else: data = form2dict(await parse_form(req))
cargs = {k: _form_arg(k, v, d) for k, v in data.items() if not d or k in d}
return anno(**cargs)
# %% ../nbs/api/00_core.ipynb
async def _find_p(req, arg:str, p:Parameter):
"In `req` find param named `arg` of type in `p` (`arg` is ignored for body types)"
anno = p.annotation
# If there's an annotation of special types, return object of that type
# GenericAlias is a type of typing for iterators like list[int] that is not a class
if isinstance(anno, type) and not isinstance(anno, GenericAlias):
if issubclass(anno, Request): return req
if issubclass(anno, HtmxHeaders): return _get_htmx(req.headers)
if issubclass(anno, Starlette): return req.scope['app']
if _is_body(anno): return await _from_body(req, p)
# If there's no annotation, check for special names
if anno is empty:
if 'request'.startswith(arg.lower()): return req
if 'session'.startswith(arg.lower()): return req.scope.get('session', {})
if arg.lower()=='auth': return req.scope.get('auth', None)
if arg.lower()=='htmx': return _get_htmx(req.headers)
if arg.lower()=='app': return req.scope['app']
if arg.lower()=='body': return (await req.body()).decode()
if arg.lower() in ('hdrs','ftrs','bodykw','htmlkw'): return getattr(req, arg.lower())
if arg!='resp': warn(f"`{arg} has no type annotation and is not a recognised special name, so is ignored.")
return None
# Look through path, cookies, headers, query, and body in that order
res = req.path_params.get(arg, None)
if res in (empty,None): res = req.cookies.get(arg, None)
if res in (empty,None): res = req.headers.get(snake2hyphens(arg), None)
if res in (empty,None): res = req.query_params.getlist(arg)
if res==[]: res = None
if res in (empty,None): res = _formitem(await parse_form(req), arg)
# Raise 400 error if the param does not include a default
if (res in (empty,None)) and p.default is empty: raise HTTPException(400, f"Missing required field: {arg}")
# If we have a default, return that if we have no value
if res in (empty,None): res = p.default
# We can cast str and list[str] to types; otherwise just return what we have
if not isinstance(res, (list,str)) or anno is empty: return res
anno = _fix_anno(anno)
try: return anno(res)
except ValueError: raise HTTPException(404, req.url.path) from None
async def _wrap_req(req, params):
return [await _find_p(req, arg, p) for arg,p in params.items()]
# %% ../nbs/api/00_core.ipynb
def flat_xt(lst):
"Flatten lists"
result = []
if isinstance(lst,(FT,str)): lst=[lst]
for item in lst:
if isinstance(item, (list,tuple)): result.extend(item)
else: result.append(item)
return result
# %% ../nbs/api/00_core.ipynb
class Beforeware:
def __init__(self, f, skip=None): self.f,self.skip = f,skip or []
# %% ../nbs/api/00_core.ipynb
async def _handle(f, args, **kwargs):
return (await f(*args, **kwargs)) if is_async_callable(f) else await run_in_threadpool(f, *args, **kwargs)
# %% ../nbs/api/00_core.ipynb
def _find_wsp(ws, data, hdrs, arg:str, p:Parameter):
"In `data` find param named `arg` of type in `p` (`arg` is ignored for body types)"
anno = p.annotation
if isinstance(anno, type):
if issubclass(anno, HtmxHeaders): return _get_htmx(hdrs)
if issubclass(anno, Starlette): return ws.scope['app']
if anno is empty:
if arg.lower()=='ws': return ws
if arg.lower()=='data': return data
if arg.lower()=='htmx': return _get_htmx(hdrs)
if arg.lower()=='app': return ws.scope['app']
if arg.lower()=='send': return partial(_send_ws, ws)
return None
res = data.get(arg, None)
if res is empty or res is None: res = hdrs.get(arg, None)
if res is empty or res is None: res = p.default
# We can cast str and list[str] to types; otherwise just return what we have
if not isinstance(res, (list,str)) or anno is empty: return res
anno = _fix_anno(anno)
return [anno(o) for o in res] if isinstance(res,list) else anno(res)
def _wrap_ws(ws, data, params):
hdrs = {k.lower().replace('-','_'):v for k,v in data.pop('HEADERS', {}).items()}
return [_find_wsp(ws, data, hdrs, arg, p) for arg,p in params.items()]
# %% ../nbs/api/00_core.ipynb
async def _send_ws(ws, resp):
if not resp: return
res = to_xml(resp, indent=fh_cfg.indent) if isinstance(resp, (list,tuple,FT)) or hasattr(resp, '__ft__') else resp
await ws.send_text(res)
def _ws_endp(recv, conn=None, disconn=None):
cls = type('WS_Endp', (WebSocketEndpoint,), {"encoding":"text"})
async def _generic_handler(handler, ws, data=None):
wd = _wrap_ws(ws, loads(data) if data else {}, _sig(handler).parameters)
resp = await _handle(handler, wd)
if resp: await _send_ws(ws, resp)
async def _connect(self, ws):
await ws.accept()
await _generic_handler(conn, ws)
async def _disconnect(self, ws, close_code): await _generic_handler(disconn, ws)
async def _recv(self, ws, data): await _generic_handler(recv, ws, data)
if conn: cls.on_connect = _connect
if disconn: cls.on_disconnect = _disconnect
cls.on_receive = _recv
return cls
# %% ../nbs/api/00_core.ipynb
def EventStream(s):
"Create a text/event-stream response from `s`"
return StreamingResponse(s, media_type="text/event-stream")
# %% ../nbs/api/00_core.ipynb
def signal_shutdown():
event = asyncio.Event()
def signal_handler(signum, frame):
event.set()
signal.signal(signum, signal.SIG_DFL)
os.kill(os.getpid(), signum)
for sig in (signal.SIGINT, signal.SIGTERM): signal.signal(sig, signal_handler)
return event
# %% ../nbs/api/00_core.ipynb
class WS_RouteX(WebSocketRoute):
def __init__(self, app, path:str, recv, conn:callable=None, disconn:callable=None, *,
name=None, middleware=None):
super().__init__(path, _ws_endp(recv, conn, disconn), name=name, middleware=middleware)
# %% ../nbs/api/00_core.ipynb
def uri(_arg, **kwargs):
return f"{quote(_arg)}/{urlencode(kwargs, doseq=True)}"
# %% ../nbs/api/00_core.ipynb
def decode_uri(s):
arg,_,kw = s.partition('/')
return unquote(arg), {k:v[0] for k,v in parse_qs(kw).items()}
# %% ../nbs/api/00_core.ipynb
from starlette.convertors import StringConvertor
# %% ../nbs/api/00_core.ipynb
StringConvertor.regex = "[^/]*" # `+` replaced with `*`
@patch
def to_string(self:StringConvertor, value: str) -> str:
value = str(value)
assert "/" not in value, "May not contain path separators"
# assert value, "Must not be empty" # line removed due to errors
return value
# %% ../nbs/api/00_core.ipynb
@patch
def url_path_for(self:HTTPConnection, name: str, **path_params):
lp = self.scope['app'].url_path_for(name, **path_params)
return URLPath(f"{self.scope['root_path']}{lp}", lp.protocol, lp.host)
# %% ../nbs/api/00_core.ipynb
_verbs = dict(get='hx-get', post='hx-post', put='hx-post', delete='hx-delete', patch='hx-patch', link='href')
def _url_for(req, t):
if callable(t): t = t.__routename__
kw = {}
if t.find('/')>-1 and (t.find('?')<0 or t.find('/')<t.find('?')): t,kw = decode_uri(t)
t,m,q = t.partition('?')
return f"{req.url_path_for(t, **kw)}{m}{q}"
def _find_targets(req, resp):
if isinstance(resp, tuple):
for o in resp: _find_targets(req, o)
if isinstance(resp, FT):
for o in resp.children: _find_targets(req, o)
for k,v in _verbs.items():
t = resp.attrs.pop(k, None)
if t: resp.attrs[v] = _url_for(req, t)
def _apply_ft(o):
if isinstance(o, tuple): o = tuple(_apply_ft(c) for c in o)
if hasattr(o, '__ft__'): o = o.__ft__()
if isinstance(o, FT): o.children = [_apply_ft(c) for c in o.children]
return o
def _to_xml(req, resp, indent):
resp = _apply_ft(resp)
_find_targets(req, resp)
return to_xml(resp, indent)
# %% ../nbs/api/00_core.ipynb
def flat_tuple(o):
"Flatten lists"
result = []
if not isinstance(o,(tuple,list)): o=[o]
o = list(o)
for item in o:
if isinstance(item, (list,tuple)): result.extend(item)
else: result.append(item)
return tuple(result)
# %% ../nbs/api/00_core.ipynb
def _xt_cts(req, resp):
resp = flat_tuple(resp)
resp = resp + tuple(getattr(req, 'injects', ()))
http_hdrs,resp = partition(resp, risinstance(HttpHeader))
http_hdrs = {o.k:str(o.v) for o in http_hdrs}
hdr_tags = 'title','meta','link','style','base'
titles,bdy = partition(resp, lambda o: getattr(o, 'tag', '') in hdr_tags)
if resp and 'hx-request' not in req.headers and not any(getattr(o, 'tag', '')=='html' for o in resp):
if not titles: titles = [Title('FastHTML page')]
resp = Html(Head(*titles, *flat_xt(req.hdrs)), Body(bdy, *flat_xt(req.ftrs), **req.bodykw), **req.htmlkw)
return _to_xml(req, resp, indent=fh_cfg.indent), http_hdrs
# %% ../nbs/api/00_core.ipynb
def _xt_resp(req, resp):
cts,http_hdrs = _xt_cts(req, resp)
return HTMLResponse(cts, headers=http_hdrs)
# %% ../nbs/api/00_core.ipynb
def _is_ft_resp(resp): return isinstance(resp, (list,tuple,HttpHeader,FT)) or hasattr(resp, '__ft__')
# %% ../nbs/api/00_core.ipynb
def _resp(req, resp, cls=empty):
if not resp: resp=()
if hasattr(resp, '__response__'): resp = resp.__response__(req)
if cls in (Any,FT): cls=empty
if isinstance(resp, FileResponse) and not os.path.exists(resp.path): raise HTTPException(404, resp.path)
if cls is not empty: return cls(resp)
if isinstance(resp, Response): return resp
if _is_ft_resp(resp): return _xt_resp(req, resp)
if isinstance(resp, str): cls = HTMLResponse
elif isinstance(resp, Mapping): cls = JSONResponse
else:
resp = str(resp)
cls = HTMLResponse
return cls(resp)
# %% ../nbs/api/00_core.ipynb
class Redirect:
"Use HTMX or Starlette RedirectResponse as required to redirect to `loc`"
def __init__(self, loc): self.loc = loc
def __response__(self, req):
if 'hx-request' in req.headers: return HtmxResponseHeaders(redirect=self.loc)
return RedirectResponse(self.loc, status_code=303)
# %% ../nbs/api/00_core.ipynb
async def _wrap_call(f, req, params):
wreq = await _wrap_req(req, params)
return await _handle(f, wreq)
# %% ../nbs/api/00_core.ipynb
class RouteX(Route):
def __init__(self, app, path:str, endpoint, *, methods=None, name=None, include_in_schema=True, middleware=None):
self.f,self.sig,self._app = endpoint,_sig(endpoint),app
super().__init__(path, self._endp, methods=methods, name=name, include_in_schema=include_in_schema, middleware=middleware)
async def _endp(self, req):
resp = None
req.injects = []
req.hdrs,req.ftrs,req.htmlkw,req.bodykw = map(deepcopy, (self._app.hdrs,self._app.ftrs,self._app.htmlkw,self._app.bodykw))
req.hdrs,req.ftrs = listify(req.hdrs),listify(req.ftrs)
for b in self._app.before:
if not resp:
if isinstance(b, Beforeware): bf,skip = b.f,b.skip
else: bf,skip = b,[]
if not any(re.fullmatch(r, req.url.path) for r in skip):
resp = await _wrap_call(bf, req, _sig(bf).parameters)
if not resp: resp = await _wrap_call(self.f, req, self.sig.parameters)
for a in self._app.after:
_,*wreq = await _wrap_req(req, _sig(a).parameters)
nr = a(resp, *wreq)
if nr: resp = nr
return _resp(req, resp, self.sig.return_annotation)
# %% ../nbs/api/00_core.ipynb
class RouterX(Router):
def __init__(self, app, routes=None, redirect_slashes=True, default=None, *, middleware=None):
self._app = app
super().__init__(routes, redirect_slashes, default, app.on_startup, app.on_shutdown,
lifespan=app.lifespan, middleware=middleware)
def add_route(self, path: str, endpoint: callable, methods=None, name=None, include_in_schema=True):
route = RouteX(self._app, path, endpoint=endpoint, methods=methods, name=name, include_in_schema=include_in_schema)
self.routes.append(route)
def add_ws(self, path: str, recv: callable, conn:callable=None, disconn:callable=None, name=None):
route = WS_RouteX(self._app, path, recv=recv, conn=conn, disconn=disconn, name=name)
self.routes.append(route)
# %% ../nbs/api/00_core.ipynb
htmxsrc = Script(src="https://unpkg.com/htmx.org@next/dist/htmx.min.js")
htmxwssrc = Script(src="https://unpkg.com/htmx-ext-ws/ws.js")
fhjsscr = Script(src="https://cdn.jsdelivr.net/gh/answerdotai/[email protected]/fasthtml.js")
htmxctsrc = Script(src="https://unpkg.com/htmx-ext-transfer-encoding-chunked/transfer-encoding-chunked.js")
surrsrc = Script(src="https://cdn.jsdelivr.net/gh/answerdotai/surreal@main/surreal.js")
scopesrc = Script(src="https://cdn.jsdelivr.net/gh/gnat/css-scope-inline@main/script.js")
viewport = Meta(name="viewport", content="width=device-width, initial-scale=1, viewport-fit=cover")
charset = Meta(charset="utf-8")
# %% ../nbs/api/00_core.ipynb
def get_key(key=None, fname='.sesskey'):
if key: return key
fname = Path(fname)
if fname.exists(): return fname.read_text()
key = str(uuid.uuid4())
fname.write_text(key)
return key
# %% ../nbs/api/00_core.ipynb
def _list(o): return [] if not o else list(o) if isinstance(o, (tuple,list)) else [o]
# %% ../nbs/api/00_core.ipynb
def _wrap_ex(f, hdrs, ftrs, htmlkw, bodykw):
async def _f(req, exc):
req.hdrs,req.ftrs,req.htmlkw,req.bodykw = map(deepcopy, (hdrs, ftrs, htmlkw, bodykw))
res = await _handle(f, (req, exc))
return _resp(req, res)
return _f
# %% ../nbs/api/00_core.ipynb
def _mk_locfunc(f,p):
class _lf:
def __init__(self): update_wrapper(self, f)
def __call__(self, *args, **kw): return f(*args, **kw)
def rt(self, **kw): return p + (f'?{urlencode(kw)}' if kw else '')
def __str__(self): return p
return _lf()
# %% ../nbs/api/00_core.ipynb
class FastHTML(Starlette):
def __init__(self, debug=False, routes=None, middleware=None, exception_handlers=None,
on_startup=None, on_shutdown=None, lifespan=None, hdrs=None, ftrs=None,
before=None, after=None, ws_hdr=False, ct_hdr=False,
surreal=True, htmx=True, default_hdrs=True, sess_cls=SessionMiddleware,
secret_key=None, session_cookie='session_', max_age=365*24*3600, sess_path='/',
same_site='lax', sess_https_only=False, sess_domain=None, key_fname='.sesskey',
htmlkw=None, **bodykw):
middleware,before,after = map(_list, (middleware,before,after))
hdrs,ftrs = listify(hdrs),listify(ftrs)
htmlkw = htmlkw or {}
if default_hdrs:
if surreal: hdrs = [surrsrc,scopesrc] + hdrs
if ws_hdr: hdrs = [htmxwssrc] + hdrs
if ct_hdr: hdrs = [htmxctsrc] + hdrs
if htmx: hdrs = [htmxsrc,fhjsscr] + hdrs
hdrs = [charset, viewport] + hdrs
self.on_startup,self.on_shutdown,self.lifespan,self.hdrs,self.ftrs = on_startup,on_shutdown,lifespan,hdrs,ftrs
self.before,self.after,self.htmlkw,self.bodykw = before,after,htmlkw,bodykw
secret_key = get_key(secret_key, key_fname)
if sess_cls:
sess = Middleware(sess_cls, secret_key=secret_key,session_cookie=session_cookie,
max_age=max_age, path=sess_path, same_site=same_site,
https_only=sess_https_only, domain=sess_domain)
middleware.append(sess)
exception_handlers = ifnone(exception_handlers, {})
if 404 not in exception_handlers:
def _not_found(req, exc): return Response('404 Not Found', status_code=404)
exception_handlers[404] = _not_found
excs = {k:_wrap_ex(v, hdrs, ftrs, htmlkw, bodykw) for k,v in exception_handlers.items()}
super().__init__(debug, routes, middleware, excs, on_startup=on_startup, on_shutdown=on_shutdown, lifespan=lifespan)
self.router = RouterX(self, routes)
def ws(self, path:str, conn=None, disconn=None, name=None):
"Add a websocket route at `path`"
def f(func):
self.router.add_ws(path, func, conn=conn, disconn=disconn, name=name)
return func
return f
# %% ../nbs/api/00_core.ipynb
all_meths = 'get post put delete patch head trace options'.split()
@patch
def route(self:FastHTML, path:str=None, methods=None, name=None, include_in_schema=True):
"Add a route at `path`"
pathstr = None if callable(path) else path
def f(func):
n,fn,p = name,func.__name__,pathstr
assert path or (fn not in all_meths), "Must provide a path when using http verb-based function name"
if methods: m = [methods] if isinstance(methods,str) else methods
else: m = [fn] if fn in all_meths else ['get','post']
if not n: n = fn
if not p: p = '/'+('' if fn=='index' else fn)
self.router.add_route(p, func, methods=m, name=n, include_in_schema=include_in_schema)
lf = _mk_locfunc(func, p)
lf.__routename__ = n
return lf
return f(path) if callable(path) else f
# %% ../nbs/api/00_core.ipynb
for o in all_meths: setattr(FastHTML, o, partialmethod(FastHTML.route, methods=o))
# %% ../nbs/api/00_core.ipynb
def serve(
appname=None, # Name of the module
app='app', # App instance to be served
host='0.0.0.0', # If host is 0.0.0.0 will convert to localhost
port=None, # If port is None it will default to 5001 or the PORT environment variable
reload=True, # Default is to reload the app upon code changes
reload_includes:list[str]|str|None=None, # Additional files to watch for changes
reload_excludes:list[str]|str|None=None # Files to ignore for changes
):
"Run the app in an async server, with live reload set as the default."
bk = inspect.currentframe().f_back
glb = bk.f_globals
code = bk.f_code
if not appname:
if glb.get('__name__')=='__main__': appname = Path(glb.get('__file__', '')).stem
elif code.co_name=='main' and bk.f_back.f_globals.get('__name__')=='__main__': appname = inspect.getmodule(bk).__name__
if appname:
if not port: port=int(os.getenv("PORT", default=5001))
print(f'Link: http://{"localhost" if host=="0.0.0.0" else host}:{port}')
uvicorn.run(f'{appname}:{app}', host=host, port=port, reload=reload, reload_includes=reload_includes, reload_excludes=reload_excludes)
# %% ../nbs/api/00_core.ipynb
class Client:
"A simple httpx ASGI client that doesn't require `async`"
def __init__(self, app, url="http://testserver"):
self.cli = AsyncClient(transport=ASGITransport(app), base_url=url)
def _sync(self, method, url, **kwargs):
async def _request(): return await self.cli.request(method, url, **kwargs)
with from_thread.start_blocking_portal() as portal: return portal.call(_request)
for o in ('get', 'post', 'delete', 'put', 'patch', 'options'): setattr(Client, o, partialmethod(Client._sync, o))
# %% ../nbs/api/00_core.ipynb
def cookie(key: str, value="", max_age=None, expires=None, path="/", domain=None, secure=False, httponly=False, samesite="lax",):
"Create a 'set-cookie' `HttpHeader`"
cookie = cookies.SimpleCookie()
cookie[key] = value
if max_age is not None: cookie[key]["max-age"] = max_age
if expires is not None:
cookie[key]["expires"] = format_datetime(expires, usegmt=True) if isinstance(expires, datetime) else expires
if path is not None: cookie[key]["path"] = path
if domain is not None: cookie[key]["domain"] = domain
if secure: cookie[key]["secure"] = True
if httponly: cookie[key]["httponly"] = True
if samesite is not None:
assert samesite.lower() in [ "strict", "lax", "none", ], "must be 'strict', 'lax' or 'none'"
cookie[key]["samesite"] = samesite
cookie_val = cookie.output(header="").strip()
return HttpHeader("set-cookie", cookie_val)
# %% ../nbs/api/00_core.ipynb
def reg_re_param(m, s):
cls = get_class(f'{m}Conv', sup=StringConvertor, regex=s)
register_url_convertor(m, cls())
# %% ../nbs/api/00_core.ipynb
# Starlette doesn't have the '?', so it chomps the whole remaining URL
reg_re_param("path", ".*?")
reg_re_param("static", "ico|gif|jpg|jpeg|webm|css|js|woff|png|svg|mp4|webp|ttf|otf|eot|woff2|txt|html|map")
@patch
def static_route_exts(self:FastHTML, prefix='/', static_path='.', exts='static'):
"Add a static route at URL path `prefix` with files from `static_path` and `exts` defined by `reg_re_param()`"
@self.route(f"{prefix}{{fname:path}}.{{ext:{exts}}}")
async def get(fname:str, ext:str): return FileResponse(f'{static_path}/{fname}.{ext}')
# %% ../nbs/api/00_core.ipynb
@patch
def static_route(self:FastHTML, ext='', prefix='/', static_path='.'):
"Add a static route at URL path `prefix` with files from `static_path` and single `ext` (including the '.')"
@self.route(f"{prefix}{{fname:path}}{ext}")
async def get(fname:str): return FileResponse(f'{static_path}/{fname}{ext}')
# %% ../nbs/api/00_core.ipynb
class MiddlewareBase:
async def __call__(self, scope, receive, send) -> None:
if scope["type"] not in ["http", "websocket"]:
await self._app(scope, receive, send)
return
return HTTPConnection(scope)
# %% ../nbs/api/00_core.ipynb
class FtResponse:
"Wrap an FT response with any Starlette `Response`"
def __init__(self, content, status_code:int=200, headers=None, cls=HTMLResponse, media_type:str|None=None, background=None):
self.content,self.status_code,self.headers = content,status_code,headers
self.cls,self.media_type,self.background = cls,media_type,background
def __response__(self, req):
cts,httphdrs = _xt_cts(req, self.content)
headers = {**(self.headers or {}), **httphdrs}
return self.cls(cts, status_code=self.status_code, headers=headers, media_type=self.media_type, background=self.background)
| py |
FastHTML Source | fasthtml/fastapp.py | """The `fast_app` convenience wrapper"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/10_fastapp.ipynb.
# %% ../nbs/api/10_fastapp.ipynb 3
from __future__ import annotations
import inspect,uvicorn
from fastcore.utils import *
from fastlite import *
from .basics import *
from .pico import *
from .starlette import *
from .live_reload import FastHTMLWithLiveReload
# %% auto 0
__all__ = ['fast_app', 'ContainerX', 'PageX']
# %% ../nbs/api/10_fastapp.ipynb
def _get_tbl(dt, nm, schema):
render = schema.pop('render', None)
tbl = dt[nm]
if tbl not in dt: tbl.create(**schema)
else: tbl.create(**schema, transform=True)
dc = tbl.dataclass()
if render: dc.__ft__ = render
return tbl,dc
# %% ../nbs/api/10_fastapp.ipynb
def _app_factory(*args, **kwargs) -> FastHTML | FastHTMLWithLiveReload:
"Creates a FastHTML or FastHTMLWithLiveReload app instance"
if kwargs.pop('live', False): return FastHTMLWithLiveReload(*args, **kwargs)
kwargs.pop('reload_attempts', None)
kwargs.pop('reload_interval', None)
return FastHTML(*args, **kwargs)
# %% ../nbs/api/10_fastapp.ipynb
def fast_app(
db_file:Optional[str]=None, # Database file name, if needed
render:Optional[callable]=None, # Function used to render default database class
hdrs:Optional[tuple]=None, # Additional FT elements to add to <HEAD>
ftrs:Optional[tuple]=None, # Additional FT elements to add to end of <BODY>
tbls:Optional[dict]=None, # Experimental mapping from DB table names to dict table definitions
before:Optional[tuple]|Beforeware=None, # Functions to call prior to calling handler
middleware:Optional[tuple]=None, # Standard Starlette middleware
live:bool=False, # Enable live reloading
debug:bool=False, # Passed to Starlette, indicating if debug tracebacks should be returned on errors
routes:Optional[tuple]=None, # Passed to Starlette
exception_handlers:Optional[dict]=None, # Passed to Starlette
on_startup:Optional[callable]=None, # Passed to Starlette
on_shutdown:Optional[callable]=None, # Passed to Starlette
lifespan:Optional[callable]=None, # Passed to Starlette
default_hdrs=True, # Include default FastHTML headers such as HTMX script?
pico:Optional[bool]=None, # Include PicoCSS header?
surreal:Optional[bool]=True, # Include surreal.js/scope headers?
htmx:Optional[bool]=True, # Include HTMX header?
ws_hdr:bool=False, # Include HTMX websocket extension header?
secret_key:Optional[str]=None, # Signing key for sessions
key_fname:str='.sesskey', # Session cookie signing key file name
session_cookie:str='session_', # Session cookie name
max_age:int=365*24*3600, # Session cookie expiry time
sess_path:str='/', # Session cookie path
same_site:str='lax', # Session cookie same site policy
sess_https_only:bool=False, # Session cookie HTTPS only?
sess_domain:Optional[str]=None, # Session cookie domain
htmlkw:Optional[dict]=None, # Attrs to add to the HTML tag
bodykw:Optional[dict]=None, # Attrs to add to the Body tag
reload_attempts:Optional[int]=1, # Number of reload attempts when live reloading
reload_interval:Optional[int]=1000, # Time between reload attempts in ms
static_path:str=".", # Where the static file route points to, defaults to root dir
**kwargs)->Any:
"Create a FastHTML or FastHTMLWithLiveReload app."
h = (picolink,) if pico or (pico is None and default_hdrs) else ()
if hdrs: h += tuple(hdrs)
app = _app_factory(hdrs=h, ftrs=ftrs, before=before, middleware=middleware, live=live, debug=debug, routes=routes, exception_handlers=exception_handlers,
on_startup=on_startup, on_shutdown=on_shutdown, lifespan=lifespan, default_hdrs=default_hdrs, secret_key=secret_key,
session_cookie=session_cookie, max_age=max_age, sess_path=sess_path, same_site=same_site, sess_https_only=sess_https_only,
sess_domain=sess_domain, key_fname=key_fname, ws_hdr=ws_hdr, surreal=surreal, htmx=htmx, htmlkw=htmlkw,
reload_attempts=reload_attempts, reload_interval=reload_interval, **(bodykw or {}))
app.static_route_exts(static_path=static_path)
if not db_file: return app,app.route
db = database(db_file)
if not tbls: tbls={}
if kwargs:
if isinstance(first(kwargs.values()), dict): tbls = kwargs
else:
kwargs['render'] = render
tbls['items'] = kwargs
dbtbls = [_get_tbl(db.t, k, v) for k,v in tbls.items()]
if len(dbtbls)==1: dbtbls=dbtbls[0]
return app,app.route,*dbtbls
# %% ../nbs/api/10_fastapp.ipynb
def ContainerX(*cs, **kwargs): return Main(*cs, **kwargs, cls='container', hx_push_url='true', hx_swap_oob='true', id='main')
def PageX(title, *con): return Title(title), ContainerX(H1(title), *con)
| py |
FastHTML Source | fasthtml/ft.py | from fastcore.xml import *
from .components import *
from .xtend import *
| py |
FastHTML Source | fasthtml/js.py | """Basic external Javascript lib wrappers"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/03_js.ipynb.
# %% auto 0
__all__ = ['marked_imp', 'npmcdn', 'light_media', 'dark_media', 'MarkdownJS', 'KatexMarkdownJS', 'HighlightJS', 'SortableJS']
# %% ../nbs/api/03_js.ipynb
import re
from fastcore.utils import *
from fasthtml.components import *
from fasthtml.xtend import *
# %% ../nbs/api/03_js.ipynb
def light_media(
css: str # CSS to be included in the light media query
):
"Render light media for day mode views"
return Style('@media (prefers-color-scheme: light) {%s}' %css)
# %% ../nbs/api/03_js.ipynb
def dark_media(
css: str # CSS to be included in the dark media query
):
"Render dark media for nught mode views"
return Style('@media (prefers-color-scheme: dark) {%s}' %css)
# %% ../nbs/api/03_js.ipynb
marked_imp = """import { marked } from "https://cdn.jsdelivr.net/npm/marked/lib/marked.esm.js";
import { proc_htmx } from "https://cdn.jsdelivr.net/gh/answerdotai/[email protected]/fasthtml.js";
"""
npmcdn = 'https://cdn.jsdelivr.net/npm/'
# %% ../nbs/api/03_js.ipynb
def MarkdownJS(
sel='.marked' # CSS selector for markdown elements
):
"Implements browser-based markdown rendering."
src = "proc_htmx('%s', e => e.innerHTML = marked.parse(e.textContent));" % sel
return Script(marked_imp+src, type='module')
# %% ../nbs/api/03_js.ipynb
def KatexMarkdownJS(
sel='.marked', # CSS selector for markdown elements
inline_delim='$', # Delimiter for inline math
display_delim='$$', # Delimiter for long math
math_envs=None # List of environments to render as display math
):
math_envs = math_envs or ['equation', 'align', 'gather', 'multline']
env_list = '[' + ','.join(f"'{env}'" for env in math_envs) + ']'
fn = Path(__file__).parent/'katex.js'
scr = ScriptX(fn, display_delim=re.escape(display_delim), inline_delim=re.escape(inline_delim),
sel=sel, env_list=env_list, type='module')
css = Link(rel="stylesheet", href=npmcdn+"[email protected]/dist/katex.min.css")
return scr,css
# %% ../nbs/api/03_js.ipynb
def HighlightJS(
sel='pre code', # CSS selector for code elements. Default is industry standard, be careful before adjusting it
langs:str|list|tuple='python', # Language(s) to highlight
light='atom-one-light', # Light theme
dark='atom-one-dark' # Dark theme
):
"Implements browser-based syntax highlighting. Usage example [here](/tutorials/quickstart_for_web_devs.html#code-highlighting)."
src = """
hljs.addPlugin(new CopyButtonPlugin());
hljs.configure({'cssSelector': '%s'});
htmx.onLoad(hljs.highlightAll);""" % sel
hjs = 'highlightjs','cdn-release', 'build'
hjc = 'arronhunt' ,'highlightjs-copy', 'dist'
if isinstance(langs, str): langs = [langs]
langjs = [jsd(*hjs, f'languages/{lang}.min.js') for lang in langs]
return [jsd(*hjs, f'styles/{dark}.css', typ='css', media="(prefers-color-scheme: dark)"),
jsd(*hjs, f'styles/{light}.css', typ='css', media="(prefers-color-scheme: light)"),
jsd(*hjs, f'highlight.min.js'),
jsd(*hjc, 'highlightjs-copy.min.js'),
jsd(*hjc, 'highlightjs-copy.min.css', typ='css'),
light_media('.hljs-copy-button {background-color: #2d2b57;}'),
*langjs, Script(src, type='module')]
# %% ../nbs/api/03_js.ipynb
def SortableJS(
sel='.sortable', # CSS selector for sortable elements
ghost_class='blue-background-class' # When an element is being dragged, this is the class used to distinguish it from the rest
):
src = """
import {Sortable} from 'https://cdn.jsdelivr.net/npm/sortablejs/+esm';
import {proc_htmx} from "https://cdn.jsdelivr.net/gh/answerdotai/[email protected]/fasthtml.js";
proc_htmx('%s', Sortable.create);
""" % sel
return Script(src, type='module')
| py |
FastHTML Source | fasthtml/live_reload.py | from starlette.routing import WebSocketRoute
from fasthtml.basics import FastHTML, Script
__all__ = ["FastHTMLWithLiveReload"]
LIVE_RELOAD_SCRIPT = """
(function() {{
var socket = new WebSocket(`ws://${{window.location.host}}/live-reload`);
var maxReloadAttempts = {reload_attempts};
var reloadInterval = {reload_interval}; // time between reload attempts in ms
socket.onclose = function() {{
let reloadAttempts = 0;
const intervalFn = setInterval(function(){{
window.location.reload();
reloadAttempts++;
if (reloadAttempts === maxReloadAttempts) clearInterval(intervalFn);
}}, reloadInterval);
}}
}})();
"""
async def live_reload_websocket(websocket):
await websocket.accept()
class FastHTMLWithLiveReload(FastHTML):
"""
`FastHTMLWithLiveReload` enables live reloading.
This means that any code changes saved on the server will automatically
trigger a reload of both the server and browser window.
How does it work?
- a websocket is created at `/live-reload`
- a small js snippet `LIVE_RELOAD_SCRIPT` is injected into each webpage
- this snippet connects to the websocket at `/live-reload` and listens for an `onclose` event
- when the `onclose` event is detected the browser is reloaded
Why do we listen for an `onclose` event?
When code changes are saved the server automatically reloads if the --reload flag is set.
The server reload kills the websocket connection. The `onclose` event serves as a proxy
for "developer has saved some changes".
Usage
>>> from fasthtml.common import *
>>> app = FastHTMLWithLiveReload()
Run:
run_uv()
"""
LIVE_RELOAD_ROUTE = WebSocketRoute("/live-reload", endpoint=live_reload_websocket)
def __init__(self, *args, **kwargs):
# Create the live reload script to be injected into the webpage
self.LIVE_RELOAD_HEADER = Script(
LIVE_RELOAD_SCRIPT.format(
reload_attempts=kwargs.get("reload_attempts", 1),
reload_interval=kwargs.get("reload_interval", 1000),
)
)
# "hdrs" and "routes" can be missing, None, a list or a tuple.
kwargs["hdrs"] = [*(kwargs.get("hdrs") or []), self.LIVE_RELOAD_HEADER]
kwargs["routes"] = [*(kwargs.get("routes") or []), self.LIVE_RELOAD_ROUTE]
super().__init__(*args, **kwargs)
| py |
FastHTML Source | fasthtml/oauth.py | """Basic scaffolding for handling OAuth"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/08_oauth.ipynb.
# %% auto 0
__all__ = ['GoogleAppClient', 'GitHubAppClient', 'HuggingFaceClient', 'DiscordAppClient', 'decode', 'OAuth']
# %% ../nbs/api/08_oauth.ipynb
from .common import *
from oauthlib.oauth2 import WebApplicationClient
from urllib.parse import urlparse, urlencode, parse_qs, quote, unquote
from httpx import get, post
import secrets
# %% ../nbs/api/08_oauth.ipynb
class _AppClient(WebApplicationClient):
def __init__(self, client_id, client_secret, code=None, scope=None, **kwargs):
super().__init__(client_id, code=code, scope=scope, **kwargs)
self.client_secret = client_secret
# %% ../nbs/api/08_oauth.ipynb
class GoogleAppClient(_AppClient):
"A `WebApplicationClient` for Google oauth2"
base_url = "https://accounts.google.com/o/oauth2/v2/auth"
token_url = "https://www.googleapis.com/oauth2/v4/token"
info_url = "https://www.googleapis.com/oauth2/v3/userinfo"
id_key = 'sub'
def __init__(self, client_id, client_secret, code=None, scope=None, **kwargs):
scope_pre = "https://www.googleapis.com/auth/userinfo"
if not scope: scope=["openid", f"{scope_pre}.email", f"{scope_pre}.profile"]
super().__init__(client_id, client_secret, code=code, scope=scope, **kwargs)
@classmethod
def from_file(cls, fname, code=None, scope=None, **kwargs):
cred = Path(fname).read_json()['web']
return cls(cred['client_id'], client_secret=cred['client_secret'], code=code, scope=scope, **kwargs)
# %% ../nbs/api/08_oauth.ipynb
class GitHubAppClient(_AppClient):
"A `WebApplicationClient` for GitHub oauth2"
base_url = "https://github.com/login/oauth/authorize"
token_url = "https://github.com/login/oauth/access_token"
info_url = "https://api.github.com/user"
id_key = 'id'
def __init__(self, client_id, client_secret, code=None, scope=None, **kwargs):
if not scope: scope="user"
super().__init__(client_id, client_secret, code=code, scope=scope, **kwargs)
# %% ../nbs/api/08_oauth.ipynb
class HuggingFaceClient(_AppClient):
"A `WebApplicationClient` for HuggingFace oauth2"
base_url = "https://huggingface.co/oauth/authorize"
token_url = "https://huggingface.co/oauth/token"
info_url = "https://huggingface.co/oauth/userinfo"
id_key = 'sub'
def __init__(self, client_id, client_secret, code=None, scope=None, state=None, **kwargs):
if not scope: scope=["openid","profile"]
if not state: state=secrets.token_urlsafe(16)
super().__init__(client_id, client_secret, code=code, scope=scope, state=state, **kwargs)
# %% ../nbs/api/08_oauth.ipynb
class DiscordAppClient(_AppClient):
"A `WebApplicationClient` for Discord oauth2"
base_url = "https://discord.com/oauth2/authorize"
token_url = "https://discord.com/api/oauth2/token"
revoke_url = "https://discord.com/api/oauth2/token/revoke"
id_key = 'id'
def __init__(self, client_id, client_secret, is_user=False, perms=0, scope=None, **kwargs):
if not scope: scope="applications.commands applications.commands.permissions.update identify"
self.integration_type = 1 if is_user else 0
self.perms = perms
super().__init__(client_id, client_secret, scope=scope, **kwargs)
def login_link(self):
d = dict(response_type='code', client_id=self.client_id,
integration_type=self.integration_type, scope=self.scope) #, permissions=self.perms, prompt='consent')
return f'{self.base_url}?' + urlencode(d)
def parse_response(self, code):
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = dict(grant_type='authorization_code', code=code)#, redirect_uri=self.redirect_uri)
r = post(self.token_url, data=data, headers=headers, auth=(self.client_id, self.client_secret))
r.raise_for_status()
self.parse_request_body_response(r.text)
# %% ../nbs/api/08_oauth.ipynb
@patch
def login_link(self:WebApplicationClient, redirect_uri, scope=None, state=None):
"Get a login link for this client"
if not scope: scope=self.scope
if not state: state=getattr(self, 'state', None)
return self.prepare_request_uri(self.base_url, redirect_uri, scope, state=state)
# %% ../nbs/api/08_oauth.ipynb
@patch
def parse_response(self:_AppClient, code, redirect_uri):
"Get the token from the oauth2 server response"
payload = dict(code=code, redirect_uri=redirect_uri, client_id=self.client_id,
client_secret=self.client_secret, grant_type='authorization_code')
r = post(self.token_url, json=payload)
r.raise_for_status()
self.parse_request_body_response(r.text)
# %% ../nbs/api/08_oauth.ipynb
def decode(code_url):
parsed_url = urlparse(code_url)
query_params = parse_qs(parsed_url.query)
return query_params.get('code', [''])[0], query_params.get('state', [''])[0], code_url.split('?')[0]
# %% ../nbs/api/08_oauth.ipynb
@patch
def get_info(self:_AppClient, token=None):
"Get the info for authenticated user"
if not token: token = self.token["access_token"]
headers = {'Authorization': f'Bearer {token}'}
return get(self.info_url, headers=headers).json()
# %% ../nbs/api/08_oauth.ipynb
@patch
def retr_info(self:_AppClient, code, redirect_uri):
"Combines `parse_response` and `get_info`"
self.parse_response(code, redirect_uri)
return self.get_info()
# %% ../nbs/api/08_oauth.ipynb
@patch
def retr_id(self:_AppClient, code, redirect_uri):
"Call `retr_info` and then return id/subscriber value"
return self.retr_info(code, redirect_uri)[self.id_key]
# %% ../nbs/api/08_oauth.ipynb
class OAuth:
def __init__(self, app, cli, skip=None, redir_path='/redirect', logout_path='/logout', login_path='/login'):
if not skip: skip = [redir_path,login_path]
self.app,self.cli,self.skip,self.redir_path,self.logout_path,self.login_path = app,cli,skip,redir_path,logout_path,login_path
def before(req, session):
auth = req.scope['auth'] = session.get('auth')
if not auth: return RedirectResponse(self.login_path, status_code=303)
info = AttrDictDefault(cli.get_info(auth))
if not self._chk_auth(info, session): return RedirectResponse(self.login_path, status_code=303)
app.before.append(Beforeware(before, skip=skip))
@app.get(redir_path)
def redirect(code:str, req, session, state:str=None):
if not code: return "No code provided!"
base_url = f"{req.url.scheme}://{req.url.netloc}"
print(base_url)
info = AttrDictDefault(cli.retr_info(code, base_url+redir_path))
if not self._chk_auth(info, session): return RedirectResponse(self.login_path, status_code=303)
session['auth'] = cli.token['access_token']
return self.login(info, state)
@app.get(logout_path)
def logout(session):
session.pop('auth', None)
return self.logout(session)
def redir_url(self, req): return f"{req.url.scheme}://{req.url.netloc}{self.redir_path}"
def login_link(self, req, scope=None, state=None): return self.cli.login_link(self.redir_url(req), scope=scope, state=state)
def login(self, info, state): raise NotImplementedError()
def logout(self, session): return RedirectResponse(self.login_path, status_code=303)
def chk_auth(self, info, ident, session): raise NotImplementedError()
def _chk_auth(self, info, session):
ident = info.get(self.cli.id_key)
return ident and self.chk_auth(info, ident, session)
| py |
FastHTML Source | fasthtml/pico.py | """Basic components for generating Pico CSS tags"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/04_pico.ipynb.
# %% auto 0
__all__ = ['picocss', 'picolink', 'picocondcss', 'picocondlink', 'set_pico_cls', 'Card', 'Group', 'Search', 'Grid', 'DialogX',
'Container', 'PicoBusy']
# %% ../nbs/api/04_pico.ipynb
from typing import Any
from fastcore.utils import *
from fastcore.xml import *
from fastcore.meta import use_kwargs, delegates
from .components import *
from .xtend import *
try: from IPython import display
except ImportError: display=None
# %% ../nbs/api/04_pico.ipynb
picocss = "https://cdn.jsdelivr.net/npm/@picocss/pico@latest/css/pico.min.css"
picolink = (Link(rel="stylesheet", href=picocss),
Style(":root { --pico-font-size: 100%; }"))
picocondcss = "https://cdn.jsdelivr.net/npm/@picocss/pico@latest/css/pico.conditional.min.css"
picocondlink = (Link(rel="stylesheet", href=picocondcss),
Style(":root { --pico-font-size: 100%; }"))
# %% ../nbs/api/04_pico.ipynb
def set_pico_cls():
js = """var sel = '.cell-output, .output_area';
document.querySelectorAll(sel).forEach(e => e.classList.add('pico'));
new MutationObserver(ms => {
ms.forEach(m => {
m.addedNodes.forEach(n => {
if (n.nodeType === 1) {
var nc = n.classList;
if (nc && (nc.contains('cell-output') || nc.contains('output_area'))) nc.add('pico');
n.querySelectorAll(sel).forEach(e => e.classList.add('pico'));
}
});
});
}).observe(document.body, { childList: true, subtree: true });"""
return display.Javascript(js)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def Card(*c, header=None, footer=None, **kwargs)->FT:
"A PicoCSS Card, implemented as an Article with optional Header and Footer"
if header: c = (Header(header),) + c
if footer: c += (Footer(footer),)
return Article(*c, **kwargs)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def Group(*c, **kwargs)->FT:
"A PicoCSS Group, implemented as a Fieldset with role 'group'"
return Fieldset(*c, role="group", **kwargs)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def Search(*c, **kwargs)->FT:
"A PicoCSS Search, implemented as a Form with role 'search'"
return Form(*c, role="search", **kwargs)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def Grid(*c, cls='grid', **kwargs)->FT:
"A PicoCSS Grid, implemented as child Divs in a Div with class 'grid'"
c = tuple(o if isinstance(o,list) else Div(o) for o in c)
return ft_hx('div', *c, cls=cls, **kwargs)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def DialogX(*c, open=None, header=None, footer=None, id=None, **kwargs)->FT:
"A PicoCSS Dialog, with children inside a Card"
card = Card(*c, header=header, footer=footer, **kwargs)
return Dialog(card, open=open, id=id)
# %% ../nbs/api/04_pico.ipynb
@delegates(ft_hx, keep=True)
def Container(*args, **kwargs)->FT:
"A PicoCSS Container, implemented as a Main with class 'container'"
return Main(*args, cls="container", **kwargs)
# %% ../nbs/api/04_pico.ipynb
def PicoBusy():
return (HtmxOn('beforeRequest', "event.detail.elt.setAttribute('aria-busy', 'true' )"),
HtmxOn('afterRequest', "event.detail.elt.setAttribute('aria-busy', 'false')"))
| py |
FastHTML Source | fasthtml/starlette.py | from starlette.applications import Starlette
from starlette.middleware import Middleware
from starlette.middleware.sessions import SessionMiddleware
from starlette.middleware.authentication import AuthenticationMiddleware
from starlette.authentication import AuthCredentials, AuthenticationBackend, AuthenticationError, SimpleUser, requires
from starlette.middleware.httpsredirect import HTTPSRedirectMiddleware
from starlette.middleware.trustedhost import TrustedHostMiddleware
from starlette.responses import Response, HTMLResponse, FileResponse, JSONResponse, RedirectResponse, StreamingResponse
from starlette.requests import Request, HTTPConnection, FormData
from starlette.staticfiles import StaticFiles
from starlette.exceptions import HTTPException
from starlette._utils import is_async_callable
from starlette.convertors import Convertor, StringConvertor, register_url_convertor, CONVERTOR_TYPES
from starlette.routing import Route, Router, Mount, WebSocketRoute
from starlette.exceptions import HTTPException,WebSocketException
from starlette.endpoints import HTTPEndpoint,WebSocketEndpoint
from starlette.config import Config
from starlette.datastructures import CommaSeparatedStrings, Secret, UploadFile, URLPath
from starlette.types import ASGIApp, Receive, Scope, Send
from starlette.concurrency import run_in_threadpool
| py |
FastHTML Source | fasthtml/svg.py | """Simple SVG FT elements"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/05_svg.ipynb.
# %% auto 0
__all__ = ['g', 'svg_inb', 'Svg', 'ft_svg', 'Rect', 'Circle', 'Ellipse', 'transformd', 'Line', 'Polyline', 'Polygon', 'Text',
'PathFT', 'Path', 'SvgOob', 'SvgInb', 'AltGlyph', 'AltGlyphDef', 'AltGlyphItem', 'Animate', 'AnimateColor',
'AnimateMotion', 'AnimateTransform', 'ClipPath', 'Color_profile', 'Cursor', 'Defs', 'Desc', 'FeBlend',
'FeColorMatrix', 'FeComponentTransfer', 'FeComposite', 'FeConvolveMatrix', 'FeDiffuseLighting',
'FeDisplacementMap', 'FeDistantLight', 'FeFlood', 'FeFuncA', 'FeFuncB', 'FeFuncG', 'FeFuncR',
'FeGaussianBlur', 'FeImage', 'FeMerge', 'FeMergeNode', 'FeMorphology', 'FeOffset', 'FePointLight',
'FeSpecularLighting', 'FeSpotLight', 'FeTile', 'FeTurbulence', 'Filter', 'Font', 'Font_face',
'Font_face_format', 'Font_face_name', 'Font_face_src', 'Font_face_uri', 'ForeignObject', 'G', 'Glyph',
'GlyphRef', 'Hkern', 'Image', 'LinearGradient', 'Marker', 'Mask', 'Metadata', 'Missing_glyph', 'Mpath',
'Pattern', 'RadialGradient', 'Set', 'Stop', 'Switch', 'Symbol', 'TextPath', 'Tref', 'Tspan', 'Use', 'View',
'Vkern', 'Template']
# %% ../nbs/api/05_svg.ipynb
from fastcore.utils import *
from fastcore.meta import delegates
from fastcore.xml import FT
from .common import *
from .components import *
from .xtend import *
# %% ../nbs/api/05_svg.ipynb
_all_ = ['AltGlyph', 'AltGlyphDef', 'AltGlyphItem', 'Animate', 'AnimateColor', 'AnimateMotion', 'AnimateTransform', 'ClipPath', 'Color_profile', 'Cursor', 'Defs', 'Desc', 'FeBlend', 'FeColorMatrix', 'FeComponentTransfer', 'FeComposite', 'FeConvolveMatrix', 'FeDiffuseLighting', 'FeDisplacementMap', 'FeDistantLight', 'FeFlood', 'FeFuncA', 'FeFuncB', 'FeFuncG', 'FeFuncR', 'FeGaussianBlur', 'FeImage', 'FeMerge', 'FeMergeNode', 'FeMorphology', 'FeOffset', 'FePointLight', 'FeSpecularLighting', 'FeSpotLight', 'FeTile', 'FeTurbulence', 'Filter', 'Font', 'Font_face', 'Font_face_format', 'Font_face_name', 'Font_face_src', 'Font_face_uri', 'ForeignObject', 'G', 'Glyph', 'GlyphRef', 'Hkern', 'Image', 'LinearGradient', 'Marker', 'Mask', 'Metadata', 'Missing_glyph', 'Mpath', 'Pattern', 'RadialGradient', 'Set', 'Stop', 'Switch', 'Symbol', 'TextPath', 'Tref', 'Tspan', 'Use', 'View', 'Vkern', 'Template']
# %% ../nbs/api/05_svg.ipynb
g = globals()
for o in _all_: g[o] = partial(ft_hx, o[0].lower() + o[1:])
# %% ../nbs/api/05_svg.ipynb
def Svg(*args, viewBox=None, h=None, w=None, height=None, width=None, **kwargs):
"An SVG tag; xmlns is added automatically, and viewBox defaults to height and width if not provided"
if h: height=h
if w: width=w
if not viewBox and height and width: viewBox=f'0 0 {width} {height}'
return ft_svg('svg', *args, xmlns="http://www.w3.org/2000/svg", viewBox=viewBox, height=height, width=width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_hx)
def ft_svg(tag: str, *c, transform=None, opacity=None, clip=None, mask=None, filter=None,
vector_effect=None, pointer_events=None, **kwargs):
"Create a standard `FT` element with some SVG-specific attrs"
return ft_hx(tag, *c, transform=transform, opacity=opacity, clip=clip, mask=mask, filter=filter,
vector_effect=vector_effect, pointer_events=pointer_events, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Rect(width, height, x=0, y=0, fill=None, stroke=None, stroke_width=None, rx=None, ry=None, **kwargs):
"A standard SVG `rect` element"
return ft_svg('rect', width=width, height=height, x=x, y=y, fill=fill,
stroke=stroke, stroke_width=stroke_width, rx=rx, ry=ry, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Circle(r, cx=0, cy=0, fill=None, stroke=None, stroke_width=None, **kwargs):
"A standard SVG `circle` element"
return ft_svg('circle', r=r, cx=cx, cy=cy, fill=fill, stroke=stroke, stroke_width=stroke_width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Ellipse(rx, ry, cx=0, cy=0, fill=None, stroke=None, stroke_width=None, **kwargs):
"A standard SVG `ellipse` element"
return ft_svg('ellipse', rx=rx, ry=ry, cx=cx, cy=cy, fill=fill, stroke=stroke, stroke_width=stroke_width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
def transformd(translate=None, scale=None, rotate=None, skewX=None, skewY=None, matrix=None):
"Create an SVG `transform` kwarg dict"
funcs = []
if translate is not None: funcs.append(f"translate{translate}")
if scale is not None: funcs.append(f"scale{scale}")
if rotate is not None: funcs.append(f"rotate({','.join(map(str,rotate))})")
if skewX is not None: funcs.append(f"skewX({skewX})")
if skewY is not None: funcs.append(f"skewY({skewY})")
if matrix is not None: funcs.append(f"matrix{matrix}")
return dict(transform=' '.join(funcs)) if funcs else {}
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Line(x1, y1, x2=0, y2=0, stroke='black', w=None, stroke_width=1, **kwargs):
"A standard SVG `line` element"
if w: stroke_width=w
return ft_svg('line', x1=x1, y1=y1, x2=x2, y2=y2, stroke=stroke, stroke_width=stroke_width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Polyline(*args, points=None, fill=None, stroke=None, stroke_width=None, **kwargs):
"A standard SVG `polyline` element"
if points is None: points = ' '.join(f"{x},{y}" for x, y in args)
return ft_svg('polyline', points=points, fill=fill, stroke=stroke, stroke_width=stroke_width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Polygon(*args, points=None, fill=None, stroke=None, stroke_width=None, **kwargs):
"A standard SVG `polygon` element"
if points is None: points = ' '.join(f"{x},{y}" for x, y in args)
return ft_svg('polygon', points=points, fill=fill, stroke=stroke, stroke_width=stroke_width, **kwargs)
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Text(*args, x=0, y=0, font_family=None, font_size=None, fill=None, text_anchor=None,
dominant_baseline=None, font_weight=None, font_style=None, text_decoration=None, **kwargs):
"A standard SVG `text` element"
return ft_svg('text', *args, x=x, y=y, font_family=font_family, font_size=font_size, fill=fill,
text_anchor=text_anchor, dominant_baseline=dominant_baseline, font_weight=font_weight,
font_style=font_style, text_decoration=text_decoration, **kwargs)
# %% ../nbs/api/05_svg.ipynb
class PathFT(FT):
def _append_cmd(self, cmd):
if not isinstance(getattr(self, 'd'), str): self.d = cmd
else: self.d += f' {cmd}'
return self
def M(self, x, y):
"Move to."
return self._append_cmd(f'M{x} {y}')
def L(self, x, y):
"Line to."
return self._append_cmd(f'L{x} {y}')
def H(self, x):
"Horizontal line to."
return self._append_cmd(f'H{x}')
def V(self, y):
"Vertical line to."
return self._append_cmd(f'V{y}')
def Z(self):
"Close path."
return self._append_cmd('Z')
def C(self, x1, y1, x2, y2, x, y):
"Cubic Bézier curve."
return self._append_cmd(f'C{x1} {y1} {x2} {y2} {x} {y}')
def S(self, x2, y2, x, y):
"Smooth cubic Bézier curve."
return self._append_cmd(f'S{x2} {y2} {x} {y}')
def Q(self, x1, y1, x, y):
"Quadratic Bézier curve."
return self._append_cmd(f'Q{x1} {y1} {x} {y}')
def T(self, x, y):
"Smooth quadratic Bézier curve."
return self._append_cmd(f'T{x} {y}')
def A(self, rx, ry, x_axis_rotation, large_arc_flag, sweep_flag, x, y):
"Elliptical Arc."
return self._append_cmd(f'A{rx} {ry} {x_axis_rotation} {large_arc_flag} {sweep_flag} {x} {y}')
# %% ../nbs/api/05_svg.ipynb
@delegates(ft_svg)
def Path(d='', fill=None, stroke=None, stroke_width=None, **kwargs):
"Create a standard `path` SVG element. This is a special object"
return ft_svg('path', fill=fill, stroke=stroke, stroke_width=stroke_width, ft_cls=PathFT, **kwargs)
# %% ../nbs/api/05_svg.ipynb
svg_inb = dict(hx_select="svg>*")
# %% ../nbs/api/05_svg.ipynb
def SvgOob(*args, **kwargs):
"Wraps an SVG shape as required for an HTMX OOB swap"
return Template(Svg(*args, **kwargs))
# %% ../nbs/api/05_svg.ipynb
def SvgInb(*args, **kwargs):
"Wraps an SVG shape as required for an HTMX inband swap"
return Svg(*args, **kwargs), HtmxResponseHeaders(hx_reselect='svg>*')
| py |
FastHTML Source | fasthtml/toaster.py | from fastcore.xml import FT
from fasthtml.core import *
from fasthtml.components import *
from fasthtml.xtend import *
tcid = 'fh-toast-container'
sk = "toasts"
toast_css = """
.fh-toast-container {
position: fixed; top: 20px; left: 50%; transform: translateX(-50%); z-index: 1000;
display: flex; flex-direction: column; align-items: center; width: 100%;
pointer-events: none; opacity: 0; transition: opacity 0.3s ease-in-out;
}
.fh-toast {
background-color: #333; color: white;
padding: 12px 20px; border-radius: 4px; margin-bottom: 10px;
max-width: 80%; width: auto; text-align: center;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
.fh-toast-info { background-color: #2196F3; }
.fh-toast-success { background-color: #4CAF50; }
.fh-toast-warning { background-color: #FF9800; }
.fh-toast-error { background-color: #F44336; }
"""
toast_js = """
export function proc_htmx(sel, func) {
htmx.onLoad(elt => {
const elements = any(sel, elt, false);
if (elt.matches && elt.matches(sel)) elements.unshift(elt);
elements.forEach(func);
});
}
proc_htmx('.fh-toast-container', async function(toast) {
await sleep(100);
toast.style.opacity = '0.8';
await sleep(3000);
toast.style.opacity = '0';
await sleep(300);
toast.remove();
});
"""
def add_toast(sess, message, typ="info"):
assert typ in ("info", "success", "warning", "error"), '`typ` not in ("info", "success", "warning", "error")'
sess.setdefault(sk, []).append((message, typ))
def render_toasts(sess):
toasts = [Div(msg, cls=f"fh-toast fh-toast-{typ}") for msg,typ in sess.pop(sk, [])]
return Div(Div(*toasts, cls="fh-toast-container"),
hx_swap_oob="afterbegin:body")
def toast_after(resp, req, sess):
if sk in sess and (not resp or isinstance(resp, (tuple,FT))): req.injects.append(render_toasts(sess))
def setup_toasts(app):
app.hdrs += (Style(toast_css), Script(toast_js, type="module"))
app.after.append(toast_after)
| py |
FastHTML Source | fasthtml/xtend.py | """Simple extensions to standard HTML components, such as adding sensible defaults"""
# AUTOGENERATED! DO NOT EDIT! File to edit: ../nbs/api/02_xtend.ipynb.
# %% auto 0
__all__ = ['sid_scr', 'A', 'AX', 'Form', 'Hidden', 'CheckboxX', 'Script', 'Style', 'double_braces', 'undouble_braces',
'loose_format', 'ScriptX', 'replace_css_vars', 'StyleX', 'Surreal', 'On', 'Prev', 'Now', 'AnyNow', 'run_js',
'HtmxOn', 'jsd', 'Titled', 'Socials', 'Favicon', 'clear']
# %% ../nbs/api/02_xtend.ipynb
from dataclasses import dataclass, asdict
from typing import Any
from fastcore.utils import *
from fastcore.xtras import partial_format
from fastcore.xml import *
from fastcore.meta import use_kwargs, delegates
from .components import *
try: from IPython import display
except ImportError: display=None
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def A(*c, hx_get=None, target_id=None, hx_swap=None, href='#', **kwargs)->FT:
"An A tag; `href` defaults to '#' for more concise use with HTMX"
return ft_hx('a', *c, href=href, hx_get=hx_get, target_id=target_id, hx_swap=hx_swap, **kwargs)
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def AX(txt, hx_get=None, target_id=None, hx_swap=None, href='#', **kwargs)->FT:
"An A tag with just one text child, allowing hx_get, target_id, and hx_swap to be positional params"
return ft_hx('a', txt, href=href, hx_get=hx_get, target_id=target_id, hx_swap=hx_swap, **kwargs)
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def Form(*c, enctype="multipart/form-data", **kwargs)->FT:
"A Form tag; identical to plain `ft_hx` version except default `enctype='multipart/form-data'`"
return ft_hx('form', *c, enctype=enctype, **kwargs)
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def Hidden(value:Any="", id:Any=None, **kwargs)->FT:
"An Input of type 'hidden'"
return Input(type="hidden", value=value, id=id, **kwargs)
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def CheckboxX(checked:bool=False, label=None, value="1", id=None, name=None, **kwargs)->FT:
"A Checkbox optionally inside a Label, preceded by a `Hidden` with matching name"
if id and not name: name=id
if not checked: checked=None
res = Input(type="checkbox", id=id, name=name, checked=checked, value=value, **kwargs)
if label: res = Label(res, label)
return Hidden(name=name, skip=True, value=""), res
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_html, keep=True)
def Script(code:str="", **kwargs)->FT:
"A Script tag that doesn't escape its code"
return ft_html('script', NotStr(code), **kwargs)
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_html, keep=True)
def Style(*c, **kwargs)->FT:
"A Style tag that doesn't escape its code"
return ft_html('style', map(NotStr,c), **kwargs)
# %% ../nbs/api/02_xtend.ipynb
def double_braces(s):
"Convert single braces to double braces if next to special chars or newline"
s = re.sub(r'{(?=[\s:;\'"]|$)', '{{', s)
return re.sub(r'(^|[\s:;\'"])}', r'\1}}', s)
# %% ../nbs/api/02_xtend.ipynb
def undouble_braces(s):
"Convert double braces to single braces if next to special chars or newline"
s = re.sub(r'\{\{(?=[\s:;\'"]|$)', '{', s)
return re.sub(r'(^|[\s:;\'"])\}\}', r'\1}', s)
# %% ../nbs/api/02_xtend.ipynb
def loose_format(s, **kw):
"String format `s` using `kw`, without being strict about braces outside of template params"
if not kw: return s
return undouble_braces(partial_format(double_braces(s), **kw)[0])
# %% ../nbs/api/02_xtend.ipynb
def ScriptX(fname, src=None, nomodule=None, type=None, _async=None, defer=None,
charset=None, crossorigin=None, integrity=None, **kw):
"A `script` element with contents read from `fname`"
s = loose_format(Path(fname).read_text(), **kw)
return Script(s, src=src, nomodule=nomodule, type=type, _async=_async, defer=defer,
charset=charset, crossorigin=crossorigin, integrity=integrity)
# %% ../nbs/api/02_xtend.ipynb
def replace_css_vars(css, pre='tpl', **kwargs):
"Replace `var(--)` CSS variables with `kwargs` if name prefix matches `pre`"
if not kwargs: return css
def replace_var(m):
var_name = m.group(1).replace('-', '_')
return kwargs.get(var_name, m.group(0))
return re.sub(fr'var\(--{pre}-([\w-]+)\)', replace_var, css)
# %% ../nbs/api/02_xtend.ipynb
def StyleX(fname, **kw):
"A `style` element with contents read from `fname` and variables replaced from `kw`"
s = Path(fname).read_text()
attrs = ['type', 'media', 'scoped', 'title', 'nonce', 'integrity', 'crossorigin']
sty_kw = {k:kw.pop(k) for k in attrs if k in kw}
return Style(replace_css_vars(s, **kw), **sty_kw)
# %% ../nbs/api/02_xtend.ipynb
def Surreal(code:str):
"Wrap `code` in `domReadyExecute` and set `m=me()` and `p=me('-')`"
return Script('''
{
const m=me();
const _p = document.currentScript.previousElementSibling;
const p = _p ? me(_p) : null;
domReadyExecute(() => {
%s
});
}''' % code)
# %% ../nbs/api/02_xtend.ipynb
def On(code:str, event:str='click', sel:str='', me=True):
"An async surreal.js script block event handler for `event` on selector `sel,p`, making available parent `p`, event `ev`, and target `e`"
func = 'me' if me else 'any'
if sel=='-': sel='p'
elif sel: sel=f'{func}("{sel}", m)'
else: sel='m'
return Surreal('''
%s.on("%s", async ev=>{
let e = me(ev);
%s
});''' % (sel,event,code))
# %% ../nbs/api/02_xtend.ipynb
def Prev(code:str, event:str='click'):
"An async surreal.js script block event handler for `event` on previous sibling, with same vars as `On`"
return On(code, event=event, sel='-')
# %% ../nbs/api/02_xtend.ipynb
def Now(code:str, sel:str=''):
"An async surreal.js script block on selector `me(sel)`"
if sel: sel=f'"{sel}"'
return Script('(async (ee = me(%s)) => {\nlet e = me(ee);\n%s\n})()\n' % (sel,code))
# %% ../nbs/api/02_xtend.ipynb
def AnyNow(sel:str, code:str):
"An async surreal.js script block on selector `any(sel)`"
return Script('(async (e = any("%s")) => {\n%s\n})()\n' % (sel,code))
# %% ../nbs/api/02_xtend.ipynb
def run_js(js, id=None, **kw):
"Run `js` script, auto-generating `id` based on name of caller if needed, and js-escaping any `kw` params"
if not id: id = sys._getframe(1).f_code.co_name
kw = {k:dumps(v) for k,v in kw.items()}
return Script(js.format(**kw), id=id, hx_swap_oob='true')
# %% ../nbs/api/02_xtend.ipynb
def HtmxOn(eventname:str, code:str):
return Script('''domReadyExecute(function() {
document.body.addEventListener("htmx:%s", function(event) { %s })
})''' % (eventname, code))
# %% ../nbs/api/02_xtend.ipynb
def jsd(org, repo, root, path, prov='gh', typ='script', ver=None, esm=False, **kwargs)->FT:
"jsdelivr `Script` or CSS `Link` tag, or URL"
ver = '@'+ver if ver else ''
s = f'https://cdn.jsdelivr.net/{prov}/{org}/{repo}{ver}/{root}/{path}'
if esm: s += '/+esm'
return Script(src=s, **kwargs) if typ=='script' else Link(rel='stylesheet', href=s, **kwargs) if typ=='css' else s
# %% ../nbs/api/02_xtend.ipynb
@delegates(ft_hx, keep=True)
def Titled(title:str="FastHTML app", *args, cls="container", **kwargs)->FT:
"An HTML partial containing a `Title`, and `H1`, and any provided children"
return Title(title), Main(H1(title), *args, cls=cls, **kwargs)
# %% ../nbs/api/02_xtend.ipynb
def Socials(title, site_name, description, image, url=None, w=1200, h=630, twitter_site=None, creator=None, card='summary'):
"OG and Twitter social card headers"
if not url: url=site_name
if not url.startswith('http'): url = f'https://{url}'
if not image.startswith('http'): image = f'{url}{image}'
res = [Meta(property='og:image', content=image),
Meta(property='og:site_name', content=site_name),
Meta(property='og:image:type', content='image/png'),
Meta(property='og:image:width', content=w),
Meta(property='og:image:height', content=h),
Meta(property='og:type', content='website'),
Meta(property='og:url', content=url),
Meta(property='og:title', content=title),
Meta(property='og:description', content=description),
Meta(name='twitter:image', content=image),
Meta(name='twitter:card', content=card),
Meta(name='twitter:title', content=title),
Meta(name='twitter:description', content=description)]
if twitter_site is not None: res.append(Meta(name='twitter:site', content=twitter_site))
if creator is not None: res.append(Meta(name='twitter:creator', content=creator))
return tuple(res)
# %% ../nbs/api/02_xtend.ipynb
def Favicon(light_icon, dark_icon):
"Light and dark favicon headers"
return (Link(rel='icon', type='image/x-ico', href=light_icon, media='(prefers-color-scheme: light)'),
Link(rel='icon', type='image/x-ico', href=dark_icon, media='(prefers-color-scheme: dark)'))
# %% ../nbs/api/02_xtend.ipynb
def clear(id): return Div(hx_swap_oob='innerHTML', id=id)
# %% ../nbs/api/02_xtend.ipynb
sid_scr = Script('''
function uuid() {
return [...crypto.getRandomValues(new Uint8Array(10))].map(b=>b.toString(36)).join('');
}
sessionStorage.setItem("sid", sessionStorage.getItem("sid") || uuid());
htmx.on("htmx:configRequest", (e) => {
const sid = sessionStorage.getItem("sid");
if (sid) {
const url = new URL(e.detail.path, window.location.origin);
url.searchParams.set('sid', sid);
e.detail.path = url.pathname + url.search;
}
});
''')
| py |
FastHTML Gallery | applications/applications/annotate_text/app.py | from fasthtml.common import *
import json
import httpx
# Set up the app, including daisyui and tailwind for the chat component
tlink = Script(src="https://cdn.tailwindcss.com?plugins=typography"),
dlink = Link(rel="stylesheet", href="https://cdn.jsdelivr.net/npm/[email protected]/dist/full.min.css")
def Arrow(arrow, hx_get, id):
# Grey out button if you're at the end
if arrow == "←": ptr_evnts = "pointer-events-none opacity-50" if id == 1 else ""
elif arrow == "→": ptr_evnts = " pointer-events-none opacity-50" if id == total_items_length - 1 else ""
# CSS Styling for both arrow buttons
common_classes = "relative inline-flex items-center bg-indigo-600 px-3 py-2 text-sm font-semibold text-white shadow-sm hover:bg-indigo-500 focus-visible:outline focus-visible:outline-2 focus-visible:outline-offset-2 focus-visible:outline-indigo-600"
return A(Span(arrow, cls="sr-only"),
Span(arrow, cls="h-5 w-5", aria_hidden="true"),
hx_get=hx_get, hx_swap="outerHTML",
cls=f" {'' if arrow=='←' else '-ml-px'} rounded-{'l' if arrow=='←' else 'r'}-md {common_classes} {ptr_evnts}")
def AnnotateButton(value, feedback):
# Different button styling if it's already marked as correct/incorrect
classes = '' if feedback=='correct' else 'btn-outline'
# Green for correct red for incorrect
classes += f" btn-{'success' if value=='correct' else 'error'}"
classes += ' mr-2' if value=='correct' else ''
return Button(value.capitalize(), name='feedback', value=value, cls='btn hover:text-white '+classes)
def render(Item):
messages = json.loads(Item.messages)
card_header = Div(cls="border-b border-gray-200 bg-white p-4")(
Div(cls="flex justify-between items-center mb-4")(
H3(f"Sample {Item.id} out of {total_items_length}" if total_items_length else "No samples in DB", cls="text-base font-semibold leading-6 text-gray-9000"),
Div(cls="flex-shrink-0")(
Arrow("←", f"{Item.id - 2}" if Item.id > 0 else "#", Item.id),
Arrow("→", f"{Item.id}" if Item.id < total_items_length - 1 else "#", Item.id))),
Div(cls="-ml-4 -mt-4 flex flex-wrap items-center justify-between sm:flex-nowrap")(
Div(cls="ml-4 mt-4")(
P(messages[0]['content'], cls="mt-1 text-sm text-gray-500 max-h-16 overflow-y-auto whitespace-pre-wrap"))))
card_buttons_form = Div(cls="mt-4")(
Form(cls="flex items-center", method="post", hx_post=f"{Item.id}", target_id=f"item_{Item.id}", hx_swap="outerHTML", hx_encoding="multipart/form-data")(
Input(type="text", name="notes", value=Item.notes, placeholder="Additional notes?", cls="flex-grow p-2 my-4 border border-gray-300 rounded-md text-sm focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:border-indigo-500 bg-transparent"),
Div(cls="flex-shrink-0 ml-4")(
AnnotateButton('correct', Item.feedback),
AnnotateButton('incorrect', Item.feedback))))
# Card component
card = Div(cls=" flex flex-col h-full flex-grow overflow-auto", id=f"item_{Item.id}",
style="min-height: calc(100vh - 6rem); max-height: calc(100vh - 16rem);")(
card_header,
Div(cls="bg-white shadow rounded-b-lg p-4 pt-0 pb-10 flex-grow overflow-scroll")(
Div(messages[1]['content'], id="main_text", cls="mt-2 w-full rounded-t-lg text-sm whitespace-pre-wrap h-auto marked")),
card_buttons_form)
return card
hdrs=(tlink, dlink, picolink, MarkdownJS(), HighlightJS())
app, rt, texts_db, Item = fast_app('texts.db',hdrs=hdrs, render=render, bodykw={"data-theme":"light"},
id=int, messages=list, feedback=bool, notes=str, pk='id')
# Get Dummy Data
data_url = 'https://raw.githubusercontent.com/AnswerDotAI/fasthtml-example/main/annotate_text/data/dummy_data.jsonl'
response = httpx.get(data_url)
# Insert Dummy Data into Db
for line in response.text.splitlines():
item = json.loads(line)
texts_db.insert(messages=json.dumps(item), feedback=None, notes='')
# Set total_items_length after inserting dummy data
total_items_length = len(texts_db())
print(f"Inserted {total_items_length} items from dummy data")
@rt("/{idx}")
def post(idx: int, feedback: str = None, notes: str = None):
print(f"Posting feedback: {feedback} and notes: {notes} for item {idx}")
items = texts_db()
item = texts_db.get(idx)
item.feedback, item.notes = feedback, notes
texts_db.update(item)
next_item = next((i for i in items if i.id > item.id), items[0])
print(f"Updated item {item.id} with feedback: {feedback} and notes: {notes} moving to {next_item.id}")
return next_item
@rt("/")
@rt("/{idx}")
def get(idx:int = 0):
items = texts_db()
index = idx
if index >= len(items): index = len(items) - 1 if items else 0
# Container for card and buttons
content = Div(cls="w-full max-w-2xl mx-auto flex flex-col max-h-full")(
H1('LLM generated text annotation tool with FastHTML (and Tailwind)',cls="text-xl font-bold text-center text-gray-800 mb-8"),
items[index])
# Gallery Navigation
navcls = 'inline-block px-4 py-2 text-sm font-medium text-white rounded transition-colors duration-300 bg-{color}-600 hover:bg-{color}-700'
def navitem(txt, color, href): return A(txt, cls=navcls.format(color=color), href=href),
navbar = Nav(cls="bg-gray-800 shadow-md")(
Div(cls="container mx-auto px-4 py-3 flex justify-between items-center")(
H1("FastHTML Gallery", cls="text-white text-xl font-semibold"),
Div(cls="space-x-2")(
navitem("Back to Gallery", color="white", href="/"),
navitem("Info", color='blue',href="/applications/annotate_text/info"),
navitem("Code", color="gray", href="/applications/annotate_text/code"))))
return Main(navbar,content,
cls="container mx-auto min-h-screen bg-gray-100 p-8 flex flex-col",
hx_target="this")
| py |
FastHTML Gallery | applications/applications/cellular_automata/app.py | from fasthtml.common import *
from starlette.responses import Response
from uuid import uuid4
generator = {}
bindict = {
(1,1,1):0,
(1,1,0):1,
(1,0,1):2,
(1,0,0):3,
(0,1,1):4,
(0,1,0):5,
(0,0,1):6,
(0,0,0):7}
initial_row = [0]*50 + [1] + [0]*50
color_map = {0:"white", 1:"black"}
####################
### HTML Widgets ###
####################
explanation = Div(
H1("Cellular Automata"),
H4("Input explanations:"),
Ul(Li(Strong("Rule: "),"Determines the next state of a cell based on the current state of the cell and its neighbors."),
Li(Strong("Generations: "),"Determines how many generations to run the automaton."),
Li(Strong("Width: "),"Determines the width of the grid."),))
def progress_bar(percent_complete: float):
return Div(hx_swap_oob="innerHTML:#progress_bar")(
Progress(value=percent_complete))
def mk_box(color,size=5):
return Div(cls="box", style=f"background-color:{color_map[color]};height:{size}px;width:{size}px;margin:0;display:inline-block;")
def mk_row(colors,font_size=0,size=5):
return Div(*[mk_box(color,size) for color in colors], cls="row",style=f"font-size:{font_size}px;")
def mk_button(show):
return Button("Hide Rule" if show else "Show Rule",
hx_get="/applications/cellular_automata/app/show_rule?show=" + ("False" if show else "True"),
hx_target="#rule", id="sh_rule", hx_swap_oob="outerHTML",
hx_include="[name='rule_number']")
########################
### FastHTML Section ###
########################
app, rt = fast_app()
nav = Nav()(
Div(cls="container")(
Div(cls="grid")(
H1("FastHTML Gallery"),
Div(cls="grid")(
A("Back to Gallery", cls="outline", href="/", role="button" ),
A("Info", cls="secondary", href="/applications/cellular_automata/info", role="button"),
A("Code", href="/applications/cellular_automata/code", role="button")))))
@app.get('/')
def homepage(sess):
if 'id' not in sess: sess['id'] = str(uuid4())
return Title("Cellular Automata"),Main(nav,Div(
Div(P(explanation,id="explanations")),
Form(Group(
Div(hx_target='this', hx_swap='outerHTML')(Label(_for="rule_number", cls="form-label")("Rule"),
Input(type='number', name="rule_number", id='rule_set', value="30",hx_post='/applications/cellular_automata/app/validate/rule_number')),
Div(hx_target='this', hx_swap='outerHTML')(Label("Generations", cls="form-label"),
Input(type='number',name="generations", id='generations_set', value="50",hx_post='/applications/cellular_automata/app/validate/generations', hx_indicator='#generationsind')),
Div(hx_target='this', hx_swap='outerHTML')(Label("Width", cls="form-label"),
Input(type='number',name="width", id='width_set', value="100", hx_post='/applications/cellular_automata/app/validate/width', hx_indicator='#widthind')),
Button(cls="btn btn-active btn-primary", type="submit", hx_get="/applications/cellular_automata/app/run",
hx_target="#grid", hx_include="[name='rule_number'],[name='generations'],[name='width']", hx_swap="outerHTML")("Run"))),
Group(
Div(style="margin-left:50px")(
Div(id="progress_bar"),
Div(id="grid")),
Div(style="margin-right:50px; max-width:200px")(
mk_button(False),
Div(id="rule"),
))))
@rt('/show_rule')
def get(rule_number: int, show: bool):
rule = [int(x) for x in f'{rule_number:08b}']
return Div(
Div(mk_button(show)),
Div(*[Group(
Div(mk_row(list(k),font_size=10,size=20),style="max-width:100px"),
Div(P(" -> "),style="max-width:100px"),
Div(mk_box(rule[v],size=20),style="max-width:100px")) for k,v in bindict.items()] if show else '')
)
@rt('/run')
def get(rule_number: int, generations: int, width: int, sess):
errors = {'rule_number': validate_rule_number(rule_number),
'generations': validate_generations(generations),
'width': validate_width(width)}
# Removes the None values from the errors dictionary (No errors)
errors = {k: v for k, v in errors.items() if v is not None}
# Return Button with error message if they exist
if errors:
return Div(Div(id="grid"),
Div(id="progress_bar",hx_swap_oob="outerHTML:#progress_bar"),
Div(id='submit-btn-container',hx_swap_oob="outerHTML:#submit-btn-container")(
Button(cls="btn btn-active btn-primary", type="submit",
hx_get="/applications/cellular_automata/app/run", hx_target="#grid",
hx_include="[name='rule_number'],[name='generations'],[name='width']", hx_swap="outerHTML")("Run"),
*[Div(error, style='color: red;') for error in errors.values()]))
start = [0]*(width//2) + [1] + [0]*(width//2)
global generator
generator[sess['id']] = run(rule=rule_number,generations=generations,start=start)
return Div(
Div(style=f"width: {(width+1)*5}px",id="progress_bar",hx_swap_oob="outerHTML:#progress_bar"),
Div(id="next",hx_trigger="every .1s", hx_get="/applications/cellular_automata/app/next", hx_target="#grid",hx_swap="beforeend"),id="grid")
@rt('/next')
def get(sess):
global generator
g,val = next(generator[sess['id']],(False,False))
if val: return Div(
progress_bar(g),
mk_row(val))
else:
del generator[sess['id']]
return Response(status_code=286)
@rt('/validate/rule_number')
def post(rule_number: int): return inputTemplate('Rule Number', 'rule_number',rule_number, validate_rule_number(rule_number))
@rt('/validate/generations')
def post(generations: int): return inputTemplate('Generations', 'generations', generations, validate_generations(generations))
@rt('/validate/width')
def post(width: int): return inputTemplate('Width', 'width', width, validate_width(width))
#########################
### Application Logic ###
#########################
def run(rule=30, start = initial_row, generations = 100):
rule = [int(x) for x in f'{rule:08b}']
yield 0, start
old_row = [0] + start + [0]
new_row = []
for g in range(1,generations):
for i in range(1,len(old_row)-1):
key=tuple(old_row[i-1:i+2])
new_row.append(rule[bindict[key]])
yield (g+1)/generations,new_row
old_row = [0] + new_row + [0]
new_row = []
########################
### Validation Logic ###
########################
def validate_rule_number(rule_number: int):
if (rule_number < 0) or (rule_number > 255 ): return "Enter an integer between 0 and 255 (inclusive)"
def validate_generations(generations: int):
if generations < 0: return "Enter a positive integer"
if generations > 200: return "Enter a number less than 200"
def validate_width(width: int):
if width < 0: return "Enter a positive integer"
if width > 200: return "Enter a number less than 200"
def inputTemplate(label, name, val, errorMsg=None, input_type='number'):
# Generic template for replacing the input field and showing the validation message
return Div(hx_target='this', hx_swap='outerHTML', cls=f"{errorMsg if errorMsg else 'Valid'}")(
Label(label), # Creates label for the input field
Input(name=name,type=input_type,value=f'{val}',style="width: 340px;",hx_post=f'/applications/cellular_automata/app/validate/{name.lower()}'), # Creates input field
Div(f'{errorMsg}', style='color: red;') if errorMsg else None) # Creates red error message below if there is an error
| py |
FastHTML Gallery | applications/applications/tic_tac_toe/app.py | from fasthtml.common import *
style = Style("""body{
min-height: 100vh;
margin:0;
background-color: #1A1A1E;
display:grid;
}""") # custom style to be applied globally.
hdrs = (Script(src="https://cdn.tailwindcss.com") ,
Link(rel="stylesheet", href="/files/applications/applications/tic_tac_toe/output.css"))
app, rt = fast_app(hdrs=(hdrs, style), pico=False)
current_state_index = -1 #Used to navigate the current snapshot of the board
button_states = [[None for _ in range(9)] for _ in range(9)] #2D array to store snapshots of board
win_states = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
] #possible win streaks/states for Xs and Os
winner_found_game_ended = False
def check_win(player) -> bool:
global button_states, current_state_index, winner_found_game_ended
"""Checks if there's a win streak present in the board. Uses the win states list to check
If text at all text indices are equal and its not the placeholder text ("."), change the global variable "winner_found_game_ended" to True"""
for cell_1, cell_2, cell_3 in win_states:
if (
button_states[current_state_index][cell_1] != None
and button_states[current_state_index][cell_1]
== button_states[current_state_index][cell_2]
and button_states[current_state_index][cell_2]
== button_states[current_state_index][cell_3]):
winner_found_game_ended = True
return f"Player {player} wins the game!"
if all(value is not None for value in button_states[current_state_index]):
#if the current snapshot of the board doesn't have any placeholder text and there is no winning streak
winner_found_game_ended = True
return "No Winner :("
#will keep returning this value [because its called after every button click], until a winner or none is found
return f'''Player {'X' if player == 'O' else 'O'}'s turn!'''
def handle_click(index: int):
"""This function handles what text gets sent to the button's face depending on whose turn it is uses a weird algorithm"""
global button_states, current_state_index
next_index = current_state_index + 1
button_states[next_index] = button_states[current_state_index][:] #make a copy of the current snapshot to add to the next snapshot
if button_states[current_state_index][index] is None:
if "X" not in button_states[current_state_index] or button_states[
current_state_index
].count("X") <= button_states[current_state_index].count("O"):
button_states[next_index][index] = "X"
else:
button_states[next_index][index] = "O"
current_state_index += 1
return button_states[next_index][index]
@app.get("/on_click") # On click, call helper function to alternate between X and O
def render_button(index:int):
global button_states, current_state_index
player = handle_click(index)
winner = check_win(player) # function that checks if there's a winner
buttons = [Button(
f'''{text if text is not None else '.' }''',
cls="tic-button-disabled" if (text is not None) or winner_found_game_ended else "tic-button",
disabled=True if (text is not None) or winner_found_game_ended else False,
hx_get=f"/applications/tic_tac_toe/app/on_click?index={idx}",
hx_target=".buttons-div", hx_swap='outerHTML')
for idx, text in enumerate(button_states[current_state_index])
]
"""rerenders buttons based on the next snapshot.
I initially made this to render only the button that gets clicked.
But to be able to check the winner and stop the game, I have to use the next snapshot instead
if you wanna see the previous implementation, it should be in one of the commits."""
board = Div(
Div(winner, cls="justify-self-center"),
Div(*buttons, cls="grid grid-cols-3 grid-rows-3"),
cls="buttons-div font-bevan text-white font-light grid justify-center")
return board
# Rerenders the board if the restart button is clicked.
# Also responsible for initial rendering of board when webpage is reloaded
@app.get("/restart")
def render_board():
global button_states, current_state_index, winner_found_game_ended
current_state_index = -1
button_states = [[None for _ in range(9)] for _ in range(9)]
winner_found_game_ended = False
# button component
buttons = [
Button(
".",
cls="tic-button",
hx_get=f"/applications/tic_tac_toe/app/on_click?index={i}",
hx_swap="outerHTML", hx_target=".buttons-div")
for i, _ in enumerate(button_states[current_state_index])
]
return Div(Div("Player X starts the game",cls="font-bevan text-white justify-self-center"),
Div(*buttons, cls="grid grid-cols-3 grid-rows-3"),
cls="buttons-div grid")
@app.get("/")
def homepage():
global button_states
def navitem(txt, cls, href): return A(txt, cls="inline-block px-4 py-2 text-sm font-medium text-white rounded transition-colors duration-300"+cls, href=href),
return Div(
Nav(cls="bg-gray-800 shadow-md")(
Div(cls="container mx-auto px-4 py-3 flex justify-between items-center")(
H1("FastHTML Gallery", cls="text-white text-xl font-semibold"),
Div(cls="space-x-2")(
navitem("Back to Gallery", cls="bg-transparent border border-white hover:bg-white hover:text-gray-800", href="/"),
navitem("Info", cls="bg-blue-600 hover:bg-blue-700", href="/applications/tic_tac_toe/info"),
navitem("Code", cls="bg-gray-600 hover:bg-gray-700", href="/applications/tic_tac_toe/code"),
),
),
),
Div(
H1("Tic Tac Toe!", cls="font-bevan text-5xl text-white"),
P("A FastHTML app by Adedara Adeloro", cls="font-bevan text-custom-blue font-light"),
cls="m-14"),
Div(
render_board.__wrapped__(), # render buttons.
Div(
Button(
"Restart!",
disabled=False,
cls="restart-button",
hx_get="/applications/tic_tac_toe/app/restart", hx_target=".buttons-div", hx_swap="outerHTML"),
cls="flex flex-col items-center justify-center m-10"),
cls="flex flex-col items-center justify-center"),
cls="justify-center items-center min-h-screen bg-custom-background")
| py |
FastHTML Gallery | applications/start_simple/csv_editor/app.py | from fasthtml.common import *
from uuid import uuid4
db = database('sqlite.db')
hdrs = (Style('''
button,input { margin: 0 1rem; }
[role="group"] { border: 1px solid #ccc; }
.edited { outline: 2px solid orange; }
'''), )
app, rt = fast_app(hdrs=hdrs)
navbar = Nav()(Div(cls="container")(Div(cls="grid")(
H1("FastHTML Gallery"),
Div(cls="grid", style="justify-content: end;")(
A("Back to Gallery", cls="outline", href="/", role="button"),
A("Info", cls="secondary", href="/start_simple/csv_editor/info", role="button"),
A("Code", href="/start_simple/csv_editor/code", role="button")))))
@rt("/get_test_file")
async def get_test_file():
import httpx
url = "https://raw.githubusercontent.com/AnswerDotAI/FastHTML-Gallery/main/applications/start_simple/csv_editor/ex_data.csv"
response = await httpx.AsyncClient().get(url)
return Response(response.text, media_type="text/csv",
headers={'Content-Disposition': 'attachment; filename="ex_data.csv"'})
@app.get("/")
def homepage(sess):
if 'id' not in sess: sess['id'] = str(uuid4())
cts = Titled("CSV Uploader",
A('Download Example CSV', href="get_test_file", download="ex_data.csv"),
Group(Input(type="file", name="csv_file", accept=".csv"),
Button("Upload", hx_post="upload", hx_target="#results",
hx_encoding="multipart/form-data", hx_include='previous input'),
A('Download', href='download', type="button")),
Div(id="results"))
return navbar,cts
def render_row(row):
vals = [Td(Input(value=v, name=k, oninput="this.classList.add('edited')")) for k,v in row.items()]
vals.append(Td(Group(Button('delete', hx_delete=remove.rt(id=row['id']).lstrip('/')),
Button('update', hx_post='update', hx_include="closest tr"))))
return Tr(*vals, hx_target='closest tr', hx_swap='outerHTML')
@rt
def download(sess):
tbl = db[sess['id']]
csv_data = [",".join(map(str, tbl.columns_dict))]
csv_data += [",".join(map(str, row.values())) for row in tbl()]
headers = {'Content-Disposition': 'attachment; filename="data.csv"'}
return Response("\n".join(csv_data), media_type="text/csv", headers=headers)
@rt('/update')
def post(d:dict, sess): return render_row(db[sess['id']].update(d))
@app.delete('/remove')
def remove(id:int, sess): db[sess['id']].delete(id)
@rt("/upload")
def post(csv_file: UploadFile, sess):
db[sess['id']].drop(ignore=True)
if not csv_file.filename.endswith('.csv'): return "Please upload a CSV file"
content = b''
for i, line in enumerate(csv_file.file):
if i >= 51: break
content += line
tbl = db.import_file(sess['id'], content, pk='id')
header = Tr(*map(Th, tbl.columns_dict))
vals = [render_row(row) for row in tbl()]
return (Span('First 50 rows only', style="color: red;") if i>=51 else '', Table(Thead(header), Tbody(*vals)))
serve()
| py |
FastHTML Gallery | applications/start_simple/sqlite_todo/app.py | from fasthtml.common import *
from fastsql import *
from sqlite_minutils.db import NotFoundError
app,rt,todos,Todo = fast_app(
'data/todos.db',
id=int, title=str, pk='id')
def tid(id): return f'todo-{id}'
@app.delete("/delete_todo",name='delete_todo')
async def delete_todo(id:int):
try: todos.delete(id)
except NotFoundError: pass # If someone else deleted it already we don't have to do anything
@patch
def __ft__(self:Todo):
show = Strong(self.title, target_id='current-todo')
delete = A('delete',
hx_delete=delete_todo.rt(id=self.id).lstrip('/'),
hx_target=f'#{tid(self.id)}',
hx_swap='outerHTML')
return Li(show, ' | ', delete, id=tid(self.id))
def mk_input(**kw): return Input(id="new-title", name="title", placeholder="New Todo", **kw)
nav = Nav()(
Div(cls="container")(
Div(cls="grid")(
H1("FastHTML Gallery"),
Div(cls="grid")(
A("Back to Gallery", cls="outline", href="/", role="button" ),
A("Info", cls="secondary", href="/start_simple/sqlite_todo/info", role="button"),
A("Code", href="/start_simple/sqlite_todo/code", role="button")))))
@app.get("/")
async def homepage():
add = Form(Group(mk_input(), Button("Add")),
post="insert_todo", target_id='todo-list', hx_swap="beforeend")
card = Card(Ul(*todos(), id='todo-list'), header=add, footer=Div(id='current-todo')),
title = 'Todo list'
return Title(title), Main(nav, H1(title), card, cls='container')
@rt("/",name='insert_todo')
async def post(todo:Todo): return todos.insert(todo), mk_input(hx_swap_oob='true')
| py |
FastHTML Gallery | examples/application_layout/bootstrap_collapsable_sidebar_1/app.py | from fasthtml.common import *
from collections import namedtuple
cdn = 'https://cdn.jsdelivr.net/npm/bootstrap'
bootstrap_links = [
Link(href=cdn+"@5.3.3/dist/css/bootstrap.min.css", rel="stylesheet"),
Script(src=cdn+"@5.3.3/dist/js/bootstrap.bundle.min.js"),
Link(href=cdn+"[email protected]/font/bootstrap-icons.min.css", rel="stylesheet")
]
app, rt = fast_app(hdrs=bootstrap_links)
def SidebarItem(text, hx_get, hx_target, **kwargs):
return Div(
I(cls=f'bi bi-{text}'),
Span(text),
hx_get=hx_get, hx_target=hx_target,
data_bs_parent='#sidebar', role='button',
cls='list-group-item border-end-0 d-inline-block text-truncate',
**kwargs)
def Sidebar(sidebar_items, hx_get, hx_target):
return Div(
Div(*(SidebarItem(o, f"{hx_get}?menu={o}", hx_target) for o in sidebar_items),
id='sidebar-nav',
cls='list-group border-0 rounded-0 text-sm-start min-vh-100'
),
id='sidebar',
cls='collapse collapse-horizontal show border-end')
sidebar_items = ('Film', 'Heart', 'Bricks', 'Clock', 'Archive', 'Gear', 'Calendar', 'Envelope')
@app.get('/')
def homepage():
return Div(
Div(
Div(
Sidebar(sidebar_items, hx_get='menucontent', hx_target='#current-menu-content'),
cls='col-auto px-0'),
Main(
A(I(cls='bi bi-list bi-lg py-2 p-1'), 'Menu',
href='#', data_bs_target='#sidebar', data_bs_toggle='collapse', aria_expanded='false', aria_controls='sidebar',
cls='border rounded-3 p-1 text-decoration-none'),
Div(
Div(
Div(
H1("Click a sidebar menu item"),
P("They each have their own content"),
id="current-menu-content"),
cls='col-12'
), cls='row'),
cls='col ps-md-2 pt-2'),
cls='row flex-nowrap'),
cls='container-fluid')
@app.get('/menucontent')
def menucontent(menu: str):
return Div(
H1(f"{menu} Content"),
P(f"This is the content for the {menu} menu item."),
id="current-menu-content")
| py |
FastHTML Gallery | examples/application_layout/two_column_grid/app.py | from fasthtml.common import *
app, rt = fast_app()
@app.get('/')
def homepage():
return Titled('Try editing fields:', Grid(
Form(post="submit", hx_target="#result", hx_trigger="input delay:200ms")(
Select(Option("One"), Option("Two"), id="select"),
Input(value='j', id="name", placeholder="Name"),
Input(value='h', id="email", placeholder="Email")),
Div(id="result")
))
@app.post('/submit')
def submit(d:dict):
return Div(*[Div(P(Strong(k),': ',v)) for k,v in d.items()])
| py |
FastHTML Gallery | examples/dynamic_user_interface/cascading_dropdowns/app.py | from fasthtml.common import *
app, rt = fast_app()
chapters = ['ch1', 'ch2', 'ch3']
lessons = {
'ch1': ['lesson1', 'lesson2', 'lesson3'],
'ch2': ['lesson4', 'lesson5', 'lesson6'],
'ch3': ['lesson7', 'lesson8', 'lesson9']
}
def mk_opts(nm, cs):
return (
Option(f'-- select {nm} --', disabled='', selected='', value=''),
*map(Option, cs))
@app.get('/get_lessons')
def get_lessons(chapter: str):
return Select(*mk_opts('lesson', lessons[chapter]), name='lesson')
@app.get('/')
def homepage():
chapter_dropdown = Select(
*mk_opts('chapter', chapters),
name='chapter',
get='get_lessons', hx_target='#lessons')
return Div(
Div(
Label("Chapter:", for_="chapter"),
chapter_dropdown),
Div(
Label("Lesson:", for_="lesson"),
Div(Div(id='lessons')),
))
| py |
FastHTML Gallery | examples/dynamic_user_interface/click_to_edit/app.py | from fasthtml.common import *
app, rt = fast_app()
flds = dict(firstName='First Name', lastName='Last Name', email='Email')
@dataclass
class Contact:
firstName:str; lastName:str; email:str; edit:bool=False
def __ft__(self):
def item(k, v):
val = getattr(self,v)
return Div(Label(Strong(k), val), Hidden(val, id=v))
return Form(
*(item(v,k) for k,v in flds.items()),
Button('Click To Edit', cls='btn btn-primary'),
post='form', hx_swap='outerHTML')
contacts = [Contact('Joe', 'Blow', '[email protected]')]
@app.get('/')
def homepage(): return contacts[0]
@app.post('/form', name='form')
def form(c:Contact):
def item(k,v): return Div(Label(k), Input(name=v, value=getattr(c,v)))
return Form(
*(item(v,k) for k,v in flds.items()),
Button('Submit', cls='btn btn-primary', name='btn', value='submit'),
Button('Cancel', cls='btn btn-secondary', name='btn', value='cancel'),
post="contact", hx_swap='outerHTML'
)
@app.post('/contact', name='contact')
def contact(c:Contact, btn:str):
if btn=='submit': contacts[0] = c
return contacts[0]
| py |
FastHTML Gallery | examples/dynamic_user_interface/infinite_scroll/app.py | from fasthtml.common import *
import uuid
column_names = ('name', 'email', 'id')
def generate_contact(id: int) -> Dict[str, str]:
return {'name': 'Agent Smith',
'email': f'void{str(id)}@matrix.com',
'id': str(uuid.uuid4())
}
def generate_table_row(row_num: int) -> Tr:
contact = generate_contact(row_num)
return Tr(*[Td(contact[key]) for key in column_names])
def generate_table_part(part_num: int = 1, size: int = 20) -> Tuple[Tr]:
paginated = [generate_table_row((part_num - 1) * size + i) for i in range(size)]
paginated[-1].attrs.update({
'get': f'page?idx={part_num + 1}',
'hx-trigger': 'revealed',
'hx-swap': 'afterend'})
return tuple(paginated)
app, rt = fast_app(hdrs=(picolink))
app.get("/")
def homepage():
return Titled('Infinite Scroll',
Div(Table(
Thead(Tr(*[Th(key) for key in column_names])),
Tbody(generate_table_part(1)))))
@rt("/page/", name="page")
def get(idx:int|None = 0):
return generate_table_part(idx)
| py |
FastHTML Gallery | examples/dynamic_user_interface/inline_validation/app.py | from fasthtml.common import *
import re
################
### FastHTML ###
################
app, rt = fast_app()
@app.get('/')
def homepage():
return Form(post='submit', hx_target='#submit-btn-container', hx_swap='outerHTML')(
# Calls /email route to validate email
Div(hx_target='this', hx_swap='outerHTML')(
Label(_for='email')('Email Address'),
Input(type='text', name='email', id='email', post='email')),
# Calls /cool route to validate cool
Div(hx_target='this', hx_swap='outerHTML')(
Label(_for='cool')('Is this cool?'),
Input(type='text', name='cool', id='cool', post='cool')),
# Calls /coolscale route to validate coolscale
Div(hx_target='this', hx_swap='outerHTML')(
Label(_for='CoolScale')('How cool (scale of 1 - 10)?'),
Input(type='number', name='CoolScale', id='CoolScale', post='coolscale')),
# Submits the form which calls /submit route to validate whole form
Div(id='submit-btn-container')(
Button(type='submit', id='submit-btn',)('Submit')))
### Field Validation Routing ###
# Validates the field and generates FastHTML with appropriate validation and template function
@app.post('/email')
def email(email: str): return inputTemplate('Email Address', 'email', email, validate_email(email))
@app.post('/cool')
def cool(cool: str): return inputTemplate('Is this cool?', 'cool', cool, validate_cool(cool))
@app.post('/coolscale')
def coolscale(CoolScale: int): return inputTemplate('How cool (scale of 1 - 10)?', 'CoolScale', CoolScale, validate_coolscale(CoolScale), input_type='number')
@app.post('/submit')
def submit(email: str, cool: str, CoolScale: int):
# Validates all fields in the form
errors = {'email': validate_email(email),
'cool': validate_cool(cool),
'coolscale': validate_coolscale(CoolScale) }
# Removes the None values from the errors dictionary (No errors)
errors = {k: v for k, v in errors.items() if v is not None}
# Return Button with error message if they exist
return Div(id='submit-btn-container')(
Button(type='submit', id='submit-btn', post='submit', hx_target='#submit-btn-container', hx_swap='outerHTML')('Submit'),
*[Div(error, style='color: red;') for error in errors.values()])
########################
### Validation Logic ###
########################
def validate_email(email: str):
# Check if email address is a valid one
email_regex = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
if not re.match(email_regex, email): return "Please enter a valid email address"
# Check if email address is already taken (in this case only [email protected] will pass)
elif email != "[email protected]": return "That email is already taken. Please enter another email (only [email protected] will pass)."
# If no errors, return None (default of python)
def validate_cool(cool: str):
if cool.lower() not in ["yes", "definitely"]: return "Yes or definitely are the only correct answers"
def validate_coolscale(CoolScale: int):
if CoolScale < 1 or CoolScale > 10: return "Please enter a number between 1 and 10"
######################
### HTML Templates ###
######################
def inputTemplate(label, name, val, errorMsg=None, input_type='text'):
# Generic template for replacing the input field and showing the validation message
return Div(hx_target='this', hx_swap='outerHTML', cls=f"{errorMsg if errorMsg else 'Valid'}")(
Label(label), # Creates label for the input field
Input(name=name,type=input_type,value=f'{val}',post=f'{name.lower()}'), # Creates input field
Div(f'{errorMsg}', style='color: red;') if errorMsg else None) # Creates red error message below if there is an error
| py |
FastHTML Gallery | examples/dynamic_user_interface/web_sockets/app.py | from fasthtml.common import *
from collections import deque
app = FastHTML(ws_hdr=True)
# All messages here, but only most recent 15 are stored
messages = deque(maxlen=15)
users = {}
# Takes all the messages and renders them
box_style = "border: 1px solid #ccc; border-radius: 10px; padding: 10px; margin: 5px 0;"
def render_messages(messages):
return Div(*[Div(m, style=box_style) for m in messages], id='msg-list')
# Input field is reset via hx_swap_oob after submitting a message
def mk_input(): return Input(id='msg', placeholder="Type your message", value="", hx_swap_oob="true")
@app.get('/')
def homepage():
return Titled("Leave a message for others!"),Div(
Form(mk_input(), ws_send=True), # input field
Div(P("Leave a Message for others to see what they left for you!"),id='msg-list'), # All the Messages
hx_ext='ws', ws_connect='ws') # Use a web socket
def on_connect(ws, send): users[id(ws)] = send
def on_disconnect(ws):users.pop(id(ws),None)
@app.ws('/ws', conn=on_connect, disconn=on_disconnect)
async def ws(msg:str,send):
await send(mk_input()) # reset the input field immediately
messages.appendleft(msg) # New messages first
for u in users.values(): # Get `send` function for a user
await u(render_messages(messages)) # Send the message to that user
serve() | py |
FastHTML Gallery | examples/vizualizations/altair_charts/app.py | from fh_altair import altair2fasthml, altair_headers
from fasthtml.common import *
import numpy as np
import pandas as pd
import altair as alt
app, rt = fast_app(hdrs=altair_headers)
count = 0
plotdata = []
def generate_chart():
global plotdata
if len(plotdata) > 250:
plotdata = plotdata[-250:]
pltr = pd.DataFrame({'y': plotdata, 'x': range(len(plotdata))})
chart = alt.Chart(pltr).mark_line().encode(x='x', y='y').properties(width=400, height=200)
return altair2fasthml(chart)
@app.get("/")
def homepage():
return Title("Altair Demo"), Main(
H1("Altair Demo"),
Div(id="chart"),
Button("Increment", get=increment, hx_target="#chart", hx_swap="innerHTML"),
style="margin: 20px"
)
@app.get("/increment")
def increment():
global plotdata, count
count += 1
plotdata.append(np.random.exponential(1))
return Div(
generate_chart(),
P(f"You have pressed the button {count} times."),
)
| py |
FastHTML Gallery | examples/vizualizations/bloch_sphere/app.py | import numpy as np
from fasthtml.common import *
import plotly.graph_objects as go
from fh_plotly import plotly2fasthtml, plotly_headers
########################
### FastHTML Section ###
########################
app, rt = fast_app(hdrs=plotly_headers)
@app.get('/')
def homepage():
desc = """
The Bloch Sphere is a 3D visualization of a single quantum state.
You can interact with the buttons (Gates) to see how the state changes. See the description below for more information on what each gate represents.
"""
hx_vals = 'js:{"gates": document.getElementById("quantum_circuit").textContent}'
return (Title("Interactive Bloch Sphere"),
Main(P(desc),
*[Button(gate, hx_get=f"/vizualizations/bloch_sphere/apply_gate/{gate}", hx_target="#chart", hx_swap="innerHTML", hx_vals=hx_vals, title=f"Apply {gate} gate") for gate in single_qubit_gates.keys()],
Button("Reset", hx_get="/vizualizations/bloch_sphere/reset", hx_target="#chart", hx_swap="innerHTML", title="Reset the circuit"),
Div(update_state_apply_gate.__wrapped__(), id="chart"),
H4("Available gates"),
Ul(Li(Strong("H :"),"Hadamard gate. Puts the state in superposition. "),
Li(Strong("X :"),"Pauli-X (NOT) gate. Rotate 180 degrees around the X-Axis."),
Li(Strong("Y :"),"Pauli-Y (\"bit-flip\") gate. Rotate 180 degrees around the Y-Axis."),
Li(Strong("Z :"),"Pauli-Z (\"phase-flip\") gate. Rotate 180 degrees around the Z-Axis."),
Li(Strong("S :"),"Phase gate. Rotates around the Z-axis by 90 degrees."),
Li(Strong("T :"),"π/8 gate. Rotates around the Z-axis by 45 degrees."))))
@app.get('/reset')
def reset(): return update_state_apply_gate.__wrapped__()
@app.get('/apply_gate/{gate}')
def update_state_apply_gate(gate: str=None, gates: str=None):
if gates is None: gates = []
else:
# Transform from circuit representation to gate names
gates = [g for g in gates if g in single_qubit_gates.keys()]
gates.append(gate)
# Create initial state
state = np.array([1, 0]) # |0> basis state
for gate in gates: state = single_qubit_gates[gate] @ state
# Create visualization
return Div(plot_bloch(state),
H4(f"Quantum Circuit: {visualize_circuit(gates)}", id="quantum_circuit"),
id="chart")
def visualize_circuit(gates: list[str]):
circuit = "|0⟩-"
circuit += "".join([f"[{gate}]─" for gate in gates]) + "|"
return circuit
############################
### Math/Quantum Section ###
############################
def calculate_coordinates(theta, phi):
x = np.sin(theta) * np.cos(phi)
y = np.sin(theta) * np.sin(phi)
z = np.cos(theta)
return x, y, z
def create_scenes():
axis2ticktext = {'X': ['|-⟩', '|+⟩'], 'Y': ['|-i⟩', '|i⟩'], 'Z': ['|1⟩', '|0⟩']}
scenes = {}
for axis in ['X','Y','Z']:
scenes[f'{axis.lower()}axis'] = dict(title=dict(text=axis, font=dict(size=25)),
range=[-1.2, 1.2], tickvals=[-1, 1],
ticktext=axis2ticktext[axis],
tickfont=dict(size=15) )
return scenes
def plot_bloch(state: np.array):
fig = go.Figure()
# State vector coordinates
alpha, beta = state[0], state[1]
theta = 2 * np.arccos(np.abs(alpha))
phi = np.angle(beta) - np.angle(alpha)
x, y, z = calculate_coordinates(theta, phi)
# Surface coordinates
surface_phi, surface_theta = np.mgrid[0:2*np.pi:100j, 0:np.pi:50j]
xs, ys, zs = calculate_coordinates(surface_theta, surface_phi)
fig.add_trace(go.Surface(x=xs, y=ys, z=zs, opacity=0.5, colorscale='Blues', showscale=False))
fig.add_trace(go.Scatter3d(x=[0, x],y=[0, y],z=[0, z], mode='lines+markers+text', marker=dict(size=10, color='green'),
line=dict(color='green', width=8), textposition="top center", showlegend=True,name=f"{alpha:.2f}|0⟩ + {beta:.2f}|1⟩"))
# Mark basis states
fig.add_trace(go.Scatter3d(x=[0, 0, 1, -1, 0, 0],y=[0, 0, 0, 0, 1, -1], z=[1, -1, 0, 0, 0, 0],
mode='markers', marker=dict(size=5, color='black'), hovertext=['|0⟩', '|1⟩', '|+⟩', '|-⟩', '|i⟩', '|-i⟩'],
showlegend=False, name="Basis states"))
# Add lines across axes
boundary_phi = np.linspace(0, 2 * np.pi, 100)
coords = [(np.cos(boundary_phi), np.sin(boundary_phi), np.zeros_like(boundary_phi)),
(np.zeros_like(boundary_phi), np.cos(boundary_phi), np.sin(boundary_phi)),
(np.cos(boundary_phi), np.zeros_like(boundary_phi), np.sin(boundary_phi)) ]
for x, y, z in coords:
fig.add_trace(go.Scatter3d(x=x, y=y, z=z, mode='lines', line=dict(color='black', width=2), showlegend=False, name="Axes"))
fig.update_layout(scene=dict(**create_scenes(), aspectmode='cube',),
legend=dict( font=dict(size=20), x=0.05,y=0.95, xanchor='left', yanchor='top', bgcolor='rgba(0,0,0,0)',),
margin=dict(l=0, r=0, t=0, b=0))
return plotly2fasthtml(fig)
single_qubit_gates = {
# Hadamard
"H": np.array([[1, 1],
[1, -1]]) / np.sqrt(2),
# Pauli matrices
"X": np.array([[0, 1],
[1, 0]]),
"Y": np.array([[0, -1j],
[1j, 0]]),
"Z": np.array([[1, 0],
[0, -1]]),
# Phase gates
"S": np.array([[1, 0],
[0, 1j]]),
"T": np.array([[1, 0],
[0, np.exp(1j * np.pi / 4)]])
}
| py |
FastHTML Gallery | examples/vizualizations/great_tables_tables/app.py | from functools import cache
import polars as pl
from great_tables import GT, html
from great_tables.data import sza
from fasthtml.common import *
app, rt = fast_app()
@cache
def get_sza_pivot():
# Filter and pivot the data
return (pl.from_pandas(sza)
.filter((pl.col("latitude") == "20") & (pl.col("tst") <= "1200"))
.select(pl.col("*").exclude("latitude"))
.drop_nulls()
.pivot(values="sza", index="month", on="tst", sort_columns=True))
def get_notstr_table(color1: str = "#663399", color2: str = "#FFA500"):
# Create the table
sza_gt = (GT(get_sza_pivot(), rowname_col="month")
.data_color(
domain=[90, 0],
palette=[color1, "white", color2],
na_color="white",)
.tab_header(
title="Solar Zenith Angles from 05:30 to 12:00",
subtitle=html("Average monthly values at latitude of 20°N."))
.sub_missing(missing_text=""))
# Return the table by converting to raw HTML
return Div(NotStr(sza_gt.as_raw_html()))
@app.post("/submit", name="submit")
def post(d: dict):
return get_notstr_table(**d)
@app.get("/")
def homepage():
return (Title("FastHTML-GT Website"),
Titled("Great Tables shown in FastHTML", style="text-align:center"),
Main(cls='container')(
Form(post="submit", hx_target="#gt", hx_trigger="input")(
Grid(Card(H2("Color1"), Input(type="color",id="color1", value="#663399")),
Card(H2("Color2"), Input(type="color",id="color2", value="#FFA500")))),
Div(get_notstr_table(), id="gt")))
| py |
FastHTML Gallery | examples/vizualizations/matplotlib_charts/app.py | from fh_matplotlib import matplotlib2fasthtml
from fasthtml.common import *
import numpy as np
import matplotlib.pylab as plt
app, rt = fast_app()
@matplotlib2fasthtml
def generate_chart(num_points):
plotdata = [np.random.exponential(1) for _ in range(num_points)]
plt.plot(range(len(plotdata)), plotdata)
@app.get("/")
def homepage():
return Div(
Div(id="chart"),
H3("Move the slider to change the graph"),
Input(
type="range",
min="1", max="10", value="1",
get=update_chart, hx_target="#chart",
name='slider')
)
@app.get("/update_charts")
def update_chart(slider: int):
return Div(
generate_chart(slider),
P(f"Number of data points: {slider}")
)
| py |
FastHTML Gallery | examples/vizualizations/plotly_charts/app.py | from fasthtml.common import *
import pandas as pd
import numpy as np
import plotly.express as px
from fh_plotly import plotly2fasthtml, plotly_headers
app, rt = fast_app(hdrs=plotly_headers)
y_data = [1, 2, 3, 2]
x_data = [3, 1, 2, 4]
def generate_line_chart():
df = pd.DataFrame({'y': y_data, 'x': x_data})
fig = px.line(df, x='x', y='y')
return plotly2fasthtml(fig)
def generate_bar_chart():
df = pd.DataFrame({'y': y_data, 'x': ['A', 'B', 'C','D']})
fig = px.bar(df, x='x', y='y')
return plotly2fasthtml(fig)
def generate_scatter_chart():
df = pd.DataFrame({'y': y_data, 'x': x_data, 'size': [10, 20, 30, 40]})
fig = px.scatter(df, x='x', y='y', size='size')
return plotly2fasthtml(fig)
def generate_3d_scatter_chart():
df = pd.DataFrame({
'x': [1, 2, 3, 4, 5, 6],
'y': [7, 8, 6, 9, 7, 8],
'z': [3, 5, 4, 6, 5, 7]
})
fig = px.scatter_3d(df, x='x', y='y', z='z')
return plotly2fasthtml(fig)
@app.get('/')
def homepage():
return Div(
H1("Plotly Interactive Charts Demo with FastHTML"),
P("Try interacting with the charts by hovering over data points, zooming in and out, panning, rotating (3D), and more!."),
Div(Div(Strong("Plot 1: Line Chart"),
Div(generate_line_chart()),),
Div(Strong("Plot 2: Bar Chart"),
Div(generate_bar_chart()),),
Div(Strong("Plot 3: Scatter Chart"),
Div(generate_scatter_chart()),),
Div(Strong("Plot 4: 3D Scatter Chart"),
Div(generate_3d_scatter_chart()),),
style="display: grid; grid-template-columns: 1fr 1fr; grid-template-rows: 1fr 1fr; gap: 20px; width: 100%; height: 800px;"
)
)
serve() | py |
FastHTML Gallery | examples/vizualizations/seaborn_svg/app.py | from fasthtml.common import *
import numpy as np, seaborn as sns, matplotlib.pylab as plt
app,rt = fast_app()
data = np.random.rand(4,10)
def fh_svg(func):
"show svg in fasthtml decorator"
def wrapper(*args, **kwargs):
func(*args, **kwargs) # calls plotting function
f = io.StringIO() # create a buffer to store svg data
plt.savefig(f, format='svg', bbox_inches='tight')
f.seek(0) # beginning of file
svg_data = f.getvalue()
plt.close()
return NotStr(svg_data)
return wrapper
@fh_svg
def plot_heatmap(matrix,figsize=(6,7),**kwargs):
plt.figure(figsize=figsize)
sns.heatmap(matrix, cmap='coolwarm', annot=False,**kwargs)
@app.get("/")
def homepage():
return Div(Label(H3("Heatmap Columns"), _for='n_cols'),
Input(type="range", min="1", max="10", value="1",
get=update_heatmap, hx_target="#plot", id='n_cols'),
Div(id="plot"))
@app.get("/update_charts")
def update_heatmap(n_cols:int):
svg_plot = plot_heatmap(data[:,:n_cols])
return svg_plot
serve()
| py |
FastHTML Gallery | examples/widgets/audio/app.py | import base64, requests
from fasthtml.common import *
app, rt = fast_app()
@app.get('/')
def homepage():
audio_path = "https://ucarecdn.com/abb35276-b3cb-4a5c-bba0-878f264e5976/AfricanFellaCumbiaDelishort.mp3"
return Audio(src=f"data:audio/mp4;base64,{load_audio_base64(audio_path)}", controls=True)
def load_audio_base64(audio_path: str):
""" Convert audio file to base64. """
response = requests.get(audio_path)
response.raise_for_status()
return base64.b64encode(response.content).decode('ascii')
| py |
FastHTML Gallery | examples/widgets/chat_bubble/app.py | from fasthtml.common import *
app, rt = fast_app()
@app.get('/')
def homepage():
return Div(*[create_chat_message(**msg, msg_num=i) for i, msg in enumerate(example_messages)])
def create_chat_message(role, content, msg_num):
if role == 'system': color = '#8B5CF6'
elif role == 'user': color = "#F000B8"
else: color = "#37CDBE"
text_color = '#1F2937'
# msg 0 = left, msg 1 = right, msg 2 = left, etc.
alignment = 'flex-end' if msg_num % 2 == 1 else 'flex-start'
message = Div(Div(
Div(# Shows the Role
Strong(role.capitalize()),
style=f"color: {text_color}; font-size: 0.9em; letter-spacing: 0.05em;"),
Div(# Shows content and applies font color to stuff other than syntax highlighting
Style(f".marked *:not(code):not([class^='hljs']) {{ color: {text_color} !important; }}"),
Div(content),
style=f"margin-top: 0.5em; color: {text_color} !important;"),
# extra styling to make things look better
style=f"""
margin-bottom: 1em; padding: 1em; border-radius: 24px; background-color: {color};
max-width: 70%; position: relative; color: {text_color} !important; """),
style=f"display: flex; justify-content: {alignment};")
return message
example_messages = [
{
"role": "system",
"content": "Hello, world! I am a chatbot that can answer questions about the world.",
},
{
"role": "user",
"content": "I have always wondered why the sky is blue. Can you tell me?",
},
{
"role": "assistant",
"content": "The sky is blue because of the atmosphere. As white light passes through air molecules cause it to scatter. Because of the wavelengths, blue light is scattered the most.",
},
{
"role": "user",
"content": "What is the meaning of life?",
},
{
"role": "assistant",
"content": "42 is the meaning of life. It is the answer to the question of life, the universe, and everything.",
}
]
| py |
FastHTML Gallery | examples/widgets/pdf/app.py | from fasthtml.common import *
app, rt = fast_app()
@rt('/')
def homepage():
pdf_path = 'https://arxiv.org/pdf/1706.03762'
return Embed(src=pdf_path, type='application/pdf',
width='100%', height='1000px')
| py |
FastHTML Gallery | examples/widgets/progress_bar/app.py | from fasthtml.common import *
import random
app, rt = fast_app()
def get_progress(percent_complete: int):
# simulate progress check
percent_complete += random.random()/3
return percent_complete
@app.get('/')
def homepage():
return Div(H3("Start the job to see progress!"),id='progress_bar'),Button("Start Job",post='update_status', hx_target="#progress_bar",)
@app.post('/job')
def update_status():
# Start the job
return progress_bar(percent_complete=0)
@app.get('/job')
def update_progress(percent_complete: float):
# Check if done
if percent_complete >= 1: return H3("Job Complete!", id="progress_bar")
# get progress
percent_complete = get_progress(percent_complete)
# Update progress bar
return progress_bar(percent_complete)
def progress_bar(percent_complete: float):
return Progress(id="progress_bar",value=percent_complete,
get='update_progress',hx_target="#progress_bar",hx_trigger="every 500ms",
hx_vals=f"js:'percent_complete': '{percent_complete}'")
| py |
FastHTML Gallery | examples/widgets/show_hide/app.py | from fasthtml.common import *
app, rt = fast_app()
content = """Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed sit amet volutpat tellus, in tincidunt magna. Vivamus congue posuere ligula a cursus. Sed efficitur tortor quis nisi mollis, eu aliquet nunc malesuada. Nulla semper lacus lacus, non sollicitudin velit mollis nec. Phasellus pharetra lobortis nisi ac eleifend. Suspendisse commodo dolor vitae efficitur lobortis. Nulla a venenatis libero, a congue nibh. Fusce ac pretium orci, in vehicula lorem. Aenean lacus ipsum, molestie quis magna id, lacinia finibus neque. Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia curae; Interdum et malesuada fames ac ante ipsum primis in faucibus. Maecenas ac ex luctus, dictum erat ut, bibendum enim. Curabitur et est quis sapien consequat fringilla a sit amet purus."""
def mk_button(show):
return Button("Hide" if show else "Show",
get="toggle?show=" + ("False" if show else "True"),
hx_target="#content", id="toggle", hx_swap_oob="outerHTML")
@app.get('/')
def homepage():
return Div(mk_button(False), Div(id="content"))
@rt('/toggle', name='toggle')
def get(show: bool):
return Div(
Div(mk_button(show)),
Div(content if show else ''))
| py |